Javascript Crash Course for Python Programmers

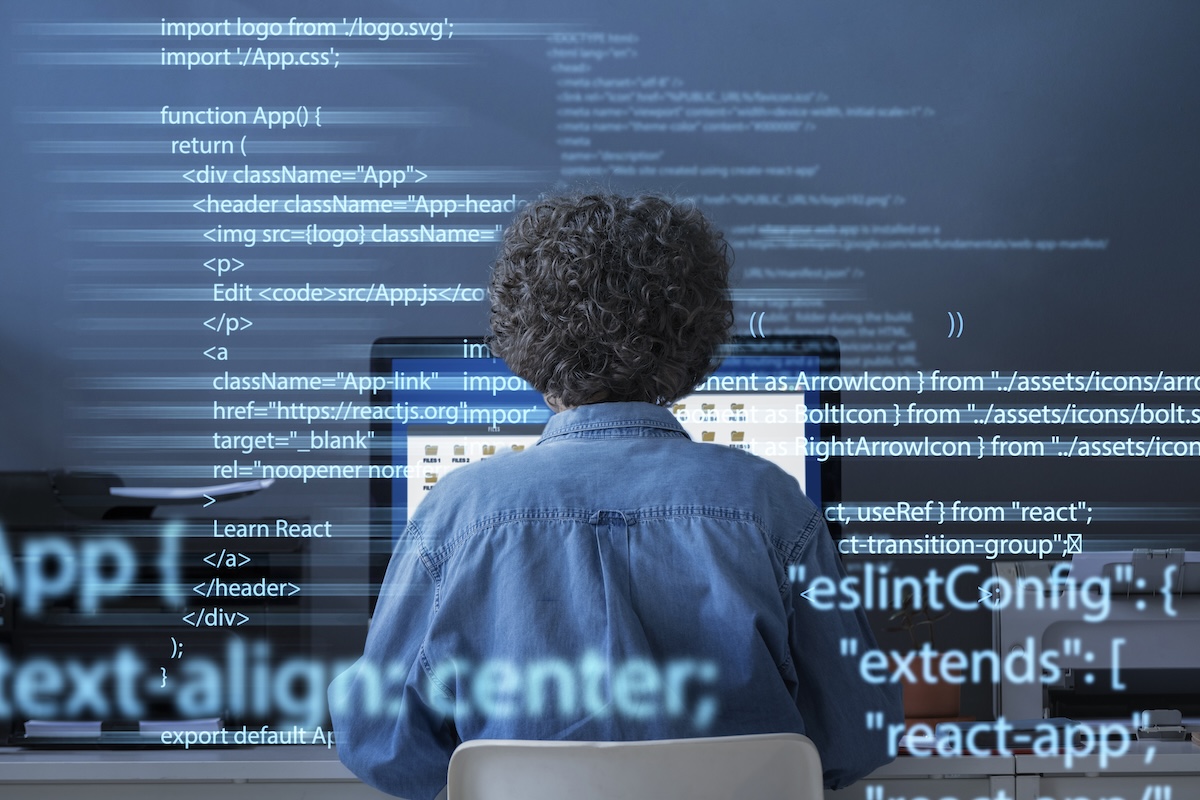
I'll focus on JavaScript, HTML, and CSS, covering only what's important for your path toward working with react vue or svelte in the future. Since you already have experience with Python and some knowledge of HTML and CSS, I'll highlight the key concepts and differences to help you transition smoothly.
JavaScript Essentials for Your Path
1. JavaScript Basics
Variables and Data Types
Variable Declarations:
let
: Declares a block-scoped variable (can be reassigned).let x = 10; x = 20; // Allowed
const
: Declares a block-scoped constant (cannot be reassigned).const y = 30; y = 40; // Error: Assignment to constant variable
Data Types:
Primitive Types:
Number
,String
,Boolean
,Null
,Undefined
,Symbol
,BigInt
.Reference Types:
Object
(includes Arrays and Functions).
Example:
let age = 25; // Number const name = 'Alice'; // String let isStudent = true; // Boolean let address = null; // Null let score; // Undefined const id = Symbol('id'); // Symbol const bigNumber = 123n; // BigInt
Operators
Arithmetic Operators:
+
,-
,*
,/
,%
,**
(exponentiation).Assignment Operators:
=
,+=
,-=
,*=
,/=
,%=
,**=
.Comparison Operators:
==
and!=
: Equality with type coercion.===
and!==
: Strict equality without type coercion.
Logical Operators:
&&
(AND),||
(OR),!
(NOT).
Control Structures
If-Else Statements:
if (condition) { // Executes if condition is truthy } else if (anotherCondition) { // Executes if anotherCondition is truthy } else { // Executes if none of the above conditions are truthy }
Switch Statement:
switch (expression) { case value1: // Code to execute when expression === value1 break; case value2: // Code to execute when expression === value2 break; default: // Code to execute if no matching case }
Loops:
For Loop:
for (let i = 0; i < 5; i++) { console.log(i); }
While Loop:
while (condition) { // Code to execute as long as condition is true }
Do-While Loop:
do { // Code to execute at least once } while (condition);
For...of Loop (iterates over iterable objects):
const array = [10, 20, 30]; for (const value of array) { console.log(value); }
For...in Loop (iterates over object properties):
const obj = { a: 1, b: 2 }; for (const key in obj) { console.log(key, obj[key]); }
2. Functions
Function Declaration
function sum(a, b) {
return a + b;
}
Function Expression
const sum = function(a, b) {
return a + b;
};
Arrow Functions (ES6)
Basic Syntax:
const sum = (a, b) => { return a + b; };
Implicit Return (if the function body is a single expression):
const sum = (a, b) => a + b;
No Parameters:
const greet = () => console.log('Hello');
Single Parameter (no parentheses needed):
const square = x => x * x;
Default Parameters
function multiply(a, b = 1) {
return a * b;
}
3. Objects and Arrays
Objects
Creating an Object:
const person = { firstName: 'John', lastName: 'Doe', age: 28, greet() { console.log(`Hello, my name is ${this.firstName}`); }, };
Accessing Properties:
console.log(person.firstName); // 'John' console.log(person['lastName']); // 'Doe'
Adding/Modifying Properties:
person.job = 'Engineer'; person.age = 29;
Arrays
Creating an Array:
const numbers = [1, 2, 3, 4, 5];
Accessing Elements:
console.log(numbers[0]); // 1
Array Methods:
Adding Elements:
numbers.push(6); // Adds to the end numbers.unshift(0); // Adds to the beginning
Removing Elements:
numbers.pop(); // Removes from the end numbers.shift(); // Removes from the beginning
Iterating Over Arrays:
numbers.forEach(num => console.log(num));
Transforming Arrays:
const doubled = numbers.map(num => num * 2);
Filtering Arrays:
const evens = numbers.filter(num => num % 2 === 0);
Reducing Arrays:
const sum = numbers.reduce((total, num) => total + num, 0);
4. ES6 and Beyond
Template Literals
String Interpolation:
const name = 'Alice'; console.log(`Hello, ${name}!`); // 'Hello, Alice!'
Multi-line Strings:
const message = `This is a multi-line string.`;
Destructuring
Arrays:
const [a, b] = [1, 2]; console.log(a); // 1 console.log(b); // 2
Objects:
const person = { firstName: 'Bob', age: 25 }; const { firstName, age } = person; console.log(firstName); // 'Bob' console.log(age); // 25
Spread and Rest Operators
Spread Operator (
...
):Arrays:
const arr1 = [1, 2]; const arr2 = [...arr1, 3, 4]; // [1, 2, 3, 4]
Objects:
const obj1 = { a: 1 }; const obj2 = { ...obj1, b: 2 }; // { a: 1, b: 2 }
Rest Parameters:
function sum(...args) { return args.reduce((total, num) => total + num, 0); } sum(1, 2, 3); // 6
Arrow Functions and this
Lexical
this
:- Arrow functions do not have their own
this
binding; they inheritthis
from the parent scope.
- Arrow functions do not have their own
const obj = {
value: 42,
regularFunction: function() {
console.log(this.value); // 42
},
arrowFunction: () => {
console.log(this.value); // Undefined or global value
},
};
obj.regularFunction();
obj.arrowFunction();
5. Asynchronous JavaScript
Callbacks
Example:
function fetchData(callback) { setTimeout(() => { const data = { name: 'John' }; callback(data); }, 1000); } fetchData(result => { console.log(result); });
Promises
Creating a Promise:
const promise = new Promise((resolve, reject) => { // Asynchronous operation if (/* success */) { resolve(result); } else { reject(error); } });
Using Promises:
promise .then(result => { // Handle success }) .catch(error => { // Handle error });
Async/Await
Simplifying Promises:
async function fetchData() { try { const response = await fetch('https://api.example.com/data'); const data = await response.json(); console.log(data); } catch (error) { console.error(error); } }
6. DOM Manipulation
Selecting Elements
By ID:
const element = document.getElementById('myId');
By Class Name:
const elements = document.getElementsByClassName('myClass');
By Tag Name:
const elements = document.getElementsByTagName('div');
Using Query Selectors:
const element = document.querySelector('.myClass'); const elements = document.querySelectorAll('.myClass');
Manipulating Elements
Changing Content:
element.textContent = 'New text'; element.innerHTML = '<span>New HTML content</span>';
Changing Attributes:
element.setAttribute('src', 'image.jpg'); const src = element.getAttribute('src');
Changing Styles:
element.style.color = 'blue'; element.style.backgroundColor = 'lightgray';
Adding and Removing Classes:
element.classList.add('active'); element.classList.remove('inactive'); element.classList.toggle('hidden');
Event Handling
Adding Event Listeners:
element.addEventListener('click', event => { console.log('Element clicked'); });
Common Events:
Mouse Events:
click
,dblclick
,mouseover
,mouseout
,mousemove
,mousedown
,mouseup
Keyboard Events:
keydown
,keyup
,keypress
Form Events:
submit
,change
,focus
,blur
7. Modules
Exporting and Importing
Named Exports:
// math.js export function add(a, b) { return a + b; } export function subtract(a, b) { return a - b; }
// main.js import { add, subtract } from './math.js';
Default Exports:
// utils.js export default function greet(name) { return `Hello, ${name}`; }
// main.js import greet from './utils.js';
8. Fetch API and HTTP Requests
GET Request:
fetch('https://api.example.com/data') .then(response => response.json()) .then(data => { // Handle data }) .catch(error => { console.error('Error:', error); });
POST Request:
fetch('https://api.example.com/data', { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify({ name: 'Alice' }) }) .then(response => response.json()) .then(data => { // Handle response }) .catch(error => { console.error('Error:', error); });
Using Async/Await:
async function postData(url = '', data = {}) { try { const response = await fetch(url, { method: 'POST', headers: { 'Content-Type': 'application/json' }, body: JSON.stringify(data) }); const jsonData = await response.json(); return jsonData; } catch (error) { console.error('Error:', error); } } postData('https://api.example.com/data', { name: 'Alice' }) .then(data => { console.log(data); });
9. Error Handling
Try...Catch:
try { // Code that may throw an error } catch (error) { console.error('Error:', error); }
Throwing Errors:
function divide(a, b) { if (b === 0) { throw new Error('Cannot divide by zero'); } return a / b; } try { divide(4, 0); } catch (error) { console.error(error.message); }
10. Important Concepts for Your Path
**Understanding the Event Loop and Call Stack in JavaScript.
Closures: Functions that have access to the parent scope, even after the parent function has closed.
function makeAdder(x) { return function(y) { return x + y; }; } const add5 = makeAdder(5); console.log(add5(2)); // 7
Prototypal Inheritance: Understanding how objects inherit properties and methods in JavaScript.
HTML and CSS Overview
1. HTML Structure and Elements
Basic Document Structure:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>Document Title</title> </head> <body> <!-- Content goes here --> </body> </html>
Common Elements:
Headings:
<h1>
to<h6>
Paragraphs:
<p>
Links:
<a href="URL">Text</a>
Images:
<img src="image.jpg" alt="Description">
Lists:
Unordered List:
<ul><li>Item</li></ul>
Ordered List:
<ol><li>Item</li></ol>
Forms:
<form>
,<input>
,<label>
,<button>
, etc.Semantic Elements:
<header>
,<nav>
,<main>
,<section>
,<article>
,<aside>
,<footer>
2. CSS Basics
Including CSS
Inline Styles:
<p style="color: red;">This is red text.</p>
Internal Stylesheet:
<head> <style> p { color: blue; } </style> </head>
External Stylesheet:
<head> <link rel="stylesheet" href="styles.css"> </head>
Selectors
Element Selector:
p { font-size: 16px; }
Class Selector:
.highlight { background-color: yellow; }
ID Selector:
#main-header { text-align: center; }
Attribute Selector:
input[type="text"] { border: 1px solid #ccc; }
Pseudo-classes and Pseudo-elements:
a:hover { color: red; } p::first-letter { font-size: 2em; }
Box Model
Properties:
width
,height
padding
border
margin
Example:
.box { width: 200px; padding: 10px; border: 1px solid #000; margin: 20px; }
Layout
Display Property:
block
,inline
,inline-block
,flex
,grid
Positioning:
static
,relative
,absolute
,fixed
,sticky
Flexbox:
.container { display: flex; flex-direction: row; justify-content: space-between; align-items: center; }
Grid:
.grid-container { display: grid; grid-template-columns: repeat(3, 1fr); grid-gap: 10px; }
Responsive Design
Media Queries:
@media (max-width: 768px) { .container { flex-direction: column; } }
Viewport Meta Tag:
<meta name="viewport" content="width=device-width, initial-scale=1.0">
3. Integrating JavaScript with HTML and CSS
Including JavaScript
External Script:
<script src="app.js"></script>
Defer and Async:
Defer: Scripts are executed after the HTML is parsed.
<script src="app.js" defer></script>
Best Practice: Place script tags just before the closing
</body>
tag if not usingdefer
.
Manipulating Styles with JavaScript
Changing CSS Classes:
element.classList.add('new-class'); element.classList.remove('old-class');
Inline Styles:
element.style.color = 'red'; element.style.backgroundColor = 'black';
Handling Form Inputs
Accessing Form Values:
const input = document.querySelector('input[name="username"]'); const username = input.value;
Form Submission:
const form = document.querySelector('form'); form.addEventListener('submit', event => { event.preventDefault(); // Prevent form from submitting normally // Handle form data });
4. Best Practices
Organize Your Code:
Keep your JavaScript, CSS, and HTML in separate files.
Use meaningful variable and function names.
Comments and Documentation:
- Use comments to explain complex logic.
Code Formatting:
- Consistent indentation and code style improve readability.
Cross-Browser Compatibility:
- Test your code in different browsers.
Accessibility:
Use semantic HTML elements.
Add
alt
attributes to images.Ensure your site is navigable via keyboard.
Applying Your Knowledge
To solidify your understanding, consider building small projects that incorporate these concepts:
Interactive To-Do List:
Use JavaScript to add, remove, and mark tasks as completed.
Manipulate the DOM to update the task list.
Simple Calculator:
Create buttons for numbers and operations.
Use event listeners to handle button clicks.
Update the display dynamically.
Fetch and Display Data:
Use the Fetch API to retrieve data from a public API.
Display the data in a structured format (e.g., list or table).
Responsive Navigation Menu:
Use CSS Flexbox or Grid for layout.
Implement a hamburger menu for mobile devices using JavaScript.
Image Gallery:
Display a grid of images.
Implement a lightbox feature to view images in larger size.
Next Steps
Practice Regularly:
Build more projects.
Solve coding challenges.
Learn More About ES6+ Features:
Generators, Symbols, WeakMaps, and WeakSets.
Advanced array methods.
Explore Web APIs:
Local Storage, Session Storage.
Geolocation API.
Canvas API.
Prepare for Frameworks:
- Understanding these fundamentals will make learning React or Vue.
Subscribe to my newsletter
Read articles from Siddharth Arora directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
