Building Reusable Web Components in a Spring Boot Thymeleaf Project

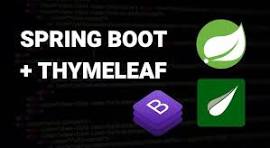
By- Tanish Dwivedi
LinkedIn - https://www.linkedin.com/in/tanish-dwivedi-java/
Twitter- https://x.com/tanish_dwivedii
In today’s web development landscape, modular and reusable components are crucial for maintaining clean, scalable, and efficient applications. Web Components, a set of standardized APIs for creating custom HTML elements, provide a powerful way to achieve this. When combined with Spring Boot and Thymeleaf, you can easily integrate reusable components into your dynamic web applications.
This blog will guide you through building reusable web components within a Spring Boot Thymeleaf project. We’ll cover the foundational setup, how to create web components, and their integration into Thymeleaf templates.
Prerequisites
To follow along, you should have:
Basic knowledge of Spring Boot and Thymeleaf.
Familiarity with HTML, CSS, and JavaScript.
An understanding of Web Components is helpful but not mandatory.
Table of Contents
What are Web Components?
Setting up Spring Boot Thymeleaf Project
Creating a Web Component
Integrating Web Components in Thymeleaf Templates
Benefits of Using Web Components in a Spring Boot Application
Conclusion
1. What Are Web Components?
Web Components are a set of web platform APIs that allow you to create reusable and encapsulated custom HTML elements. They consist of three main technologies:
Custom Elements: Define new HTML elements.
Shadow DOM: Encapsulates the component’s internal structure and styling.
HTML Templates: Declares markup structures that you can reuse.
This standard enables the creation of modular and reusable UI components that work seamlessly across modern web applications.
2. Setting up Spring Boot Thymeleaf Project
To get started, we need to set up a Spring Boot application that uses Thymeleaf as the template engine.
Step 1: Project Initialization
Use Spring Initializr to initialize the project with the following dependencies:
Spring Web: To create web-based applications.
Thymeleaf: For server-side templating.
Alternatively, you can use the following Maven structure:
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
</dependencies>
Step 2: Directory Structure
After creating the project, your directory structure should look something like this:
src/
├── main/
│ ├── java/
│ │ └── com.example.demo/
│ │ └── DemoApplication.java
│ ├── resources/
│ │ ├── templates/
│ │ │ └── index.html
│ │ └── static/
│ │ ├── css/
│ │ └── js/
Blog Title: Building Reusable Web Components in a Spring Boot Thymeleaf Project
In today’s web development landscape, modular and reusable components are crucial for maintaining clean, scalable, and efficient applications. Web Components, a set of standardized APIs for creating custom HTML elements, provide a powerful way to achieve this. When combined with Spring Boot and Thymeleaf, you can easily integrate reusable components into your dynamic web applications.
This blog will guide you through building reusable web components within a Spring Boot Thymeleaf project. We’ll cover the foundational setup, how to create web components, and their integration into Thymeleaf templates.
Prerequisites
To follow along, you should have:
Basic knowledge of Spring Boot and Thymeleaf.
Familiarity with HTML, CSS, and JavaScript.
An understanding of Web Components is helpful but not mandatory.
Table of Contents
What are Web Components?
Setting up Spring Boot Thymeleaf Project
Creating a Web Component
Integrating Web Components in Thymeleaf Templates
Benefits of Using Web Components in a Spring Boot Application
Conclusion
1. What Are Web Components?
Web Components are a set of web platform APIs that allow you to create reusable and encapsulated custom HTML elements. They consist of three main technologies:
Custom Elements: Define new HTML elements.
Shadow DOM: Encapsulates the component’s internal structure and styling.
HTML Templates: Declares markup structures that you can reuse.
This standard enables the creation of modular and reusable UI components that work seamlessly across modern web applications.
2. Setting up Spring Boot Thymeleaf Project
To get started, we need to set up a Spring Boot application that uses Thymeleaf as the template engine.
Step 1: Project Initialization
Use Spring Initializr to initialize the project with the following dependencies:
Spring Web: To create web-based applications.
Thymeleaf: For server-side templating.
Alternatively, you can use the following Maven structure:
xmlCopy code<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
</dependencies>
Step 2: Directory Structure
After creating the project, your directory structure should look something like this:
cssCopy codesrc/
├── main/
│ ├── java/
│ │ └── com.example.demo/
│ │ └── DemoApplication.java
│ ├── resources/
│ │ ├── templates/
│ │ │ └── index.html
│ │ └── static/
│ │ ├── css/
│ │ └── js/
3. Creating a Web Component
Let’s create a simple custom web component—a reusable button that changes color on hover.
Step 1: Define the Custom Element
First, we’ll define a custom HTML element using Custom Elements API and Shadow DOM. Create a JavaScript file under src/main/resources/static/js/
named custom-button.js
:
class CustomButton extends HTMLElement {
constructor() {
super();
const shadow = this.attachShadow({ mode: 'open' });
// Create a button element
const button = document.createElement('button');
button.textContent = this.getAttribute('label') || 'Click Me';
// Add styles
const style = document.createElement('style');
style.textContent = `
button {
background-color: #6200EA;
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s ease;
}
button:hover {
background-color: #3700B3;
}
`;
shadow.appendChild(style);
shadow.appendChild(button);
}
}
// Define the new element
customElements.define('custom-button', CustomButton);
Step 2: Register the Web Component in Thymeleaf
Now, we’ll include this custom button in our Thymeleaf template. Open the index.html
file under src/main/resources/templates/
and add the following code:
<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Web Component Example</title>
<script src="/js/custom-button.js"></script>
</head>
<body>
<h1>Reusable Web Components in Spring Boot Thymeleaf</h1>
<!-- Use the custom button component -->
<custom-button label="Click Me!"></custom-button>
</body>
</html>
Here, we load our custom-button.js
file and use the <custom-button>
tag, passing the label
attribute.
Blog Title: Building Reusable Web Components in a Spring Boot Thymeleaf Project
In today’s web development landscape, modular and reusable components are crucial for maintaining clean, scalable, and efficient applications. Web Components, a set of standardized APIs for creating custom HTML elements, provide a powerful way to achieve this. When combined with Spring Boot and Thymeleaf, you can easily integrate reusable components into your dynamic web applications.
This blog will guide you through building reusable web components within a Spring Boot Thymeleaf project. We’ll cover the foundational setup, how to create web components, and their integration into Thymeleaf templates.
Prerequisites
To follow along, you should have:
Basic knowledge of Spring Boot and Thymeleaf.
Familiarity with HTML, CSS, and JavaScript.
An understanding of Web Components is helpful but not mandatory.
Table of Contents
What are Web Components?
Setting up Spring Boot Thymeleaf Project
Creating a Web Component
Integrating Web Components in Thymeleaf Templates
Benefits of Using Web Components in a Spring Boot Application
Conclusion
1. What Are Web Components?
Web Components are a set of web platform APIs that allow you to create reusable and encapsulated custom HTML elements. They consist of three main technologies:
Custom Elements: Define new HTML elements.
Shadow DOM: Encapsulates the component’s internal structure and styling.
HTML Templates: Declares markup structures that you can reuse.
This standard enables the creation of modular and reusable UI components that work seamlessly across modern web applications.
2. Setting up Spring Boot Thymeleaf Project
To get started, we need to set up a Spring Boot application that uses Thymeleaf as the template engine.
Step 1: Project Initialization
Use Spring Initializr to initialize the project with the following dependencies:
Spring Web: To create web-based applications.
Thymeleaf: For server-side templating.
Alternatively, you can use the following Maven structure:
xmlCopy code<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
</dependencies>
Step 2: Directory Structure
After creating the project, your directory structure should look something like this:
cssCopy codesrc/
├── main/
│ ├── java/
│ │ └── com.example.demo/
│ │ └── DemoApplication.java
│ ├── resources/
│ │ ├── templates/
│ │ │ └── index.html
│ │ └── static/
│ │ ├── css/
│ │ └── js/
3. Creating a Web Component
Let’s create a simple custom web component—a reusable button that changes color on hover.
Step 1: Define the Custom Element
First, we’ll define a custom HTML element using Custom Elements API and Shadow DOM. Create a JavaScript file under src/main/resources/static/js/
named custom-button.js
:
javascriptCopy codeclass CustomButton extends HTMLElement {
constructor() {
super();
const shadow = this.attachShadow({ mode: 'open' });
// Create a button element
const button = document.createElement('button');
button.textContent = this.getAttribute('label') || 'Click Me';
// Add styles
const style = document.createElement('style');
style.textContent = `
button {
background-color: #6200EA;
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s ease;
}
button:hover {
background-color: #3700B3;
}
`;
shadow.appendChild(style);
shadow.appendChild(button);
}
}
// Define the new element
customElements.define('custom-button', CustomButton);
Step 2: Register the Web Component in Thymeleaf
Now, we’ll include this custom button in our Thymeleaf template. Open the index.html
file under src/main/resources/templates/
and add the following code:
htmlCopy code<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Web Component Example</title>
<script src="/js/custom-button.js"></script>
</head>
<body>
<h1>Reusable Web Components in Spring Boot Thymeleaf</h1>
<!-- Use the custom button component -->
<custom-button label="Click Me!"></custom-button>
</body>
</html>
Here, we load our custom-button.js
file and use the <custom-button>
tag, passing the label
attribute.
4. Integrating Web Components in Thymeleaf Templates
Thymeleaf's powerful template engine allows you to use Web Components seamlessly. It integrates with your existing Spring Boot backend, letting you pass dynamic data from controllers to these components.
Example: Passing Dynamic Data
Imagine you want to pass dynamic data (like a user’s name) to your custom button. In your Spring Boot controller (DemoApplication.java
), add the following method:
@Controller
public class HomeController {
@GetMapping("/")
public String index(Model model) {
model.addAttribute("userName", "Tanish");
return "index";
}
}
Now, update your index.html
to dynamically set the button label using Thymeleaf:
<custom-button th:attr="label='Hello, ' + ${userName}"></custom-button>
This integration allows you to combine the dynamic nature of Thymeleaf with the encapsulation of Web Components.
Blog Title: Building Reusable Web Components in a Spring Boot Thymeleaf Project
In today’s web development landscape, modular and reusable components are crucial for maintaining clean, scalable, and efficient applications. Web Components, a set of standardized APIs for creating custom HTML elements, provide a powerful way to achieve this. When combined with Spring Boot and Thymeleaf, you can easily integrate reusable components into your dynamic web applications.
This blog will guide you through building reusable web components within a Spring Boot Thymeleaf project. We’ll cover the foundational setup, how to create web components, and their integration into Thymeleaf templates.
Prerequisites
To follow along, you should have:
Basic knowledge of Spring Boot and Thymeleaf.
Familiarity with HTML, CSS, and JavaScript.
An understanding of Web Components is helpful but not mandatory.
Table of Contents
What are Web Components?
Setting up Spring Boot Thymeleaf Project
Creating a Web Component
Integrating Web Components in Thymeleaf Templates
Benefits of Using Web Components in a Spring Boot Application
Conclusion
1. What Are Web Components?
Web Components are a set of web platform APIs that allow you to create reusable and encapsulated custom HTML elements. They consist of three main technologies:
Custom Elements: Define new HTML elements.
Shadow DOM: Encapsulates the component’s internal structure and styling.
HTML Templates: Declares markup structures that you can reuse.
This standard enables the creation of modular and reusable UI components that work seamlessly across modern web applications.
2. Setting up Spring Boot Thymeleaf Project
To get started, we need to set up a Spring Boot application that uses Thymeleaf as the template engine.
Step 1: Project Initialization
Use Spring Initializr to initialize the project with the following dependencies:
Spring Web: To create web-based applications.
Thymeleaf: For server-side templating.
Alternatively, you can use the following Maven structure:
xmlCopy code<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
</dependencies>
Step 2: Directory Structure
After creating the project, your directory structure should look something like this:
cssCopy codesrc/
├── main/
│ ├── java/
│ │ └── com.example.demo/
│ │ └── DemoApplication.java
│ ├── resources/
│ │ ├── templates/
│ │ │ └── index.html
│ │ └── static/
│ │ ├── css/
│ │ └── js/
3. Creating a Web Component
Let’s create a simple custom web component—a reusable button that changes color on hover.
Step 1: Define the Custom Element
First, we’ll define a custom HTML element using Custom Elements API and Shadow DOM. Create a JavaScript file under src/main/resources/static/js/
named custom-button.js
:
javascriptCopy codeclass CustomButton extends HTMLElement {
constructor() {
super();
const shadow = this.attachShadow({ mode: 'open' });
// Create a button element
const button = document.createElement('button');
button.textContent = this.getAttribute('label') || 'Click Me';
// Add styles
const style = document.createElement('style');
style.textContent = `
button {
background-color: #6200EA;
color: white;
padding: 10px 20px;
border: none;
border-radius: 5px;
cursor: pointer;
transition: background-color 0.3s ease;
}
button:hover {
background-color: #3700B3;
}
`;
shadow.appendChild(style);
shadow.appendChild(button);
}
}
// Define the new element
customElements.define('custom-button', CustomButton);
Step 2: Register the Web Component in Thymeleaf
Now, we’ll include this custom button in our Thymeleaf template. Open the index.html
file under src/main/resources/templates/
and add the following code:
htmlCopy code<!DOCTYPE html>
<html xmlns:th="http://www.thymeleaf.org">
<head>
<title>Web Component Example</title>
<script src="/js/custom-button.js"></script>
</head>
<body>
<h1>Reusable Web Components in Spring Boot Thymeleaf</h1>
<!-- Use the custom button component -->
<custom-button label="Click Me!"></custom-button>
</body>
</html>
Here, we load our custom-button.js
file and use the <custom-button>
tag, passing the label
attribute.
4. Integrating Web Components in Thymeleaf Templates
Thymeleaf's powerful template engine allows you to use Web Components seamlessly. It integrates with your existing Spring Boot backend, letting you pass dynamic data from controllers to these components.
Example: Passing Dynamic Data
Imagine you want to pass dynamic data (like a user’s name) to your custom button. In your Spring Boot controller (DemoApplication.java
), add the following method:
javaCopy code@Controller
public class HomeController {
@GetMapping("/")
public String index(Model model) {
model.addAttribute("userName", "Tanish");
return "index";
}
}
Now, update your index.html
to dynamically set the button label using Thymeleaf:
htmlCopy code<custom-button th:attr="label='Hello, ' + ${userName}"></custom-button>
This integration allows you to combine the dynamic nature of Thymeleaf with the encapsulation of Web Components.
5. Benefits of Using Web Components in a Spring Boot Application
Reusability: Web Components enable you to write reusable and modular components that can be shared across different projects or pages.
Encapsulation: Styles and logic are encapsulated within the Web Component, preventing them from interfering with the rest of your application.
Interoperability: Web Components work seamlessly with any front-end framework (React, Angular, Vue.js) or vanilla JavaScript. Thymeleaf allows smooth integration of server-side rendered templates with client-side components.
Modularity: Using Web Components allows you to create a more maintainable and scalable codebase, as each component can be developed and tested independently.
6. Conclusion
Web Components provide a modern approach to building modular, reusable, and encapsulated UI components. Combining Web Components with Spring Boot and Thymeleaf allows you to leverage server-side templating while integrating interactive and reusable front-end components.
By following the steps in this guide, you can now author Web Components and use them in your Spring Boot applications, adding a new level of modularity and reusability to your projects.
Subscribe to my newsletter
Read articles from Tanish Dwivedi directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
