Singly link list traversal
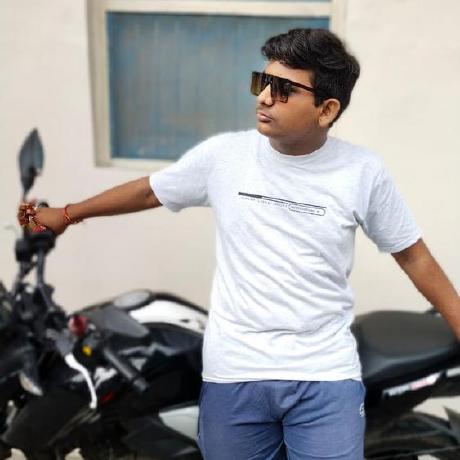
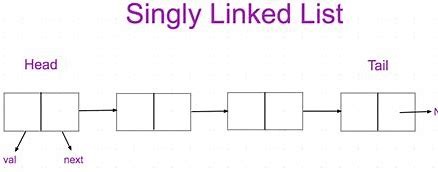
In todayโs blog we are going to see how we can make singly link list in any language today we will see how we can make it in C.
Creation of Node:
- As we know Link list contain the data in the form of NODE so first, we will create node.
struct Node {
int data;
struct Node* next;
};
Here node will contain 2 things that are data and address of next node.
So, the first entry we will take is of data and the next entry will be of address of next node.
Insertion of data
- Now after creating the node, we will have to add data in front of the link list.
The complete code for insertion of node is-
void insert(struct Node** head, int newData) {
// Allocate memory for new node
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
// Set data for the new node
newNode->data = newData;
// Set next of new node as head
newNode->next = *head;
// Move the head to point to the new node
*head = newNode;
}
In this code we are inserting of the data for that we will use FUNCTIONS.
The
newData
value (passed to the function) is assigned to thedata
field of the new node.The
next
field of the new node is set to the current head of the list.
Printing the link list
We will make a function so that we can call the function when we require to print the data.
void printList(struct Node* head) {
struct Node* temp = head;
// Traverse the list and print each node
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
struct Node* head
This is a pointer to the head of the linked list.A temporary pointer
temp
is initialized to point to the head of the list.This loop continues as long as
temp
is notNULL
, meaning we have not reached the end of the list.For each node, the
data
field is printed followed by an arrow (->
) to visually indicate the link between nodes.temp
is updated to point to the next node in the list (temp->next
), moving the traversal forward.
Main function
This is the main function where we will write the main part and without it we cant do anything in our code.
int main() {
// Initialize an empty linked list
struct Node* head = NULL;
// Insert nodes at the beginning
insertAtBeginning(&head, 5);
insertAtBeginning(&head, 10);
insertAtBeginning(&head, 15);
// Print the linked list
printf("Linked List: ");
printList(head);
return 0;
}
We declare a pointer
head
that will point to the first node of the linked list.Initially, the list is empty, so
head
is set toNULL
.We call the
insertAtBeginning
function three times to insert three nodes at the beginning of the list.The data values
5
,10
, and15
are inserted, with15
being the first element after all insertions.The
printList
function is called to print the contents of the list.The final list will look like
15 -> 10 -> 5 -> NULL
.
The complete code for the link list is as follows-
#include <stdio.h>
#include <stdlib.h>
// Define a node structure
struct Node {
int data;
struct Node* next;
};
// Function to insert a new node at the beginning of the list
void insertAtBeginning(struct Node** head, int newData) {
// Allocate memory for new node
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
// Set data for the new node
newNode->data = newData;
// Set next of new node as head
newNode->next = *head;
// Move the head to point to the new node
*head = newNode;
}
// Function to print all nodes in the linked list
void printList(struct Node* head) {
struct Node* temp = head;
// Traverse the list and print each node
while (temp != NULL) {
printf("%d -> ", temp->data);
temp = temp->next;
}
printf("NULL\n");
}
// Main function to demonstrate the linked list operations
int main() {
// Initialize an empty linked list
struct Node* head = NULL;
// Insert nodes at the beginning
insertAtBeginning(&head, 5);
insertAtBeginning(&head, 10);
insertAtBeginning(&head, 15);
// Print the linked list
printf("Linked List: ");
printList(head);
return 0;
}
In this way we can write our first code of link list.
Donโt forget to use comments to increase understanding of code for the viewer.
Subscribe to my newsletter
Read articles from Jalaj Singhal directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
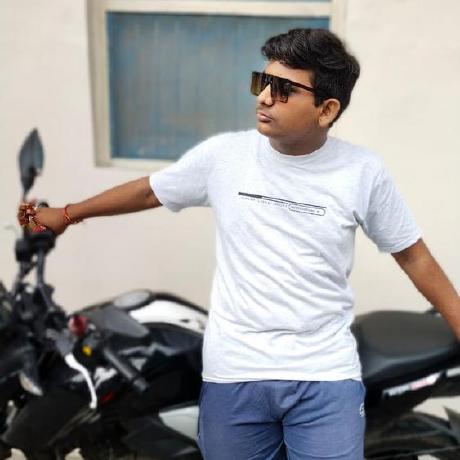
Jalaj Singhal
Jalaj Singhal
๐ Greetings, Jalaj Singhal here! ๐ I'm an enthusiastic blogger who enjoys delving into the world of technology and imparting my knowledge to the community. ๐ Having experience in HTML and CSS, I enjoy creating interesting and educational content that demystifies difficult ideas and gives readers the tools they need to advance their knowledge. ๐ I try to contribute to the active tech community and encourage relevant discussions on Hash Node, where you can find my writings on the subject of web development. ๐ก Together, let's connect and go out on this fascinating path of invention and learning!