Implement an Abstract Class in Go!
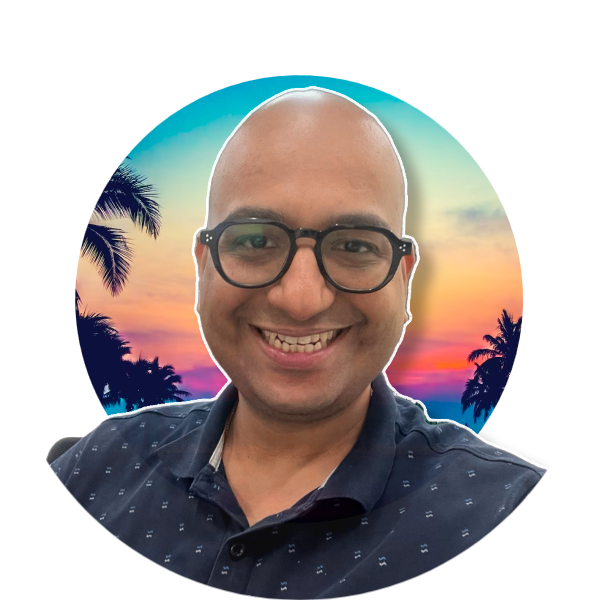
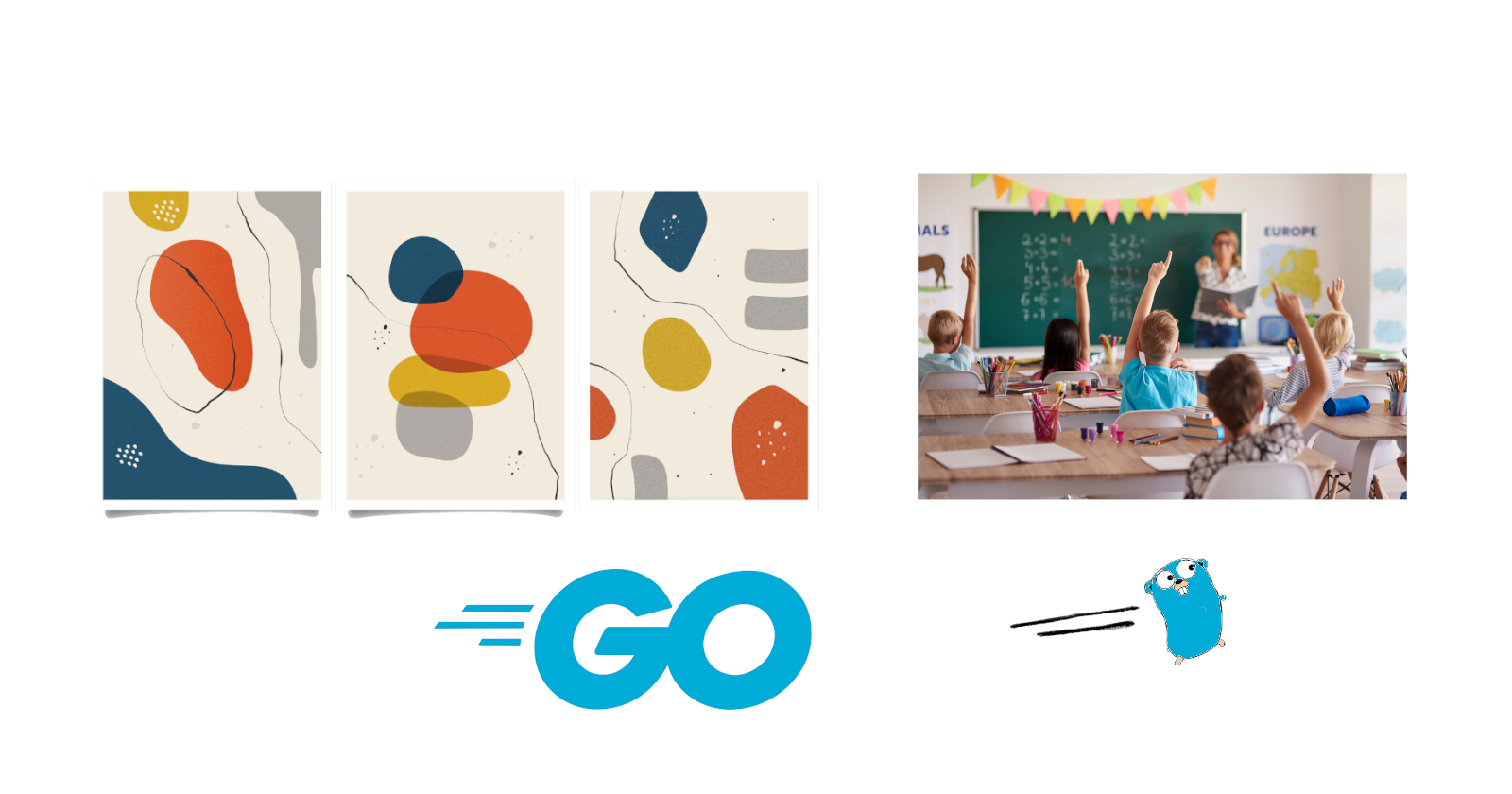
Well in Go, there is no direct equivalent of Python's abstract classes, but you can achieve similar functionality using interfaces and struct embedding. Here's how you can replicate the behavior of an abstract class in Go.
Python Abstract Class Example:
from abc import ABC, abstractmethod
class Animal(ABC):
@abstractmethod
def make_sound(self):
pass
class Dog(Animal):
def make_sound(self):
return "Woof"
Go Equivalent Using Interfaces and Struct Embedding:
In Go, you can define an interface to represent the abstract methods and use struct embedding to simulate shared behavior.
Define the interface to represent the abstract method.
Use structs to implement that interface.
Here’s an equivalent example in Go:
package main
import "fmt"
// Define an interface similar to an abstract method
type Animal interface {
MakeSound() string
}
// Define a struct representing the base class (optional if you need shared fields)
type BaseAnimal struct {
// you can add fields common to all animals here
}
// Define another struct implementing the abstract method
type Dog struct {
BaseAnimal // Embedding BaseAnimal struct to inherit shared fields (if any)
}
// Implement the interface method for Dog
func (d Dog) MakeSound() string {
return "Woof"
}
func main() {
var animal Animal = Dog{}
fmt.Println(animal.MakeSound()) // Output: Woof
}
Key Concepts:
Animal
interface is equivalent to the abstract class method in Python.BaseAnimal
struct is used if you need shared fields or behavior, similar to a base class in Python (though it's not necessary if you're only using abstract methods).Struct
Dog
implements theAnimal
interface by providing theMakeSound()
method, similar to a subclass overriding an abstract method in Python.
Summary
In Go, interfaces define abstract methods, and structs implement those methods. You can combine them with struct embedding to share common functionality across different types.
References
https://gobyexample.com/interfaces
https://www.alexedwards.net/blog/interfaces-explained
Image Attribution
Subscribe to my newsletter
Read articles from Nikhil Akki directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
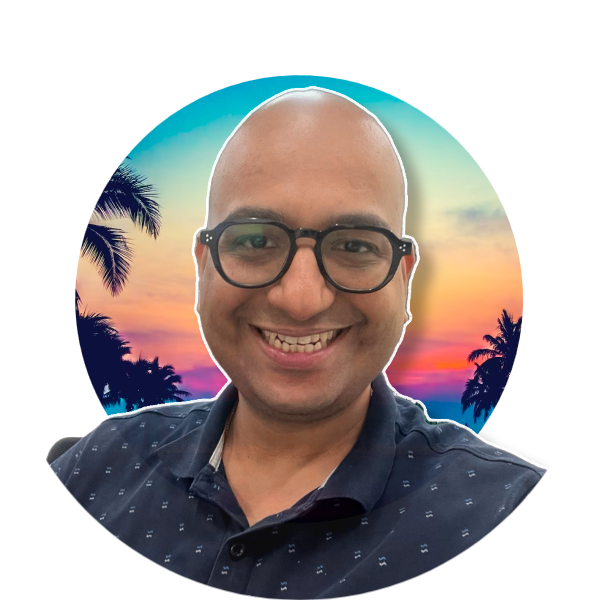
Nikhil Akki
Nikhil Akki
I am a Full Stack Solution Architect at Deloitte LLP. I help build production grade web applications on major public clouds - AWS, GCP and Azure.