Git and Github

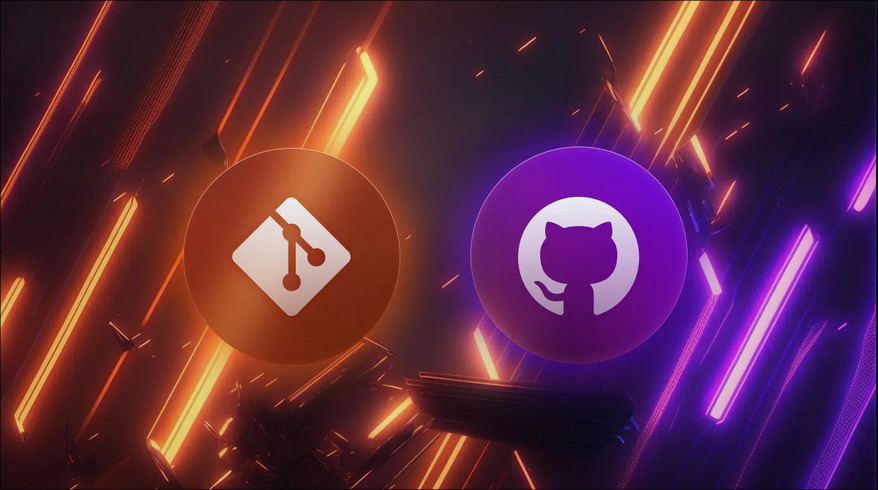
Imagine you're working on a puzzle. You're arranging pieces, but suddenly realize you misplaced a few earlier. Wouldn't it be great if you could hit "undo" and try again? Well, in the world of software development, we deal with code instead of puzzle pieces. And what if I told you that there's a tool that lets you go back in time, make changes, and even work with your friends on the same puzzle? Welcome to the world of Version Control!
What is Version Control?
Version Control is like a magical undo button for developers. It lets you track changes in your code, allowing you to revert to previous versions or even compare different versions side by side. Think of it as a timeline of your project, where each change is carefully recorded and can be revisited at any point.
Overview of Git
In comes Git, the superhero of version control systems. Git helps you manage and track changes in your code, but what sets it apart is that it does this in a decentralized way. This means you and your team can work on different parts of the same project, independently, without stepping on each other's toes. Git lets you save different versions of your code (called "commits") and even creates "branches" to keep different features or ideas separate.
Overview of GitHub
But Git doesn't work alone. Enter GitHub, Git's partner in crime. GitHub is a platform that allows developers to store their Git repositories online, making collaboration easier than ever. It's like a social media platform, but instead of selfies and memes, it's filled with code, projects, and collaboration tools. Whether you're working solo or with a team, GitHub makes sharing code, reviewing changes, and working on projects together a breeze.
Git Basics: Starting with the Essentials
Now that you know the stars of the show, let’s dive into how you can get started with Git and GitHub.
Installing Git
To use Git, you need to install it on your computer. Don't worry, it's super easy. For most systems, a simple command or download from the official Git website will do the trick. Once installed, you're ready to start your coding journey!
Git Configuration (Username, Email, Aliases)
Before jumping in, Git needs to know who you are. This is where you'll configure your username and email. These details will appear in every change you make, so your team knows who wrote what. You can also set up helpful aliases (shortcuts) to make your Git commands quicker to type.
git config --global user.name "Your Name"
git config --global user.email "youremail@example.com"
Understanding Repositories
A repository (or repo for short) is like a folder for your project. It contains your code, files, and every change you've ever made. You can create a new repository using Git, where all your work will live.
Basic Git Workflow: Your Day-to-Day Git Operations
Here’s how you’ll typically use Git on any project:
git init – This command initializes a new Git repository. It's like starting a fresh diary where your code will live and grow.
git add – Once you make changes to your code, you'll use
git add
to stage them, marking them ready for the next save.git commit – After adding your changes, you use
git commit
to save them permanently. It's like taking a snapshot of your project at that exact moment.git status – This handy command shows you what's changed in your project and which files are ready to be committed.
Viewing Commit History: git log
Imagine flipping through the pages of your code diary. The git log
command shows you all the commits you've made, complete with dates, messages, and details of what was changed.
Branching and Merging: Working on Multiple Features
Branches in Git are like parallel worlds. They let you work on new features without affecting the main project. Here's how you can use them:
Creating Branches: You create a branch to work on a specific feature. It's like creating a parallel timeline where you can experiment freely.
Switching Between Branches: Once your new feature is ready, you can switch back to the main timeline and bring your new changes along.
Merging Branches: After finishing work on your branch, you merge it into the main branch, adding all the changes you've made.
Resolving Merge Conflicts: Sometimes, two branches change the same piece of code differently. This leads to a conflict, which Git asks you to resolve manually.
Working with Remote Repositories: Going Global
Now that you've mastered working locally, let's talk about collaborating using remote repositories.
Introduction to Remotes
A remote is like an online version of your Git repository, stored on GitHub. This allows you to sync your work between your computer and GitHub.
Adding Remote Repositories: Use
git remote add
to link your local repo to a GitHub repo.Pushing Changes: When you're ready to share your changes, you push them to the remote repository using
git push
.Fetching and Pulling Changes:
git fetch
brings down changes from the remote repo but doesn’t apply them. On the other hand,git pull
fetches and merges them automatically.Cloning Repositories: Want to work on an existing project? Use
git clone
to download a remote repository to your local machine.
GitHub Basics: Bringing Your Code Online
What is GitHub?
GitHub is like a cloud-based house where your repositories live, share, and grow. It's where the magic of collaboration happens.
Creating a GitHub Account
First, create an account on GitHub.com. Once you're set up, you can start creating repositories, collaborating, and contributing to projects!
Creating a Repository on GitHub
Think of creating a repo on GitHub as setting up a new home for your project. It gives your code an online space to live and be shared.
Connecting Local Repositories to GitHub
To connect your local Git repo to the GitHub repo, you'll use a simple command that links them together. Now, whatever changes you make locally can be pushed to GitHub.
Git and GitHub Collaboration
Forking a Repository: Want to work on someone else's project? Forking creates a copy that you can modify and contribute to.
Contributing to Open Source: Once you fork a project and make changes, you can submit a Pull Request to propose your updates.
GitHub Actions: Want to automate tests or deployments? GitHub Actions lets you set up workflows that trigger automatically.
Advanced Git Techniques: Next-Level Skills
Stashing Changes: Need to switch gears but aren’t ready to commit?
git stash
lets you save your work temporarily.Reverting Commits: Mistake in your last commit?
git revert
orgit reset
will help undo those changes.Rebasing: Want to clean up your commit history?
git rebase
can help tidy things up before merging.
GitHub Advanced Features
GitHub has so much more to offer! From GitHub Pages (free website hosting), GitHub CLI, to GitHub Sponsors, it’s an ecosystem that grows with you.
Conclusion: Git and GitHub
Whether you're coding solo or with a team, Git and GitHub are your best friends in managing and sharing code. These tools aren't just about version control—they're about collaboration, automation, and taking your development process to the next level. Happy coding!
Subscribe to my newsletter
Read articles from Gaurav Daultani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Gaurav Daultani
Gaurav Daultani
I'm on a journey of learning DevOps, cloud technologies, and mastering Linux. With a strong curiosity and a passion for growth, I’m always eager to explore new challenges and improve my skills.