Spring Boot Learning Path
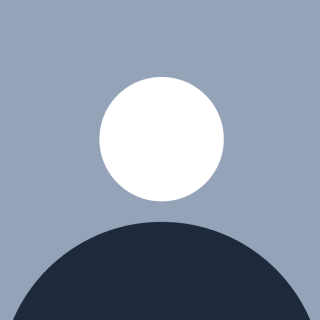
Table of contents
- Prerequisites:
- 1. Getting Started with Spring Framework
- 2. Spring Boot Basics
- 3. Spring Boot Advanced
- 4. Building REST APIs
- 5. Persistence
- 6. Security
- 7. Microservices
- 8. Logging, Monitoring, and Deployment
- 9. Testing
- 10. Spring RestTemplate and WebClient
- 11. Async Programming
- 12. Advanced Topics
- 13. Reactive Programming
- 14. Event-Driven Architecture
- 15. Serverless Applications
- 16. Exploring Behind the Scenes
Prerequisites:
Java Programming:
Basics: Classes, variables, loops, object-oriented programming (OOP), collections, and generics.
Advanced: Design patterns, JVM, threads, servlets and JSPs, concurrency, and garbage collection.
Build Tools: Maven, Gradle, Ant.
Servers: Tomcat, WebLogic, JBOSS, WebSphere, Jetty.
Testing: Unit testing, integration testing with JUnit and Mockito.
Logging: Log4j, Log4j2, Logback.
Spring Framework:
Core: Bean life cycle, dependency injection, inversion of control, bean factory, and application context.
Web: Annotations, MVC structure, configurations, integrating different libraries/frameworks, profiles.
AOP: Understanding how AOP works, creating pointcuts, join points, and aspects.
1. Getting Started with Spring Framework
Overview of the Spring Framework and its ecosystem.
Key terms and concepts used in Spring.
Understanding the architecture of the Spring Framework.
Why Spring? Benefits and reasons for using Spring.
Different ways to configure Spring applications.
Dependency Injection: Core concept of Spring for managing dependencies.
Spring IOC: Inversion of Control and its implementation in Spring.
Spring AOP: Aspect-Oriented Programming in Spring for cross-cutting concerns.
Join Points: Points in the program execution where aspects can be applied.
Pointcuts: Expressions that define where advice should be applied.
Advice: Action taken by an aspect at a particular join point.
Aspects: Modules that encapsulate cross-cutting concerns.
Weaving: Process of linking aspects with other application types or objects to create an advised object.
Spring MVC:
Servlet: Java classes for handling requests and responses.
JSP Files: JavaServer Pages for building dynamic web content.
Architecture: Understanding the architecture of Spring MVC.
Components: Key components of the Spring MVC framework.
Annotations: Common annotations used in Spring for configuration and development.
Spring Bean Scope: Different scopes of beans in Spring.
2. Spring Boot Basics
Understand the difference between Spring and Spring Boot.
Create a "Hello World" application using Spring Boot.
Learn about Spring Boot Starter Parent and create a multi-module Maven project.
Build a basic CRUD REST API using annotations like
@GetMapping
,@PostMapping
,@PutMapping
, and@DeleteMapping
Change the default port and context path of an embedded server.
Disable the startup banner and get all loaded beans with class type information.
Configure custom PropertyEditor and CommandLineRunner.
Configure Jetty and embedded Tomcat servers.
Read files from the resources folder and inject application arguments.
Manage application properties and YAML configuration.
Understand Spring Boot logging and application configuration.
3. Spring Boot Advanced
Learn how Spring Boot simplifies application development with its opinionated approach.
Understand how Spring Boot auto-configures beans and components.
Learn to customize and add configurations as needed.
Manage application settings using properties and YAML files.
Integrations with Other Libraries/Frameworks.
4. Building REST APIs
Design REST APIs using Spring Boot and Spring MVC.
Implement internationalization, response compression, and JSON data handling.
Add custom headers and retry mechanisms.
Document APIs with Swagger and Springdoc-OpenAPI.
Use OpenFeign for REST client creation.
Learn REST API best practices, including exception handling and testing.
Understand HTTP methods (POST, GET, PUT, DELETE, OPTIONS, TRACE) and status codes (1XX, 2XX, 3XX, 4XX, 5XX).
5. Persistence
Learn Database:
SQL: MySQL, PostgreSQL, Oracle.
NoSQL: MongoDB, Cassandra.
Spring Data: Spring Data JPA, Spring Data MongoDB, Spring Data JDBC.
Hibernate:
Transactions: Managing database transactions in Hibernate.
Relationships: Mapping relationships between entities.
Entity Lifecycle: Understanding the lifecycle of entities in Hibernate..
Work with SQL databases using Spring Boot, JPA, Hibernate, and HikariCP.
Configure multiple data sources and caching with Ehcache and Caffeine.
Implement CRUD operations and pagination.
Use database migration tools like Flyway or Liquibase.
6. Security
Authentication: Mechanisms for verifying user identity.
Authorization: Controlling access to resources based on user roles.
OAuth2: Protocol for authorization and secure API access.
JWT Authentication: Using JSON Web Tokens for stateless authentication.
Implement CORS, SSL, and role-based security.
Secure REST APIs with OAuth2, JWT, and basic authentication.
Learn Spring Security basics and advanced concepts like OAuth2 and form authentication.
7. Microservices
Learn Microservices:
Spring Cloud: Gateway, Config, Circuit Breaker, OpenFeign, Sleuth, and other projects as needed.
DevOps: Docker, Kubernetes, cloud platforms (AWS, GCP, Azure).
Microservices Patterns: Aggregator, CQRS, SAGA, Event Sourcing.
Microservices Queues: SQS, Kafka, RabbitMQ.
Understand microservices architecture and its benefits.
Implement design patterns and secure microservices with OAuth2.
8. Logging, Monitoring, and Deployment
Use Spring Boot profiles, Dev Tools, and Actuator for monitoring.
Implement AOP and package applications as WAR or JAR.
Dockerize applications and configure logging with Log4j2 and Lombok.
Deploy applications using Docker, Kubernetes, and cloud platforms.
9. Testing
Test Spring Boot applications using JUnit, Mockito, and TestRestTemplate.
Perform integration testing with MockMVC and WireMock.
Explore advanced testing techniques with @RestClientTest and @DataJpaTest.
10. Spring RestTemplate and WebClient
Use RestTemplate for REST API interactions, including basic auth and timeouts.
Transition to WebClient for non-blocking requests and explore its features.
11. Async Programming
Build asynchronous REST APIs with
@EnableAsync
and SseEmitter.Test async controllers with MockMvc and explore RSocket.
12. Advanced Topics
Dive into Spring Boot auto-configuration and custom starters.
Explore Spring Boot's integration with GraphQL, SOAP, and JMS.
Learn about new features in Spring Boot 3, such as ProblemDetail and ErrorResponse.
13. Reactive Programming
Explore reactive programming with Spring WebFlux.
Understand the Reactor library and its components like Mono and Flux.
Implement reactive REST APIs and integrate with reactive data sources.
14. Event-Driven Architecture
Learn about event-driven architecture and its benefits.
Use Spring Cloud Stream for building event-driven microservices.
Integrate with messaging systems like Kafka and RabbitMQ.
15. Serverless Applications
Explore serverless architecture and its use cases.
Deploy Spring Boot applications as serverless functions using AWS Lambda or Azure Functions.
16. Exploring Behind the Scenes
Understand how Spring Boot auto-configuration works.
Learn about architecture concepts like Domain Driven Design (DDD) and modular monoliths.
Explore Spring Modulith for building modular monolithic applications.
Subscribe to my newsletter
Read articles from Dilip Patel directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
Dilip Patel
Dilip Patel
Software Developer