Essential String Methods in Python β Part 1 π

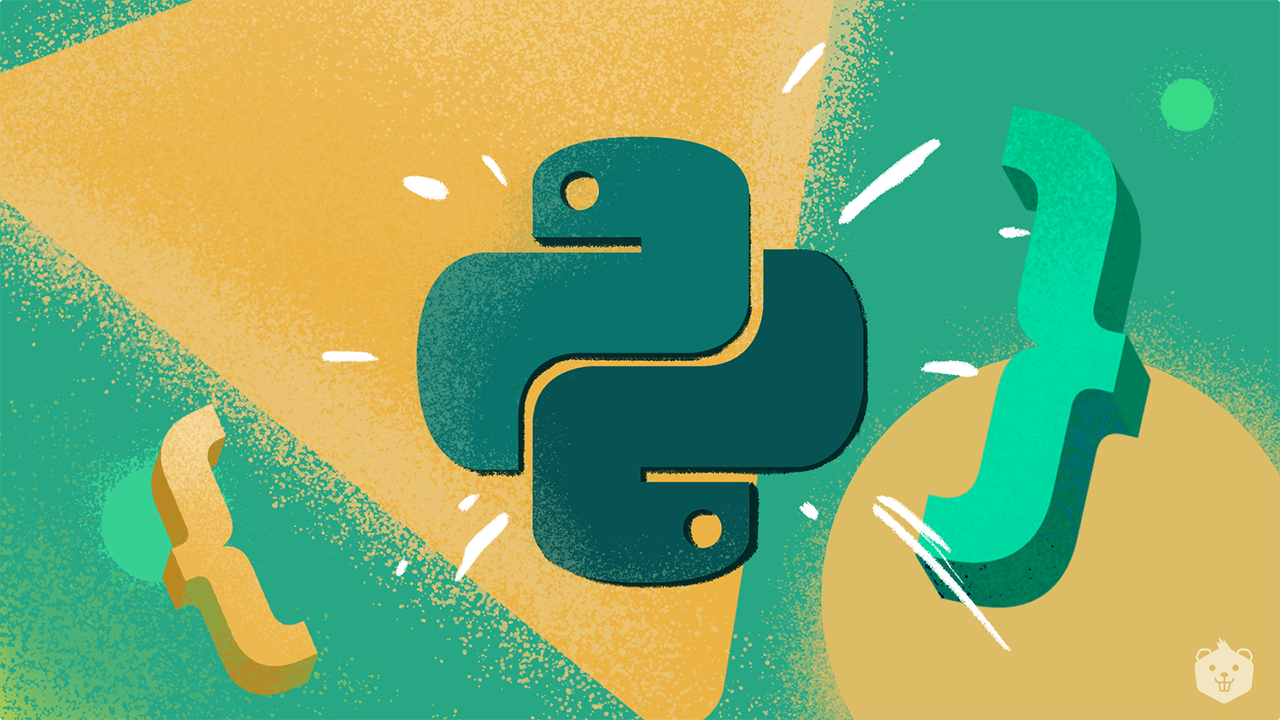
Introduction
String methods in Python make it easy to manipulate and process text-based data. These built-in methods allow you to perform a variety of operations, such as searching, transforming, and validating strings. In this blog, weβll cover some commonly used string methods that will simplify your coding tasks.
1. capitalize()
: Capitalize the First Letter β¨
The capitalize()
method returns a string where the first character is uppercase and all other characters are lowercase.
pythonCopy codetext = "python is fun"
capitalized_text = text.capitalize()
print(capitalized_text) # Output: Python is fun
2. title()
: Capitalize Each Wordβs First Letter π
If you need to capitalize the first letter of each word in a string, use the title()
method.
pythonCopy codetext = "python is fun"
title_text = text.title()
print(title_text) # Output: Python Is Fun
3. swapcase()
: Switch Letter Cases π
The swapcase()
method switches lowercase letters to uppercase and vice versa.
pythonCopy codetext = "Hello World"
swapped_text = text.swapcase()
print(swapped_text) # Output: hELLO wORLD
4. find()
: Find the Position of a Substring π΅οΈββοΈ
The find()
method returns the index of the first occurrence of the specified substring. If the substring is not found, it returns -1
.
pythonCopy codetext = "Python programming"
position = text.find("program")
print(position) # Output: 7
5. count()
: Count the Occurrence of a Substring π
The count()
method returns the number of times a substring occurs in a string.
pythonCopy codetext = "banana"
count_a = text.count('a')
print(count_a) # Output: 3
6. startswith()
and endswith()
: Check Start and End π¦
These methods allow you to check if a string starts or ends with a specific substring.
pythonCopy codetext = "Hello World"
print(text.startswith("Hello")) # Output: True
print(text.endswith("World")) # Output: True
7. replace()
: Replace Substrings βοΈ
The replace()
method replaces a substring within the string with another substring.
pythonCopy codetext = "I love apples"
new_text = text.replace("apples", "bananas")
print(new_text) # Output: I love bananas
8. split()
and join()
: Splitting and Joining Strings π
The split()
method breaks a string into a list of substrings based on a delimiter, while join()
combines a list of strings into one string.
pythonCopy codetext = "Python is fun"
words = text.split(' ') # Split by space
print(words) # Output: ['Python', 'is', 'fun']
joined_text = '-'.join(words)
print(joined_text) # Output: Python-is-fun
In this first part of string methods, we explored some essential tools that help you manipulate and transform strings efficiently. These methods are widely used and can significantly enhance your text processing capabilities. In the next blog, weβll dive deeper into additional string methods that provide even more flexibility and power.
Subscribe to my newsletter
Read articles from Shrey Dikshant directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Shrey Dikshant
Shrey Dikshant
Aspiring data scientist with a strong foundation in adaptive quality techniques. Gained valuable experience through internships at YT Views, focusing on operation handling. Proficient in Python and passionate about data visualization, aiming to turn complex data into actionable insights.