Optimizing Your Dockerfile
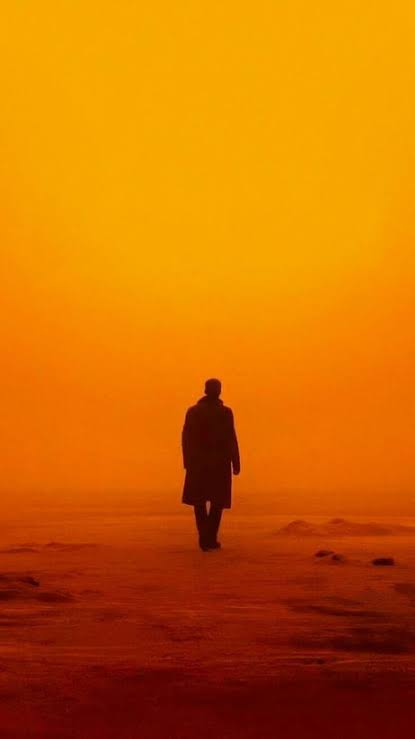
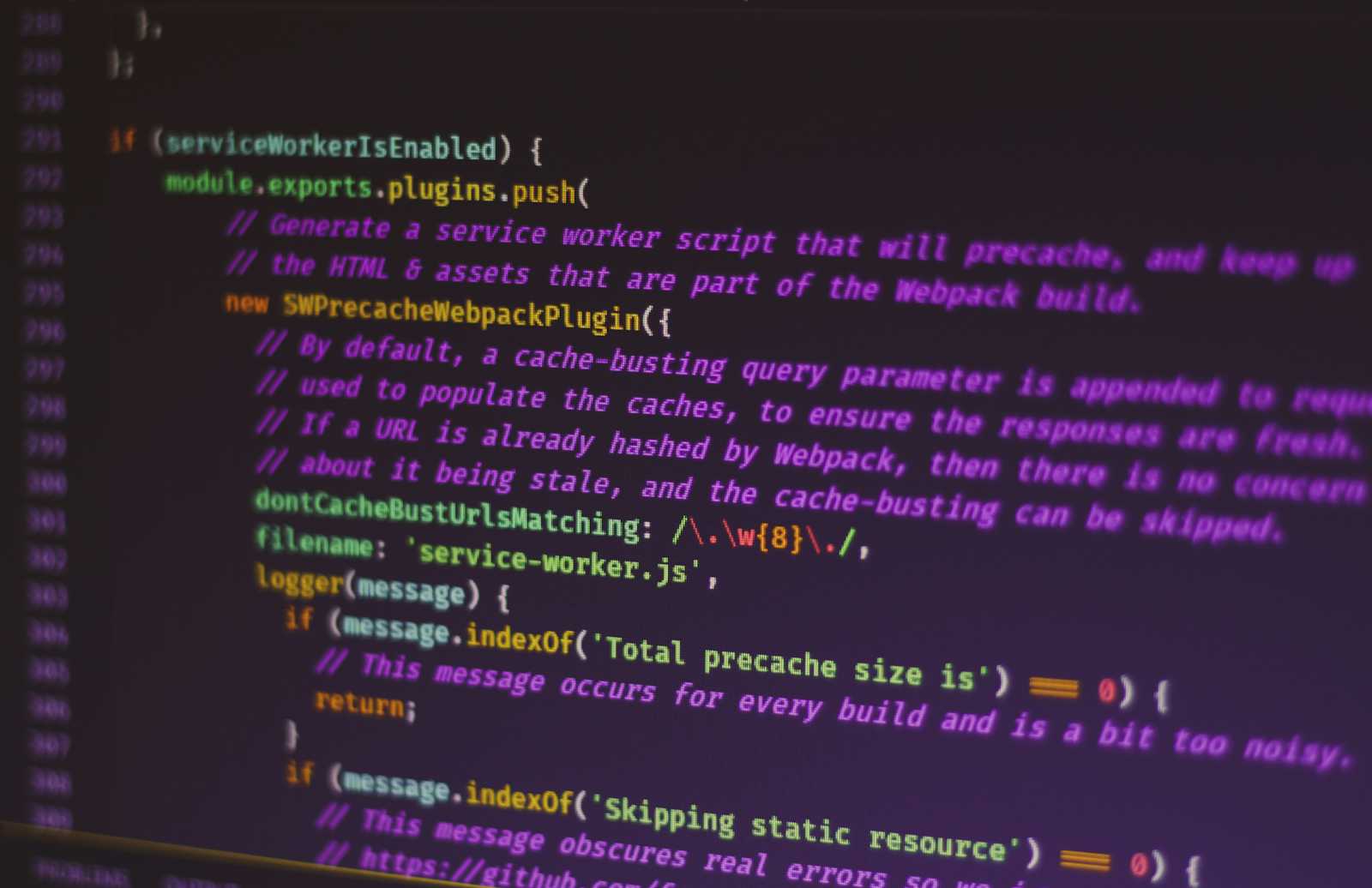
In the world of containerization, creating efficient Docker images is crucial for improved performance, faster deployments, and reduced costs. This guide will walk you through essential Dockerfile optimization techniques with practical examples.
Multi-stage Builds
Multi-stage builds are one of the most powerful optimization techniques. They allow you to use multiple intermediate containers for building your application while keeping the final image lean.
❌ Bad Practice (Single Stage)
FROM node:16
WORKDIR /app
COPY . .
RUN npm install
RUN npm run build
EXPOSE 3000
CMD ["npm", "start"]
✅ Good Practice (Multi-stage)
# Build stage
FROM node:16-alpine AS builder
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
RUN npm run build
# Production stage
FROM node:16-alpine
WORKDIR /app
COPY --from=builder /app/dist ./dist
COPY package*.json ./
RUN npm install --production
EXPOSE 3000
CMD ["npm", "start"]
The multi-stage build separates the build environment from the runtime environment, significantly reducing the final image size.
Layer Optimization
Docker images are built in layers, and each instruction in a Dockerfile creates a new layer. Optimizing these layers is crucial for efficiency.
❌ Bad Practice
FROM ubuntu:20.04
RUN apt-get update
RUN apt-get install -y python3
RUN apt-get install -y python3-pip
RUN pip3 install flask
RUN pip3 install redis
✅ Good Practice
FROM ubuntu:20.04
RUN apt-get update && \
apt-get install -y \
python3 \
python3-pip \
&& rm -rf /var/lib/apt/lists/*
COPY requirements.txt .
RUN pip3 install --no-cache-dir -r requirements.txt
Base Image Selection
Choosing the right base image can significantly impact your final image size and security posture.
❌ Bad Practice
FROM ubuntu:20.04
RUN apt-get update && apt-get install -y python3
✅ Good Practice
FROM python:3.9-slim
Consider using official slim or alpine-based images when possible:
python:3.9-slim
: ~122MBpython:3.9-alpine
: ~45MBpython:3.9
: ~885MB
Caching Considerations
Properly ordering your Dockerfile instructions can significantly improve build times by leveraging Docker's build cache.
❌ Bad Practice
FROM node:16-alpine
WORKDIR /app
COPY . .
RUN npm install
✅ Good Practice
FROM node:16-alpine
WORKDIR /app
COPY package*.json ./
RUN npm install
COPY . .
By copying only the package files first, we ensure that the npm install
layer is cached unless dependencies change.
Security Best Practices
Security should never be compromised for optimization.
❌ Bad Practice
FROM node:16
USER root
COPY . .
RUN npm install
✅ Good Practice
FROM node:16
RUN addgroup --system appgroup && \
adduser --system --ingroup appgroup appuser
WORKDIR /app
COPY --chown=appuser:appgroup . .
RUN npm install
USER appuser
Advanced Optimization Techniques
1. Using .dockerignore
Create a .dockerignore
file to exclude unnecessary files:
node_modules
npm-debug.log
Dockerfile
.dockerignore
.git
.gitignore
README.md
2. Optimize for Production
For production images, consider:
Removing development dependencies
Minimizing layers
Using specific versions for reproducibility
FROM node:16-alpine AS builder
WORKDIR /app
COPY package*.json ./
RUN npm ci --production
COPY . .
RUN npm run build
FROM node:16-alpine
ENV NODE_ENV production
WORKDIR /app
COPY --from=builder /app/dist ./dist
COPY --from=builder /app/node_modules ./node_modules
USER node
CMD ["node", "dist/main.js"]
Conclusion
Optimizing Dockerfiles is a crucial skill for modern developers. By following these best practices, you can create efficient, secure, and maintainable container images. Remember to:
Use multi-stage builds
Optimize layer caching
Choose appropriate base images
Implement security best practices
These optimizations will lead to faster builds, smaller images, and more efficient deployments.
Subscribe to my newsletter
Read articles from Rohit directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
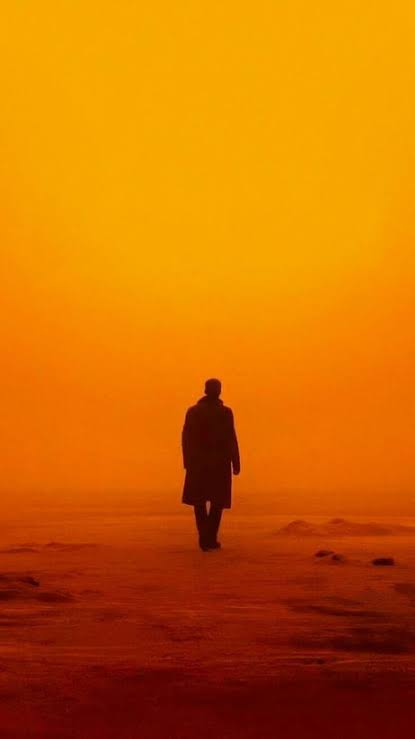
Rohit
Rohit
I'm a results-driven professional skilled in both DevOps and Web Development. Here's a snapshot of what I bring to the table: 💻 DevOps Expertise: AWS Certified Solutions Architect Associate: Proficient in deploying and managing applications in the cloud. Automation Enthusiast: Leveraging Python for task automation, enhancing development workflows. 🔧 Tools & Technologies: Ansible, Terraform, Docker, Prometheus, Kubernetes, Linux, Git, Github Actions, EC2, S3, VPC, R53 and other AWS services. 🌐 Web Development: Proficient in HTML, CSS, JavaScript, React, Redux-toolkit, Node.js, Express.js and Tailwind CSS. Specialized in building high-performance websites with Gatsby.js. Let's connect to discuss how my DevOps skills and frontend expertise can contribute to your projects or team. Open to collaboration and always eager to learn! Aside from my work, I've also contributed to open-source projects, like adding a feature for Focalboard Mattermost.