Strings in Data Structures: Essential Information and Tips

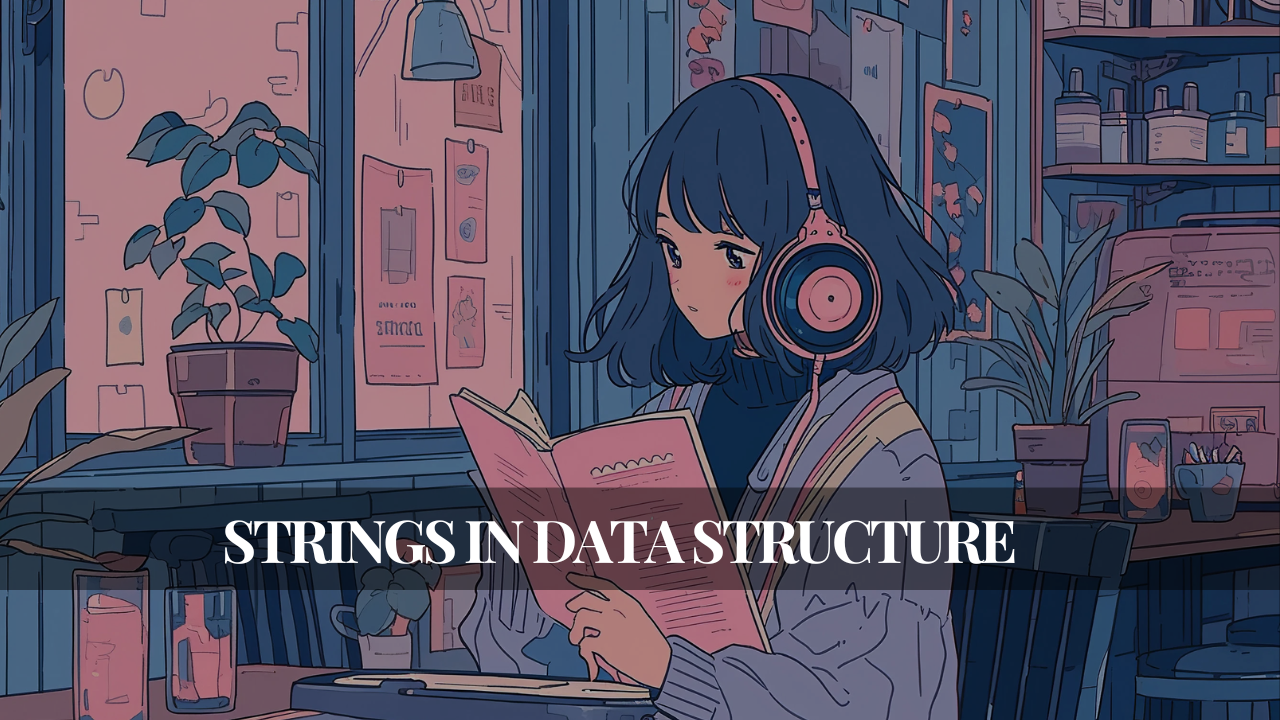
Strings are a fundamental data structure in programming that plays a crucial role in handling text-based data. From user inputs and file handling to data processing and representation, strings are essential in virtually every application. They provide a means to store and manipulate sequences of characters, enabling developers to perform a wide range of operations efficiently. Understanding strings is vital for anyone delving into programming, as they form the backbone of data manipulation and processing in numerous algorithms and applications. In this article, we will explore the concept of strings, their properties, operations, and applications in Data Structures and Algorithms.
What is a String?
A string is a sequence of characters enclosed in quotes, either single ('
) or double ("
). Strings can contain letters, digits, symbols, and whitespace, making them versatile for representing textual data. In many programming languages, including Python, Java, and C++, strings are treated as objects and come with a variety of built-in methods that facilitate their manipulation.
String Declaration in Python
In Python, you can declare a string by enclosing a sequence of characters in either single or double quotes. This flexibility allows for the inclusion of quotes within the string without the need for escape characters. Here’s a simple example of string declaration:
# String declarations
single_quoted_string = 'Hello, World!'
double_quoted_string = "Hello, World!"
# Including quotes within strings
string_with_single_quote = "It's a sunny day."
string_with_double_quote = 'She said, "Hello!"'
Properties of Strings
Immutable: In languages like Python, strings are immutable, meaning that once a string is created, its content cannot be changed. Any operation that modifies a string results in the creation of a new string.
original_string = "Hello" modified_string = original_string.replace("H", "J") # Creates a new string "Jello" print(original_string) # Output: Hello print(modified_string) # Output: Jello
In this example,
original_string
remains unchanged, and a new string is created with the modification.Length: You can determine the length of a string using built-in functions. In Python, the
len()
function returns the number of characters in a string.my_string = "Hello, World!" length = len(my_string) # Returns 13 print(length) # Output: 13
Indexing and Slicing: Strings can be indexed to access individual characters, and you can slice strings to create substrings. The indexing starts at 0.
my_string = "Hello, World!" first_character = my_string[0] # 'H' substring = my_string[0:5] # 'Hello' print(first_character) # Output: H print(substring) # Output: Hello
Common String Operations
Concatenation: You can concatenate two or more strings using the
+
operator.string1 = "Hello" string2 = "World" concatenated = string1 + ", " + string2 + "!" # 'Hello, World!' print(concatenated) # Output: Hello, World!
Splitting: Strings can be split into lists using a specified delimiter.
sentence = "This is a string." words = sentence.split(" ") # ['This', 'is', 'a', 'string.'] print(words) # Output: ['This', 'is', 'a', 'string.']
Searching: You can check if a substring exists within a string using the
in
operator.sentence = "This is a string." is_present = "string" in sentence # Returns True print(is_present) # Output: True
String Formatting: Strings can be formatted using various methods, such as f-strings in Python.
name = "Alice" age = 30 greeting = f"Hello, {name}! You are {age} years old." # 'Hello, Alice! You are 30 years old.' print(greeting) # Output: Hello, Alice! You are 30 years old.
Replacing: The
replace()
method allows you to replace occurrences of a specified substring with another substring.text = "I like cats." new_text = text.replace("cats", "dogs") # 'I like dogs.' print(new_text) # Output: I like dogs.
Upper and Lower Case: You can change the case of a string using the
upper()
andlower()
methods.my_string = "Hello, World!" upper_case = my_string.upper() # 'HELLO, WORLD!' lower_case = my_string.lower() # 'hello, world!' print(upper_case) # Output: HELLO, WORLD! print(lower_case) # Output: hello, world!
For more information on string operations, you can refer to the following resources: -
Applications of Strings in DSA
Text Processing: Strings are fundamental in applications that involve manipulating or analyzing text data. This includes tasks such as parsing, searching, and transforming text. For example, text editors use strings to represent the content of documents, enabling features like search and replace.
Example: A text editor may allow users to search for a word and highlight all its occurrences in the document, using string searching algorithms.
Data Serialization: Strings are commonly used to serialize data for storage or transmission. Data serialization converts complex data structures into a string format, which can then be easily saved to a file or sent over a network.
Example: JSON (JavaScript Object Notation) is a string-based format used to represent structured data. It allows for easy sharing of data between a server and a client.
Database Queries: In database systems, strings are used to formulate SQL queries. Strings allow developers to construct dynamic queries based on user input or application logic.
Example: A web application might use strings to build a query like:
SELECT * FROM users WHERE username = 'JohnDoe';
Cryptography: Strings play a crucial role in cryptography, where they are often used to represent keys, messages, and hash values. String manipulation techniques are essential for encoding, decoding, and hashing data securely.
Example: In password hashing, a string representing a user's password is transformed into a fixed-size hash string, making it difficult for unauthorized users to retrieve the original password.
Search Algorithms: Many algorithms, such as the Knuth-Morris-Pratt (KMP) or Rabin-Karp algorithms, are designed specifically to search for substrings within a larger string efficiently. These algorithms are vital in applications like search engines and text editors.
Example: A search engine uses string matching algorithms to find relevant documents containing specific keywords in its index.
Pattern Matching: Strings are used extensively in pattern matching applications, such as in bioinformatics for DNA sequence analysis or in computer vision for image recognition.
Example: In bioinformatics, strings can represent DNA sequences, and algorithms can be used to find patterns that correspond to specific genes.
Data Compression: Compression algorithms often manipulate strings to reduce the size of data for storage or transmission. Techniques like Huffman coding utilize strings to represent frequently occurring characters with shorter binary codes.
Example: In a text file, the string "aaabbbbcc" can be compressed to a more efficient representation like "3a4b2c" using run-length encoding.
Practice Problems
To enhance your understanding of strings and their manipulation, consider practicing with the following resources:
LeetCode: String Problems
HackerRank: Python Strings Challenges
CodeSignal: String Challenges
Conclusion
Strings are a versatile and essential data structure in programming, providing a way to represent and manipulate text-based data efficiently. Understanding the properties and operations of strings is crucial for developing effective algorithms and data structures in various applications. By practicing string manipulation and exploring its applications, you can enhance your programming skills and prepare for challenges in Data Structures and Algorithms.
As part of our DSA series, we will continue to explore fundamental concepts, allowing you to build a strong foundation in data structures and algorithms, which is essential for success in software development and coding interviews.
Subscribe to my newsletter
Read articles from Keerthi Ravilla Subramanyam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Keerthi Ravilla Subramanyam
Keerthi Ravilla Subramanyam
Hi, I'm Keerthi Ravilla Subramanyam, a passionate tech enthusiast with a Master's in Computer Science. I love diving deep into topics like Data Structures, Algorithms, and Machine Learning. With a background in cloud engineering and experience working with AWS and Python, I enjoy solving complex problems and sharing what I learn along the way. On this blog, you’ll find articles focused on breaking down DSA concepts, exploring AI, and practical coding tips for aspiring developers. I’m also on a journey to apply my skills in real-world projects like predictive maintenance and data analysis. Follow along for insightful discussions, tutorials, and code snippets to sharpen your technical skills.