Mastering React State: The Key to Dynamic and Interactive UIs
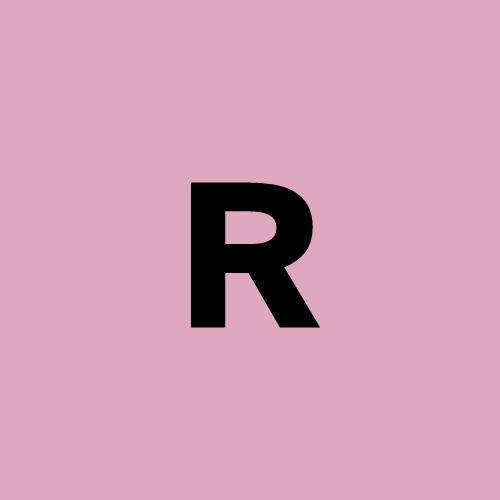
Have you ever wondered, "How does React keep track of all the changes happening on my page?" The answer lies in a powerful feature called state. Don’t worry if the term sounds a bit abstract—by the end of this article, you’ll understand how state works in React and how to use it effectively in your own projects.
We’ll break down what state is, why it's so important in React, and how you can manage it within your components. We’ll also provide some easy-to-follow examples to illustrate how it all works. Whether you’re new to React or looking to deepen your understanding, mastering state is essential to building dynamic, interactive user interfaces (UI). Let’s dive in!
What is State in React?
Imagine wearing a mood ring that changes color based on how you’re feeling. The mood ring's color represents your current state, and every time your emotions change, the ring updates to reflect that. React’s state works in much the same way. It represents the current "mood" or status of your component, and as users interact with your app, the state changes, causing the UI (user interface) to update automatically, just like the mood ring changes color.
In React, state is like a special memory box inside a component that holds information that can change over time. For example, if you’re building a to-do list app, the list of tasks is stored in the component’s state. When the user adds or removes tasks, the state changes, and React updates the screen to show the updated list.
Here’s how you can think of it:
Props: These are like gifts that someone gives to your component. You can use them, but you don’t control them.
State: This is your mood ring—it reflects what’s happening in the component right now, and it can change based on what users do.
Why is State Important?
State is the beating heart of your React app. Without it, your app would be like a static picture—it would never change, no matter what users do. But with state, your app can react (pun intended!) to user interactions, like clicking buttons, filling out forms, or scrolling through content.
State allows your app to keep track of things like:
The number of items in a shopping cart.
Whether a button has been clicked.
The current value of a text input.
Data retrieved from an external service, like a list of movies or weather updates.
When state changes, React automatically updates the UI to reflect the new data. This process is called re-rendering, and it’s what makes React apps dynamic and responsive. You don’t have to manually change the UI—React handles it for you.
Updating State: A Simple Example
Let’s continue with our mood ring analogy. Imagine you have a button that represents the "mood" of the app. Every time you click it, the mood (state) changes between "Happy" and "Excited." The ring (your UI) will change colors based on the mood (state) of the app.
Here’s how you could build something like that in React using state:
import React, { useState } from 'react'; // Import React and useState
function MoodButton() {
const [mood, setMood] = useState('Happy'); // Declare state with initial value 'Happy'
return (
<div>
<p>Your mood is: {mood}</p> {/* Display current mood */}
{/* Toggle mood between 'Happy' and 'Excited' when button is clicked */}
<button onClick={() => setMood(mood === 'Happy' ? 'Excited' : 'Happy')}>
Change Mood
</button>
</div>
);
}
export default MoodButton; // Export the component
In this example:
We use the
useState
hook to create a state variable calledmood
, with an initial value of'Happy'
.The
setMood
function updates the mood when the button is clicked, toggling it between'Happy'
and'Excited'
.Whenever the mood (state) changes, React re-renders the component and updates the displayed mood.
Just like a mood ring changes color, your app’s mood changes based on the state, and React updates the UI to match.
Rules for Using State in React
When working with state in React, there are a few important rules to remember:
State is managed inside the component: Think of each React component as having its own mood ring. Each ring represents only the mood (state) of that specific component. If you want one component to know about another’s mood (state), you’ll need to pass that information through props.
State updates are asynchronous: When you change the state, React doesn’t update it instantly. It’s like putting in an order for pizza—you tell the pizza place what you want, but it takes a little time before the pizza arrives. Similarly, React schedules state updates to keep your app running smoothly.
Never modify state directly: Imagine trying to change the color of a mood ring by painting it yourself—it wouldn’t work! Similarly, in React, you shouldn’t try to change the state directly. Always use functions like
setMood
to update the state.State can store any type of data: State isn’t limited to numbers or strings. It can store arrays, objects, booleans (true/false), or anything else your app needs to track.
State in Class Components vs. Function Components
There are two types of components in React: class components and function components. Today, most React developers prefer using function components, thanks to hooks like useState
that make managing state easier and more intuitive. But you may still see class components in older codebases.
Function Components with Hooks: The modern way to manage state is by using the
useState
hook inside function components, as we saw in the example above.Class Components: In the past, state was managed inside class components. Here’s how the same mood button example would look using a class component:
import React, { useState } from 'react'; // Import React and the useState hook
function MoodButton() {
// Declare a state variable called "mood" with an initial value of 'Happy'
// "setMood" is the function that will update the state
const [mood, setMood] = useState('Happy');
return (
<div>
{/* Display the current mood */}
<p>Your mood is: {mood}</p>
{/* Button to change the mood when clicked.
If mood is 'Happy', change it to 'Excited'. Otherwise, change it back to 'Happy'. */}
<button onClick={() => setMood(mood === 'Happy' ? 'Excited' : 'Happy')}>
Change Mood
</button>
</div>
);
}
export default MoodButton; // Export the component so it can be used in other parts of your app
Class components work fine but are more complex and harder to read than function components with hooks.
To sum up:
State in React is like a mood ring that stores and tracks changes in your component. Just like how the color of a mood ring changes based on your feelings, the state changes based on what’s happening in your app.
When state changes, React automatically updates the UI to reflect the new state.
Function components use the
useState
hook to manage state, while class components usethis.state
andthis.setState
.Always use the setter function (like
setMood
) to update state, never modify it directly.
What’s next?
Now that you’ve learned about state, you’re ready to take your React skills to the next level! In the next article, we’ll dive into props—the way components communicate with each other in React. Just like passing notes between friends, props let components send data back and forth, creating a smooth and connected user experience. Stay tuned, and you’ll soon understand how to make your React components truly work together!
References
React documentation. (n.d.). State and Lifecycle. Retrieved from https://reactjs.org/docs/state-and-lifecycle.html
Mozilla. (n.d.). State in React. MDN Web Docs. Retrieved from https://developer.mozilla.org/en-US/docs/Web/JavaScript
Subscribe to my newsletter
Read articles from Aristil directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
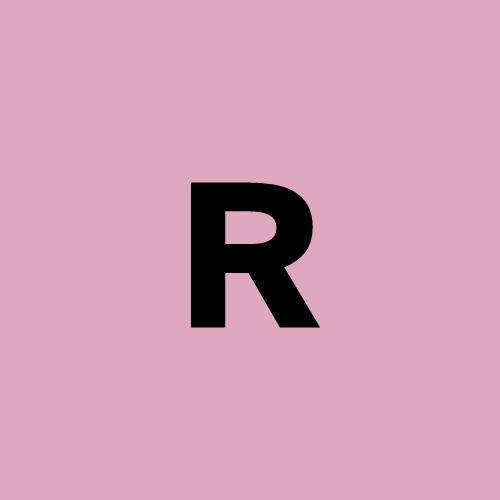