In-Depth Guide to Java Reflection: Unveiling the Power Behind the Curtains

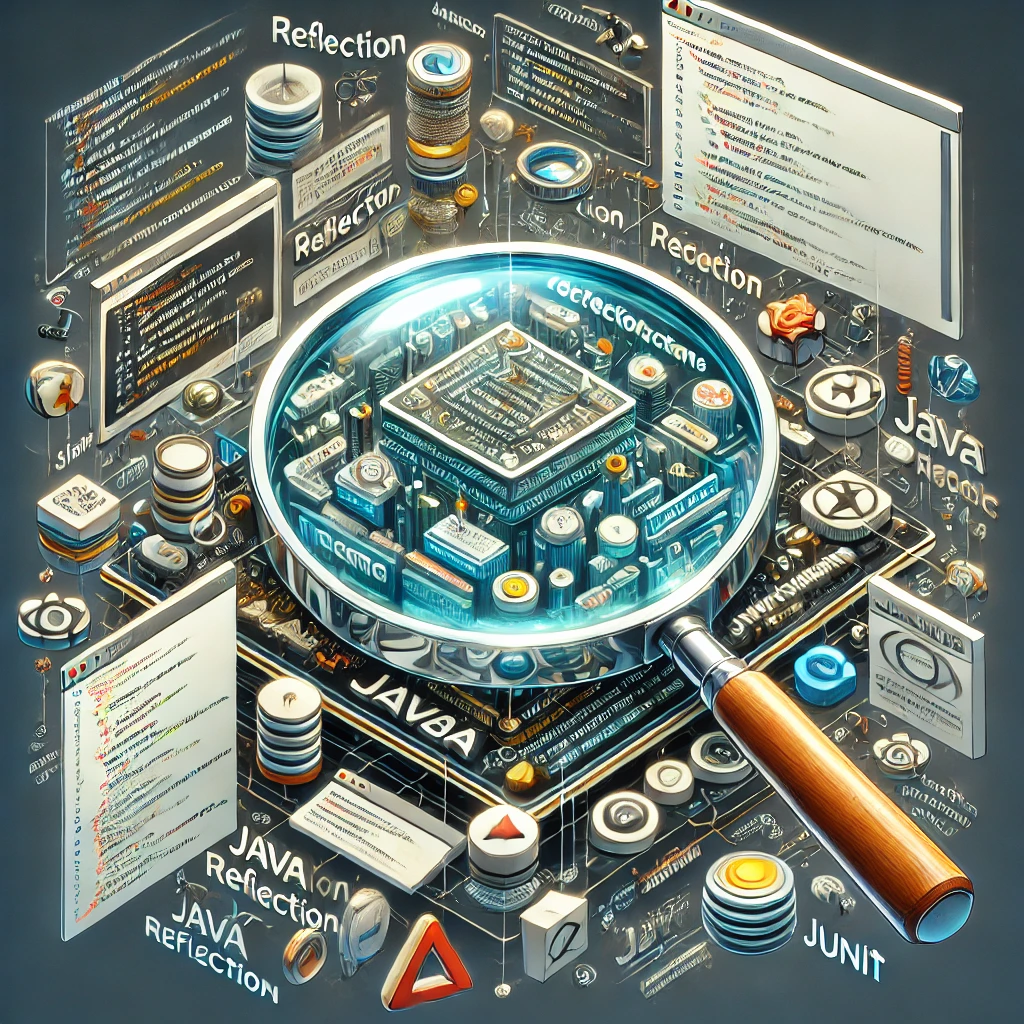
Java Reflection is a powerful tool in the Java programming language that allows developers to inspect and manipulate classes, methods, and fields at runtime, even if they are private. While often associated with advanced programming, reflection is also essential for various common operations like frameworks, libraries, and dependency injection. In this blog, we will explore what Java Reflection is, how it works, and when to use it (or avoid it), with detailed examples along the way.
What is Java Reflection?
Reflection is a feature in Java that allows a program to inspect or modify its structure at runtime. It’s a mechanism by which an object or class’s internal properties—such as fields, methods, or constructors—can be accessed dynamically, without prior knowledge of their names during compilation.
Example Use Cases:
Frameworks like Spring, Hibernate use reflection extensively to inject dependencies, proxy objects, or map fields to database columns.
Serialization libraries convert objects to JSON or XML using reflection to access fields.
Test frameworks like JUnit use reflection to discover and invoke test methods dynamically.
How Java Reflection Works: Key Concepts
To work with reflection in Java, the key classes provided in the java.lang.reflect
package are:
Class<T>
: Represents the blueprint of the class (and its methods, fields, constructors).Field
: Represents a field in a class.Method
: Represents a method in a class.Constructor
: Represents a constructor in a class.
You can obtain a Class
object either through an instance or by using the class name:
// From an object instance
Class<?> objClass = obj.getClass();
// From the class name
Class<?> objClass = Class.forName("com.example.MyClass");
Once you have the Class
object, you can explore its fields, methods, constructors, and annotations.
Basic Operations with Java Reflection
1. Accessing Fields
With reflection, you can access fields (including private fields) at runtime.
import java.lang.reflect.Field;
class MyClass {
private String name = "Reflection in Java";
}
public class ReflectionExample {
public static void main(String[] args) throws Exception {
MyClass obj = new MyClass();
Class<?> objClass = obj.getClass();
// Accessing private field
Field field = objClass.getDeclaredField("name");
field.setAccessible(true); // Enable access to private field
String fieldValue = (String) field.get(obj);
System.out.println("Field Value: " + fieldValue); // Reflection in Java
// Modify the field
field.set(obj, "Updated using Reflection");
System.out.println("Updated Field Value: " + field.get(obj)); // Updated using Reflection
}
}
Explanation:
getDeclaredField()
fetches any field, including private ones.setAccessible(true)
bypasses Java’s access control checks, allowing modification of private fields.
2. Invoking Methods
You can invoke methods dynamically using reflection. This includes both public and private methods.
import java.lang.reflect.Method;
class MyClass {
private void printMessage(String message) {
System.out.println("Message: " + message);
}
}
public class ReflectionExample {
public static void main(String[] args) throws Exception {
MyClass obj = new MyClass();
Class<?> objClass = obj.getClass();
// Accessing private method
Method method = objClass.getDeclaredMethod("printMessage", String.class);
method.setAccessible(true); // Allow access to private method
// Invoke method
method.invoke(obj, "Hello, Reflection!"); // Message: Hello, Reflection!
}
}
Explanation:
getDeclaredMethod()
is used to fetch the method by its name and parameter types.invoke()
is used to call the method on the object, passing the required arguments.
3. Accessing Constructors
Using reflection, you can also access constructors and instantiate objects.
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
class MyClass {
private String name;
public MyClass(String name) {
this.name = name;
}
public String getName() {
return this.name;
}
}
public class ReflectionExample {
public static void main(String[] args) throws Exception {
Class<?> objClass = MyClass.class;
Field[] field = objClass.getFields();
// field.setAccessible(true);
// Get constructor with a single String argument
Constructor[] constructorsList = objClass.getDeclaredConstructors();
// Instantiate object using constructor
for(Constructor myclassCons: constructorsList) {
myclassCons.setAccessible(true);
MyClass obj = (MyClass) myclassCons.newInstance("Reflection Object");
System.out.println("Object Created with name: " + obj.getName());
}
}
}
When to Use Reflection?
Advantages:
Framework Development: Many Java frameworks like Spring, Hibernate, and JUnit heavily rely on reflection to offer flexible configurations and object manipulation.
Dynamic Code Execution: Reflection allows dynamic invocation of methods or access to fields without compile-time knowledge of them.
Testing: It helps test private methods and fields by bypassing access restrictions.
Disadvantages:
Performance Overhead: Reflection involves types of operations that are resolved at runtime, which incurs performance penalties.
Security Concerns: By accessing private members, reflection breaks encapsulation, increasing security risks.
Compile-time Safety: Reflection defeats compile-time type checking, making code more prone to runtime errors.
Best Practices:
Use Sparingly: Avoid using reflection in performance-critical code or regular business logic. It is best reserved for libraries, frameworks, or situations requiring high flexibility.
Use Access Control Responsibly: Overuse of
setAccessible(true)
can lead to security vulnerabilities, so it should be used with care.Prefer Alternatives: If possible, use other language features such as interfaces, generics, or polymorphism instead of reflection for everyday tasks.
Java Reflection utilizes the Interpreter and Proxy design patterns. Let's explore both:
1. Interpreter Pattern
The Interpreter design pattern is used to evaluate and interpret structures at runtime. Java Reflection interprets metadata about classes, methods, fields, and other elements during the runtime of a program.
When you call methods like
getDeclaredFields()
,getMethods()
, orgetConstructor()
, reflection interprets this structure dynamically based on the runtime state rather than compile-time knowledge.
2. Proxy Pattern
The Proxy pattern is used to provide a placeholder or substitute for another object to control access to it. Reflection is used in frameworks like Spring, Hibernate, and JUnit to create proxy classes at runtime.
In dynamic proxying, reflection allows frameworks to intercept method calls, add behaviors (such as logging, security, etc.), or delegate them to different objects without knowing about them at compile-time.
The
java.lang.reflect.Proxy
class is explicitly designed to create proxy instances that implement interfaces dynamically during runtime.
Conclusion
Java Reflection is a powerful and flexible feature that allows runtime inspection and manipulation of code, making it indispensable for framework developers. However, it comes with trade-offs in terms of performance and security, so it should be used wisely. Understanding when and how to use reflection will empower you to build dynamic, flexible, and efficient systems while minimizing potential drawbacks.
By peeling back the layers of your code and understanding how reflection works, you'll unlock new possibilities in the way you design and interact with your Java applications.
Subscribe to my newsletter
Read articles from krishna chaitanya directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
