Understanding Java Data Structures: Stacks, Queues, Lists, and Trees.
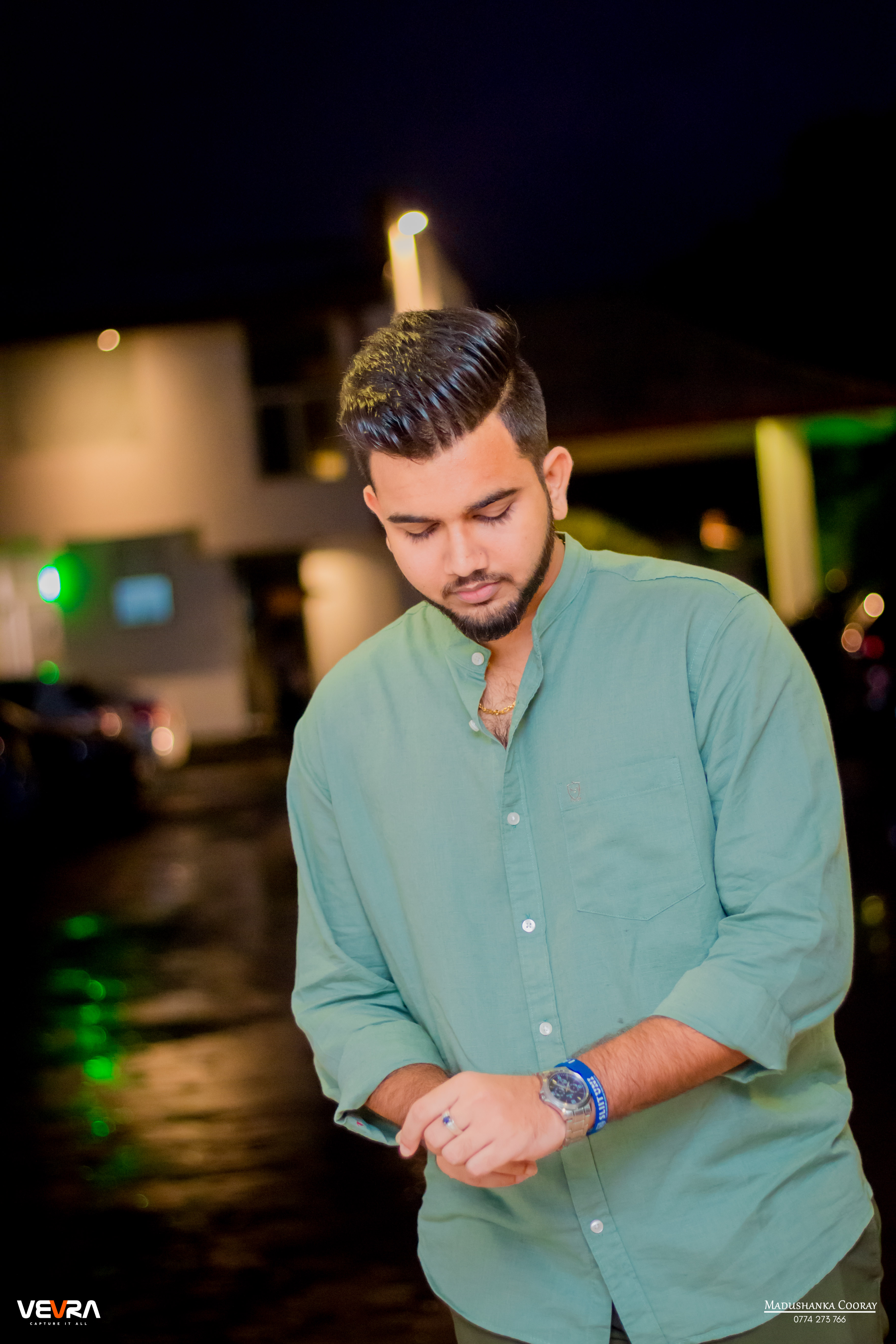
Java is a sophisticated programming language and comes with a variety of data structures to make managing data easy. Learning these data structures is important for all computer science oriented students as they are the foundation of algorithms and development of software. In this blog, we will cover the four main basic data structures in Java namely stacks, queues, lists and trees.
Stacks
A stack is a sequential arrangement of things whereby the principle of Last In First Out (LIFO) is maintained. This means that any unit of storage is last in, will be first out. Stacks are used in many cases such as: Where function calls are involved: In a program, the call stack manages all active function that has been called but their execution has not completed.
Backtracking algorithms: Stacks can be used to navigate through potential solutions before retracting steps due to the impossibility of finding a solution. Implementation in Java In Java, the Stack class found in the java.util package may be utilized:
Queues
A queue is a different linear structure, but one that works under the First In First Out (FIFO) rule. In other words, the person waiting in line, that is the first one, is the first one to be served. Queues are used in:
Processing of orders: Handling the requests made in a service-oriented system. BFS: In graph theory, a queue is used to traverse the graph level by level. Tackling wrecks in Java In java, the Queue interface and its sections such as LinkedList or ArrayDeque can be used:
Lists
A list is considered a collection of elements which can be accessed by their index. It is flexible in that it can hold items of different kinds. In Java, the List interface provides several implementations such as ArrayList and LinkedList. Lists are meant for:
Changing the size of an array: This is a situation where an array has to be created whose size is variable. Order of elements: Lists, unlike sets, maintain the order of the elements. Using Lists in Java This is how to work with an ArrayList:
Trees
A tree can be described as a structure consisting of nodes organized in a tree-like hierarchy such that every node except the root has one parent node and may have several children nodes. Trees are applied in various uses including:
File management systems: Used to model structure of folders and files. Databases: arranging and indexing information in order to be retrieved faster.
Types of Trees Binary Tree: A tree in which every node can have either 0, 1 or 2 children at once. Binary Search Tree: A binary tree impressed with a condition where left child is less than the parent and right is greater than the parent. AVL Trees: A type of self-adjusting binary search tree.
Implementation in Java Here is a basic illustration of a binary tree:
Final Words Familiarisation with different data structures such as stacks, queues, lists, trees, etc., is essential when one is learning how to program in Java. Every structure has its own characteristics and application which makes them effective in solving different problems. As you progress in your studies, I would urge you to look for more advanced data structures and algorithms that will help advance your programming and problem-solving skills.
Subscribe to my newsletter
Read articles from Akash De Alwis directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
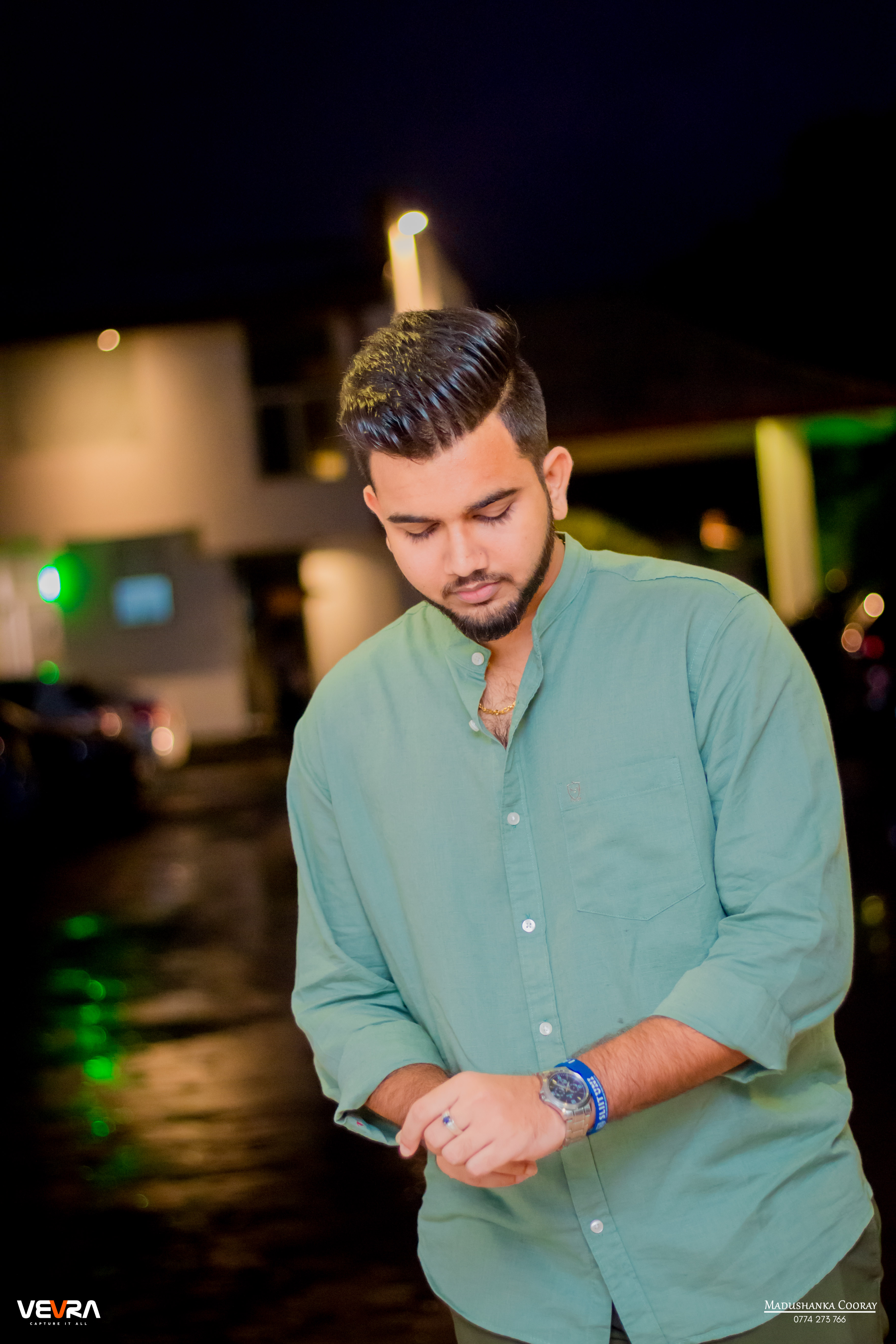
Akash De Alwis
Akash De Alwis
Undergraduate student ๐๐ค