Functions in JavaScript
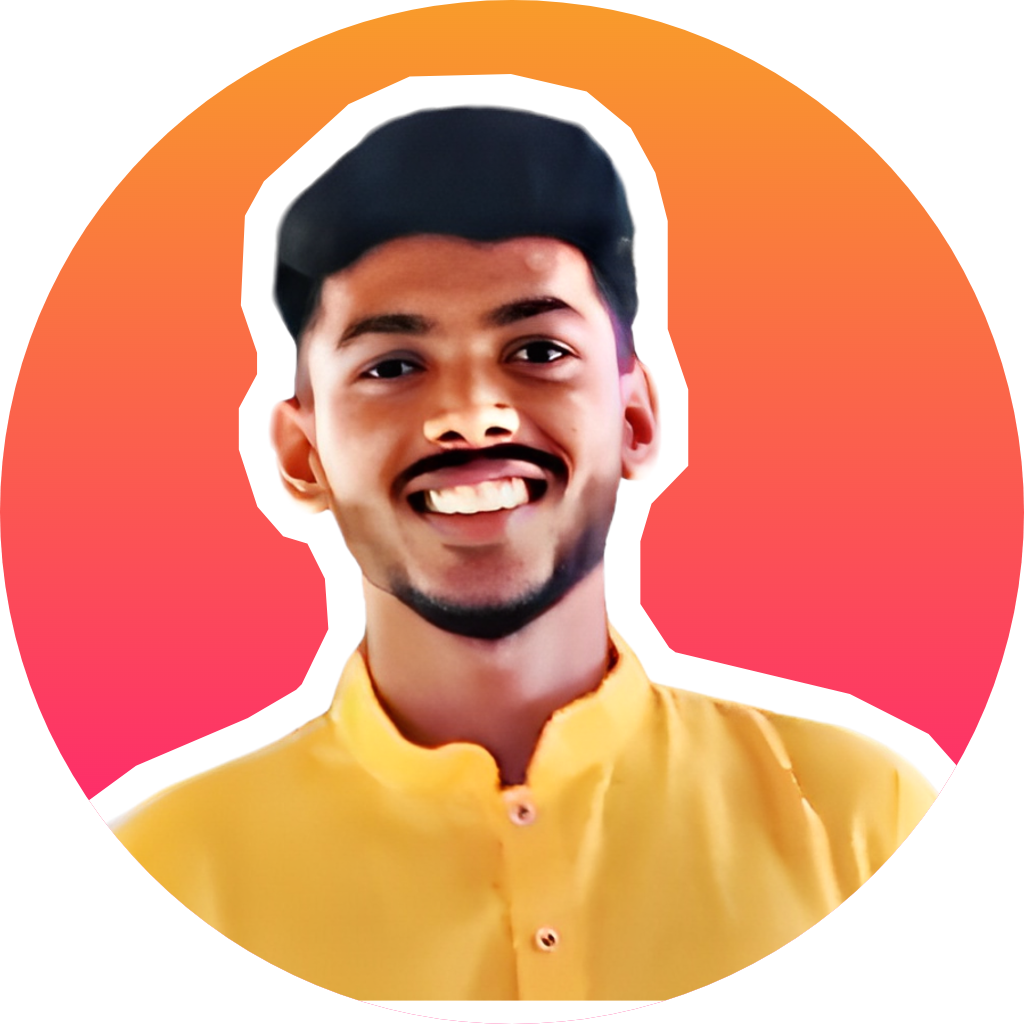
A function is a reusable block of code designed to perform a specific task. You define a function once, and you can call (or "invoke") it as many times as needed, which helps reduce repetition and makes the code more modular and easier to maintain. It takes input(s), processes them, and optionally returns an output. Functions are standalone entities and are called directly by their name.
function greet() {
console.log("Hello, World!");
}
greet() // "Hello, world!"
//passing parameter
function greet(name) {
console.log("Hello, " + name + "!");
}
greet("priya") // "Hello, priya!"
//return value
function add(a, b) {
return a + b;
}
add(4, 9) // 13
There are several types of functions in programming, categorized based on their behavior, parameters, and return values.
Here are some common types:
Function Definition
This is the most common type of function that is used.
//Syntax:
//Defining a function
function function_name(parameters-> not mendentory){
//block of code
}
//example
function functionName(parameters) {
// Code to be executed
return value; // Optional
}
Function Expression
//syntax
function variable_name = function_name(){
//block of code
}
const name = function fullname(fname, lname){
console.log(`The firstname is ${fname} and lastname is ${lname}`);
}
name("ariana", "jonas");
Arrow Functions
//syntax
function variable_name = () => {
//block of code
}
//example:
const name = () => {
console.log(`The firstname is ${fname} and lastname is ${lname}`);
};
name();
Higher order function
Functions that take another function as an argument, return a function, or both. These are commonly used in functional programming.
function performOperation(operation, a, b) { return operation(a, b); }
function add(x, y) { return x + y; }
performOperation(add, 3, 4); // Output: 7
IIFE(Immediate invoke function)
A function that is executed immediately after it is defined. IIFEs are commonly used to create a local scope to avoid polluting the global scope.
(function () {
console.log('Hello geeta');
})();
Hoisting
Hoisting in JavaScript refers to the behavior where variable and function declarations are moved to the top of their containing scope (global or function scope) during the compilation phase, before the code is executed. This means you can use functions and variables before they are actually declared in the code.
greet(); // Output: "Hello, Geetika!"
function greet() {
console.log("Hello, Geetika!");
}
Interview questions
What is Function Declaration?
What is Function Expression?
What is Anonymous Function?
What is IIFE?
IIFE based Question
(function(x){ return (function (y) { console.log(x) })(2) })(1)
What is closures?
What is function Scope?
What is Hoisting?
Parameter Vs Argument
What is callback function?
What is Arrow function?
What is difference between Arrow function and Normal function?
Subscribe to my newsletter
Read articles from Vitthal Korvan directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
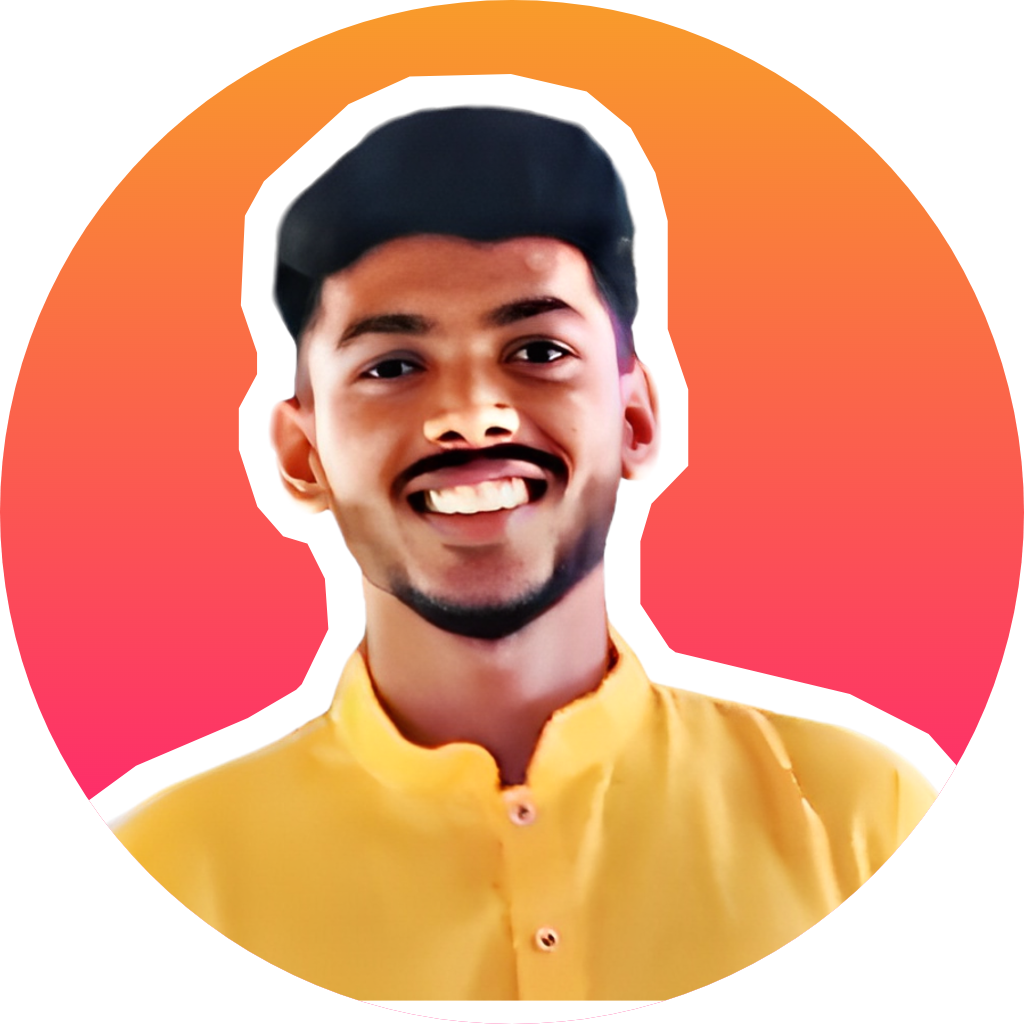
Vitthal Korvan
Vitthal Korvan
๐ Hello, World! I'm Vitthal Korvan ๐ As a passionate front-end web developer, I transform digital landscapes into captivating experiences. you'll find me exploring the intersection of technology and art, sipping on a cup of coffee, or contributing to the open-source community. Life is an adventure, and I bring that spirit to everything I do.