A Developer's Guide to Path Management in VS Code for React and Python
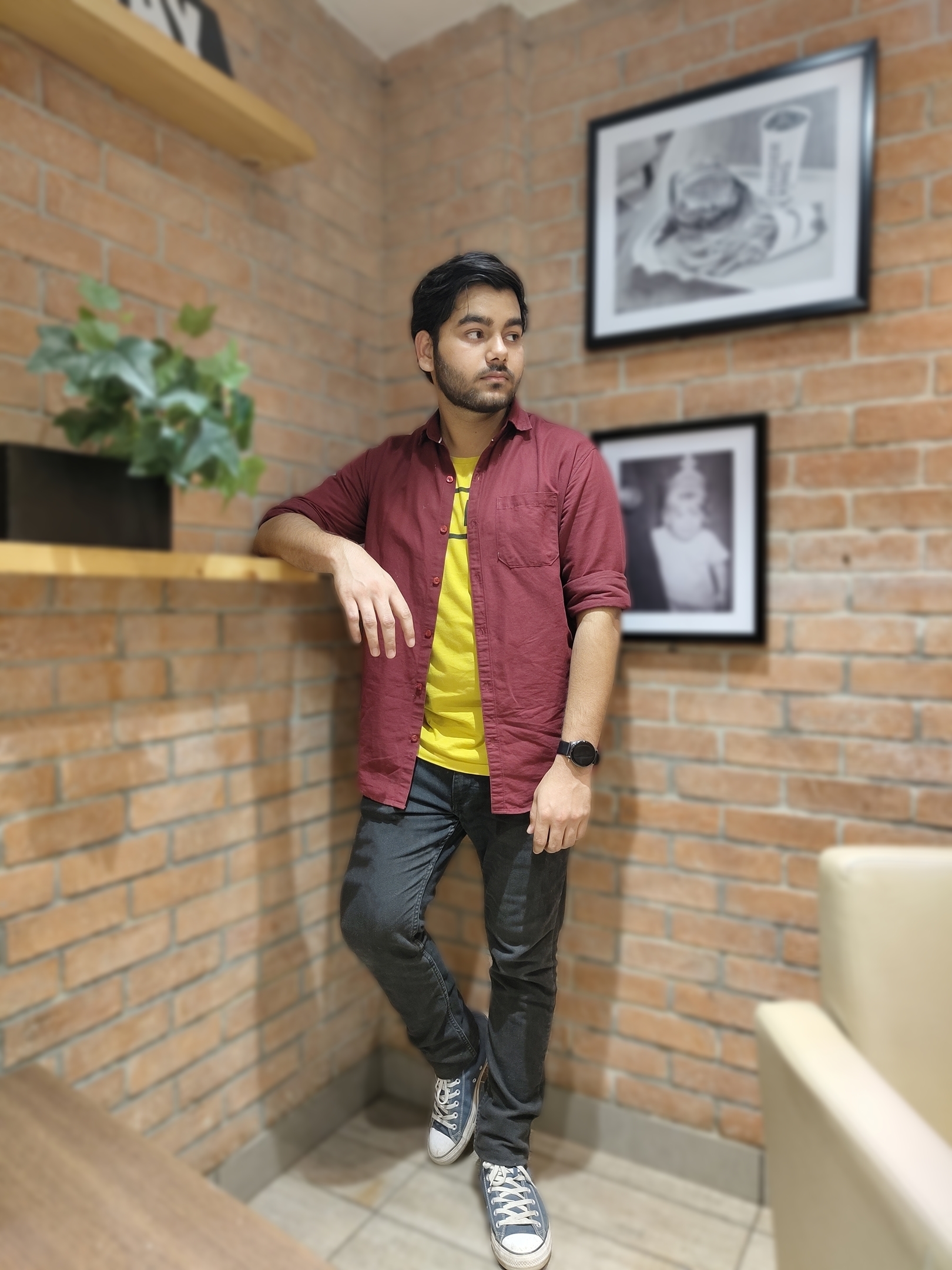
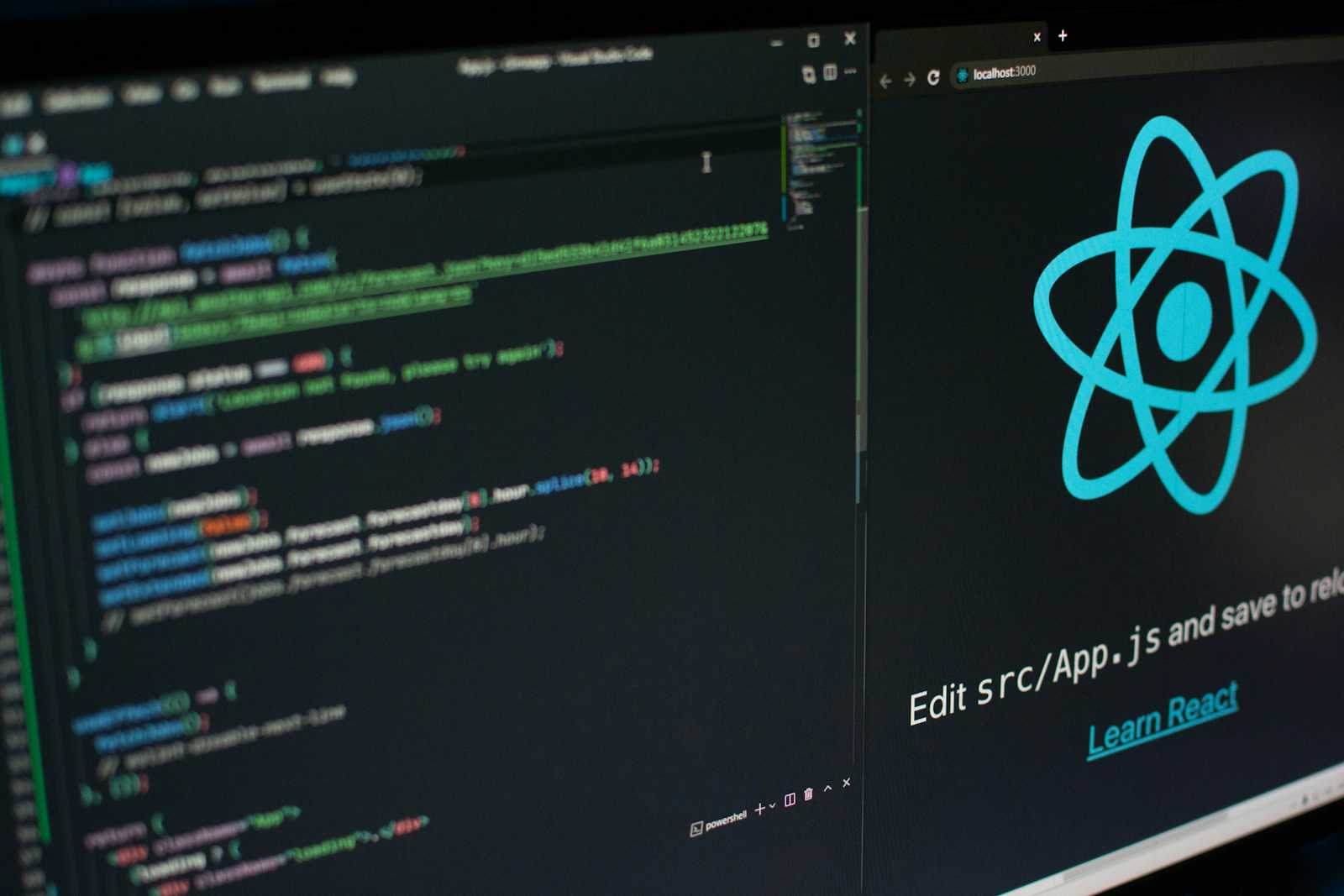
If you're anything like me, you've undoubtedly encountered this situation: You're working in VS Code on a Python or React project when all of a sudden you're faced with absurd path difficulties. Your code works perfectly, but you're receiving "Module not found" warnings or your files aren't being recognized. Fixing path-related problems can be tedious and time-consuming, but there is an effective approach to address this. Trust me.
I'll explain how to become great at route management in VS Code for Python and React applications in this article. Regardless of your level of experience as a developer, these pointers will spare you many hours of headaches!
Why Path Issues Happen in Projects?
Let's explore why routes may become an issue in your projects before moving on to solutions:
Absolute vs. Relative Paths: The incorrect kind of path could damage your code, depending on how you import modules.
Directory Structure: Deeply nested files might quickly cause you to lose sight of where things are as your project grows.
Python Virtual Environments: When working on Python projects, you may have problems with packages that are not installed correctly, leading to import failures.
Your project structure will be better organized and typical mistakes will be avoided if you understand how VS Code maintains routes.
1. Understanding Relative vs. Absolute Paths
One of the most frequent problems is mixing up relative and absolute paths, particularly with React. Let me give you a little explanation first:
The location of the current file determines the relative paths. For instance, a file in the components folder of the same directory is indicated by the path./components/Navbar.js.
Absolute Paths begin at your project's root directory. Importing can be made easier by using absolute paths, particularly in larger applications.
How to Set Up Absolute Imports in React
React projects usually start with relative imports, but absolute imports make your code cleaner.
Open the
jsconfig.json
(or create one if it doesn’t exist).Add the following configuration:
{
"compilerOptions": {
"baseUrl": "src"
},
"include": ["src"]
}
This tells your React project that all imports should start from the src
folder, so you can import components like:
import Navbar from 'components/Navbar';
No more ../../../components/Navbar
! Clean, right?
2. Fixing Import Errors in Python Projects
When using virtual environments or executing scripts from various folders in Python, things might get complicated. Python often fails to locate modules even when they are present in your project.
Key Steps to Avoid Path Issues:
Use Virtual Environments: Ensure that your Python project is in a virtual environment to avoid conflicting dependencies.
python3 -m venv venv source venv/bin/activate # On macOS/Linux .\venv\Scripts\activate # On Windows
Set PYTHONPATH in VS Code: In Python projects, you can set your project’s root directory to the
PYTHONPATH
environment variable. This ensures your modules are found no matter where you run the script.- Create a
.env
file in your project root and add:
- Create a
PYTHONPATH=./
- Then, in your
settings.json
(VS Code settings):
{
"python.envFile": "${workspaceFolder}/.env"
}
This will make sure VS Code always knows where to look for your project files.
3. Using the Correct Working Directory
When the working directory is incorrect, another frequent problem occurs. Perhaps you are trying to run a file that expects the root folder to be the working directory while you are in a deeply nested folder. How to correct that is as follows:
React Projects: When executing npm start or yarn start, make sure you're always in the root folder if you're using the terminal in Visual Studio Code.
To avoid mistakes, use the integrated terminal in VS Code, which defaults to the root folder.
Python Projects: To dynamically set your working directory, use the os module:
import os
# Set working directory to the root of the project
os.chdir(os.path.dirname(os.path.abspath(__file__)))
This ensures your script always runs from the intended folder, no matter where it’s executed from.
4. VS Code Extensions to Simplify Path Management
Path management can be further simplified with the help of a few useful VS Code extensions:
Path Intellisense: This add-on automatically fills in filenames, which helps you save time and minimize path import mistakes.
Auto Import: When you utilize components in React applications, this addon looks for and inserts import statements automatically.
These two tools can stop the annoying "module not found" warnings and smoothly integrate with your workflow.
5. Organizing Your Directory Structure
A well-structured directory is key to reducing path issues. Here are some recommendations for organizing your files:
React:
/src
/components
/pages
/utils
Python:
/project-root
/src
/module1
/module2
/tests
venv/
Keeping a consistent structure helps both you and VS Code understand where things are.
Conclusion
To sum up, maintaining a seamless development workflow for Python and React or any other projects requires efficient path management in VS Code.
You may drastically cut down on the amount of time you spend debugging path-related problems by comprehending the distinctions between absolute and relative paths, properly configuring your environment, and making use of useful extensions.
Your productivity will increase and your projects will be scalable and well-structured if you use the appropriate tools and organize your directory structure.
You'll be more prepared to tackle any path management obstacles with these tactics in hand.
###
Subscribe to my newsletter
Read articles from Krishna Ketan Rai directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
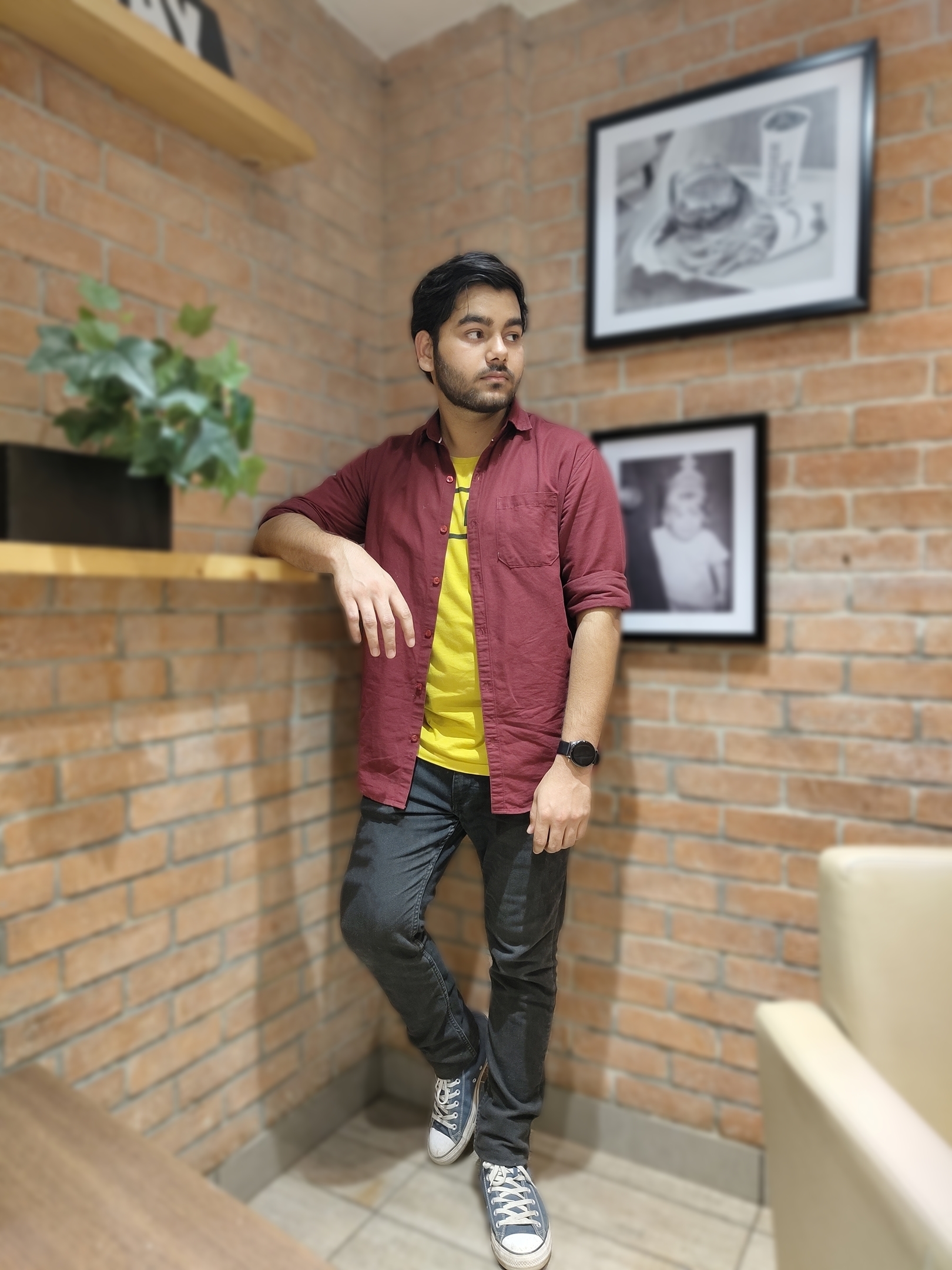
Krishna Ketan Rai
Krishna Ketan Rai
A computer science student trying to understand and build.