Mastering Neural Networks with TensorFlow: A Comprehensive Guide

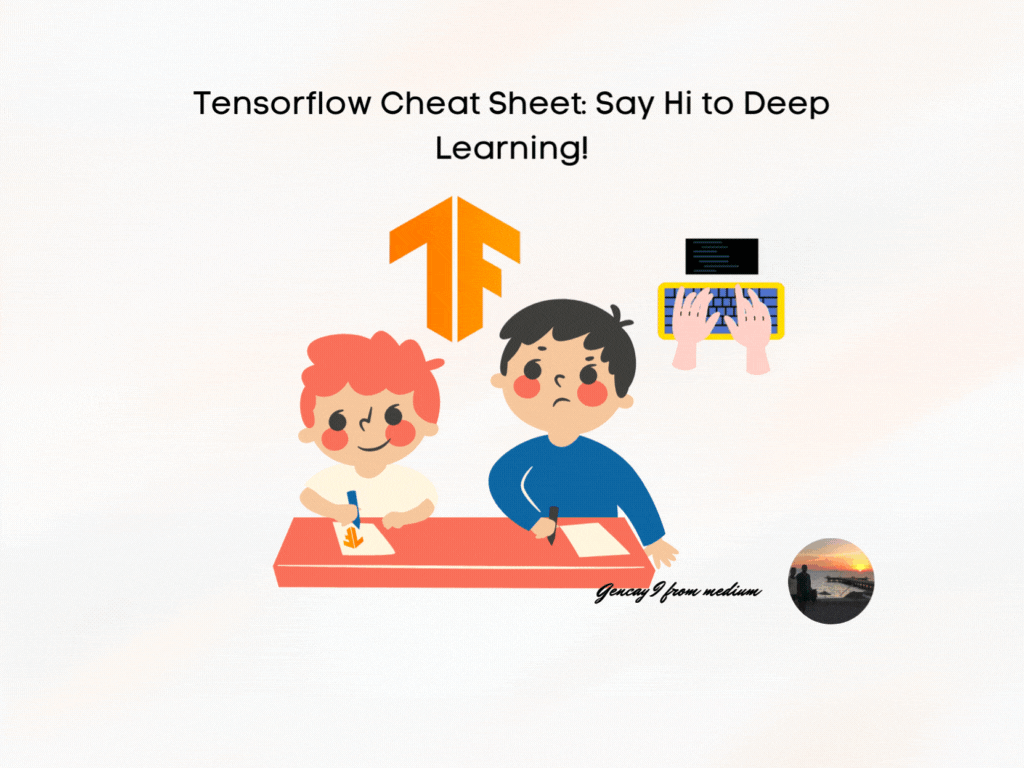
Introduction:
Welcome to the world of neural networks! In this blog post, we'll delve into the fascinating realm of neural networks, focusing on their implementation using TensorFlow. Whether you're a beginner or have some experience, this guide will provide you with a thorough understanding of various neural network architectures, including CNNs, RNNs, KNNs, and LNNs. We'll also include visual aids, animations, and code snippets to enhance your learning experience.
1. Getting Started with TensorFlow:
Installation and Setup: Begin by setting up your environment. Install TensorFlow using pip and ensure your system is ready for development.
pip install tensorflow
Basic TensorFlow Example: Create a simple "Hello World" program to familiarize yourself with TensorFlow's syntax.
import tensorflow as tf print("TensorFlow version:", tf.__version__)
2. Understanding Neural Network Architectures:
Convolutional Neural Networks (CNNs):
Overview: CNNs are designed for image processing tasks. They use convolutional layers to automatically and adaptively learn spatial hierarchies of features.
Visual Explanation: Use animations to show how convolutional layers detect edges, textures, and patterns in images.
Code Snippet:
model = tf.keras.models.Sequential([ tf.keras.layers.Conv2D(32, (3, 3), activation='relu', input_shape=(28, 28, 1)), tf.keras.layers.MaxPooling2D((2, 2)), tf.keras.layers.Flatten(), tf.keras.layers.Dense(64, activation='relu'), tf.keras.layers.Dense(10, activation='softmax') ])
Recurrent Neural Networks (RNNs):
Overview: RNNs are ideal for sequence prediction tasks, such as time series analysis and natural language processing.
Visual Explanation: Use animations to demonstrate how RNNs process sequences of data over time.
Code Snippet:
model = tf.keras.models.Sequential([ tf.keras.layers.SimpleRNN(50, input_shape=(timesteps, features)), tf.keras.layers.Dense(1) ])
K-Nearest Neighbors (KNN):
Overview: Although not a neural network, KNN is a simple, intuitive method for classification tasks.
Visual Explanation: Use diagrams to illustrate how KNN classifies data points based on their proximity to neighbors.
Linear Neural Networks (LNNs):
Overview: LNNs are used for linear regression tasks, providing a foundation for understanding more complex networks.
Code Snippet:
model = tf.keras.models.Sequential([ tf.keras.layers.Dense(1, input_shape=(n_features,)) ])
3. Advanced Concepts:
Transfer Learning: Discuss how to leverage pre-trained models for new tasks, saving time and computational resources.
Fine-Tuning: Explain the process of fine-tuning a model to improve its performance on specific datasets.
4. Interactive Learning:
Encourage readers to experiment with the provided code snippets using platforms like Google Colab.
Include quizzes or challenges to test their understanding of the concepts.
5. Learning Resources:
Books:
"Deep Learning" by Ian Goodfellow, Yoshua Bengio, and Aaron Courville.
"Hands-On Machine Learning with Scikit-Learn, Keras, and TensorFlow" by Aurélien Géron.
Online Courses:
"Deep Learning Specialization" by Andrew Ng on Coursera.
"Introduction to TensorFlow for Artificial Intelligence, Machine Learning, and Deep Learning" by Google on edX.
Tutorials and Documentation:
TensorFlow Official Documentation.
Kaggle for datasets and notebooks.
Conclusion: Summarize the key points covered in the blog post and encourage readers to continue exploring neural networks with TensorFlow. Invite them to share their projects and experiences in the comments section.
Call to Action: Encourage readers to subscribe to your blog for more insightful content on machine learning and AI. Invite them to leave comments, ask questions, and engage with the community.
#Keep Learning !!
#Keep Coding !!!
#Coding Inferno!!!
Subscribe to my newsletter
Read articles from Sujit Nirmal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Sujit Nirmal
Sujit Nirmal
👋 Hi there! I'm Sujit Nirmal, a AI /ML Developer with a passion for creating intelligent, seamless ML applications. With a strong foundation in both machine learning and Deep Learning I thrive at the intersection of data and technology.