Creating Interactive Data Visualizations with JavaScript and D3.js: A Comprehensive Guide
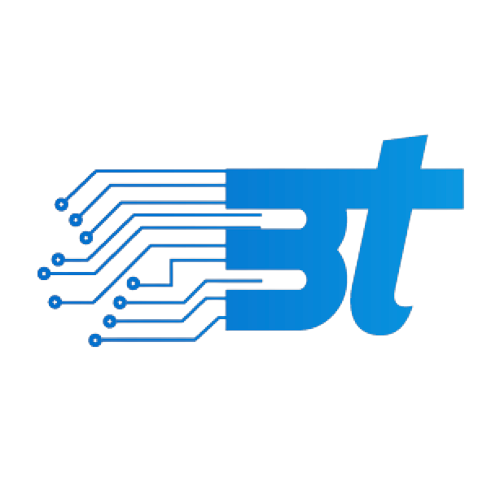
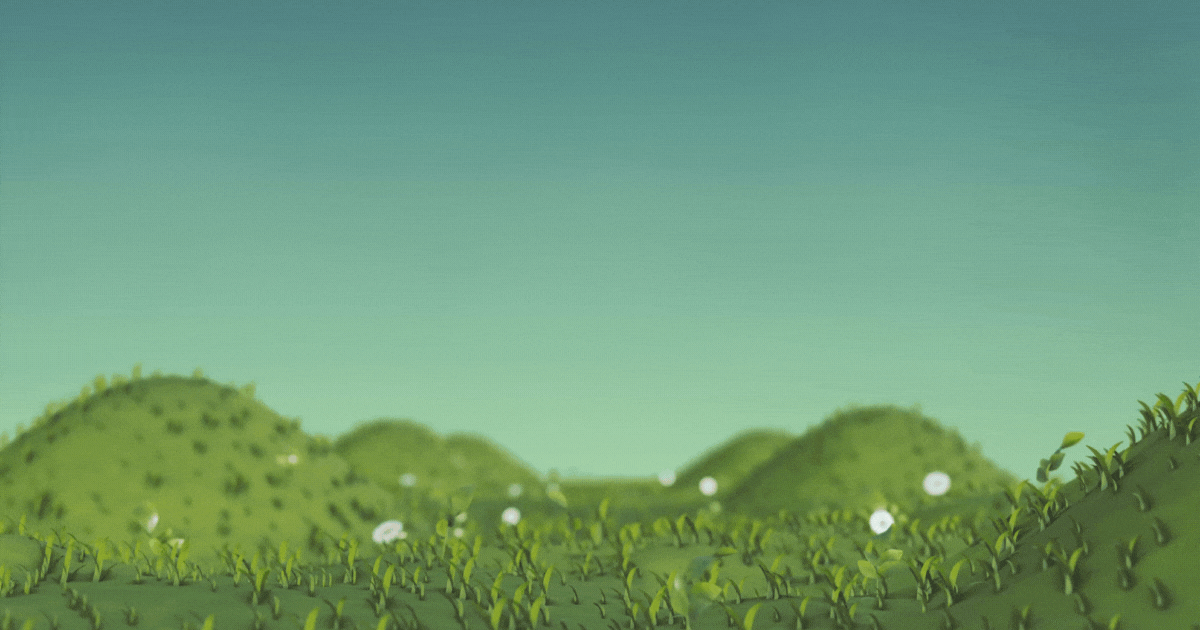
In today's data-driven world, visualizing complex data in an interactive and intuitive manner is crucial for businesses, researchers, and developers. JavaScript, combined with libraries like D3.js (Data-Driven Documents), provides powerful tools for building sophisticated, interactive data visualizations right in the browser.
In this blog, we’ll dive deep into how to create interactive data visualizations using JavaScript and D3.js, from setting up your environment to building bar charts, line graphs, and adding interactive elements like tooltips, transitions, and dynamic data updates.
Why D3.js?
D3.js is a versatile JavaScript library designed for manipulating documents based on data. Unlike other charting libraries, D3 gives you full control over the appearance and behavior of your visualizations. You can:
Bind data to HTML, SVG, and Canvas elements.
Animate transitions and updates.
Create complex visualizations like bar charts, scatterplots, heatmaps, etc.
Build interactive and dynamic visual elements like tooltips and zooming.
Setting Up the Environment
To start using D3.js, you'll need a basic HTML page with a reference to the D3.js library.
Create a basic HTML file:
<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <meta name="viewport" content="width=device-width, initial-scale=1.0"> <title>D3.js Data Visualization</title> <script src="https://d3js.org/d3.v7.min.js"></script> <style> body { font-family: Arial, sans-serif; } svg { background-color: #f9f9f9; } </style> </head> <body> <h1>D3.js Interactive Visualization</h1> <div id="chart"></div> <script src="app.js"></script> </body> </html>
Create a JavaScript file (
app.js
) where we will add our D3.js code.
Building a Simple Bar Chart
Let’s start by creating a basic bar chart. We’ll use D3 to bind data to rect
elements inside an SVG container, scaling the bars based on the data values.
Step 1: Create a Dataset
First, we define our data as an array of values:
const data = [30, 80, 45, 60, 20, 90, 50];
Step 2: Set Up SVG Canvas
We’ll define an SVG canvas where our chart will be drawn:
const width = 500;
const height = 300;
const margin = {top: 20, right: 30, bottom: 40, left: 40};
const svg = d3.select("#chart")
.append("svg")
.attr("width", width)
.attr("height", height)
.style("border", "1px solid black");
Step 3: Create Scales
Scales map your data values to pixel values. For a bar chart, we need to map the data values to bar heights and the index values to x-coordinates.
const xScale = d3.scaleBand()
.domain(d3.range(data.length))
.range([margin.left, width - margin.right])
.padding(0.1);
const yScale = d3.scaleLinear()
.domain([0, d3.max(data)])
.range([height - margin.bottom, margin.top]);
Step 4: Draw Bars
Now we can create the bars by binding our data to rect
elements inside the SVG.
svg.selectAll("rect")
.data(data)
.enter()
.append("rect")
.attr("x", (d, i) => xScale(i))
.attr("y", d => yScale(d))
.attr("width", xScale.bandwidth())
.attr("height", d => height - margin.bottom - yScale(d))
.attr("fill", "steelblue");
At this point, you should see a simple bar chart rendered in the browser.
Adding Axes
Axes are essential for giving context to your visualizations. D3 provides built-in axis generators that make it easy to add x and y axes to your chart.
const xAxis = d3.axisBottom(xScale).tickFormat((d, i) => `Item ${i+1}`);
const yAxis = d3.axisLeft(yScale);
svg.append("g")
.attr("transform", `translate(0,${height - margin.bottom})`)
.call(xAxis);
svg.append("g")
.attr("transform", `translate(${margin.left},0)`)
.call(yAxis);
This adds a labeled x-axis and y-axis to your chart, giving viewers more context about the data being presented.
Adding Interactivity
Let’s enhance the visualization by adding interactivity—specifically, tooltips that show the exact value of each bar when hovered.
Step 1: Create a Tooltip
Add a div
for the tooltip in your HTML:
<div id="tooltip" style="position: absolute; background: lightgray; padding: 5px; display: none;"></div>
Step 2: Add Mouse Events to Bars
Modify the rect
elements to show the tooltip on mouseover:
const tooltip = d3.select("#tooltip");
svg.selectAll("rect")
.on("mouseover", function(event, d) {
tooltip.style("display", "inline-block")
.html(`Value: ${d}`)
.style("left", `${event.pageX + 10}px`)
.style("top", `${event.pageY - 20}px`);
})
.on("mouseout", () => tooltip.style("display", "none"));
Now, when users hover over each bar, the tooltip will display the exact data value.
Animating Transitions
Transitions can make your visualizations more engaging. D3 provides smooth animations for things like bar height changes, colors, and other attributes.
Step 1: Adding a Transition to Bar Height
To animate the bar height, you can modify the bar drawing code to include a transition:
svg.selectAll("rect")
.data(data)
.enter()
.append("rect")
.attr("x", (d, i) => xScale(i))
.attr("y", height - margin.bottom)
.attr("width", xScale.bandwidth())
.attr("height", 0) // Start with height 0
.attr("fill", "steelblue")
.transition()
.duration(1000)
.attr("y", d => yScale(d))
.attr("height", d => height - margin.bottom - yScale(d));
Now, when the chart is rendered, the bars will smoothly animate into place.
Updating the Chart with Dynamic Data
To take your visualization further, you can allow for dynamic data updates and transitions. For example, let’s add a button that, when clicked, updates the data and smoothly transitions the bars to reflect the new values.
Step 1: Add a Button to HTML
<button id="updateBtn">Update Data</button>
Step 2: Handle Data Update
You can create a function that generates new random data and updates the chart:
document.getElementById('updateBtn').addEventListener('click', () => {
const newData = Array.from({ length: data.length }, () => Math.floor(Math.random() * 100));
yScale.domain([0, d3.max(newData)]);
svg.selectAll("rect")
.data(newData)
.transition()
.duration(1000)
.attr("y", d => yScale(d))
.attr("height", d => height - margin.bottom - yScale(d));
svg.select(".y-axis")
.transition()
.duration(1000)
.call(yAxis.scale(yScale));
});
Now, when the "Update Data" button is clicked, the chart will smoothly transition to reflect the new dataset.
Conclusion
Building a simple bar chart.
Adding scales and axes for better context.
Enhancing interactivity with tooltips.
Adding smooth transitions for better user experience.
Dynamically updating charts with new data.
D3.js is a powerful tool for creating complex, data-driven visualizations. Whether you're working with small datasets or large-scale data, the customization and interactivity D3.js offers makes it a top choice for web-based visualizations. Start experimenting with D3 and unlock the potential of your data!
Happy coding! 🎨📊
Subscribe to my newsletter
Read articles from ByteScrum Technologies directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
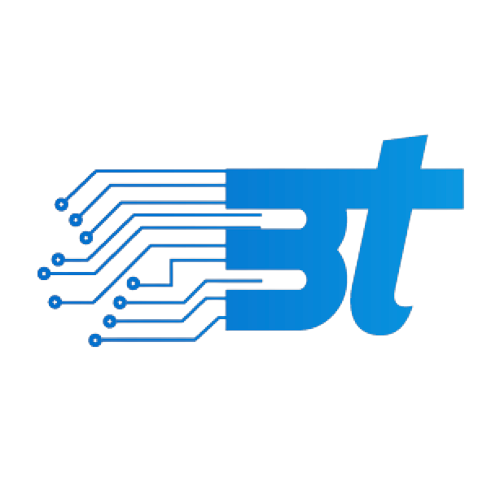
ByteScrum Technologies
ByteScrum Technologies
Our company comprises seasoned professionals, each an expert in their field. Customer satisfaction is our top priority, exceeding clients' needs. We ensure competitive pricing and quality in web and mobile development without compromise.