How to Implement a Throttle Function in React
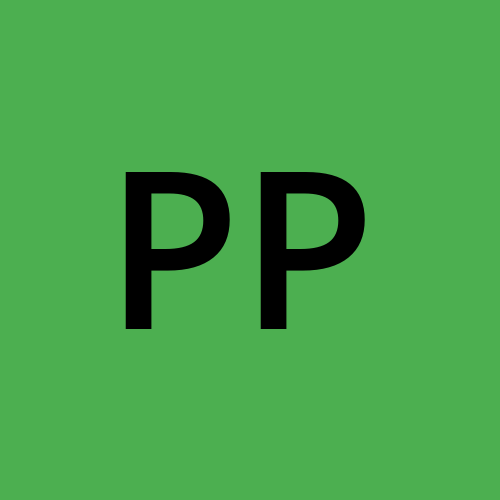
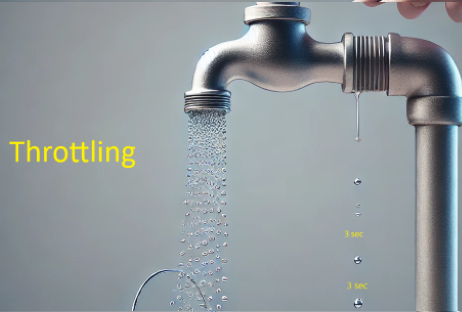
When building modern web applications, controlling the rate at which certain functions are executed can be crucial, especially when dealing with performance-sensitive operations like event listeners (e.g., scroll, resize, or input events). One common approach to this is throttling, which limits the number of times a function can be executed within a specific time period.
While utility libraries like lodash provide a handy throttle function, it's useful to understand how to create your own version, especially if you're trying to avoid unnecessary dependencies in your project.
What is Throttling?
Throttling ensures that a function is only called once in a defined time interval, regardless of how many times it’s invoked. For example, if you have a scroll event handler that executes many times per second, throttling can limit the number of function calls, preventing performance issues and improving user experience.
Debouncing Vs Throttling
Both function accept a function and a delay and both used to control performance of function. Suppose in input box while typing there is a function which will call on each click. Then by Debouncing function, it will call after delay when you will stop typing in input and by Throttling function will call after delay continuously. Throttle will not wait for user to stop typing, will be called continuously after given delay.
Implementing a Throttle Function in React
To create a throttle function in React, we’ll use the useRef hook, which allows us to persist values between renders without causing unnecessary re-renders. Let’s start by writing a custom hook called useThrottle.
import { useRef } from "react";
function useThrottle(callback, delay) {
const isThrottled = useRef(false);
const throttledFunction = (...args) => {
if (!isThrottled.current) {
callback(...args);
isThrottled.current = true;
setTimeout(() => {
isThrottled.current = false;
}, delay);
}
};
return throttledFunction;
}
export default useThrottle;
Explanation:
useRef: We use useRef to hold a reference to the throttle state (isThrottled). This value persists across renders without causing the component to re-render when updated.
callback: This is the function that you want to throttle. It could be any event handler or action that you want to control.
delay: This is the throttle time in milliseconds. After the delay, the function can be triggered again.
setTimeout: Once the function is called, the isThrottled flag is set to true for the specified delay period. After this period, it is reset to false, allowing the function to be called again.
How It Works:
When the throttled function is invoked, it checks if the function is currently throttled by checking isThrottled.
If it’s not throttled, the callback is executed and the function becomes throttled (i.e., temporarily locked from further execution).
After the specified delay, the throttle lock is released, and the function can be executed again.
import React, { useState } from "react";
import useThrottle from "./useThrottle";
function ThrottledComponent() {
const [value, setValue] = useState(0);
// Throttled function to update the state
const throttledFunction = useThrottle(() => {
setValue((prev) => prev + 1);
}, 3000); // 3 second delay
return (
<div>
<h1>Throttle Example</h1>
<p>Value: {value}</p>
<button onClick={throttledFunction}>Click me (Throttled)</button>
</div>
);
}
export default ThrottledComponent;
output:-
Counter will increase after every 3 second in spite of clicking many times.
Benefits of Throttling in React
Improved Performance: Throttling prevents excessive function calls, which can be especially beneficial for high-frequency events like scrolling or resizing.
Optimized User Experience: By reducing unnecessary re-renders and state updates, your application will feel more responsive.
Custom Control: Creating your own throttle function allows you to tailor its behavior according to your app's specific needs.
Conclusion
Building a custom throttle function in React is a simple yet powerful way to control the frequency of function calls, improving performance and enhancing the user experience. By leveraging hooks like useRef and controlling the state manually, you can easily avoid dependencies like lodash and maintain clean, efficient code.
Whether you're optimizing for scroll events, form inputs, or button clicks, a throttled function is a smart way to keep your React app running smoothly.
Subscribe to my newsletter
Read articles from Prashant Pathak directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
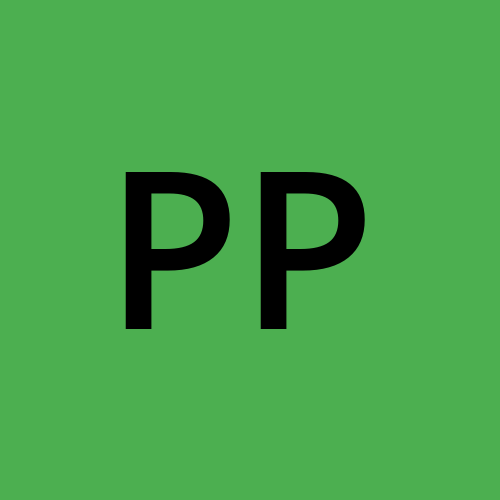