Python Functions Explained: Day 17 Highlights

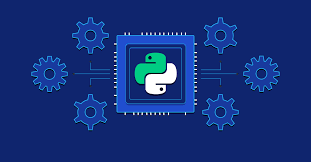
Introduction :
Welcome back to my Python journey! Yesterday, I laid the foundation with control structures in python.
Today, I dove into Function arguments, Let's explore what I learned!
Function
ARGUMENT OR PARAMETER
. to enjoy the local attribute of one function in another function .
parameter or argument is basically two types in python .
ACTUAL PARAMETER
FORMAL PARAMETER
. the parameter related to function call is known as actual parameter.
. the parameter related to function definition is known as formal param.
. actual parameter name and formal parameter name may be same or may be different.
. the name used for parameter is an Identifier.
. the name of parameter will be decided by the user as per the demand of situation.
. parameter can be any type of object in python
. we can use any type of object as parameter in python.
parameter predefined like int , float , complex , bool, NoneType ,list , tuple , str , set , dictionary ,range , frozenset , user defined type .
we can use N of object as parameter
def main () :
a = 10
b = 1.2
c = "india"
d = [ 11, 22, 33 ]
print ( 'i am main enjoying ' , a , b , c , d )
bbsr ( a , b , c , d ) # function call statement
def bbsr ( a , b , c , d ) : # function def statement
print ( 'i am bbsr enjoying ' , a , b , c , d )
main ()
. function definition is not allowed inside another function in c ,cpp, java, but this is permitted in python.
. nested function mechanism is not supported in c, cpp ,java but this is supported in python.
def main () : print ( 'i am main !') def fun () : #nested fun print ( 'i am local fun to main !!!') fun () bbsr ( ) def bbsr ( ) : print ( 'i am bbsr !') main ()
. in python we can use function as actual parameter also as formal parameter
i.e, function is technically called FIRST CLASS OBJECT.
def main () :
print ( 'i am main !')
def fun () :
print ( 'i am local fun to main !!!')
fun ()
bbsr ( fun ) # actual param
def bbsr ( fun ) : # formal param
print ( 'i am bbsr !')
fun ()
main ()
nested function
. nested function can enjoy the attribute of that function where it is nested without gobal keyword or parameter mechanism.
. nested function is not permitted to modify the attribute of that function where is it nested, if try to modify it will raise UnboundLocalError exception.
. to modify the attribute by nested function of that function where it is nested nonlocal keyword is used in python.
def function () : var = 10 print ( 'i am function enjoy ' , var ) def nestedFunction () : nonlocal var var = var + 10 print ( 'i am nested function enjoy ', var ) nestedFunction () function ()
Challenges :
Understanding global and local variable.
Handling errors.
Resources :
Official Python Documentation: Functions
W3Schools' Python Tutorial: Functions
Scaler's Python Course: Functions
Goals for Tomorrow :
Explore something more on Functions.
what is call by value and call by reference.
Conclusion :
Day 17’s a success!
What are your favorite Python resources? Share in the comments below.
Connect with me :
GitHub: [ https://github.com/p-archana1 ]
LinkedIn : [ linkedin.com/in/archana-prusty-4aa0b827a ]
Join the conversation :
Share your own learning experiences or ask questions in the comments.
HAPPY LEARNING!!
THANK YOU!!
Subscribe to my newsletter
Read articles from Archana Prusty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Archana Prusty
Archana Prusty
I'm Archana, pursuing Graduation in Information technology and Management. I'm a fresher with expertise in Python programming. I'm excited to apply my skills in AI/ML learning , Python, Java and web development. Looking forward to collaborating and learning from industry experts.