Chapter 8: Operators, Conditionals, and Switch Case in Java
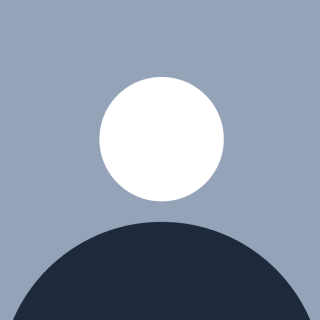
Java offers a wide variety of operators, which allow you to perform operations on variables, manipulate data, and control program flow. We will explore different types of operators and understand their behavior.
1. Operators in Java:
Operators are special symbols that perform operations on variables and values. Java provides a rich set of operators categorized into different types based on their functionality.
i) Arithmetic Operators:
Arithmetic operators perform basic mathematical operations like addition, subtraction, multiplication, and division.
Operator | Description | Example |
+ | Addition | a + b (adds a and b ) |
- | Subtraction | a - b (subtracts b from a ) |
* | Multiplication | a * b (multiplies a and b ) |
/ | Division | a / b (divides a by b ) |
% | Modulus (remainder of division) | a % b (remainder when a is divided by b ) |
Example:
int a = 15; int b = 4; System.out.println("Addition: " + (a + b)); // Output: 19 System.out.println("Subtraction: " + (a - b)); // Output: 11 System.out.println("Multiplication: " + (a * b)); // Output: 60 System.out.println("Division: " + (a / b)); // Output: 3 (integer division) System.out.println("Modulus: " + (a % b)); // Output: 3 (remainder when 15 is divided by 4)
Key Points:
Division
/
returns the quotient, and for integer division, it ignores any remainder.Modulus
%
returns the remainder of the division.
ii) Increment and Decrement Operators:
These are unary operators (operators that work on a single operand) used to increase or decrease the value of a variable by 1.
Operator | Description | Example |
++ | Increment (increases by 1) | a++ or ++a |
-- | Decrement (decreases by 1) | a-- or --a |
There are two types of increment/decrement:
Pre-Increment (
++a
) / Pre-Decrement (--a
): The value is incremented/decremented before it is used in the expression.Post-Increment (
a++
) / Post-Decrement (a--
): The value is used in the expression first, and then it is incremented/decremented.
Example:
int x = 10; int y = ++x; // Pre-increment: x becomes 11, y is assigned 11. int z = x--; // Post-decrement: z is assigned 11, then x becomes 10. System.out.println("x: " + x); // Output: 10 System.out.println("y: " + y); // Output: 11 System.out.println("z: " + z); // Output: 11
iii) Logical Operators:
Logical operators are used to evaluate two or more conditions/expressions and return a Boolean result (true
or false
). They are mainly used in decision-making statements like if
, while
, for
.
Operator | Description | Example |
&& | Logical AND (returns true if both conditions are true) | a && b |
` | ` | |
! | Logical NOT (reverses the condition) | !a (if a is true , it returns false ) |
1) AND Operator (&&
):
The AND operator checks whether both the conditions are true
. If both are true, it returns true
; otherwise, it returns false
.
Truth Table:
| A | B | A && B | | --- | --- | --- | | T | T | T | | T | F | F | | F | T | F | | F | F | F |
Example:
int a = 10; int b = 20; boolean result = (a > 5) && (b < 30); // true && true = true System.out.println(result); // Output: true
2) OR Operator (||
):
The OR operator returns true
if at least one of the conditions is true
. It returns false
only if both conditions are false
.
Example:
int a = 5; int b = 15; boolean result = (a < 10) || (b > 20); // true || false = true System.out.println(result); // Output: true
3) NOT Operator (!
):
The NOT operator reverses the result of the condition. If the condition is true
, it returns false
, and vice versa.
Example:
boolean flag = true; System.out.println(!flag); // Output: false
iv) Relational Operators:
Relational operators are used to compare two values. They return a Boolean result (true
or false
) based on the comparison.
Operator | Description | Example |
== | Equal to (checks if two values are equal) | a == b |
!= | Not equal to (checks if two values are not equal) | a != b |
< | Less than | a < b |
> | Greater than | a > b |
<= | Less than or equal to | a <= b |
>= | Greater than or equal to | a >= b |
Example:
int a = 10; int b = 20; System.out.println(a == b); // Output: false System.out.println(a != b); // Output: true System.out.println(a < b); // Output: true System.out.println(a > b); // Output: false
Key Points:
==
checks if two values are equal.!=
checks if two values are not equal.<
,>
,<=
, and>=
are used for numerical comparisons.
v) Assignment Operators:
Assignment operators are used to assign values to variables. The most common assignment operator is =
, but Java also supports compound assignment operators.
1) Basic Assignment (=
):
Assigns the value on the right-hand side to the variable on the left-hand side.
int a = 10;
2) Chained Assignment:
Allows you to assign the same value to multiple variables in one line.
int a, b, c; a = b = c = 10;
3) Compound Assignment:
Provides a shorter syntax for performing arithmetic or bitwise operations and assignment in one step.
Example:
int a = 5; a += 2; // Equivalent to: a = a + 2; a becomes 7 a -= 3; // Equivalent to: a = a - 3; a becomes 4 a *= 2; // Equivalent to: a = a * 2; a becomes 8
Unary Operator in Java:
A unary operator is an operator that works with a single operand (a variable or value). Unary operators are typically used to perform operations such as incrementing/decrementing a value, changing the sign of a number, or logically inverting a boolean value.
- Definition: Unary operators are the type of operators that need only one operand to perform an operation. Examples include increment (
++
), decrement (--
), and negation (-
).
Types of Unary Operators:
Increment Operator (
++
):Increases the value of a variable by 1.
Can be used as pre-increment (
++a
) or post-increment (a++
).
Decrement Operator (
--
):Decreases the value of a variable by 1.
Can be used as pre-decrement (
--a
) or post-decrement (a--
).
Negation Operator (
-
):- Changes the sign of a number (positive to negative, or negative to positive).
Logical NOT Operator (
!
):- Inverts the truth value of a boolean expression (true becomes false, false becomes true).
Examples:
int x = 10; int y = -x; // Negation operator: changes 10 to -10 System.out.println(y); // Output: -10 boolean flag = true; System.out.println(!flag); // Output: false (Logical NOT operator) int a = 5; a++; // Post-increment: increases the value of a by 1 System.out.println(a); // Output: 6
Key Points:
Unary operators work on a single operand.
Increment and decrement operators have both pre and post variations, with slightly different behavior.
The negation operator changes the sign of numeric values.
Binary Operator in Java:
A binary operator is an operator that operates on two operands. These operators are widely used for performing arithmetic, comparison, and logical operations.
- Definition: Binary operators require two operands to perform any operation. The operation results in a value of the same type as the operands. For example, arithmetic operators like addition (
+
) or comparison operators like greater-than (>
) operate on two operands.
Types of Binary Operators:
Arithmetic Operators:
- Addition (
+
), Subtraction (-
), Multiplication (*
), Division (/
), and Modulus (%
).
- Addition (
Relational Operators:
- Used to compare two values: Equal (
==
), Not Equal (!=
), Greater Than (>
), Less Than (<
), Greater Than or Equal To (>=
), Less Than or Equal To (<=
).
- Used to compare two values: Equal (
Logical Operators:
- Logical AND (
&&
), Logical OR (||
): Used in conditional statements to evaluate multiple conditions.
- Logical AND (
Assignment Operators:
- Basic assignment (
=
), Compound assignment (+=
,-=
,*=
,/=
, etc.).
- Basic assignment (
Examples:
// Arithmetic example int a = 10; int b = 20; int sum = a + b; // Binary operator '+' performs addition between a and b System.out.println(sum); // Output: 30 // Compound assignment example a += 20; // Equivalent to: a = a + 20; System.out.println(a); // Output: 30 // Comparison example System.out.println(a > b); // Output: false (Binary comparison operator '>')
Key Points:
Binary operators always require two operands.
They can perform operations such as arithmetic, relational comparisons, and logical evaluations.
Compound assignment operators combine arithmetic and assignment in a single step, e.g.,
a += 10
meansa = a + 10
.
Unary vs. Binary Operators:
Category | Unary Operators | Binary Operators |
Number of Operands | Operate on one operand | Operate on two operands |
Common Operators | ++ , -- , - , ! | + , - , * , / , % , > , < , == , != , etc. |
Example | int a = 5; a++; (Unary increment) | int sum = a + b; (Binary addition) |
Corrected Example:
int a = 10;
a += 20; // This is a binary operator (compound assignment)
a++; // This is a unary operator (post-increment)
a += 20
is a binary operator because it involves two operands:a
and20
.a++
is a unary operator because it operates on the single operanda
.
Introduction to Conditional Operators:
Conditional operators in Java allow the program to make decisions and execute code blocks based on certain conditions. They help in controlling the flow of the program by checking if an expression is true or false. There are several ways to implement conditions in Java, such as:
if-else statement
else if ladder
nested if statements
ternary operator
switch statement
By using these conditional statements, you can execute specific sections of code depending on whether a condition evaluates to true or false.
1) if-else
Statement:
The if-else
statement checks a condition, and if it is true, the block inside the if
statement is executed. If it is false, the block inside the else
statement is executed.
Example: Finding the Minimum of Two Numbers:
int a = 10;
int b = 5;
if (a < b) {
System.out.println("a is the minimum number"); // Output: a is the minimum number
} else {
System.out.println("b is the minimum number");
}
In this example, the condition a < b
is true, so the if
block is executed, printing that a
is the minimum number.
2) else if
Ladder:
The else if
ladder is used when you need to check multiple conditions. The program evaluates each condition in sequence, and when one condition is true, its corresponding block is executed. If none of the conditions is true, the else
block is executed.
Example: Finding the Minimum of Three Numbers:
int a = 10;
int b = 20;
int c = 5;
if (a < b && a < c) {
System.out.println("a is the minimum number"); // Output: a is the minimum number
} else if (b < a && b < c) {
System.out.println("b is the minimum number");
} else {
System.out.println("c is the minimum number");
}
In this example, a
is less than both b
and c
, so the first condition a < b && a < c
is true, and the message "a is the minimum number" is printed.
3) Nested if
Statements:
A nested if
statement is when an if
or else if
statement is placed inside another if
or else
block. This structure allows checking conditions in a more hierarchical way, where the inner if
is executed only if the outer if
condition is true.
Example: Nested if
for Minimum of Three Numbers:
int a = 10;
int b = 20;
int c = 5;
if (a < b) {
if (a < c) {
System.out.println("a is the minimum number"); // Output: a is the minimum number
} else {
System.out.println("c is the minimum number");
}
} else {
if (b < c) {
System.out.println("b is the minimum number");
} else {
System.out.println("c is the minimum number");
}
}
Here, the outer if
checks if a < b
. Since this is true, the inner if
checks if a < c
, which is also true, so the program prints "a is the minimum number."
4) Ternary Operator:
The ternary operator is a shorthand form of the if-else
statement and provides a more concise way to make a decision between two values based on a condition. It takes three operands: a condition, the value if the condition is true, and the value if the condition is false.
Syntax:
result = (condition) ? value_if_true : value_if_false;
Example: Minimum of Two Numbers Using Ternary Operator:
int a = 10;
int b = 5;
int min = (a < b) ? a : b; // If a is less than b, min = a; otherwise, min = b
System.out.println("Minimum number is: " + min); // Output: Minimum number is: 5
In this case, since a < b
is false, the value of b
is assigned to min
.
Example: Minimum of Three Numbers Using Ternary Operator:
int a = 10, b = 20, c = 5;
int min = (a < b && a < c) ? a : (b < c) ? b : c;
System.out.println("Minimum number is: " + min); // Output: Minimum number is: 5
This example first checks if a
is less than both b
and c
. If true, it assigns a
as the minimum. If not, it compares b
and c
, and the smaller value is assigned.
5) switch
Statement:
The switch
statement allows you to execute one block of code out of multiple possibilities based on the value of a variable. It is especially useful when you have a number of conditions to check based on the same variable.
Syntax:
switch (expression) { case value1: // Code for value1 break; case value2: // Code for value2 break; // Add more cases if needed default: // Code if no case matches }
Example: Finding Day of the Week:
int day = 2;
switch (day) {
case 1:
System.out.println("Sunday");
break;
case 2:
System.out.println("Monday"); // Output: Monday
break;
case 3:
System.out.println("Tuesday");
break;
default:
System.out.println("Invalid day");
}
In this example, since day
equals 2, the program prints "Monday". If no cases match, the default
block is executed.
Break Statement:
The break
statement is crucial in a switch
to terminate the switch block after the matching case is executed. If the break
is omitted, the program continues executing the next cases even if they don't match.
Example Without break
:
int day = 2;
switch (day) {
case 1:
System.out.println("Sunday");
case 2:
System.out.println("Monday"); // Output: Monday
case 3:
System.out.println("Tuesday"); // Output: Tuesday
default:
System.out.println("Invalid day"); // Output: Invalid day
}
In this example, after matching case 2
, all subsequent cases are executed due to the absence of break
statements.
Default Case:
If no cases match the value of the expression, the default
block is executed. If there is no default
block, no action is taken if no cases match.
This is how conditional operators like if-else
, else if
, nested if
, ternary, and switch
statements can be used to control the flow of a Java program. Each has its own use case, depending on the complexity and number of conditions being checked.
Summary: Operators, Conditionals, and Switch Case in Java
Operators in Java: Java provides a variety of operators to perform different tasks, such as arithmetic, logical operations, comparisons, and bitwise manipulations.
Arithmetic Operators: Used for basic math operations like addition (
+
), subtraction (-
), multiplication (*
), division (/
), and modulus (%
).Increment/Decrement Operators: Increase (
++
) or decrease (--
) a value by 1.Logical Operators: Used for combining conditions:
&&
(AND) β True if both conditions are true.||
(OR) β True if at least one condition is true.!
(NOT) β Inverts the truth value.
Relational Operators: Compare two values:
==
(equals),!=
(not equal),<
,>
,<=
,>=
.
Assignment Operators: Assign values to variables (
=
) and include compound assignment (+=
,-=
, etc.).Unary Operators: Operate on one operand (e.g.,
++
,--
).Binary Operators: Operate on two operands (e.g.,
+
,-
,*
).Conditional (Ternary) Operator: Evaluates conditions and assigns values based on the result
(condition) ? valueIfTrue : valueIfFalse
.Bitwise and Shift Operators: Perform operations on individual bits (
&
,|
,^
,<<
,>>
).
This chapter introduces how these operators are used in Java to build logical statements, manipulate data, and perform arithmetic and relational operations.
See My Other Related Posts:
Chapter 7: Data Types Continuation
In this chapter, I explore the various data types in Java in greater depth, including primitive and non-primitive types, memory allocations, and the differences between value and reference types.Chapter 6: Classes and Identifiers
This chapter provides an in-depth understanding of classes and identifiers in Java. I explain how classes form the foundation of object-oriented programming (OOP), the structure of a class, and the importance of constructors, methods, and fields. You'll also learn about Java naming conventions for identifiers and their role in improving the readability and maintainability of the code. Examples include defining classes, creating objects, and working with constructors.
See My Other Series:
Full Stack Java Development
This series is dedicated to mastering the full stack of Java development, from basic Java programming to advanced concepts like Spring Framework, Hibernate, RESTful APIs, microservices, and front-end integration. Whether you're a beginner or an intermediate learner, this series covers everything needed to build dynamic web applications and deploy them on the cloud.Full Stack JavaScript Development
In this series, I cover both client-side and server-side development using JavaScript. Starting with the fundamentals like ES6 features, DOM manipulation, and asynchronous programming, I progress to modern front-end frameworks like React, Angular, and Vue. Additionally, I dive into Node.js, Express, and MongoDB for building scalable back-end applications.Big Data BootCamp
This series focuses on big data technologies and tools. From understanding the Hadoop ecosystem to working with Spark, Hive, and Kafka, you'll learn how to process and analyze large datasets efficiently. This boot camp is ideal for those looking to build careers in data engineering and analytics.
Connect with Me on Social Media:
GitHub: Check out my repositories and coding projects on GitHub to see what I've been working on. I regularly update my repositories with Java, JavaScript, and DSA projects.
LinkedIn: Connect with me on LinkedIn to stay updated on my latest professional activities, coding challenges, and blog posts. I also share insights on Full Stack Development and Data Structures.
LeetCode: Follow me on LeetCode to track my progress in solving data structure and algorithm challenges. You can check out my solutions, coding practices, and competitive programming journey.
Subscribe to my newsletter
Read articles from Rohit Gawande directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Rohit Gawande
Rohit Gawande
π Tech Enthusiast | Full Stack Developer | System Design Explorer π» Passionate About Building Scalable Solutions and Sharing Knowledge Hi, Iβm Rohit Gawande! πI am a Full Stack Java Developer with a deep interest in System Design, Data Structures & Algorithms, and building modern web applications. My goal is to empower developers with practical knowledge, best practices, and insights from real-world experiences. What Iβm Currently Doing πΉ Writing an in-depth System Design Series to help developers master complex design concepts.πΉ Sharing insights and projects from my journey in Full Stack Java Development, DSA in Java (Alpha Plus Course), and Full Stack Web Development.πΉ Exploring advanced Java concepts and modern web technologies. What You Can Expect Here β¨ Detailed technical blogs with examples, diagrams, and real-world use cases.β¨ Practical guides on Java, System Design, and Full Stack Development.β¨ Community-driven discussions to learn and grow together. Letβs Connect! π GitHub β Explore my projects and contributions.πΌ LinkedIn β Connect for opportunities and collaborations.π LeetCode β Check out my problem-solving journey. π‘ "Learning is a journey, not a destination. Letβs grow together!" Feel free to customize or add more based on your preferences! π