Understanding Python Functions: Day 16 Summary

Table of contents
- Introduction :
- core python :
- FUNCTION :
- . syntax :
- . syntax :
- example :
- MAIN FUNCTION IN PYTHON ~
- HOW TO EXECUTE A FUNCTION IN PYTHON ( HOW TO CALL ) :
- example :
- CONTROL FLOW DURING FUNCTION DEF AND CALL ~~~~~~~~~~
- GLOBAL VARIABLE : ~
- LOCAL VARIABLE : ~
- Example :
- global keyword mechanism
- argument or parameter mechanism
- Challenges :
- Resources :
- Goals for Tomorrow :
- Conclusion :
- Connect with me :
- Join the conversation :
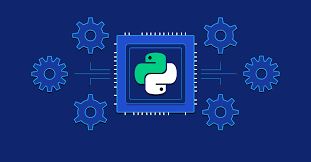
Introduction :
Welcome back to my Python journey! Yesterday, I laid the foundation with control structures in python.
Today, I dove into Functions, Let's explore what I learned!
core python :
introduction
operators
data types
control structure
control statement
FUNCTION :
~~~~ WHAT IS FUNCTION \~~~ .
function is collection of expression and statements.
HOW TO DEFINE A FUNCTION IN PYTHON
. to define a function in python
def
keyword we have to use.. to define the body of a function in python INDENTATION mechanism will be used.
. to define empty body we can use pass control statement.
. syntax :
def FunctionName ( ) :
body
. syntax :
def FunctionName ( ) :
pass
. the name which is used to represent a function is known as IDENTIFIER.
. function name is depends on user as per the demand of situation.
example :
def Test() : #definition
print ("i am fun")
MAIN FUNCTION IN PYTHON ~
. in c cpp java program starts execution from main function.
. main function defination is compulsory in c cpp java.
. main is a special function in c cpp java.
. in python main is an ordinary function.
. in python program does not starts execution from main.
. main function defination is not compulsory in python.
HOW TO EXECUTE A FUNCTION IN PYTHON ( HOW TO CALL ) :
. function definition is not sufficient to execute a function.
. to execute a function, with definition, call is required.
. until function is not called, that function is not going to execute.
. FUNCTION def MUST BE AVALIABLE BEFORE THE FUNCTION CALL, due to python is an interpreted programming language.
. if we call a function N times that function will execute N times.
example :
def Test() : #defination
print ("i am fun")
Test () #calling
CONTROL FLOW DURING FUNCTION DEF AND CALL ~~~~~~~~~~
def main () : # stmt-1
print ( 'i am main !') # stmt-2
bbsr () # stmt-3
def bbsr () : # stmt-4
print ( 'i am bbsr !') # stmt-5
main () # stmt-6
CONTROL FLOW : Statement 1 -> 4 -> 6 -> 2 -> 3 -> 5
while working with function variable is basically two types in python,
. GLOBAL VARIABLE
. LOCAL VARIABLE
GLOBAL VARIABLE : ~
. if a variable is not belongs to any function that var is known as global var.
. global var is not belongs to any function but any function can enjoy global var.
var = 10
def main () :
print ( 'i am main enjoying ' , var )
bbsr ()
def bbsr () :
print ( 'i am bbsr enjoying ', var )
. any function can enjoy global var, but can not modify the global var.
. if we try to modify the global var from a function it will raise UnboundLocalError exception.
var = 10
def main () :
var = var + 10 # modify the global data
print ( 'i am main enjoying ' , var )
bbsr ()
def bbsr () :
print ( 'i am bbsr enjoying ', var )
. to avoid UnboundLocalError exception while modifying the global var we have use
global
keyword.. if a function modified the global var that modification is going to reflect in other function also.
var = 10
def main () :
global var
var = var + 10 # modify the global data
print ( 'i am main enjoying ' , var )
bbsr ()
def bbsr () :
print ( 'i am bbsr enjoying ', var )
LOCAL VARIABLE : ~
. the var which is belongs to a function is known as local var.
. local var of a function can only be enjoyed in that function where it is local, not from other function.
. if we try to enjoy the local var of a function in another function it will raise NameError exception.
Example :
def main () :
var = 10
print ( 'i am main enjoying ', var )
bbsr ()
def bbsr () :
print ( 'i am bbsr enjoying ', var )
main ()
. to avoid NameError exception while enjoying the local var of one function in another function there are two different mechanisms are avaliable in python,
. GLOBAL KEYWORD MECHANISM
. ARGUMENT OR PARAMETER MECHANISM ( most preferable )
global keyword mechanism
def main () :
global var
var = 10
print ( 'i am main enjoying ', var )
bbsr ()
def bbsr () :
print ( 'i am bbsr enjoying ', var , 'by global keyword mechanism !')
main ()
argument or parameter mechanism
def main () :
var = 10
print ( 'i am main enjoying ', var )
bbsr ( var )
def bbsr ( var ) :
print ( 'i am bbsr enjoying ', var , 'by argument or param mechanism!')
main ()
Challenges :
Understanding global and local variable.
Handling errors.
Resources :
Official Python Documentation: Functions
W3Schools' Python Tutorial: Functions
Scaler's Python Course: Functions
Goals for Tomorrow :
Explore something more on Functions.
Conclusion :
Day 16’s a success!
What are your favorite Python resources? Share in the comments below.
Connect with me :
GitHub: [ https://github.com/p-archana1 ]
LinkedIn : [ https://www.linkedin.com/in/archana-prusty-4aa0b827a/ ]
Join the conversation :
Share your own learning experiences or ask questions in the comments.
HAPPY LEARNING!!
THANK YOU!!
Subscribe to my newsletter
Read articles from Archana Prusty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Archana Prusty
Archana Prusty
I'm Archana, pursuing Graduation in Information technology and Management. I'm a fresher with expertise in Python programming. I'm excited to apply my skills in AI/ML learning , Python, Java and web development. Looking forward to collaborating and learning from industry experts.