How to Create Efficient Database Indexes for Optimal Long-Term Performance

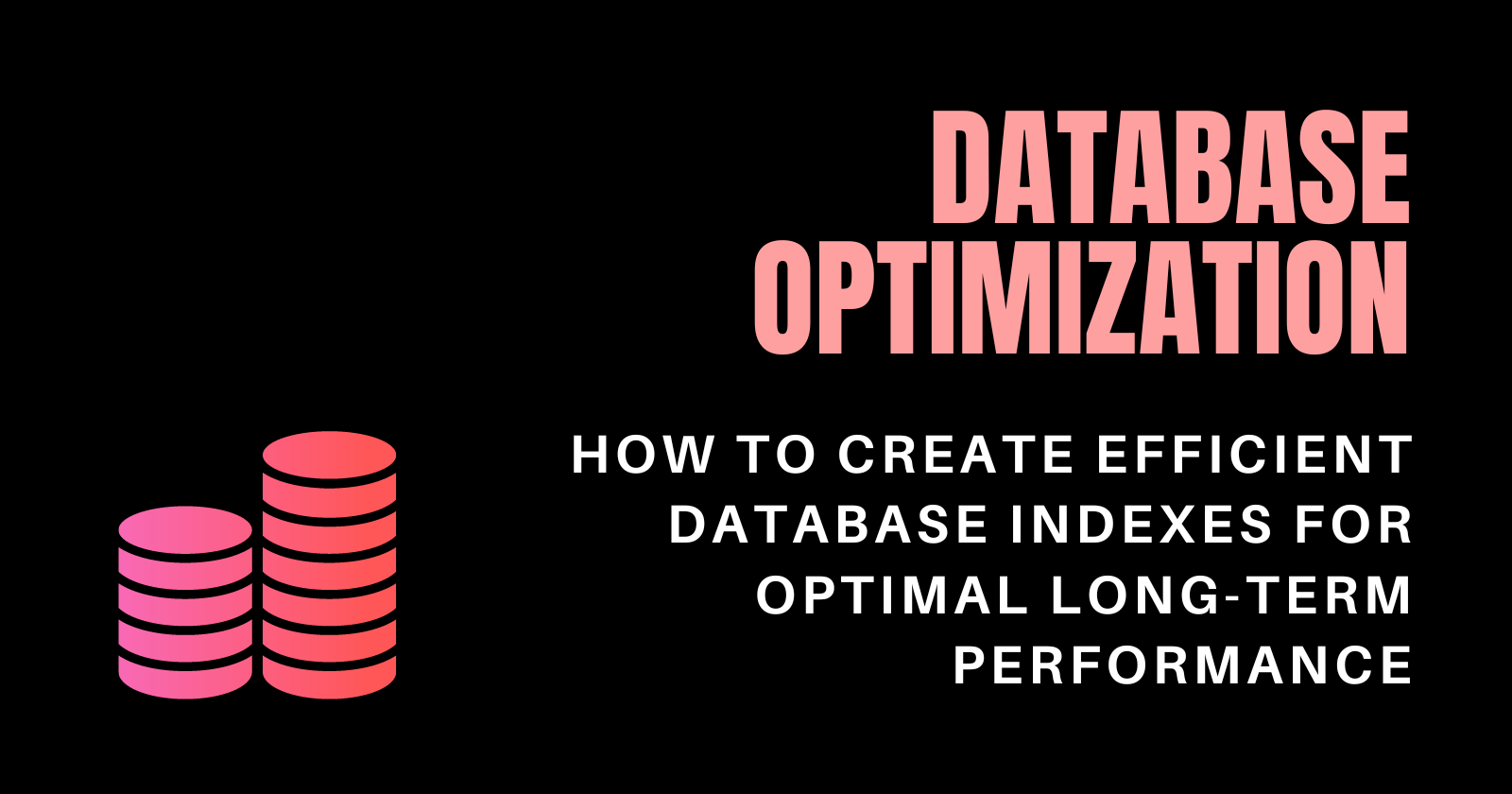
When building a database-driven application, especially one that deals with large datasets, efficient indexing is key to maintaining performance as your data grows. Poorly designed indexes can lead to slow queries, bloated storage, and frustrating user experiences. On the other hand, well-planned indexes can drastically improve query performance and reduce database load, ensuring your system scales smoothly over time.
In this post, we’ll dive deep into the importance of indexes, how to create better indexes, and the long-term impact on performance, using a social media application that manages posts as an example.
What Are Indexes?
An index is a data structure that improves the speed of data retrieval operations on a database table at the cost of additional storage and maintenance overhead. Think of it like an index in a book—it helps you find the information you need without flipping through every page.
While indexes can speed up read operations (queries), they can slow down write operations (inserts, updates, and deletes) because the index must be updated whenever the data in the table changes.
How Indexes Impact Long-Term Performance
As your data grows, simple queries that once ran quickly can become painfully slow. This is where indexing comes into play—by organizing your data in a way that allows the database to find it quickly, you avoid full table scans, which can take exponentially longer as the dataset grows.
In the long run, well-designed indexes ensure:
Faster query execution: Even with millions or billions of rows, the database can fetch the required data efficiently.
Reduced server load: Indexes reduce CPU and I/O operations, meaning the database can serve more queries with the same hardware.
Scalability: As your application grows, properly indexed databases can handle more users and larger datasets without performance degradation.
However, over-indexing or creating unnecessary indexes can result in:
Increased storage: Indexes consume disk space, so it’s important to only index the necessary columns.
Slower writes: Every time data is written to the table, all relevant indexes must be updated, which can lead to slower inserts and updates.
Now, let's go through an example of designing efficient indexes using a social media posts database.
Example: Indexing a Social Media Posts Table
Note: Using postgres compatible SQL here.
Imagine you’re building a social media platform where users can post content, and the system tracks likes, comments, and shares for each post. You have a table for posts that looks like this:
CREATE TABLE posts (
id SERIAL PRIMARY KEY,
user_id IN,
content TEXT,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP,
like_count INT DEFAULT 0,
comment_count INT DEFAULT 0,
share_count INT DEFAULT 0
);
Users typically perform the following operations:
Retrieve posts made in the last 24 hours, ordered by the number of likes.
Find all posts by a specific user, ordered by the most recent.
Fetch the most popular posts based on likes, comments, or shares, regardless of when they were posted.
Let's walk through how you can index this table for these operations.
1. Retrieving Recent Posts, Ordered by Likes
SELECT * FROM posts
WHERE created_at > NOW() - INTERVAL '1 day'
ORDER BY like_count DESC;
Without an index, this query will require a full table scan, which means the database has to check every row in the posts
table to find the relevant records. As your table grows, this becomes inefficient.
Solution: Create a composite index on created_at
and like_count
.
CREATE INDEX idx_posts_created_at_like_count
ON posts (created_at, like_count DESC);
Why this index works: The index allows the database to first filter the rows by
created_at
(to get posts from the last 24 hours) and then order them bylike_count
in descending order.Impact: This index speeds up retrieval of posts based on recent activity, which is a common query pattern in social media applications.
2. Finding Posts by a Specific User
SELECT * FROM posts
WHERE user_id = 123
ORDER BY created_at DESC;
If you don’t index user_id
, the database will need to scan the entire table to find the posts for user 123
. Again, this becomes slower as the table grows.
Solution: Create an index on user_id
and created_at
.
CREATE INDEX idx_posts_user_id_created_at
ON posts (user_id, created_at DESC);
Why this index works: The index will help the database quickly find all posts by the specified user and then sort them by
created_at
in descending order.Impact: Querying for posts by specific users becomes fast, which is a common use case in most social media apps.
3. Fetching Popular Posts Based on Likes, Comments, or Shares
SELECT * FROM posts
ORDER BY like_count DESC, comment_count DESC, share_count DESC;
Without an index, this query could potentially scan and sort all rows in the table, which becomes very slow for large datasets.
Solution: Create a composite index on like_count
, comment_count
, and share_count
.
CREATE INDEX idx_posts_popularity
ON posts (like_count DESC, comment_count DESC, share_count DESC);
Why this index works: The index allows the database to directly retrieve posts ordered by their popularity metrics (likes, comments, and shares) without scanning the whole table.
Impact: This index improves the performance of retrieving posts based on engagement, which is crucial for highlighting popular content.
Best Practices for Creating Indexes
Index Columns You Query Frequently: Only index columns that are frequently used in
WHERE
,ORDER BY
, orJOIN
clauses. Indexing unnecessary columns wastes storage and increases write overhead.Use Composite Indexes for Multiple Columns: If your queries filter by multiple columns or need data ordered by multiple columns, consider composite indexes. However, the order of columns in the index matters—always put the most selective column first.
Monitor and Remove Unused Indexes: Unused indexes take up space and slow down
INSERT
andUPDATE
operations. Regularly monitor index usage and remove any that are no longer needed.Understand Indexing Overhead: Indexes make read operations faster but slow down write operations. Always consider this trade-off when adding an index.
Leverage Partial Indexes for Large Tables: For very large tables, consider using partial indexes that only index a subset of rows. For example, you could index only the most recent posts:
CREATE INDEX idx_recent_posts ON posts (like_count DESC) WHERE created_at > NOW() - INTERVAL '1 month';
This index only applies to posts from the last month, making it much smaller and more efficient for certain queries.
Long-Term Impact of Good Indexing
Efficient indexing ensures your application can handle:
Scalability: As your user base grows and data accumulates, well-indexed tables maintain high performance.
Faster Read Operations: Queries that previously took minutes (or more) on large datasets can be reduced to milliseconds with the right indexes.
Better User Experience: Users expect real-time or near-instantaneous results, and efficient indexing is key to delivering a responsive experience.
On the flip side, poorly indexed databases can lead to:
Slow Queries: Without the right indexes, queries that scan large tables become bottlenecks.
Increased Server Costs: Slow queries put unnecessary load on the database server, leading to higher cloud costs or the need for expensive hardware upgrades.
Conclusion
Indexes are a powerful tool to ensure the long-term scalability and performance of your application. However, creating efficient indexes requires careful consideration of your query patterns and the trade-offs between read and write performance.
By following best practices and regularly reviewing your indexing strategy, you can build an application that scales gracefully and delivers a great experience to users, even as the underlying data grows.
Subscribe to my newsletter
Read articles from Aditi Polkam directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Aditi Polkam
Aditi Polkam
I write on how to build better backend for your apps