Let's Learn How Programs Are Compiled and Executed
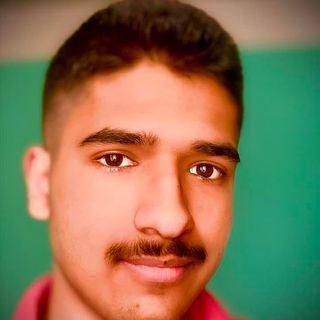
Table of contents
- What Is a Language System?
- The Classical Sequence: From Source Code to Running Program
- Modern Variations on the Classical Sequence
- Binding Times: When Things Get Connected
- Debuggers: Hunting Down Bugs
- Runtime Support: Making Programs Run Smoothly
- Delayed Linking and Dynamic Libraries
- Conclusion: Language Systems Behind the Scenes
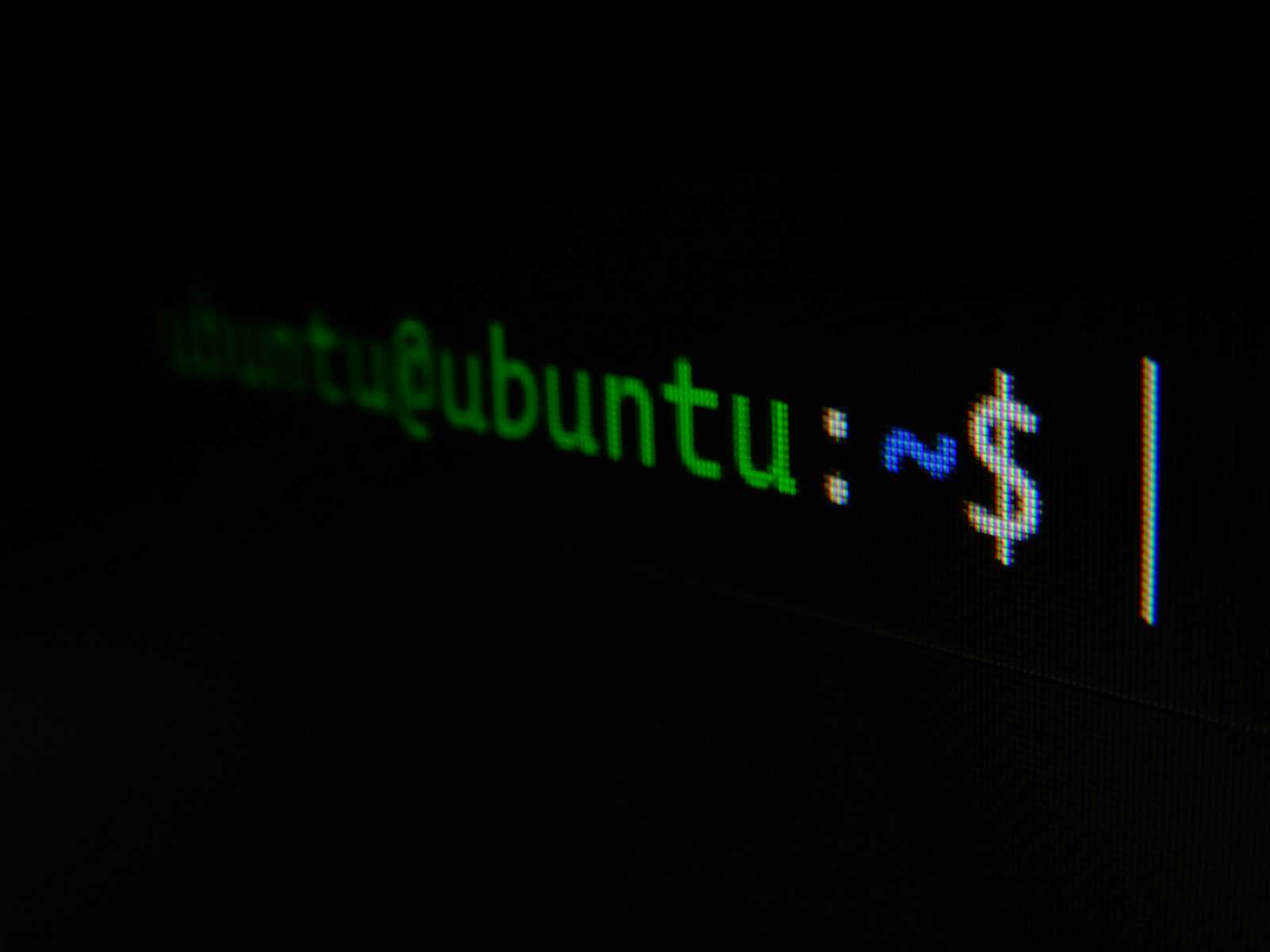
Ever wondered what happens when you press “Run” after writing code? Whether you’re coding in C, Python, or Java, your source code goes through several steps before becoming a fully functional program. These steps are governed by the language system, which handles everything from translating your code to linking it with external libraries. In this blog, we’ll dive deep into the classical sequence of program execution, explore how virtual machines and interpreters change the game, and learn about important concepts like binding times, debugging tools, and runtime support.
What Is a Language System?
A language system implements a programming language by handling all the necessary steps to turn source code into an executable program. These steps include editing, compiling, assembling, linking, loading, and finally running the program. While modern Integrated Development Environments (IDEs) often make this process seamless, understanding the underlying operations is crucial for anyone working with programming languages.
The Classical Sequence: From Source Code to Running Program
The classical sequence represents the traditional process of turning source code into an executable file. While modern systems simplify this process, understanding the classical steps provides insight into the inner workings of language systems.
Step 1: Editing
The programmer writes the source code in a high-level programming language such as C, Python, or Java. This is a machine-independent representation of the program, written in human-readable code.
int i;
void main() {
for (i = 1; i <= 100; i++)
fred(i);
}
Step 2: Compiling
The compiler translates the high-level code into assembly language, which is machine-specific. Each line of assembly represents either a piece of data or a single machine-level instruction.
i: data word 0
main: move 1 to i
t1: compare i with 100
jump to t2 if greater
push i
call fred
add 1 to i
go to t1
t2: return
Step 3: Assembling
The assembler converts the assembly-language file into machine language (binary format). This creates an object file that can be understood by the computer, though it is not yet ready to execute.
Step 4: Linking
The linker combines the object files into an executable file. It resolves external function references, like calls to libraries or functions defined in other files. For example, if the function fred
is compiled separately, the linker finds it and combines it with the rest of the program.
Step 5: Loading
The loader loads the executable file into memory, assigns memory addresses to variables and functions, and prepares the program for execution.
Step 6: Running
Once the program is loaded into memory, it’s ready to be executed by the processor, which follows the machine language instructions.
Modern Variations on the Classical Sequence
Integrated Development Environments (IDEs)
In modern IDEs, many of the classical steps are hidden. When you press "Run" in an IDE, the system compiles, links, and loads your code automatically. IDEs also provide additional features like syntax highlighting and version control integration, making them a powerful tool for developers.
Interpreters
In contrast to compilers, interpreters execute code directly without translating it into machine language. This makes them more flexible but slower since they process the code line by line. Python and JavaScript are examples of interpreted languages, although hybrid models that combine compilation and interpretation exist as well.
Virtual Machines (VMs)
Languages like Java compile code into an intermediate form known as bytecode, which is executed by a Virtual Machine (VM), such as the Java Virtual Machine (JVM). Virtual machines allow programs to run on any platform that has the VM installed, providing cross-platform compatibility and enhanced security.
Binding Times: When Things Get Connected
Binding refers to associating properties (like types or memory locations) with variables or functions. Different bindings occur at different stages of a program’s lifecycle:
Language Definition Time: Keywords and constructs are bound when the language is defined (e.g.,
for
,if
in C).Language Implementation Time: Some properties, like the range of values for an
int
, are set when the language system is built.Compile Time: Variables' types are typically bound at compile time in statically-typed languages like C.
Link Time: The addresses for external function calls are resolved when object files are linked.
Load Time: Memory locations for variables and functions are assigned when the program is loaded into memory.
Run Time: Variable values and dynamic properties are only determined during execution (e.g., in dynamically-typed languages like Python).
Early Binding occurs before the program runs (compile time or earlier), leading to faster execution, while Late Binding occurs at runtime, offering greater flexibility but often slower performance.
Debuggers: Hunting Down Bugs
Debuggers are essential tools for developers to find and fix bugs in their programs. A debugger allows you to:
Set Breakpoints: Pause program execution at specific lines.
Single-Step: Execute one line at a time to observe the program’s flow.
Inspect Variables: Check the values of variables during execution.
Traceback: View the sequence of function calls leading up to a specific point.
By using a debugger (e.g., gdb), developers can examine the state of a program, identify logic errors, and step through code to track down issues.
Runtime Support: Making Programs Run Smoothly
When a program is running, it relies on various runtime support systems to handle tasks like memory management and exception handling. Runtime support is usually included automatically by the language system, ensuring smooth execution of programs. Key aspects include:
Memory Management: Allocating and reclaiming memory as needed.
Exception Handling: Dealing with errors that occur during execution.
OS Interaction: Facilitating communication between the program and the operating system for tasks like file I/O or network access.
Delayed Linking and Dynamic Libraries
Many modern systems use delayed linking (also known as dynamic linking) to allow programs to share libraries and functions, improving efficiency. In Windows, these libraries are stored in .dll (Dynamic-Link Library) files, while in Unix-based systems, they are stored in .so (Shared Object) files.
Two Types of Dynamic Linking:
Load-Time Dynamic Linking: The necessary libraries are linked when the program is loaded into memory.
Run-Time Dynamic Linking: The program makes explicit calls to load libraries during execution.
Conclusion: Language Systems Behind the Scenes
Language systems manage the entire process of turning human-readable code into machine-executable programs. From compiling and linking to loading and running, these systems handle the complex task of transforming code into action. Whether you’re working with compiled languages, interpreted languages, or virtual machines, understanding the role of language systems is key to writing efficient and effective programs.
By mastering the concepts of binding times, debugging, and runtime support, you can take your programming skills to the next level and gain a deeper appreciation for the invisible systems that make coding possible.
Subscribe to my newsletter
Read articles from Dristanta Silwal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
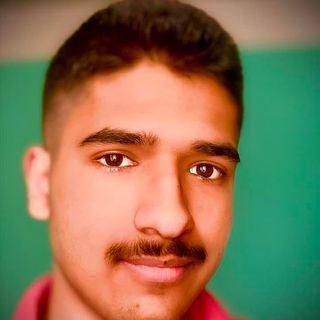
Dristanta Silwal
Dristanta Silwal
Bio: 🖥️ Computer Science Student | ✍️ Passionate Blogger 💡 Exploring the Intersection of Tech and Creativity 🎓 Currently pursuing a degree in Computer Science, I am a curious and driven student with a deep passion for all things technology. I am constantly seeking new avenues to expand my knowledge and skills in this ever-evolving field. 📚 As an aspiring computer professional, I am immersed in the world of programming languages, algorithms, and software development. However, my true excitement lies in blending my technical expertise with my creative spirit. ✏️ I have recently embarked on an exciting journey as a blogger, where I channel my love for writing to explore the fascinating world of technology, digital trends, and innovative ideas. Through my blog, I aim to share valuable insights, tutorials, and thought-provoking content that inspires others to embrace the wonders of the digital age. 🌐 When I'm not busy coding or crafting blog posts, you can find me tinkering with gadgets, experimenting with new software, or exploring the latest tech innovations. I'm always on the lookout for fresh perspectives and innovative ideas to incorporate into my work. 🤝 Let's connect and explore the limitless possibilities of the tech realm together! Feel free to reach out if you have any questions, collaboration opportunities, or just want to geek out over the latest trends. Let's shape the future of technology one blog post at a time! #ComputerScienceStudent #BloggingEnthusiast #TechGeek #CodeAndCreativity