How to Develop Apps for Shopify App Store Using the Node.js Shopify App Template
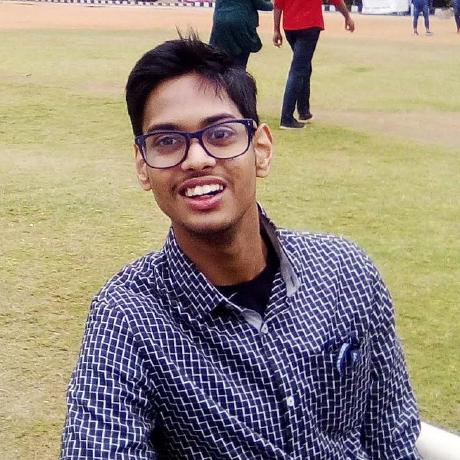
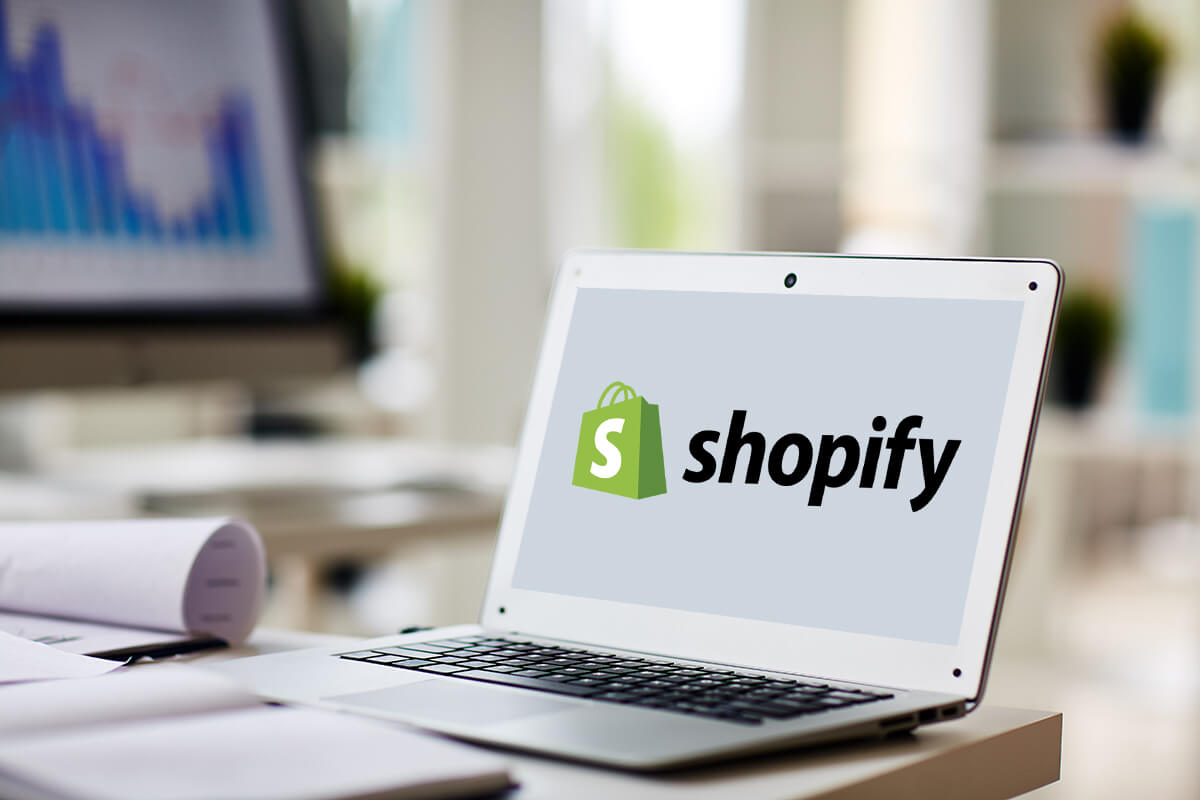
If you're a developer looking to build and deploy apps for the Shopify App Store, Shopify offers a Node.js app template to help you get started. The command npm init @shopify/app@latest -- --template=node
simplifies the setup process and gets your app environment ready for development in just a few minutes.
This guide will walk you through the steps to access the Node.js Shopify template using this command, develop an app, and prepare it for the Shopify App Store.
Prerequisites
Before starting, make sure you have the following installed:
Node.js (v14.0.0 or higher) and npm.
Shopify Partner account (to create and manage apps).
Development store in Shopify (to test your app).
ngrok (to expose your app's local server for Shopify to communicate with it securely).
Step 1: Shopify CLI Installation
To interact with Shopify’s tools, you’ll need to install the Shopify CLI, which simplifies app creation, authentication, and theme management.
Run the following command to install Shopify CLI globally:
Once installed, you’ll have access to the CLI commands needed to create, manage, and deploy your app.
npm install -g @shopify/cli @shopify/theme
Step 2: Create a Shopify App Using the Node.js Template
The Shopify CLI provides a convenient way to create new Shopify apps. To create a Node. js-based Shopify app, you will use the following command:
npm init @shopify/app@latest -- --template=node
This command will:
Set up a new Shopify app project using Node.js as the backend framework.
Install dependencies for Node.js and Express.js.
Include a basic structure for building out your app.
Step 3: Configure the Environment
After the command runs, you’ll have a new app project directory with all the necessary files. The next step is configuring your environment. Navigate into the project folder:
cd your-app-name
Then, locate and open the .env
file in the root of your project. This file contains crucial settings that allow your app to communicate with Shopify’s API, as well as other configuration details like your app’s URL and Shopify store.
Here’s a sample .env
file structure:
SHOPIFY_API_KEY=your_shopify_api_key
SHOPIFY_API_SECRET=your_shopify_api_secret
SCOPES=write_products,read_products
SHOP=your-development-store.myshopify.com
HOST=https://your-ngrok-url.ngrok.io
You can retrieve your SHOPIFY_API_KEY
and SHOPIFY_API_SECRET
from your Shopify Partner dashboard by creating an app there. The SHOP
variable should point to your Shopify development store, and the HOST
variable should be your ngrok URL, which exposes your local environment to Shopify.
Step 4: Install Dependencies and Start Development
Once the environment is configured, you’ll need to install the app’s dependencies. This can be done with the following npm command:
npm install
With the dependencies installed, you can start your development environment using:
npm run dev
This will launch your app locally, and ngrok will expose your app to a secure HTTPS URL, which is required for Shopify app communication.
Step 5: Authenticate with Shopify
The Node.js Shopify app template comes with built-in authentication using Shopify’s OAuth. This makes it easy to integrate your app with a Shopify store for development.
When you run your app for the first time, visit the ngrok URL, and the app will prompt you to install it on your Shopify development store. After authentication, Shopify will generate an access token for your app, allowing it to make API calls on behalf of the store.
Step 6: App Structure Overview
The Node.js Shopify app template follows a well-organized structure that helps you easily build scalable apps. The main components include:
Backend (Express.js): Your app’s backend is built with Express.js. You can find and modify the server-side logic in the
server.js
file.Frontend (React.js + Polaris): The app's admin UI is built using React.js and Shopify Polaris, which offers pre-built components for a native Shopify experience.
GraphQL/REST APIs: The app template provides examples of making API requests to Shopify using REST and GraphQL endpoints.
/app
/client
/server
/components
/pages
/app/client
: Contains the React.js code for the front end./app/server
: Includes the backend server logic using Express.js./components
: Reusable components for the frontend built using React./pages
: Define the routes and pages in your app.
Step 7: Customizing Your App
With your app set up and running, you can now begin customizing it. Whether you're adding features, modifying the user interface, or integrating more API calls, the template gives you a foundation for fast, iterative development.
For example, if you want to add a new route in your Express backend to fetch products from the Shopify store, you could do so like this in server.js
app.get('/products', async (req, res) => {
try {
const session = await Shopify.Utils.loadCurrentSession(req, res);
const client = new Shopify.Clients.Rest(session.shop, session.accessToken);
const products = await client.get({ path: 'products' });
res.status(200).send(products);
} catch (error) {
res.status(500).send(error);
}
});
Step 8: Testing Your App
Shopify provides tools for testing your app before submitting it to the App Store. Once your app runs on your development store, you should extensively test all the features.
Ensure you test:
OAuth flow: The app installation and uninstallation process.
API interactions: Reading, writing, and updating store data.
Billing integration: If you’re charging for your app, test Shopify’s Billing API to ensure that merchants are correctly billed.
Step 9: Deploy Your App
Once you’ve tested your app thoroughly and it's ready for production, you can deploy it to a hosting provider. Shopify requires that your app is hosted on a secure HTTPS domain, and some popular hosting services include:
Heroku: Great for deploying Node.js apps quickly.
Vercel: Ideal for full-stack apps with serverless functions.
AWS: Use services like AWS Elastic Beanstalk or EC2 for scalable deployments.
Once your app is hosted, update the HOST
in your .env
file to point to your production URL.
Step 10: Submit to the Shopify App Store
When your app is live and working as intended, the next step is to submit it to the Shopify App Store.
Log in to the Shopify Partner Dashboard.
Fill out the App Store listing information: Add a detailed description, app features, pricing, and screenshots.
Submit for review: Shopify’s review process may take a few days to weeks, depending on the complexity of your app.
Prepare for feedback: Shopify might ask for changes or improvements before publishing your app.
Conclusion
Using npm init @shopify/app@latest -- --template=node
is a quick and efficient way to set up a Node.js app for Shopify. It streamlines the development process by providing all the necessary tools and a well-structured app template, allowing you to focus on building features and scaling your app. Once you’ve developed your app, tested it thoroughly, and deployed it, you’ll be ready to submit it to the Shopify App Store and start helping Shopify merchants enhance their stores.
Subscribe to my newsletter
Read articles from Anish Agrawal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
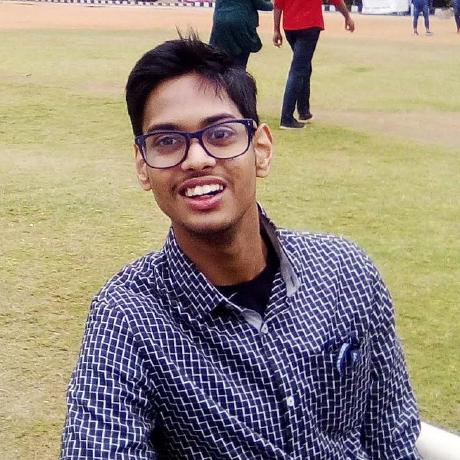