Understanding Composite SLA Calculations in Kubernetes Systems
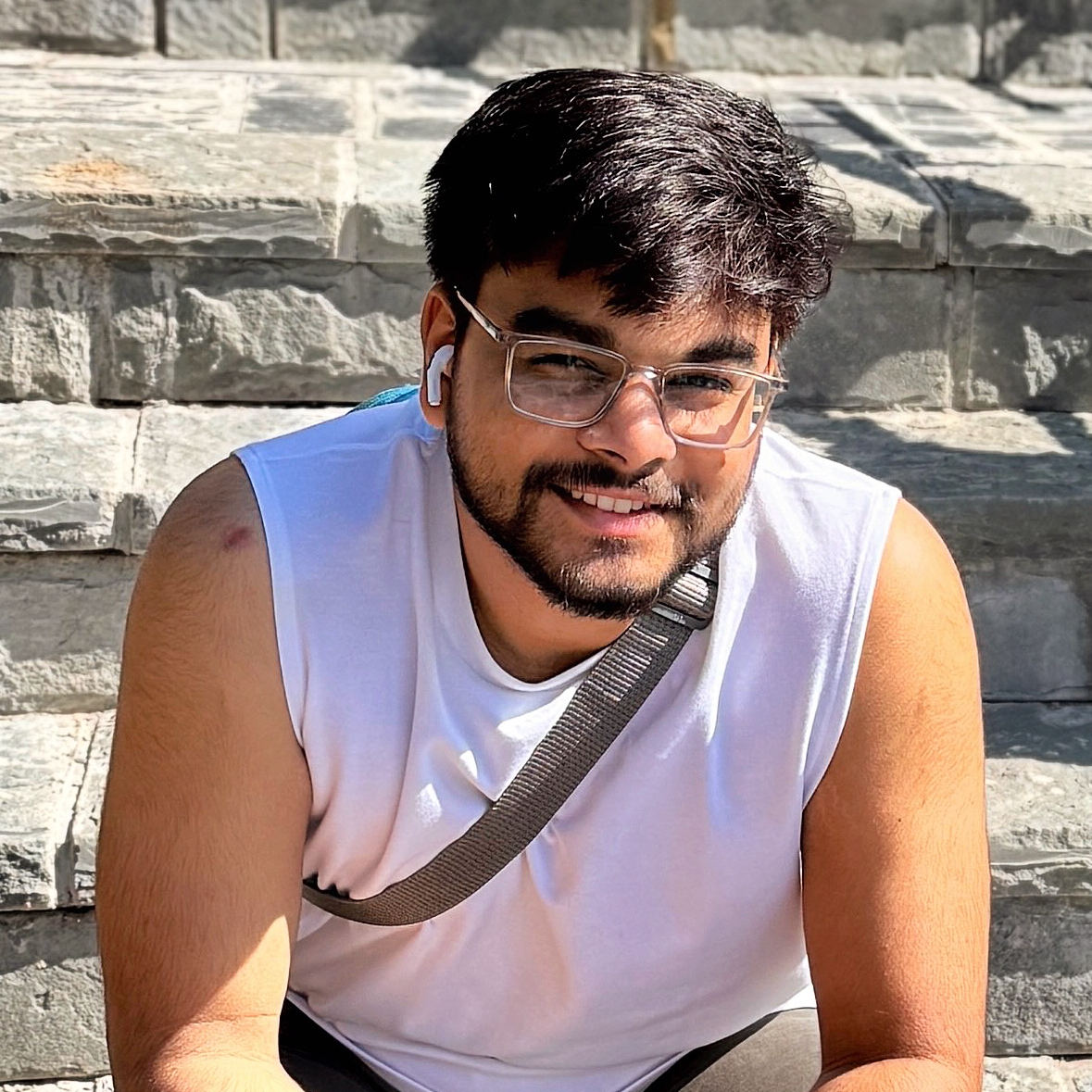
Table of contents
- Introduction
- What is a Composite SLA?
- Formula for Composite SLA Calculation
- Real-World example: E-commerce Application on Kubernetes
- Step-by-Step Implementation of Composite SLA Calculation in Kubernetes
- Step 1: Set Up Kubernetes Cluster
- Step 2: Deploy Microservices and External Services
- Step 3: Install Prometheus for SLA Monitoring
- Step 4: Install Grafana for SLA Visualization
- Step 5: Automate Composite SLA Calculation
- Step 6: Chaos Testing with Chaos Mesh
- Step 7: Architecture Diagram
- Step 8: Testing and Validating SLAs
- Conclusion
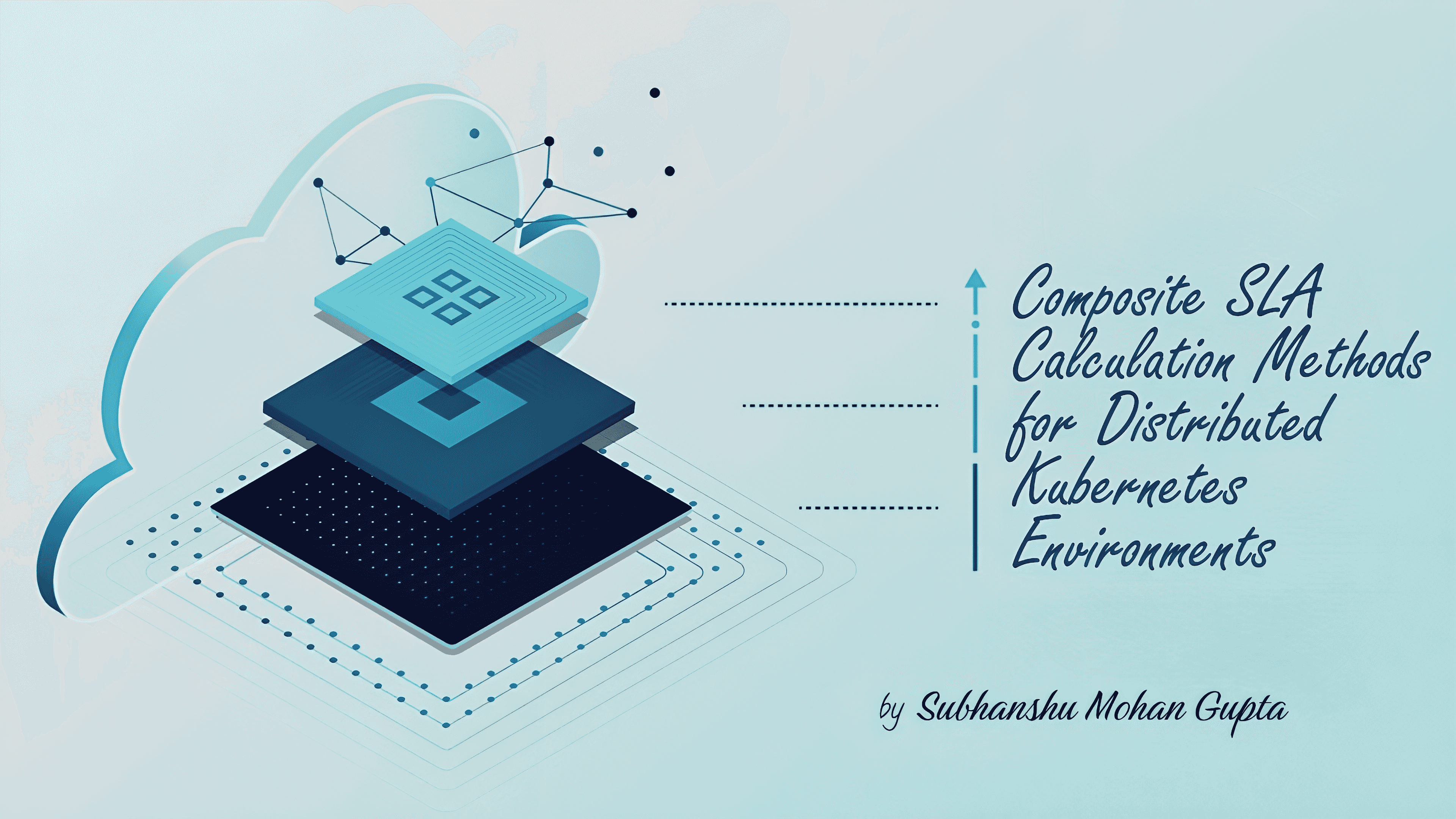
Welcome to Part V of my Kubernetes series! In this installment, we’re going to explore the complex yet crucial process of calculating the Composite Service Level Agreement (SLA) for distributed applications running on Kubernetes. As microservices grow and scale, keeping track of their individual SLAs across databases, third-party APIs, and cloud providers becomes essential for ensuring overall system reliability. We'll walk through real-world examples, and by the end, you’ll have a clear understanding of how to combine multiple SLAs into a single, comprehensive metric for your distributed Kubernetes environment.
But before diving into the details, it's worth reflecting on why calculating a Composite SLA is so important. In a typical Kubernetes setup, services rely on each other and sometimes on external providers. If even one component fails, it can degrade the entire system's reliability. By combining SLAs, we get a clear picture of the weakest links in our infrastructure and where we need to improve.
Introduction
In today's cloud-native world, distributed systems are the backbone of modern applications. These systems often involve multiple microservices, external APIs, cloud providers, and databases, all running seamlessly in Kubernetes environments. One of the most critical challenges for DevOps teams is ensuring these systems meet high availability and reliability expectations. This is where Service Level Agreements (SLAs) come into play.
In this article, we’ll explore how to calculate the Composite SLA for distributed applications running on Kubernetes. We will dive into the intricate process of combining SLAs from various components (microservices, APIs, databases, and cloud services) to form an end-to-end SLA. Through a real-world example, you’ll gain a concrete understanding of how to implement and measure this effectively. Let’s begin by understanding the essence of SLAs and how they impact system reliability.
What is a Composite SLA?
A Composite SLA is an aggregate SLA that considers all the different components a system depends on. In Kubernetes-based distributed systems, multiple microservices, third-party APIs, databases, and infrastructure providers are often used to deliver a complete application. Each of these components has its own individual SLA, which guarantees a specific level of performance, uptime, or availability.
The challenge is that the system's overall availability depends on all its parts. If one microservice has downtime, the entire system may suffer, even if the rest of the services are up and running. Calculating the composite SLA allows you to predict the cumulative effect of these individual SLAs on the overall system reliability.
Formula for Composite SLA Calculation
To calculate the Composite SLA, you combine the individual SLAs using the following formula:
Where:
SLA_i is the individual SLA of each component.
n is the total number of components.
For example, if you have three services with SLAs of 99.9%, 99.5%, and 99%, the composite SLA would be:
— 𝐂𝐨𝐦𝐩𝐨𝐬𝐢𝐭𝐞 𝐒𝐋𝐀 = 𝟎.𝟗𝟗𝟗×𝟎.𝟗𝟗𝟓×𝟎.𝟗𝟗≈𝟎.𝟗𝟖𝟒𝟓 𝐨𝐫 𝟗𝟖.𝟒𝟓% —
This means that even though each service is highly available, the overall system’s availability decreases due to the dependency on multiple components.
Real-World example: E-commerce Application on Kubernetes
Let’s consider an e-commerce application hosted on a distributed Kubernetes system. This application consists of:
Frontend service (SLA: 99.9%)
Payment gateway (third-party API with SLA: 99.5%)
Database service (SLA: 99.9%)
Cloud provider infrastructure (SLA: 99.95%)
To calculate the Composite SLA for this e-commerce system, we combine the individual SLAs:
— 𝐂𝐨𝐦𝐩𝐨𝐬𝐢𝐭𝐞 𝐒𝐋𝐀 = 𝟎.𝟗𝟗𝟗×𝟎.𝟗𝟗𝟓×𝟎.𝟗𝟗𝟗×𝟎.𝟗𝟗𝟗𝟓≈𝟎.𝟗𝟗𝟐𝟓 𝐨𝐫 𝟗𝟗.𝟐𝟓% —
This means that the overall availability of your e-commerce application is about 99.25%, meaning the system is expected to be down for approximately 6.5 hours per year.
Step-by-Step Implementation of Composite SLA Calculation in Kubernetes
To implement the architecture for calculating the Composite SLA for distributed Kubernetes systems, we will use Kubernetes, Prometheus for monitoring, Grafana for visualization, and a script to automate the Composite SLA calculation. Below is a precise, step-by-step guide with code samples to achieve this.
Step 1: Set Up Kubernetes Cluster
First, ensure you have a Kubernetes cluster running. If you don’t have a cluster, you can use Minikube or a managed Kubernetes service like GKE (Google Kubernetes Engine) or EKS (Amazon Elastic Kubernetes Service).
To set up a local Kubernetes cluster using Minikube: minikube start
Once the cluster is running, you can deploy your microservices and third-party services onto Kubernetes.
Step 2: Deploy Microservices and External Services
Assuming you have multiple microservices and databases in the distributed system, you can deploy them to Kubernetes. Here’s a simple deployment of three microservices (frontend, payment gateway, and database).
- Create deployment YAMLs for each service (frontend, payment API, and database):
# frontend.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: frontend
spec:
replicas: 3
selector:
matchLabels:
app: frontend
template:
metadata:
labels:
app: frontend
spec:
containers:
- name: frontend
image: your-registry/frontend:latest
ports:
- containerPort: 80
---
apiVersion: v1
kind: Service
metadata:
name: frontend
spec:
selector:
app: frontend
ports:
- protocol: TCP
port: 80
targetPort: 80
You would repeat this for payment API and database. For external services (like third-party APIs), these could be represented by external services using Kubernetes service objects.
# payment-gateway.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: payment-gateway
spec:
replicas: 2
selector:
matchLabels:
app: payment-gateway
template:
metadata:
labels:
app: payment-gateway
spec:
containers:
- name: payment-gateway
image: your-registry/payment-gateway:latest
ports:
- containerPort: 8080
---
apiVersion: v1
kind: Service
metadata:
name: payment-gateway
spec:
selector:
app: payment-gateway
ports:
- protocol: TCP
port: 8080
targetPort: 8080
# database.yaml
apiVersion: apps/v1
kind: Deployment
metadata:
name: database
spec:
replicas: 1
selector:
matchLabels:
app: database
template:
metadata:
labels:
app: database
spec:
containers:
- name: database
image: your-registry/database:latest
ports:
- containerPort: 5432
---
apiVersion: v1
kind: Service
metadata:
name: database
spec:
selector:
app: database
ports:
- protocol: TCP
port: 5432
targetPort: 5432
Apply the manifests to your cluster:
kubectl apply -f frontend.yaml
kubectl apply -f payment-gateway.yaml
kubectl apply -f database.yaml
Step 3: Install Prometheus for SLA Monitoring
Prometheus is a powerful monitoring tool to track the uptime and performance of services in a Kubernetes cluster.
Install Prometheus
using Helm (the Kubernetes package manager):
helm repo add prometheus-community https://prometheus-community.github.io/helm-charts
helm repo update
helm install prometheus prometheus-community/prometheus
Expose Prometheus
kubectl port-forward deploy/prometheus-server 9090
Prometheus is now available at http://localhost:9090
.
Set up Service Level Indicator (SLI) metrics
for each microservice. Here’s an example of an SLI rule to monitor uptime and error rate for the frontend service:
groups:
- name: sla_rules
rules:
- alert: HighErrorRate
expr: rate(http_requests_total{job="frontend", status!~"2.."}[5m]) > 0.05
for: 10m
labels:
severity: critical
annotations:
summary: "High error rate detected in frontend"
description: "The error rate for frontend is above 5%."
Store this as a YAML file (e.g., frontend_sla_rules.yaml
) and configure Prometheus to pick it up in its config map.
Step 4: Install Grafana for SLA Visualization
Install Grafana
with Helm:
helm repo add grafana https://grafana.github.io/helm-charts
helm repo update
helm install grafana grafana/grafana
Expose Grafana
kubectl port-forward deploy/grafana 3000
Grafana will be accessible at http://localhost:3000
. The default username is admin
and the password is generated by Helm:
kubectl get secret --namespace default grafana -o jsonpath="{.data.admin-password}" | base64 --decode ; echo
Connect Prometheus to Grafana
Log in to Grafana.
Navigate to Configuration > Data Sources > Add data source.
Choose Prometheus and add your Prometheus server URL (
http://prometheus-server.default.svc.cluster.local:9090
).
Create Dashboards
Create custom dashboards to visualize the SLAs for each service and the overall Composite SLA. You can track metrics like uptime, error rate, and response time.
Step 5: Automate Composite SLA Calculation
- Create a Python script to calculate the Composite SLA based on individual SLAs collected via Prometheus.
import requests
# Prometheus query URLs for each service's uptime (for simplicity)
frontend_sla = "http://localhost:9090/api/v1/query?query=avg_over_time(up{job='frontend'}[1d])"
payment_sla = "http://localhost:9090/api/v1/query?query=avg_over_time(up{job='payment-gateway'}[1d])"
database_sla = "http://localhost:9090/api/v1/query?query=avg_over_time(up{job='database'}[1d])"
def get_sla(url):
response = requests.get(url)
result = response.json()['data']['result']
if result:
return float(result[0]['value'][1])
return 0.0
# Fetch SLAs for all services
frontend = get_sla(frontend_sla)
payment = get_sla(payment_sla)
database = get_sla(database_sla)
# Calculate Composite SLA
composite_sla = frontend * payment * database
print(f"Composite SLA: {composite_sla * 100:.2f}%")
Run this script periodically (e.g., using CronJobs in Kubernetes or a Jenkins pipeline) to calculate and log the Composite SLA.
Step 6: Chaos Testing with Chaos Mesh
To ensure your SLAs and Composite SLA are resilient, test your system with Chaos Mesh to simulate failures and observe the impact on SLAs.
Install Chaos Mesh
kubectl apply -f https://mirrors.chaos-mesh.org/v2.1.2/chaos-mesh.yaml
Create chaos experiments
to simulate downtime for microservices (like shutting down the database):
apiVersion: chaos-mesh.org/v1alpha1
kind: PodChaos
metadata:
name: database-chaos
spec:
action: pod-kill
mode: one
selector:
labelSelectors:
"app": "database"
duration: "30s"
scheduler:
cron: "@every 1m"
Deploy this to simulate downtime, then observe how it impacts the Composite SLA.
Step 7: Architecture Diagram
Here’s a simple architecture diagram showing the components for calculating Composite SLA:
Kubernetes Cluster: Hosts microservices, APIs, and databases.
Prometheus: Collects uptime and performance metrics.
Grafana: Visualizes the SLAs and system performance.
Python Script: Calculates the Composite SLA.
Chaos Mesh: Injects failures to test SLA resilience.
Step 8: Testing and Validating SLAs
Simulate Failures: Use Chaos Mesh to simulate failures and measure how each service’s downtime impacts the Composite SLA.
Verify Alerts: Ensure Prometheus alerts are triggered when SLAs are breached.
Test Dashboards: Monitor Grafana to ensure all SLAs are being correctly visualized.
Conclusion
Calculating and maintaining the Composite SLA for distributed Kubernetes systems is crucial for ensuring reliable and robust applications. By following the steps outlined in this blog, you can automate the monitoring, calculation, and visualization of SLAs to provide transparency to stakeholders and ensure high availability.
By understanding how each component affects your overall system SLA, you can better plan for redundancy, handle failures, and optimize your Kubernetes architecture for uptime.
Real-world problem solved: Now that you know how to calculate the composite SLA for your distributed Kubernetes system, you'll be able to ensure that your system meets its reliability goals. Whether it's an e-commerce application or a mission-critical system, calculating composite SLAs provides a holistic view of system performance, helping you maintain high availability while reducing downtimes.
References
Here are some reference links that can provide additional insights and details for your article on Calculating the Composite SLA for Distributed Kubernetes Systems:
Service Level Agreements (SLAs):
Microservices and SLAs:
CDN and Performance Optimization:
Caching Strategies in Kubernetes:
Rate Limiting in Kubernetes:
Composite SLA Calculation:
What’s next?
Get ready for Part VI, where we’ll dive into the exciting world of optimizing Kubernetes performance through Caching, Content Delivery Networks (CDNs), and Rate Limiting.
An article you definitely don’t want to miss!
Subscribe to my newsletter
Read articles from Subhanshu Mohan Gupta directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
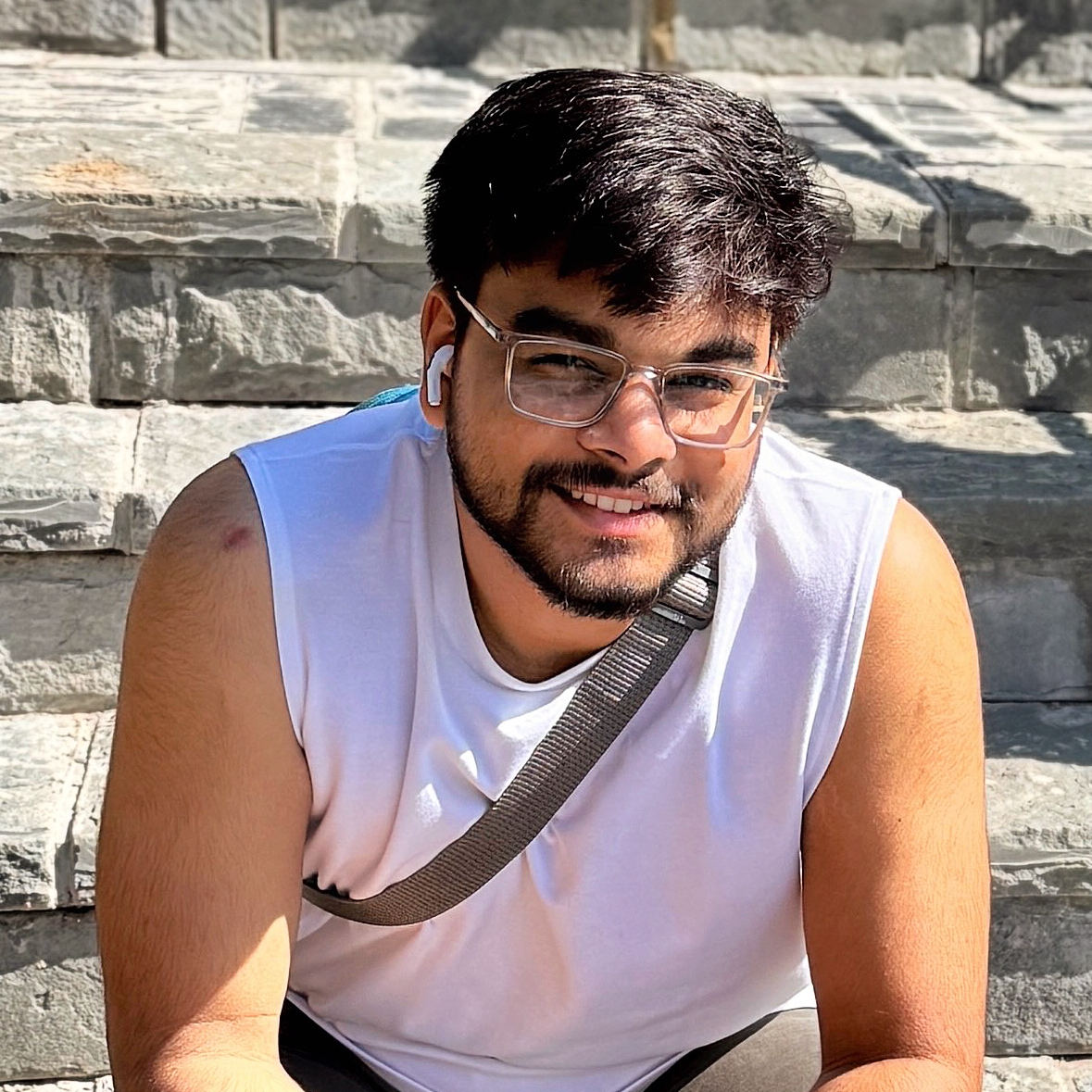
Subhanshu Mohan Gupta
Subhanshu Mohan Gupta
A passionate AI DevOps Engineer specialized in creating secure, scalable, and efficient systems that bridge development and operations. My expertise lies in automating complex processes, integrating AI-driven solutions, and ensuring seamless, secure delivery pipelines. With a deep understanding of cloud infrastructure, CI/CD, and cybersecurity, I thrive on solving challenges at the intersection of innovation and security, driving continuous improvement in both technology and team dynamics.