How the Host property Works in Angular @Component

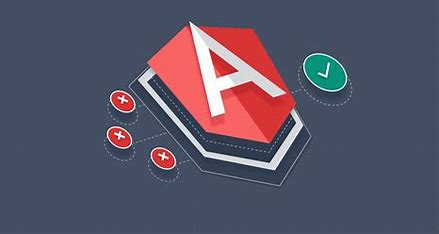
In Angular, the host property is used to bind properties, attributes, and events to the component’s host element, which is the DOM element matching the component’s selector. In Angular, the component’s selector is visible in the DOM tree. As shown in the image below, app-host
and app-root
are the component selectors present in the DOM tree.
We use the host property to bind attributes, properties, and events to these component selectors. These property values can be static or dynamically calculated based on the component's current state.
How to Use the Host Property?
We can bind events, properties, and attributes to host elements by using the host property provided in the @Component
decorator. The host property takes an object where we specify these properties as key-value pairs.
import { Component } from '@angular/core';
@Component({
selector: 'app-host',
standalone: true,
imports: [],
templateUrl: './host.component.html',
styleUrl: './host.component.css',
host:{
'class':'host-class',
'id':'test',
'(click)':'hostClick()'
}
})
export class HostComponent {
hostClick(){
console.log('Host clicked');
}
}
@HostBinding and @HostListener in Angular
We can also use the @HostBinding
and @HostListener
decorators to bind events, attributes, and properties to our host element.
@HostBinding
lets us bind properties and attributes.@HostListener
lets us bind event listeners to the host element.
import { Component, HostBinding, HostListener } from '@angular/core';
@Component({
selector: 'app-host',
standalone: true,
imports: [],
templateUrl: './host.component.html',
styleUrl: './host.component.css',
})
export class HostComponent {
@HostBinding() id = 'host-id';
@HostBinding('class') cl ='host-class'
@HostListener('click',['$event'])
onClick(event: MouseEvent) {
console.log('click on host', event);
}
}
As you can see, we can add different attributes and properties to the host element using @HostBinding, and we can add event listeners using the @HostListener property.
When building modern applications with Angular, we should prefer using the host property over the @HostBinding and @HostListener decorators, as these decorators are mainly for backward compatibility.
If a component has both @HostBinding/@HostListener and the host property, and there is a conflict, the properties inside the host property in the component decorator will take precedence.
Binding Collison
Binding collision can occur if a developer also provides the same attributes/listener while using the component instance in other components' templates. In those cases, the collision is resolved according to these rules:
If both values are static, the instance binding wins.
If one value is static and the other dynamic, the dynamic value wins.
If both values are dynamic, the component's host binding wins.
import { Component, HostBinding, HostListener } from '@angular/core';
@Component({
selector: 'app-host',
standalone: true,
imports: [],
templateUrl: './host.component.html',
styleUrl: './host.component.css',
host:{
'id': 'test-class',
}
})
export class HostComponent {}
<app-host id="abc"/>
As you can see, the first rule applies as the instance value overrides the host binding when both values are static. The same is true for the other two rules.
A Quick Summary
The host property in Angular’s @Component decorator offers a simpler way to attach various properties, attributes, and event listeners to a component's instance in the DOM. It allows us to dynamically update the host element based on the component's state. Additionally, Angular automatically checks for any changes in the host properties during its change detection cycle. While @HostBinding and @HostListener provide backward compatibility and better maintainability, the host property lets us declare multiple properties in one place in a very compact way.
Every Angular developer should learn about the host property because it is essential for better development, easy to use, and improves code readability.
Thank you for your time! Please let me know if there are any opportunities for improvement in this blog or any additions I can make in the comment section.
Subscribe to my newsletter
Read articles from Alok Raturi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
