Activity 17: Data Structure Again | Aligan, Rhed N.
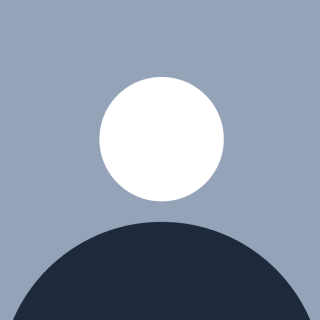
🪻 LIST, OBJECT, AND LIST OF OBJECTS 🪻
🌺 List
- List with data structure shows the collection of data or elements within the variable. Each value has a own index started in 0.
To simplified, the example analogy is in the house, there’s a standard one family, the parents is like the variable that holds of different value. The value is the number of siblings or number of childs they have. For instances, if parents have a first child, middle child, and last child. The value has a 3 with their own name. In LIST, we can access them through their indexes starting in 0. So sequentially, the 0 indexes are the first child born (Panganay), and the 2 indexes are last child born (Bunso). In this case, we help organized the collection of elements based on their variable that hold to them.
CONFUSE? : DIFFERENCE OF ARRAY AND LIST?
The difference of Array and List in data structure. Array and List are same of collection of method but there’s a catch and constraints each of them.
- The Array is can able to push and add more than value within array. But the one thing to take note that Array is DEFINED datatypes for example the code snippets below:
In addition, Array also has contraints that the size of elements defined. for example, the code below. It shows that the array has contraints of five (5) elements. So, in case they want to add that exceed of 5 elements, it will give an ExemptionArray that the adding elements was exceed in the parameters of array.
numberngpila: number[] = new Array(5)
Furthermore, below code snippet display the known elements with 0-3 indexes.
numberngpila: number[] = [1, 2, 3, 4]
This example of Array defined of Integers or Numbers you can do a string but, in my example, number are used. therefore, the value or collection of elements are only integers.
While List, are also collection of elements, but the difference is List can add different data types within the List such as INTEGERS and STRINGS, the behavior and characteristics of List are they allow duplicates elements inside. In addition, you don’t need to specify the variable data types since it can adapt the various data types. In this example
NameandAge = ['Rhed', 22, "Jonalyn", 20];
This syntax shows automatically without explicitly define the data types So, the data types will infer based on the value of List.
NameandAge: (string | number)[] = ["Rhed", 22, "Jonalyn", 20]; //EKIS TO || BAWAL TO GUYS! || INVALID OR EXEMPTIONERROR NameandAge: (string | number)[] = ["Rhed", 22, "Jonalyn", 20, true];
You can able specify the only data types need include collection of elements. This will help for safety typos. The second syntax are example also of specify a data types and the boolean value inside of list You can’t able input a boolean value. It cause in parameter error.
EXAMPLE OF LIST
//define as string student_name_list: string [] = ["Rhed Aligan", "Jonalyn Nebril", "Jepony Odong", "Zeph Chan"]; //automatically defined based on data types elements, but in this case, //section_list are only strings student_section_list = ["BSIT-4A","BSIT-4B", "SECTION-1", "SECTION-2"]; //define the list as number, it's an option sometimes need for specifying //and avoid typos mistake student_id_list:number[] = [1,2,3,4,5]; //automatically defined strings student_email_list = ["rhed123@gmail.com","jonalyn123@gmail.com", "aligannebril@gmail.com"];
GIVEN TABLE IN ACTIVITY USING LIST:
1. Product Table 🎯
| ID | Name | Category | Price | Stock | Supplier Email | | | --- | --- | --- | --- | --- | --- | --- | | 1 | Laptop | Electronics | 750 | 50 | supplier1@gmail.com | | | 2 | Desk Chair | Furniture | 100 | 200 | supplier2@gmail.com | | | 3 | Smartwatch | Electronics | 200 | 150 | supplier3@gmail.com | | | 4 | Notebook | Stationery | 5 | 500 | supplier4@gmail.com | | | 5 | Running Shoes | Apparel | 80 | 100 | supplier5@gmail.com | |
product_tbl_id: number[]=[1,2,3,4,5]; //specify data types for avoid typos product_tbl_name = ["Laptop", "Desk Chair", "SmartWatch", "Notebook", "Running Shoes"]; product_tbl_category = ["Electronics", "Furniture", "Electronics", "Stationery", "Apparel"]; product_tbl_price = [750, 100, 200, 5, 80]; product_tbl_stock = [50,200,150,500,100]; product_tbl_suppemail = ["supplier1@gmail.com", "supplier2@gmail.com", "supplier3@gmail.com", "supplier4@gmail.com", "supplier5@gmail.com"];
2. Employee Table 🎯
| ID | Name | Department | Age | Email | | --- | --- | --- | --- | --- | | 1 | John Doe | Sales | 30 | john.doe@company.com | | 2 | Jane Smith | Human Resources | 25 | jane.smith@company.com | | 3 | Mark Johnson | IT | 40 | mark.johnson@company.com | | 4 | Lisa Wong | Marketing | 28 | lisa.wong@company.com | | 5 | Paul McDonald | Finance | 35 | paul.mcdonald@company.com |
employee_tbl_id:number[] =[1,2,3,4,5]; employee_tbl_name = ["John Doe", "Jane Smith", "Mark Johnson","Lisa Wong", "Paul McDonald"]; employee_tbl_dept = ["Sales", "Human Resources", "IT", "Marketing", "Finance"]; //You can do a string this if your system is hasn't not use operation include age employee_tbl_age = [30,25, 40, 28, 35]; employee_tbl_email = ["john.doe@company.com","jane.smith@company.com","mark.johnson@company.com", "lisa.wong@company.com", "paul.mcdonald@company.com"];
3.Books Table 🎯
ID | Title | Author | Genre | Published Year | ISBN | Stock | Price | |
1 | The Great Gatsby | F. Scott Fitzgerald | Fiction | 1925 | 978-0743273565 | 20 | 15.99 | |
2 | To Kill a Mockingbird | Harper Lee | Fiction | 1960 | 978-0060935467 | 35 | 10.99 | |
3 | 1984 | George Orwell | Dystopian | 1949 | 978-0451524935 | 40 | 9.99 | |
4 | The Catcher in the Rye | J.D. Salinger | Fiction | 1951 | 978-0316769488 | 25 | 8.99 | |
5 | A Brief History of Time | Stephen Hawking | Non-fiction | 1988 | 978-0553380163 | 10 | 18.99 |
books_tbl_id:number[] =[1,2,3,4,5];
books_tbl_title = ["The Greate Gatsby", "To Kill a Mockingbird", "1984", "The Catcher in the Rye", "A Brief History of Time "];
books_tbl_author = ["F. Scott Fitzgerald", "Harper Lee", "George Orwell", "J.D Salinger", "Stephen Hawking"];
books_tbl_genre = ["Fiction", "Fiction", "Dystopian", "Fiction", "Non-Fiction"];
books_tbl_publishedyear = ["1925", "1960", "1949", "1951", "1988"];
books_tbl_ISBN = ["978-0743273565", "978-0060935467", "978-0451524935", "978-0316769488", "978-0553380163"];
books_tbl_stock = [20,35,40,25,10];
books_tbl_price = [15.99, 10.99, 9.99, 8.99, 18.99]
4.University Table 🎯
ID | Name | Location | Established Year | Type | Website |
1 | University of the Philippines | Quezon City | 1908 | Public | www.up.edu.ph |
2 | Ateneo de Manila University | Quezon City | 1859 | Private | www.ateneo.edu |
3 | De La Salle University | Manila | 1911 | Private | www.dlsu.edu.ph |
4 | University of Santo Tomas | Manila | 1611 | Private | www.ust.edu.ph |
5 | Polytechnic University of the Philippines | Manila | 1904 | Public | www.pup.edu.ph |
univ_tbl_id = [1,2,3,4,5];
univ_tbl_name = ["University of the Philippines", "Ateneo de Manila University", "De La Salle University", "University of Santo Tomas", "Polytechnic University of the Philippines"];
univ_tbl_location = ["Quezon City", "Quezon City", "Manila", "Manila", "Manila"];
univ_tbl_establishedYear = ["1908","1859", "1911", "1611", "1904"];
univ_tbl_type = ["Public", "Private", "Private", "Private", "Private", "Public"];
univ_tbl_website = ["www.up.edu.ph", "www.ateneo.edu", "www.dlsu.edu.ph", "www.ust.edu.ph", "www.pup.edu.ph"];
5.Restaurant Table 🎯
ID | Name | Location | Cuisine Type | Established Year | Website or Contact |
1 | Vikings Luxury Buffet | Pasay City | Buffet | 2011 | www.vikings.ph |
2 | Antonio's Restaurant | Tagaytay | Fine Dining | 2002 | www.antoniosrestaurant.ph |
3 | Mesa Filipino Moderne | Makati City | Filipino | 2009 | www.mesa.ph |
4 | Manam Comfort Filipino | Quezon City | Filipino | 2013 | www.manam.ph |
5 | Ramen Nagi | Various Locations | Japanese | 2013 | www.ramennagi.com.ph |
rest_tbl_id = [1,2,3,4,5];
rest_tbl_name = ["Vikings Luxury Buffer", "Antonio's Restaurant", "Mesa Filipino Moderne", "Manam Comfort Filipino", "Ramen Nagi"];
rest_tbl_location = ["Pasay City", "Tagaytay", "Makati City", "Quezon City", "Various Locations"];
rest_tbl_cuisineType = ["Buffet", "Fine Dining", "Filipino", "Filipino", "Japanese"];
rest_tbl_establishedYear = ["2011", "2002", "2009", "2013", "2013"];
rest_tbl_website = ["www.vikings.ph", "www.antoniosrestaurant.ph", "www.mesa.ph", "www.manam.ph", "wwww.ramennagi.com.ph"];
🌺 Object
Object with data structures is an instance of a class that encapsulates both data such as attributes and properties and behavior (methods or functions) that operate on that data. In addition, object is a collection of key-value pairs, where each key is a string, and each value can be of any data type.
Me = { name: 'Rhed Aligan', age: 22, email: 'rhed123@example.com' };
In this code snippets, it shows under the variable “Me” hold the 3 attributes and its properties.
Object Creation:
- The object
Me
is created using curly braces{}
. This syntax defines a new object in JavaScript.
- The object
Property Assignment:
Inside the curly braces, properties are defined in key-value pairs:
name: This key is associated with the value ‘Rhed Aligan‘, which is a string.
age: This key is associated with the value
22
, which is a number.email: This key is associated with the value ‘rhed123@gmail.com‘, which is a string.
Accessing Properties:
You can access the properties of the object using dot notation or bracket notation. For example:
Me.name would return “Rhed Aligan“.
Me.age would return 22.
Me[‘Email‘] would return ‘rhed123@gmail.com‘.
This object serves to organize and encapsulate related data about a person, making it easy to manage and retrieve information in a structured manner.
GIVEN TABLE IN ACTIVITY USING OBJECT
1. Product Table 🎯
ID | Name | Category | Price | Stock | Supplier Email |
1 | Laptop | Electronics | 750 | 50 | supplier1@gmail.com |
2 | Desk Chair | Furniture | 100 | 200 | supplier2@gmail.com |
3 | Smartwatch | Electronics | 200 | 150 | supplier3@gmail.com |
4 | Notebook | Stationery | 5 | 500 | supplier4@gmail.com |
5 | Running Shoes | Apparel | 80 | 100 | supplier5@gmail.com |
product_obj1 = {
"id": 1,
"name": "Laptop",
"category": "Electronics",
"price": 750,
"Stock": 50;
"SupplierEmail": "supplier1@gmail.com"
}
product_obj2 = {
"id": 2,
"name": "Desk Chair",
"category": "Furniture",
"price": 100,
"Stock": 200;
"SupplierEmail": "supplier2@gmail.com"
}
product_obj3 = {
"id": 3,
"name": "Smartwatch",
"category": "Electronics",
"price": 200,
"Stock": 150;
"SupplierEmail": "supplier3@gmail.com"
}
product_obj4 = {
"id": 4,
"name": "Notebook",
"category": "Stationery",
"price": 5,
"Stock": 500;
"SupplierEmail": "supplier4@gmail.com"
}
product_obj5 = {
"id": 5,
"name": "Running Shoes",
"category": "Apparel",
"price": 80,
"Stock": 100;
"SupplierEmail": "supplier5@gmail.com"
}
2. Employee Table 🎯
ID | Name | Department | Age | |
1 | John Doe | Sales | 30 | john.doe@company.com |
2 | Jane Smith | Human Resources | 25 | jane.smith@company.com |
3 | Mark Johnson | IT | 40 | mark.johnson@company.com |
4 | Lisa Wong | Marketing | 28 | lisa.wong@company.com |
5 | Paul McDonald | Finance | 35 | paul.mcdonald@company.com |
employee_ob1 = {
"id": 1,
"name":"John Doe",
"department":"Sales",
"age":30,
"email": "john.doe@company.com"
}
employee_ob2 = {
"id": 2,
"name":"Jane Smith",
"department":"Human Resources",
"age":25 ,
"email": "jane.smith@company.com"
}
employee_ob3 = {
"id": 3,
"name":"Mark Johnson",
"department":"IT",
"age":40,
"email": "mark.johnson@company.com"
}
employee_ob4 = {
"id": 4,
"name":"Lisa Wong",
"department":"Marketing",
"age":28,
"email": "lisa.wong@company.com"
}
employee_ob5 = {
"id": 5,
"name":"Paul McDonald",
"department":"Finance",
"age":35,
"email": "paul.mcdonald@company.com"
}
Books Table 🎯
ID | Title | Author | Genre | Published Year | ISBN | Stock | Price |
1 | The Great Gatsby | F. Scott Fitzgerald | Fiction | 1925 | 978-0743273565 | 20 | 15.99 |
2 | To Kill a Mockingbird | Harper Lee | Fiction | 1960 | 978-0060935467 | 35 | 10.99 |
3 | 1984 | George Orwell | Dystopian | 1949 | 978-0451524935 | 40 | 9.99 |
4 | The Catcher in the Rye | J.D. Salinger | Fiction | 1951 | 978-0316769488 | 25 | 8.99 |
5 | A Brief History of Time | Stephen Hawking | Non-fiction | 1988 | 978-0553380163 | 10 | 18.99 |
books_obj1 = {
"id": 1,
"title":"The Greate Gatsby",
"Author":"F. Scott Fitzgerald",
"Genre":"Fiction",
"PublishedYear":"1925",
"ISBN":"978-0743273565",
"Stock":20,
"Price":15.99
}
books_obj2 = {
"id": 2,
"title":"To Kill a Mockingbird",
"Author":"Harper Lee",
"Genre":"Fiction",
"PublishedYear":"1960",
"ISBN":"978-0060935467",
"Stock":35,
"Price":10.99
}
books_obj3 = {
"id": 3,
"title":"1984",
"Author":"George Orwell",
"Genre":"Dystopian",
"PublishedYear":"1949",
"ISBN":"978-0451524935",
"Stock":40,
"Price":9.99
}
books_ob4 = {
"id": 4,
"title":"The Catcher in the Rye",
"Author":"J.D Salinger",
"Genre":"Fiction",
"PublishedYear":"1951",
"ISBN":"978-0316769488",
"Stock":25,
"Price": 8.99
}
books_ob5 = {
"id": 5,
"title":"A Brief History of Time",
"Author":"Stephen Hawking",
"Genre":"Non-Fiction",
"PublishedYear":"1988",
"ISBN":"978-0553380163",
"Stock":10,
"Price": 18.99
}
University Table 🎯
ID | Name | Location | Established Year | Type | Website |
1 | University of the Philippines | Quezon City | 1908 | Public | www.up.edu.ph |
2 | Ateneo de Manila University | Quezon City | 1859 | Private | www.ateneo.edu |
3 | De La Salle University | Manila | 1911 | Private | www.dlsu.edu.ph |
4 | University of Santo Tomas | Manila | 1611 | Private | www.ust.edu.ph |
5 | Polytechnic University of the Philippines | Manila | 1904 | Public | www.pup.edu.ph |
university_obj1 = {
"id":1,
"name":"University of the Philippines",
"location":"Quezon City",
"EstablishedYear": "1908",
"type":"Public"
"website": "www.up.edu.ph"
}
university_obj2 = {
"id":2,
"name":"Ateneo de Manila University",
"location":"Quezon City",
"EstablishedYear": "1859",
"type":"Private"
"website": "www.ateneo.edu.ph"
}
university_obj3 = {
"id":3,
"name":"De La Salle University",
"location":"Manila",
"EstablishedYear": "1911",
"type":"Private"
"website": "www.dlsu.edu.ph"
}
university_obj4 = {
"id":4,
"name":"University of Santo Tomas",
"location":"Manila",
"EstablishedYear": "1611",
"type":"Private"
"website": "www.ust.edu.ph"
}
university_obj5 = {
"id":5,
"name":"Polytechnic University of the Philippines",
"location":"Manila",
"EstablishedYear": "1904",
"type":"Public"
"website": "www.pup.edu.ph"
}
Restaurant Table 🎯
ID | Name | Location | Cuisine Type | Established Year | Website or Contact |
1 | Vikings Luxury Buffet | Pasay City | Buffet | 2011 | www.vikings.ph |
2 | Antonio's Restaurant | Tagaytay | Fine Dining | 2002 | www.antoniosrestaurant.ph |
3 | Mesa Filipino Moderne | Makati City | Filipino | 2009 | www.mesa.ph |
4 | Manam Comfort Filipino | Quezon City | Filipino | 2013 | www.manam.ph |
5 | Ramen Nagi | Various Locations | Japanese | 2013 | www.ramennagi.com.ph |
restaurant_obj1 {
"id":1,
"name":"Vikings Luxury Buffet",
"Location":"Pasay City",
"CuisineType":"Buffet",
"EstablishedYear": "2011",
"website":"www.vikings.ph"
}
restaurant_obj2 {
"id":2,
"name":"Antonio's Restaurant",
"Location":"Tagaytay",
"CuisineType":"Fine Dining",
"EstablishedYear": "2002",
"website":"www.antoniosrestaurant.ph"
}
restaurant_obj3 {
"id":3,
"name":"Mesa Filipino Moderne",
"Location":"Makati City",
"CuisineType":"Filipino",
"EstablishedYear": "2009",
"website":"www.mesa.ph"
}
restaurant_obj4 {
"id":4,
"name":"Manam Comfort Filipino",
"Location":"Quezon City",
"CuisineType":"Filipino",
"EstablishedYear": "2013",
"website":"www.manam.ph"
}
restaurant_obj5 {
"id":5,
"name":"Ramen Nagi",
"Location":"Various Locations",
"CuisineType":"Japanese",
"EstablishedYear": "2013",
"website":"www.ramennagi.ph"
}
🌺 List of Objects
List of Objects is a collection where each element is an object. It combines the concepts of a list (which is an ordered collection of elements) and an object (a structure that contains data in the form of attributes or properties).
We will breakdown the characteristics of List of Object and its advantage.
Ordered: The objects in the list are arranged in a specific order (index-based), meaning you can access each object using its position (index).
Heterogeneous/Inheritance: The objects in the list can belong to the same class/type or different classes/types.
Dynamic Size: Many list implementations (e.g., arrays or linked lists) can dynamically grow as objects are added.
Object-Oriented: Each element in the list is a fully-fledged object, meaning it has its own methods and attributes.
MyConnections = { "name": "Jason Galicia", "age":22, "location":"Camarin Caloocan City", "relationship": "friends" }, { "name": "Jonalyn Nebril", "age":20, "location":"Hillcrest Caloocan City", "relationship": "Partner" } { "name": "Ivan Aligan", "age":24, "location":"Caloocan City", "relationship": "Brother" } { "name": "Ayiesha Aligan", "age":14, "location":"Caloocan City", "relationship": "Younger Sister" }
In this example, it shows a variable or the parent child named “MyConnections”. Getting of their indexes to get a specific object wherein it includes the attributes and a properties, The list allows you to store multiple connections in one collection, so you can easily retrieve information about any connection by simply accessing the list. For example, you can loop through the list and print out names or details.
GIVEN TABLE IN ACTIVITY USING OBJECT
1. Product Table 🎯
ID | Name | Category | Price | Stock | Supplier Email |
1 | Laptop | Electronics | 750 | 50 | supplier1@gmail.com |
2 | Desk Chair | Furniture | 100 | 200 | supplier2@gmail.com |
3 | Smartwatch | Electronics | 200 | 150 | supplier3@gmail.com |
4 | Notebook | Stationery | 5 | 500 | supplier4@gmail.com |
5 | Running Shoes | Apparel | 80 | 100 | supplier5@gmail.com |
ProductTable = [
{
"id":1,
"name":"Laptop",
"category":"Electronics",
"price":750,
"stock":50,
"suppliername":"supplier1@gmail.com"
},
{
"id":2,
"name":"Desk Chair",
"category":"Furniture",
"price":100,
"stock":200,
"suppliername":"supplier2@gmail.com"
},
{
"id":3,
"name":"Smartwatch",
"category":"Electronics",
"price":200,
"stock":150,
"suppliername":"supplier3@gmail.com"
},
{
"id":4,
"name":"Notebook",
"category":"Stationery",
"price":5,
"stock":100,
"suppliername":"supplier4@gmail.com"
},
{
"id":5,
"name":"Running Shoes",
"category":"Apparel",
"price":80,
"stock":100,
"suppliername":"supplier5@gmail.com"
}
]
2. Employee Table 🎯
ID | Name | Department | Age | |
1 | John Doe | Sales | 30 | john.doe@company.com |
2 | Jane Smith | Human Resources | 25 | jane.smith@company.com |
3 | Mark Johnson | IT | 40 | mark.johnson@company.com |
4 | Lisa Wong | Marketing | 28 | lisa.wong@company.com |
5 | Paul McDonald | Finance | 35 | paul.mcdonald@company.com |
EmployeeTable = [
{
"id": 1,
"name":"John Doe",
"department":"Sales",
"age": 30,
"email": "john.doe@company.com"
},
{
"id": 2,
"name":"Jane Smith",
"department":"Human Resources",
"age": 25,
"email": "jane.smith@company.com"
},
{
"id": 3,
"name":"Mark Jognson",
"department":"IT",
"age": 40,
"email": "mark.johnson@company.com"
},
{
"id": 4,
"name":"Lisa Wong",
"department":"Marketing",
"age": 28,
"email": "lisa.wong@company.com"
},
{
"id": 5,
"name":"Paul McDonald",
"department":"Finance",
"age": 35,
"email": "paul.mcdonald@company.com"
},
]
3.Books Table 🎯
ID | Title | Author | Genre | Published Year | ISBN | Stock | Price |
1 | The Great Gatsby | F. Scott Fitzgerald | Fiction | 1925 | 978-0743273565 | 20 | 15.99 |
2 | To Kill a Mockingbird | Harper Lee | Fiction | 1960 | 978-0060935467 | 35 | 10.99 |
3 | 1984 | George Orwell | Dystopian | 1949 | 978-0451524935 | 40 | 9.99 |
4 | The Catcher in the Rye | J.D. Salinger | Fiction | 1951 | 978-0316769488 | 25 | 8.99 |
5 | A Brief History of Time | Stephen Hawking | Non-fiction | 1988 | 978-0553380163 | 10 | 18.99 |
BooksTable = [
{
"id":1,
"title":"The Great Gatsby",
"author":"F. Scott Fitzgerald",
"genre":"Fiction",
"publishedyear": "1925",
"ISBN": "978-0743273565",
"stock":20,
"price": 15.99
},
{
"id":2,
"title":"To Kill a Mockingbird",
"author":"Harper Lee",
"genre":"Fiction",
"publishedyear": "1960",
"ISBN": "978-0060935467",
"stock":35,
"price": 10.99
},
{
"id":3,
"title":"1984",
"author":"George Orwell",
"genre":"Dystopian",
"publishedyear": "1949",
"ISBN": "978-0451524935",
"stock":40,
"price": 9.99
},
{
"id":4,
"title":"The Catcher in the Rye",
"author":"J.D Salinger",
"genre":"Fiction",
"publishedyear": "1951",
"ISBN": "978-0316769488",
"stock":25,
"price": 8.99
},
{
"id":5,
"title":"A Brief History of Time",
"author":"Stephen Hawking",
"genre":"Non-Fiction",
"publishedyear": "1988",
"ISBN": "978-0553380163",
"stock":10,
"price": 18.99
},
]
University Table
ID | Name | Location | Established Year | Type | Website |
1 | University of the Philippines | Quezon City | 1908 | Public | www.up.edu.ph |
2 | Ateneo de Manila University | Quezon City | 1859 | Private | www.ateneo.edu |
3 | De La Salle University | Manila | 1911 | Private | www.dlsu.edu.ph |
4 | University of Santo Tomas | Manila | 1611 | Private | www.ust.edu.ph |
5 | Polytechnic University of the Philippines | Manila | 1904 | Public | www.pup.edu.ph |
UniversityTable = [
{
"id":1,
"name": "University of the Philippines",
"location": "Quezon City",
"establishedyear": "1908",
"type": "Public",
"website": "www.up.edu.ph"
},
{
"id":2,
"name": "Ateneo de Manila University",
"location": "Quezon City",
"establishedyear": "1859",
"type": "Private",
"website": "www.ateneo.edu.ph"
},
{
"id":3,
"name": "De La Salle University",
"location": "Manila",
"establishedyear": "1911",
"type": "Private",
"website": "www.dlsu.edu.ph"
},
{
"id":4,
"name": "University of Santo Tomas",
"location": "Manila",
"establishedyear": "1611",
"type": "Private",
"website": "www.ust.edu.ph"
},
{
"id":5,
"name": "Polytechnic University of the Philippines",
"location": "Manila",
"establishedyear": "1904",
"type": "Public",
"website": "www.pup.edu.ph"
},
]
Restaurant Table
ID | Name | Location | Cuisine Type | Established Year | Website or Contact |
1 | Vikings Luxury Buffet | Pasay City | Buffet | 2011 | www.vikings.ph |
2 | Antonio's Restaurant | Tagaytay | Fine Dining | 2002 | www.antoniosrestaurant.ph |
3 | Mesa Filipino Moderne | Makati City | Filipino | 2009 | www.mesa.ph |
4 | Manam Comfort Filipino | Quezon City | Filipino | 2013 | www.manam.ph |
5 | Ramen Nagi | Various Locations | Japanese | 2013 | www.ramennagi.com.ph |
RestaurantsTable = [
{
"id": 1,
"name": "Vikings Luxury Buffet",
"location": "Pasay City",
"cuisinetype": "Buffet",
"establishedyear": "2011",
"website": "www.vikings.ph"
},
{
"id": 2,
"name": "Antonio's Restaurant ",
"location": "Tagaytay",
"cuisinetype": "Fine Dining",
"establishedyear": "2002",
"website": "www.antoniosrestaurant.ph"
},
{
"id": 3,
"name": "Mesa Filipino Moderne",
"location": "Makati City",
"cuisinetype": "Filipino",
"establishedyear": "2009",
"website": "www.mesa.ph"
},
{
"id": 4,
"name": "Manam Comfort Filipino",
"location": "Filipino",
"cuisinetype": "Filipino",
"establishedyear": "2013",
"website": "www.manam.ph"
},
{
"id": 5,
"name": "Ramen Nagi",
"location": "Various Locations",
"cuisinetype": "Japanese",
"establishedyear": "2013",
"website": "www.ramennagi.com.ph
},
]
In summary, A List is an ordered collection of items, typically of the same type, arranged sequentially and easily accessible through indexing. It allows efficient storage and management of related data like names, numbers, or even objects. On the other hand, an Object is a structure that holds data (as properties) and functionality (as methods) related to a specific entity, which makes it essential for modeling real-world concepts in an intuitive and maintainable manner. Objects are a core part of Object-Oriented Programming (OOP) due to their ability to encapsulate data and behavior. When you combine these two, a List of Objects emerges as a powerful tool a collection of multiple objects, each containing its own distinct properties and methods, making it highly useful when managing groups of complex entities like a list of students or products. Together, these structures are crucial in programming, helping developers organize data efficiently, write maintainable code, and handle both simple collections and complex real-world scenarios effectively.
In conclusion, applying data structure in our project makes sense and readable, reusable, and scalable. Applying such List to get the elements within array without worrying the parameters since it is adapting any data types infer in index array. Using object to have a set of attributes and properties makes more reusable and easier to navigate and access the value sets. While the List of Objects gives more scalable matter showcasing the potential to scalable and useable objects. All of them are part of Data Structure that makes our workload project convenient and maintainable code.
Subscribe to my newsletter
Read articles from Rhed Aligan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rhed Aligan
Rhed Aligan
I'm very enthusiast in things that gives me new material to learn.