Navigation Strapi with Angular
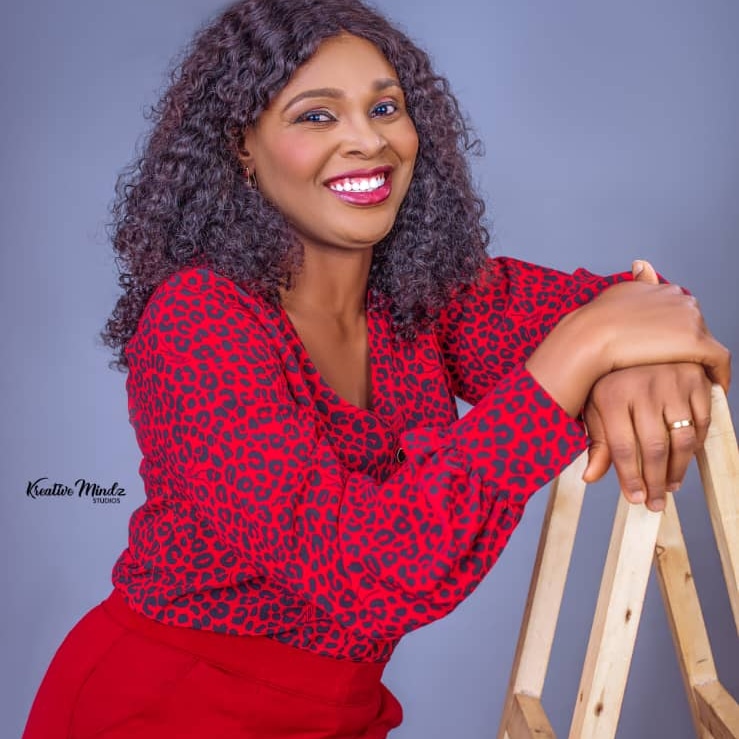
Table of contents
Introduction
In the field of web development, content management systems (CMS) play a pivotal role in shaping project competence and flexibility. Angular developers seeking a dynamic solution find Strapi CMS to be a formidable contender. This article explores the symbiotic relationship between Strapi—a self-hosted CMS—and Angular, unraveling its features, integration, and real-world benefits.
Before we get into the business of the day, let’s define some terms
Strapi: Strapi is a headless CMS that is used to develop websites, mobile applications, eCommerce sites, and APIs. It also allows the developer to create and use an API without knowing anything about the backend or databases.
Angular: Angular is an open-source Javascript framework written in Typescript, and it is maintained by Google. It has a primary purpose of developing a single-page application.
Why Choose Strapi for Angular:
Using Strapi for Angular has many advantages that make it a good choice for developers. Here are some reasons why Strapi is chosen as a CMS for Angular projects.
Features:
Versatility and simplicity
Efficient development
Extension and customisation
Rich interfaces
Popularity
API-first approach
Self-hosting
Open-source
Time-saving
Headless architecture
Content Types:
Developers can create custom content types using Strapi, which enables them to modify the data structure to meet the particular demands of the project.
Data Flexibility:
Strapi is a flexible tool that can handle a wide range of data types, including photos, text,
and more. Complex data structures can be defined and managed by developers.
RESTful API :
For every defined content type, Strapi automatically creates a RESTful API. Simple interaction and communication with the stored data are made possible by this API.
Strapi Setup for Angular:
Step-by-Step Guide: Installation and Configuration of Strapi for Angular
Ensure that your machine has both npm (Node Package Manager) and Node.js installed in it, or you can download from here.
Install Strapi CLI:
From the terminal or command prompt, run the following commands to install Strapi CLI globally:
“npm install -g strapi@latest”
- Create a Strapi Project:
Run the following command to create a new project.
“Strapi new my-strapi-project”
Follow the prompt to choose your preferred database and set up the project.
- Navigate to the new Strapi project.
Move to the newly created Strapi project directory
“cd my-strapi-project”
- Run the Strapi development server
“Strapi develop”
It will launch Strapi locally, and you will be able to access the admin panel at
Creating Content Type:
Open the browser and go to http://localhost:1337/admin
From the admin interface, create a new content type and specify the different types of attributes and properties you want for your content.
Configure the content type permissions:
To limit access, set permissions for the type of content you prefer. You can achieve this by clicking "Roles & Permissions" in the Strapi admin panel.
Expose API Endpoints:
Select "Settings" > "Roles & Permissions" and activate the appropriate permissions for your content types.
Access the Strapi API:
Test the Strapi API by navigating to http://localhost:1337/(your-content-type) in your browser. Be sure to replace your (your-content-type) with the actual content type you created.
Integrate with Angular:
Install the necessary dependencies to make HTTP requests to the Strapi API by using the command below
ng add @angular/common/http
Integration of Angular with Strapi:
Making HTTP calls to the Strapi API endpoints from your Angular application is the procedure for integrating Angular with Strapi.
- Create the Angular Services:
In your Angular project, create a service to handle HTTP requests to the Strapi API by running the following command to generate a service:
ng generate service strapi
- Configure service for Strapi API:
Open the generated service file (strapi.service.ts) and configure it to handle HTTP requests. Import Angular’s HttpClient module and make a request to Strapi API.
Code sample:
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Observable } from 'rxjs';
@Injectable({
providedIn: 'root',
})
export class StrapiService {
private apiUrl = 'http://localhost:1337';
constructor(private http: HttpClient) {}
getStrapiData(): Observable<any> {
return this.http.get<any>(`${this.apiUrl}/your-content-type`);
}
}
3. Inject Strapi Service into Angular Components:
To utilise Strapi data, inject the Strapi service into your created Angular components.
Code Sample:
import { Component, OnInit } from '@angular/core';
import { StrapiService } from './path-to-your-strapi-service';
@Component({
selector: 'app-your-component',
templateUrl: './your-component.component.html',
styleUrls: ['./your-component.component.css'],
})
export class YourComponent implements OnInit {
strapiData: any;
constructor(private strapiService: StrapiService) {}
ngOnInit(): void {
this.fetchStrapiData();
}
fetchStrapiData(): void {
this.strapiService.getStrapiData().subscribe(
(data) => {
this.strapiData = data;
},
(error) => {
console.error('Error fetching Strapi data:', error);
}
);
}
}
4. Use Strapi Data in Angular Templates:
Use the Strapi data information the service fetched for your Angular component templates (HTML files).
Code sample
<div *ngFor="let item of strapiData">
<p>{{ item.title }}</p>
<p>{{ item.description }}</p>
</div>
Accessing Strapi's RESTful API with Angular:
Open the Angular service file (strapi.service.ts) and add the methods to perform CRUD operations on Strapi data.
Code sample
// Inside StrapiService class
// ...
createStrapiData(newData: any): Observable<any> {
return this.http.post<any>(`${this.apiUrl}/your-content-type`, newData);
}
updateStrapiData(id: number, updatedData: any): Observable<any> {
return this.http.put<any>(`${this.apiUrl}/your-content-type/${id}`, updatedData);
}
// Example method to delete an item
deleteStrapiData(id: number): Observable<any> {
return this.http.delete<any>(`${this.apiUrl}/your-content-type/${id}`);
}
Building Components for Strapi Data Interaction:
Create Angular components that handle or show Strapi data and communicate with the Strapi service.
Here is a sample:
import { Component, OnInit } from '@angular/core';
import { StrapiService } from './path-to-your-strapi-service';
@Component({
selector: 'app-strapi-data',
templateUrl: './strapi-data.component.html',
styleUrls: ['./strapi-data.component.css'],
})
export class StrapiDataComponent implements OnInit {
strapiData: any;
constructor(private strapiService: StrapiService) {}
ngOnInit(): void {
this.fetchStrapiData();
}
fetchStrapiData(): void {
this.strapiService.getStrapiData().subscribe(
(data) => {
this.strapiData = data;
},
(error) => {
console.error('Error fetching Strapi data:', error);
}
);
}
}
Displaying Contents in Angular:
In the HTML template of your Angular component, use Angular directives like ngFor to loop through and display Strapi data.
Code sample:
<!-- Inside strapi-data.component.html -->
<div *ngFor="let item of strapiData">
<p>{{ item.title }}</p>
<p>{{ item.description }}</p>
<!-- Add more HTML to structure and style your Strapi data -->
</div>
Best Practices:
Optimize Data Fetching:
When working with huge datasets, use pagination.
To obtain only the fields that are required, use the choose query parameter.
Use caching techniques to cut down on recurrent queries.
Authentication and Authorization:
Define the roles and permissions for a secured API endpoint.
For safe authentication, use JSON Web Tokens (JWT).
Make sure that Angular and Strapi are communicating via HTTPS.
For improved security, put in place token expiration and renewal procedures.
Effective Data Relationship Management in Angular-Strapi Integration
In Strapi, clearly define the relationships between different content categories.
GraphQL is a good choice for intricate queries.
Carefully manage nested relationships to achieve the best possible outcome.
By indexing fields used in relationships, you can optimize queries.
Use Cases:
Showcase Angular Projects with Strapi:
Dashboard for E-Commerce:
Benefits include a dynamic product catalog, easy updates, and real-time inventory.
Result: optimized user experience and effective e-commerce data management.
Portal for Education:
Benefits include safe access controls, multimedia integration, and simple course content administration.
Result: A streamlined content delivery platform that is interactive.
Website for a portfolio:
Benefits include quick content updates, engaging project presentations, and effective media management.
Result: Angular-Strapi integrated portfolio management that is easy to use.
Teamwork in Blogging:
Benefits include dynamic blog displays, tailored user experiences, and organized content generation.
Result: A user-friendly blogging platform that encourages interaction.
Benefits and Outcomes:
Effective Management of Content:
Outcome: Easy-to-use admin interface for quick adjustments to content and smooth data management.
Presenting data dynamically:
Outcome: Angular-Strapi synergy enables interactive user experiences and real-time updates.
Flexibility and Scalability:
Outcome: modular projects that grow to meet changing needs
Enhanced Protection:
Outcome: By using Strapi's authentication measures, user data and transactions are secure.
Simplified Process for Development Work:
Outcome: Angular developers will experience quicker development cycles and more effective workflows.
Conclusion:
The synergy of Angular and Strapi CMS empowers developers for modern web challenges. Strapi’s flexibility and Angular's robust framework create a potent alliance for data-driven applications. Best practices ensure sound relationship management, secure authentication, and optimal performance. As you navigate web development, consider the Angular-Strapi narrative a tale of harmonious integration inviting you to elevate your projects.
Subscribe to my newsletter
Read articles from Achu Ijeoma Cynthia directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
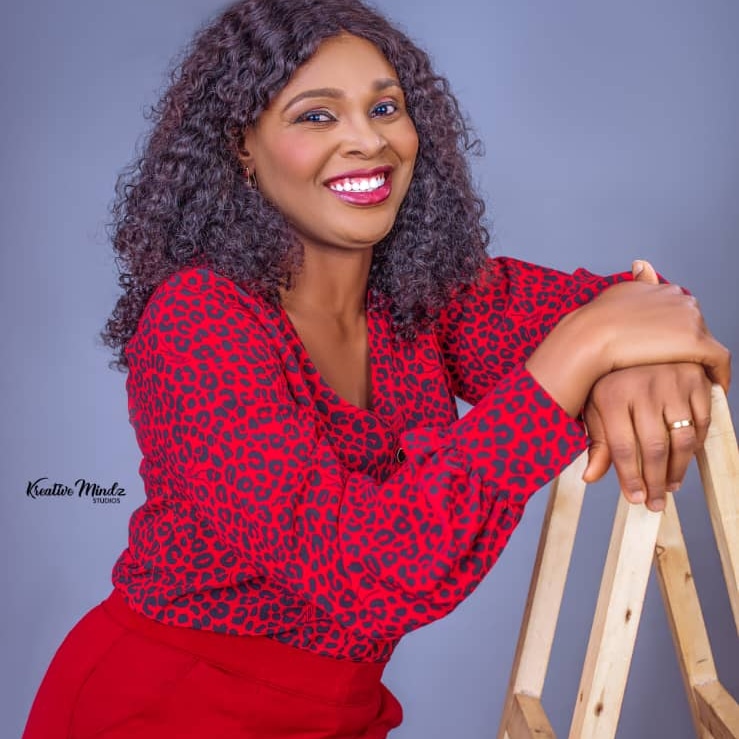
Achu Ijeoma Cynthia
Achu Ijeoma Cynthia
Hello, and welcome to my blog! I'm Ijeoma Achu, a passionate and dedicated mobile app developer with expertise in Dart, Git and Github, Flutter, and Firebase. What sets me apart is not just my technical skills, but also my unique journey into the world of technology. I began my professional journey in the field of dental technology, where precision and attention to detail were paramount. While I enjoyed the challenges it presented, my curiosity for technology and innovation led me to explore new horizons. Driven by a deep-seated passion for mobile app development, I embarked on a transformative career transition to pursue my true calling.