Understanding Throttling in Django: A Friendly Guide to Our Application

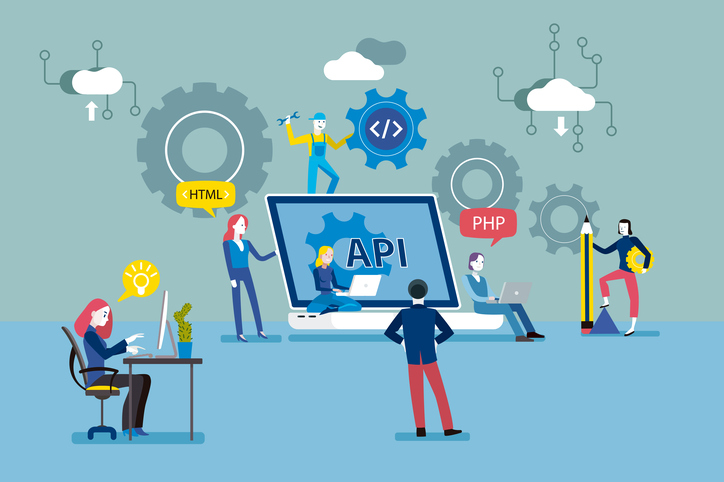
If you've ever used a website or an app that felt slow or unresponsive, you might have experienced the effects of too many users trying to access it at once. This is where throttling comes into play! Throttling is like a traffic cop for web applications, ensuring that users don’t overwhelm the server with requests. In our Django application, we’ve implemented a smart throttling system using the Django REST Framework (DRF) to manage user interactions effectively, particularly for sensitive actions like logging in or accessing user profiles.
What Is Throttling?
Imagine a busy restaurant. If everyone tries to order at the same time, chaos ensues! To keep things running smoothly, the restaurant staff might limit how many customers can place orders every few minutes. Similarly, throttling helps manage how many requests a user can make to our API in a set amount of time. This not only keeps our server happy and healthy but also ensures that everyone gets a fair chance to use the application without frustration.
How Throttling Helps Prevent DoS Attacks
Imagine a crowded concert where too many people are trying to get in at once. If everyone pushes through the entrance, it can create a dangerous situation and keep others from entering. Similarly, in the digital world, a Denial of Service (DoS) attack happens when someone tries to overwhelm a server with too many requests, making it unavailable to legitimate users.
Throttling acts like a bouncer at that concert. By limiting the number of requests a user can make in a given time, we prevent any one person (or automated script) from flooding the server with requests.
How It Works in Our Application
Rate Limits: For instance, with our throttling rules, if a user is limited to 5 login attempts per minute, it helps keep an attacker from trying countless password combinations quickly.
Resource Protection: By controlling how often users can access certain views, like user profiles or drone data, we ensure the server isn't overwhelmed, keeping it available for everyone.
In short, throttling is our way of ensuring that our application remains accessible and responsive, even when faced with malicious attempts to disrupt service.
How We Set Up Throttling
In our Django settings, we’ve configured the throttling behavior using a section called REST_FRAMEWORK. Here’s a peek at how we’ve set it up:
REST_FRAMEWORK = {
'DEFAULT_AUTHENTICATION_CLASSES': [
'rest_framework_simplejwt.authentication.JWTAuthentication',
],
'DEFAULT_THROTTLE_CLASSES': [
'rest_framework.throttling.AnonRateThrottle',
'rest_framework.throttling.UserRateThrottle',
],
'DEFAULT_THROTTLE_RATES': {
'anon': '1/min',
'user': '2/min',
'login': '5/min',
'user_profile': '3/min'
}
}
Key Components
AnonRateThrottle: Limits guests (unauthenticated users) to 1 request per minute. This prevents random users from bombarding our server.
UserRateThrottle: Allows authenticated users to make 2 requests per minute. It gives regular users a bit more leeway while still protecting our resources.
Scoped Throttling: For specific actions, we use a technique called ScopedRateThrottle, which lets us set unique limits for different views.
Why ScopedRateThrottle vs. UserRateThrottle?
When it comes to managing user requests, we have two main options:
UserRateThrottle: This type applies a blanket limit for all authenticated users. For instance, if we set it to 2 requests per minute, it means that any logged-in user can only make 2 requests within that time. This is great for general usage but doesn’t allow us to be very specific about what actions might need tighter controls.
ScopedRateThrottle: This type lets us create custom rules for particular actions. For example, we can impose a stricter limit on login attempts, which is crucial for preventing unauthorized access. This flexibility helps us secure sensitive operations while keeping the rest of the API accessible.
Why Use throttle_scope = ‘login’ in ScopedRateThrottle?
In our LoginView, we set throttle_scope = 'login', allowing users 5 login attempts per minute. Here’s why that’s important:
Stricter Control: By having a specific rule for logins, we can better manage how many times someone can try to log in. This is vital to prevent brute-force attacks, where someone repeatedly tries different passwords to gain access.
Flexibility: This allows us to change the login limits independently of other actions in the API. If we see increased attempts at unauthorized access, we can tighten this limit without affecting other parts of our application.
Why Use throttle_scope = ‘user_profie’ in ScopedRateThrottle?
Similarly, in the UserProfile view, we set throttle_scope = 'user_profile', which limits requests to 3 per minute. Here’s why we do this:
Custom Limits: By creating a specific scope for user profile access, we ensure that users can only make a limited number of requests. This helps protect the application from being overloaded with profile requests, keeping it responsive for everyone.
Resource Management: Retrieving user profiles can sometimes involve more processing and data retrieval than other requests. By limiting the number of requests, we make sure the server can handle these operations smoothly without slowing down.
LoginView: Scoped Throttling in Action
Let’s look at our LoginView class where we enforce this throttling:
class LoginView(APIView):
throttle_scope = 'login' # Define the throttle scope for login
throttle_classes = [ScopedRateThrottle] # Apply scoped throttling to login
By restricting login attempts to 5 per minute, we significantly reduce the risk of someone trying to guess a password by making multiple attempts in a short period.
UserProfile: Customized Throttling
We also have a specific throttle for accessing user profiles, limiting requests to 3 per minute. This is how it looks:
class UserProfile(generics.RetrieveAPIView):
permission_classes = [IsAuthenticated]
throttle_classes = [ScopedRateThrottle]
throttle_scope = 'user_profile'
queryset = User.objects.all()
serializer_class = UserProfileSerializer
This throttling prevents users from overwhelming the profile retrieval endpoint, ensuring our application remains fast and responsive.
DroneListCreate: User-Level Throttling
In the DroneListCreate view, we use UserRateThrottle
:
class DroneListCreate(generics.ListCreateAPIView):
permission_classes = [IsAuthenticated]
throttle_classes = [UserRateThrottle]
queryset = Drone.objects.all()
serializer_class = DroneSerializer
Here, authenticated users can make 2 requests per minute, which keeps drone-related operations efficient without putting too much strain on our resources.
Conclusion
Throttling is a vital part of our Django application, helping us maintain security, optimize performance, and ensure fair resource usage. By customizing throttle rules for specific actions like login attempts, user profile access, and using general limits for other operations, we create a balanced and efficient API environment.
For a deeper dive into the code and to explore our application further, check out my GitHub repository.
Subscribe to my newsletter
Read articles from Wayne Musungu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
