React.FC In typescript

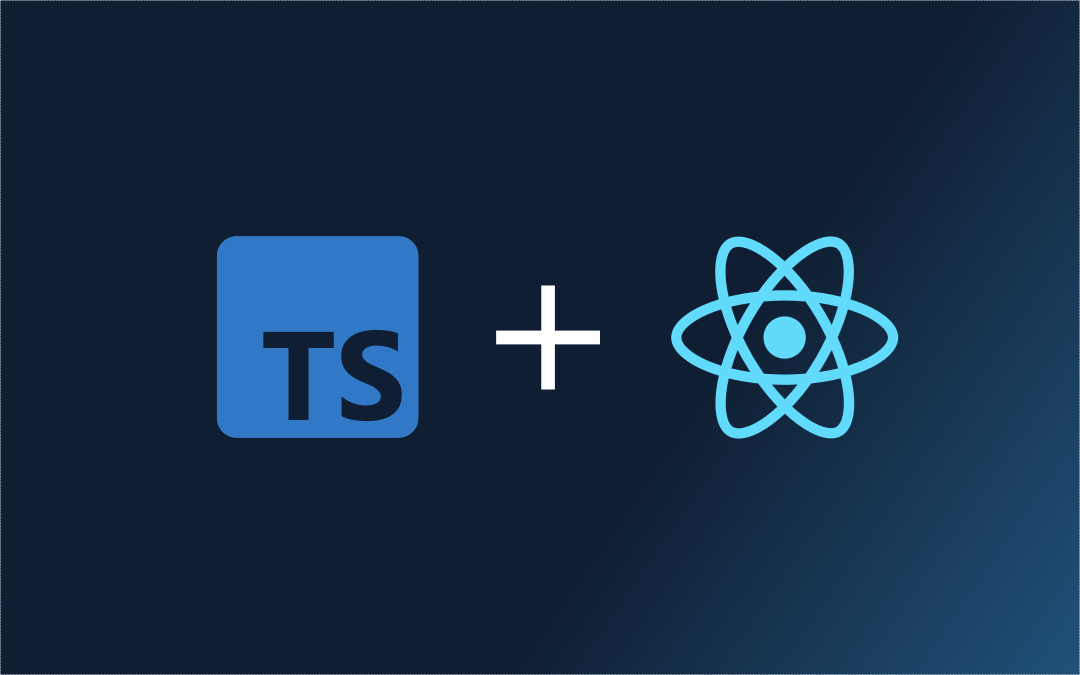
React.FC stands for "Function Component" in React and allows you to specify the props that a function component will accept.
It can be helpful in many ways
Makes it easier to see the expected shape of a component's props just by looking at its type definition.
Provides type safety for the props that a component expects to receive. This can help catch bugs early on and make your code easier to understand.
Makes easier to refactor component's props. For example, if you rename a prop, typeScript compiler will catch any places where that prop is used and help you update them.
An example of how to use React.FC:
import React from 'react';
interface Props {
name: string;
age: number;
onClick: () => void;
}
const MyComponent: React.FC<Props> = ({ name, age, onClick }) => {
return (
<div>
<h1>{name}</h1>
<p>Age: {age}</p>
<button onClick={onClick}>Click me</button>
</div>
);
};
MyComponent.defaultProps = {
name: 'Parveen',
age: 35,
onClick: () => {},
};
Subscribe to my newsletter
Read articles from Parveen Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Parveen Kumar
Parveen Kumar
I am a Full-stack MERN engineer with a product centric approach, specializing in the design and development of scalable, reliable, production ready web application, I bring expertise in JavaScript, React, and Node Js. Get in touch! paulparveen01@gmail.com