Python's Memory Model (Reference Counting, Garbage Collection, and sys.getrefcount())
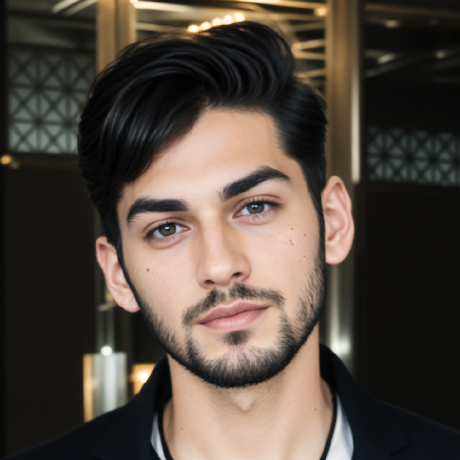
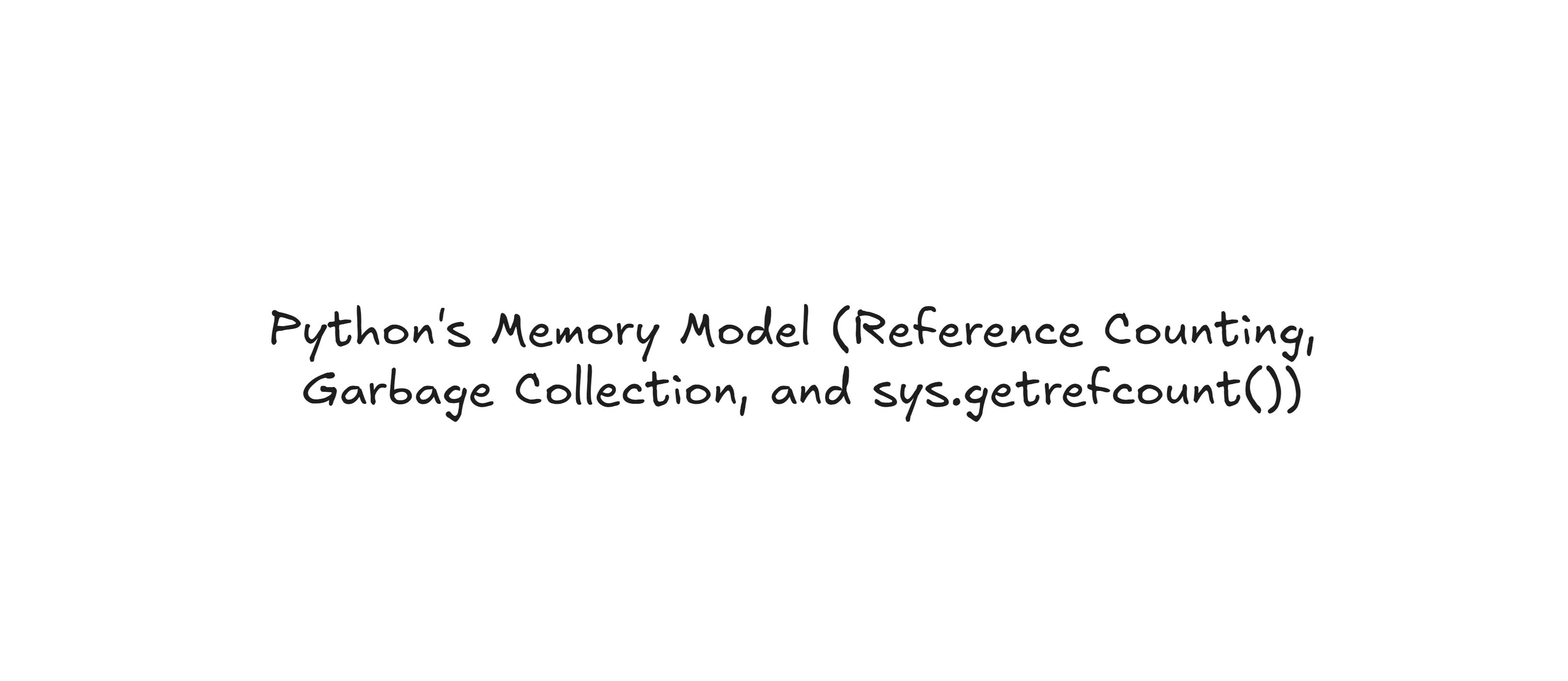
Introduction:
Have you ever wondered why Python seems so good at managing memory?
You happily create objects left and right, and somehow, when you’re done with them, they just disappear into the abyss. Well, the secret sauce behind Python’s memory management is a trio of interesting features: reference counting, garbage collection, and the sys.getrefcount()
function.
Imagine this: You’re throwing a party, and every guest brings a friend (or two). You’re constantly counting how many people are there, and as soon as they leave, you make sure to check if anyone else is left hanging around. Once everyone’s gone, you can relax and tidy up. Python’s memory model works in a similar way. Except, instead of leftover cups and confetti, it’s managing memory efficiently so your program doesn’t run out of resources. No one likes memory leaks, after all!
But here’s the twist. While the system is super helpful, many developers struggle to understand how Python actually manages memory under the hood. Is it magic? Is there a secret memory-cleaning gnome? Nope! It’s a bit more technical, but don’t worry—we’re diving in with humor and clarity, no magic wands needed.
Understanding Python’s Memory Model:
Python’s memory model isn’t just a simple allocation and deallocation mechanism. It uses reference counting as its primary technique, combined with an additional garbage collector to catch any “leftovers” that reference counting can’t handle.
Let’s break this down into three core concepts:
- Reference Counting
- Garbage Collection
- sys.getrefcount() (The unsung hero of debugging memory issues)
1. Reference Counting:
Python’s reference counting is like a watchful babysitter, constantly keeping track of how many hands (references) are reaching out for a toy (object).
In Python, every object has a reference count — that is, the number of places in your code that are referring to that object. Each time an object is referenced (e.g., assigned to a variable), its reference count increases. When the reference count drops to zero, Python immediately deallocates the object, freeing up memory.
How it Works:
- Create an Object: Reference count starts at 1.
- Assign to a Variable: The reference count increases.
- Delete a Reference: The reference count decreases.
- When Reference Count Hits Zero: The object is deleted.
[Object Creation]
↓
+-----------------+
| Ref Count = 1 |
+-----------------+
↓
[Object assigned to another variable]
↓
+-----------------+
| Ref Count + 1 |
+-----------------+
↓
[Reference removed (del or reassigned)]
↓
+-----------------+
| Ref Count - 1 |
+-----------------+
↓
[Reference count reaches 0 → Object deleted]
Code Example:
import sys
# Create an object
x = [1, 2, 3]
print(f"Initial reference count for x: {sys.getrefcount(x)}")
# Assign the object to another variable
y = x
print(f"Reference count after assignment to y: {sys.getrefcount(x)}")
# Delete a reference
del y
print(f"Reference count after deleting y: {sys.getrefcount(x)}")
# Once x is deleted, the object will be garbage collected.
del x
Explanation:
- Initially, the list
[1, 2, 3]
has a reference count of 1 when assigned tox
. - When assigned to
y
, the reference count increases to 2. - After
del y
, the reference count decreases to 1 again. - Finally, when
x
is deleted, the reference count drops to 0, and the list is removed from memory.
2. Garbage Collection:
Python’s garbage collector is like a ninja janitor that silently clears out hidden memory messes, especially when reference counting can’t do it alone.
While reference counting is Python’s main tool for memory management, it has a weakness — it can’t deal with circular references. A circular reference happens when two objects reference each other, but nothing else in the program references them. Even though they’re useless, their reference counts never drop to zero because they’re keeping each other alive!
Enter Python’s garbage collector, which specifically hunts down circular references.
How Garbage Collection Works:
- Python periodically runs a garbage collection cycle.
- During this cycle, it looks for objects that are no longer accessible (even if their reference counts aren’t zero).
- Python uses generational garbage collection, meaning objects are divided into generations, and older objects are checked less frequently.
[Circular Reference Detected]
↓
+---------------------+
| Collecting Garbage |
+---------------------+
↓
[Objects removed if inaccessible]
Code Example:
import gc
class CircularRef:
def __init__(self):
self.ref = None
# Create two objects with circular references
a = CircularRef()
b = CircularRef()
a.ref = b
b.ref = a
# Manually trigger garbage collection
gc.collect()
print("Circular references cleaned up by garbage collector.")
Explanation:
- Here,
a
referencesb
, andb
referencesa
. Even though their reference counts don’t hit zero, they are unreachable from the rest of the code, making them garbage. - The
gc.collect()
manually triggers Python’s garbage collector to clean up circular references.
3. Using sys.getrefcount()
:
sys.getrefcount()
is like a magnifying glass that lets you inspect how many fingers are holding onto your precious objects.
Sometimes, you want to understand why an object isn’t being cleaned up — maybe there’s a lingering reference somewhere, and you’re left scratching your head. This is where sys.getrefcount()
comes in.
How sys.getrefcount()
Works:
- This function returns the current reference count of an object.
- Keep in mind that the reference count is always at least 1 more than expected because Python holds a temporary reference to the object when calling
sys.getrefcount()
.
Flowchart:
[sys.getrefcount() called]
↓
+-------------------------+
| Returns object's ref count |
+-------------------------+
Code Example:
import sys
# Create a list object
my_list = [1, 2, 3]
# Check its reference count
ref_count = sys.getrefcount(my_list)
print(f"Reference count for my_list: {ref_count}")
# Add a new reference
another_ref = my_list
print(f"Reference count after another reference: {sys.getrefcount(my_list)}")
# Delete the new reference
del another_ref
print(f"Reference count after deleting another_ref: {sys.getrefcount(my_list)}")
Explanation:
sys.getrefcount()
shows the number of active references tomy_list
.- The reference count increases when
my_list
is assigned toanother_ref
. - After deleting
another_ref
, the reference count returns to its previous value.
Conclusion:
- You have mysterious memory leaks, but you don’t know why Python isn't freeing memory.
- Circular references are keeping your memory hostage.
- You’re left wondering how many references are dangling around your objects, refusing to let them die.
Understanding Python’s memory model — reference counting, garbage collection, and using sys.getrefcount()
— is key to mastering memory management and avoiding frustrating memory leaks in your code.
The next time your Python program feels bloated or starts hogging memory, don’t panic. Just remember, Python’s memory model is working hard behind the scenes to manage references and clean up garbage. But it’s not perfect — reference counting can’t handle circular references on its own. That’s where the garbage collector steps in like a superhero janitor, saving the day. And, if you’re ever in doubt about what’s going on, sys.getrefcount()
is your trusty sidekick to help you debug reference counts.
In short: learn to trust, but verify. Python does a fantastic job with memory management, but a little understanding goes a long way in making sure your code is memory-leak free and efficient!
Time to take control of your code’s memory and let Python's memory model do the heavy lifting.
Subscribe to my newsletter
Read articles from Akshay Parihar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
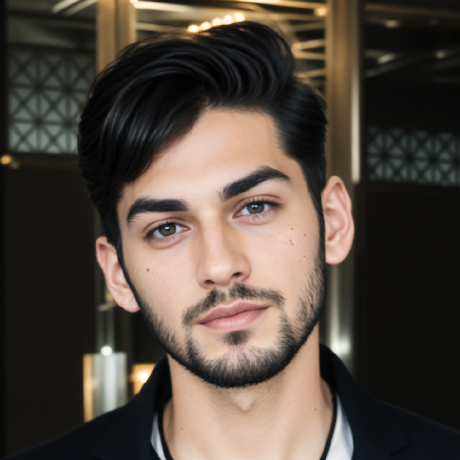