Understanding Variables in Python: A Beginner’s Guide

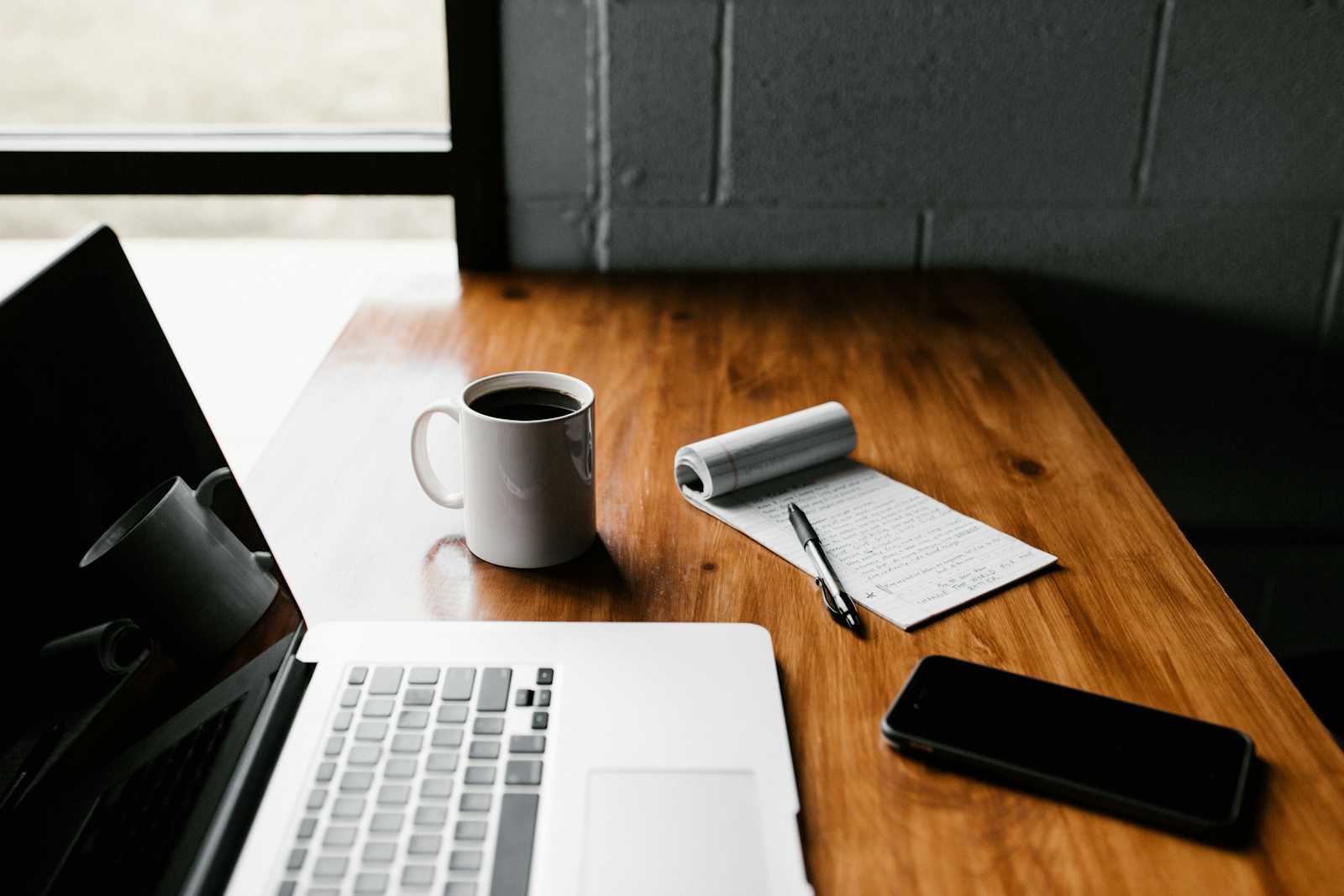
Introduction
Welcome to the world of Python programming! One of the first concepts you'll encounter is the idea of variables. Understanding how to use variables is fundamental to writing effective code. In this blog post, we’ll explore what variables are, how to use them, and some best practices to keep in mind.
What is a Variable?
In programming, a variable is like a container that holds data. It allows you to store information and refer to it later in your code. Think of a variable as a label that points to a value in memory.
For example, if you want to store the age of a person, you can create a variable named age
and assign it a value:
pythonCopy codeage = 25
Here, age
is the variable name, and 25
is the value stored in it.
Naming Variables
When creating variables, it’s essential to follow some naming conventions:
Descriptive Names: Use meaningful names that describe the data. Instead of
x
, useage
ortotal_price
.Snake Case: In Python, it's common to use lowercase letters and underscores for variable names (e.g.,
user_name
).No Spaces or Special Characters: Variable names can’t contain spaces or symbols (like
@
,#
, etc.), though you can use underscores.Start with a Letter or Underscore: Variable names must start with a letter (a-z, A-Z) or an underscore (_).
Examples of Valid and Invalid Variable Names
Valid:
pythonCopy codeuser_name = "Alice"
total_price = 99.99
_is_active = True
Invalid:
pythonCopy codeuser name = "Alice" # Contains a space
2nd_place = 10 # Starts with a digit
total-price = 99.99 # Contains a hyphen
Assigning Values to Variables
You can assign different types of values to variables, including:
Integers: Whole numbers (e.g.,
age = 30
)Floats: Decimal numbers (e.g.,
price = 19.99
)Strings: Text enclosed in quotes (e.g.,
name = "Bob"
)Booleans: True or false values (e.g.,
is_student = False
)
Example of Different Data Types
pythonCopy codename = "Alice" # String
age = 25 # Integer
height = 5.5 # Float
is_student = True # Boolean
Reassigning Variables
In Python, you can change the value of a variable at any time. For example:
pythonCopy codeage = 25
print(age) # Output: 25
age = 26 # Reassigning a new value
print(age) # Output: 26
Dynamic Typing
Python is a dynamically typed language, which means you don’t need to declare the type of a variable when you create it. The interpreter determines the type based on the value assigned:
pythonCopy codemy_variable = "Hello" # my_variable is a string
my_variable = 42 # Now my_variable is an integer
Best Practices for Using Variables
Keep It Simple: Use simple, descriptive names to make your code more readable.
Avoid Global Variables: Try to limit the use of global variables. They can lead to confusing bugs in larger programs.
Comment Your Code: Use comments to explain the purpose of your variables, especially if their meaning isn’t immediately clear.
Conclusion
Variables are a foundational concept in Python programming. Understanding how to use them effectively will empower you to write better code and create more complex programs. As you continue your Python journey, remember to practice naming conventions, data types, and dynamic typing. Happy coding!
If you have any questions or topics you’d like to explore further, feel free to leave a comment below. Let’s learn together!
Subscribe to my newsletter
Read articles from utenge Essien directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
