Java for Beginners

Table of contents
- Why do you think Java is still used everywhere from toasters to space missions? Maybe it’s just really good at multitasking like a caffeinated programmer?”
- ‘ Kicking Off with‘ Hello, World ! ’ — Because Every Great Programmer Starts with a “ Hello World”!
- Java: The Object-Oriented Playground
- 3. Polymorphism: One Name, Many Forms
- 4. Abstraction: Simplifying Complexity
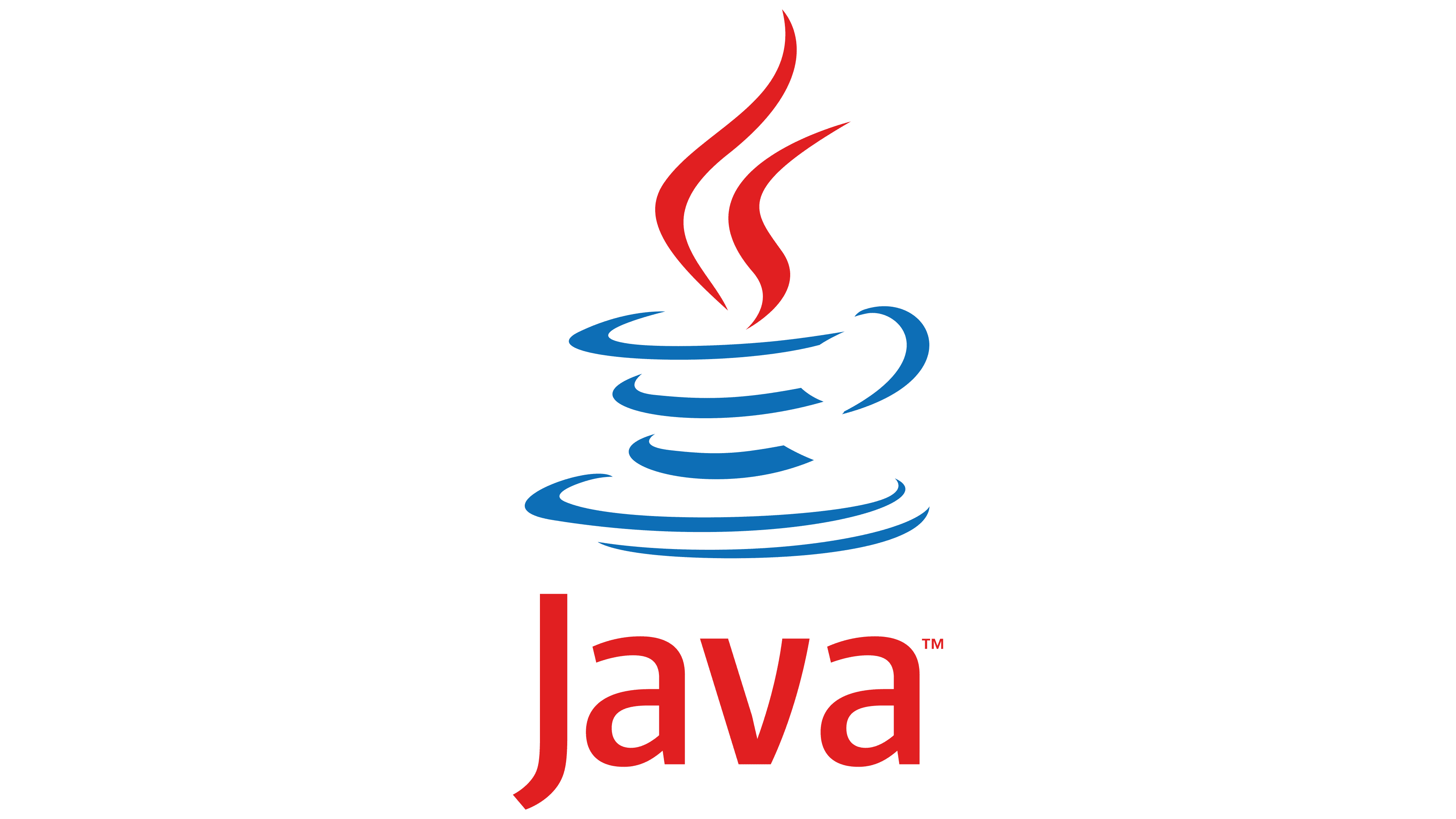
Ever wanted to create your own games, apps, or websites? Java is the perfect language to start your journey into the exciting world of programming. Join us as we explore the basics of Java and learn how to build your first digital creations.
No prior programming experience needed! I will break down complex concepts into simple terms and provide plenty of hands-on examples. Let’s dive in and discover the endless possibilities of Java together.
Why do you think Java is still used everywhere from toasters to space missions? Maybe it’s just really good at multitasking like a caffeinated programmer?”
Java, first released by Sun Microsystems in 1995, is now 29 years old as of 2024. Despite its age, it remains a top choice for developers, especially when building enterprise-ready applications. According to the Stack Overflow Developer Survey 2024, 30.3% of professional developers still actively use Java — proof that some classics never go out of style!
Lets Drive Into the world of Java;
‘ Kicking Off with‘ Hello, World ! ’ — Because Every Great Programmer Starts with a “ Hello World”!
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
This little piece of code is your ticket to the Java club. It defines a class named HelloWorld
(because what else would it be called?) and has a main
method that prints "Hello, World!" to the console. It’s simple, it’s classic, and yes, it’s a mandatory tradition—like wearing mismatched socks on the first day of school!
Java: The Object-Oriented Playground
Now that you’ve dipped your toes into the world of Java with “Hello, World!”, let’s talk about what makes Java tick — its object-oriented nature. Yes, Java is an object-oriented programming (OOP) language, which means it revolves around the concepts of classes and objects.
Classes and Objects: The Dynamic Duo of Java
Welcome to the wild world of classes and objects in Java! Think of classes as the blueprints for a secret club, and objects as the members who actually show up to the party.
Classes: The Secret Club Blueprints
Imagine you want to create a super exclusive club called “The Cool Cats Club.” To do this, you need a blueprint — a class! This class outlines all the important details:
Name of the Club: The Cool Cats Club
Membership Criteria: Must have a fabulous sense of style and a love for catnip.
Club Activities: Daily naps, chasing laser pointers, and the occasional catnip party!
So, your class would look something like this:
public class CoolCatsClub {
String clubName = "The Cool Cats Club"; // Name of the club
int membershipLimit = 10; // Maximum members
// Method for club activities
public void napTime() {
System.out.println("Time for a cozy nap!");
}
}
Objects: The Members Who Actually Show Up :)
Now that you have your classy blueprint, it’s time to invite some members! Each member of your club is an object — a unique cat that follows the rules of the club. Here’s how you might create a few members:
public class CatDemo {
public static void main(String[] args) {
CoolCatsClub cat1 = new CoolCatsClub(); // Member 1
CoolCatsClub cat2 = new CoolCatsClub(); // Member 2
System.out.println(cat1.clubName + " welcomes you!");
cat1.napTime(); // Calling the nap time activity for Member 1
System.out.println(cat2.clubName + " also welcomes you!");
cat2.napTime(); // Calling the nap time activity for Member 2
}
}
Key Concepts of Object-Oriented Programming in Java
Now that you understand classes and objects, let’s talk about some cool features of object-oriented programming (OOP) in Java. Think of these features as helpful tools that make coding easier and more organized!
Oops Pillars for Java .
1. Encapsulation: Keeping Secrets Safe
Encapsulation is like having a secret diary. You can decide who gets to read your diary and who can’t. In Java, we use private and public to protect our data:
Private means “Only I can see this!”
Public means “Everyone can see this!”
For example, if you have a class called Diary
, you might want to keep the diary’s content private but allow your friends to read it through public methods:
public class Diary {
private String entry; // Only I can see this!
// Public method to share the entry
public String readEntry() {
return entry;
}
// Public method to write a new entry
public void writeEntry(String newEntry) {
entry = newEntry;
}
public static void main(String[] args) {
Diary myDiary = new Diary();
myDiary.writeEntry("Today was a great day!");
System.out.println("Diary Entry: " + myDiary.readEntry());
}
}
2. Inheritance: The Family Tree
Inheritance is like when kids inherit traits from their parents. If you have a Pet
class, you can create a Dog
class that gets all the features of Pet
but also adds its own special things, like barking !
public class Pet {
public void eat() {
System.out.println("Eating...");
}
}
public class Dog extends Pet {
public void bark() {
System.out.println("Woof!");
}
public static void main(String[] args) {
Dog myDog = new Dog();
myDog.eat(); // Inherited from Pet
myDog.bark(); // Specific to Dog
}
}
3. Polymorphism: One Name, Many Forms
Polymorphism means “many shapes,” and it allows methods to do different things based on the object they are acting on. It’s like having a remote control that works on different devices — TVs, air conditioners, etc., but behaves differently for each.
How it works: Methods can be overridden (inherited class can change how a method works) or overloaded (multiple methods with the same name but different parameters).
Why it’s useful: It allows flexibility in your code and makes it easier to handle different types of objects in a uniform way
publIc class Animal {
public void sound() {
System.out.println("Some generic animal sound.");
}
}
public class Dog extends Animal {
@Override
public void sound() { // Polymorphism: Overriding
System.out.println("Bark!");
}
}
public class Cat extends Animal {
@Override
public void sound() {
System.out.println("Meow!");
}
}
4. Abstraction: Simplifying Complexity
Abstraction hides the complex details and shows only the essential features of an object, like how a car dashboard shows only the important controls (steering, pedals) and hides the complex mechanics underneath.
How it works: You can create abstract classes or interfaces with method declarations but no implementation. Other classes will fill in the details.
Why it’s useful: It helps manage complexity by focusing on what an object does rather than how it does it.
abstract class Animal {
// Abstract method (no implementation)
public abstract void sound();
}
class Dog extends Animal {
@Override
public void sound() {
System.out.println("Bark!");
}
}
class Cat extends Animal {
@Override
public void sound() {
System.out.println("Meow!");
}
}
public class Main {
public static void main(String[] args) {
Animal myDog = new Dog();
Animal myCat = new Cat();
myDog.sound(); // Outputs: Bark!
myCat.sound(); // Outputs: Meow!
}
}
These four pillars are the building blocks of writing clean, efficient, and scalable code in Java! .
Stay Tuned for More Java Adventures!
This is just the beginning! There’s so much more to explore in the world of Java, and I’ll be diving into some awesome new topics in the next post. Whether it’s tackling Java’s advanced features, diving into real-world projects, or cracking some tricky coding puzzles, the next part of our Java series will be coming your way soon.
So, grab your coffee ☕ and keep an eye out — I’ll be back before you know it with more Java goodness to fuel your coding journey!
Until then, happy coding! 👨💻👩💻
This comprehensive guide was contributed by Harsh Chaudhari. Check out more insightful posts on his account !
Subscribe to my newsletter
Read articles from MicrosoftLearnStudentClub directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
