Understanding Shell Scripting: Basics for Beginners
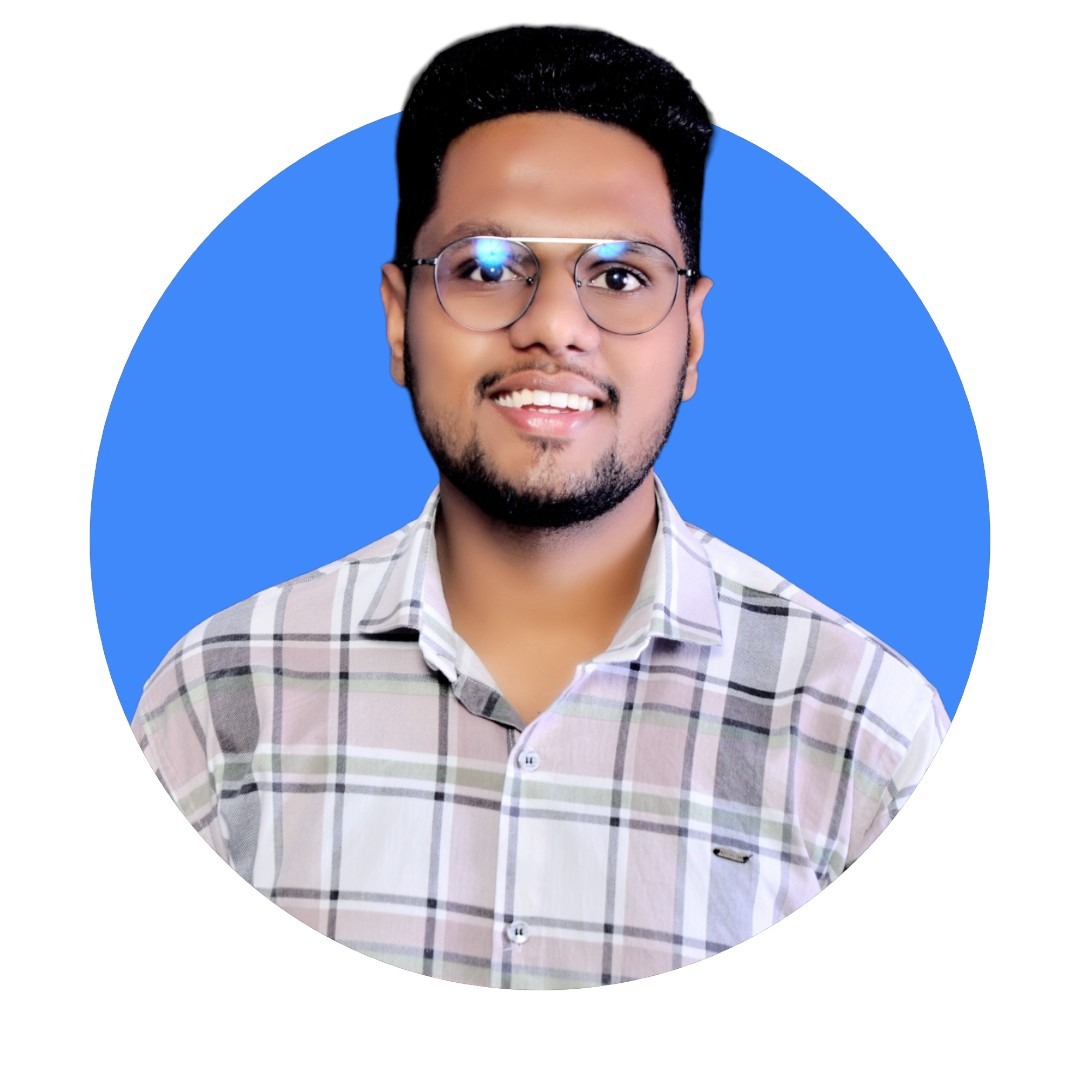
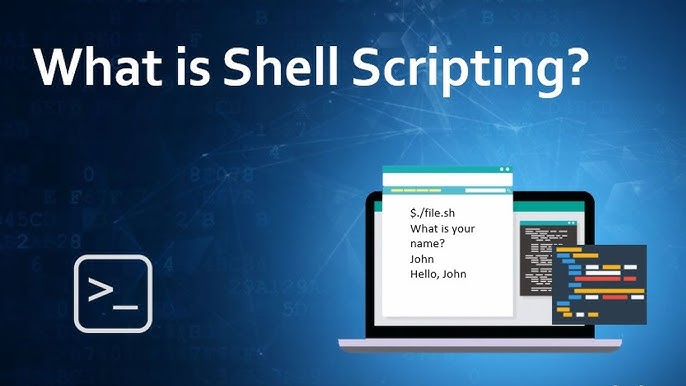
What Is Shell Scripting ?
Usually, shells are interactive, which means they accept commands as input from users and execute them.
However, sometimes we want to execute a bunch of commands routinely, so we have to type in all commands each time in the terminal.
As a shell can also take commands as input from file, we can write these commands in a file and can execute them in shell to avoid this repetitive work.
These files are called Shell Scripts or Shell Programs.
Shell scripts are similar to the batch file in MS-DOS. Each shell script is saved with .sh file extension
e.g., myscript.sh.
A shell script has syntax just like any other programming language.
If you have any prior experience with any programming language like Python, C/C++ etc. It would be very easy to get started with it.
A shell script comprises the following elements –
Shell Keywords – if, else, break etc.
Shell commands – cd, ls, echo, pwd, touch etc.
Functions
Control flow – if..then..else, case and shell loops etc.
Why do we need shell scripts?
There are many reasons to write shell scripts:
To avoid repetitive work and automation
System admins use shell scripting for routine backups.
System monitoring
Adding new functionality to the shell etc.
Some Advantages of shell scripts
The command and syntax are exactly the same as those directly entered in the command line, so programmers do not need to switch to entirely different syntax
Writing shell scripts are much quicker
Quick start
Interactive debugging etc.
Some Disadvantages of shell scripts
Prone to costly errors, a single mistake can change the command which might be harmful.
Slow execution speed
Design flaws within the language syntax or implementation
Not well suited for large and complex task
Provide minimal data structure unlike other scripting languages. etc.
How to Write Bash Scripts?
To write a Bash Script we will follow the steps –
First, we will create a file with the .sh extension.
Next, we will write down the bash scripts within it
After that, we will provide execution permission to it.
To create and write a file with the .sh extension we can use vim text editor. The command for it will be –
vim scriptname.sh
Add the shebang (#!) line.The first line of our script file will be –
#!/bin/bash
This will tell, the system to use Bash for execution. Then we can write our own scripts.
Let’s write down just a simple script that will print some lines in the terminal. The code for it will be –
#!/bin/bash
echo "Hello, Aditya"
Now we will save the file and provide the execution permission to it. To do so use the following command –
chmod +x scriptname.sh
Next to execute the following script we will use the following command –
./scriptname.sh
Basic Shell Commands
echo: Print text or variables to the terminal.
echo "Hello, World!"
read: Get user input.
read name echo "Welcome, $name"
ls
,cd
,pwd
: List files, change directories, print the working directory.touch
,mkdir
,rm
: Create files, create directories, and delete files or directories.
Variables :
We can use variables in bash scripting.
Below is a sample program to understand the usage of variables in Bash scripts.
Example Script:
Name="Aditya Gadhave"
Age=22
echo "The name is $Name and Age is $Age"
Output of Variables:
The name is Aditya Gadhave and Age is 21
So, here is have declared two variables Name and another one is Age.
These variables are accessible using $Name and $Age.
That means, we can declare a variable in a bash script using VariableName=Value and can access it using $VariableName
Special Variables:
$0
: Script name.$1
,$2
, …: Arguments passed to the script.$#
: Number of arguments.$?
: Exit status of the last command.
Decision Making :
In programming, Decision Making is one of the important concepts. The programmer provides one or more conditions for the execution of a block of code. If the conditions are satisfied then those block of codes only gets executed.
Two types of decision-making statements are used within shell scripting. They are –
1. If-else statement:
If else statement is a conditional statement. It can be used to execute two different codes based on whether the given condition is satisfied or not.
There are a couple of varieties present within the if-else statement. They are –
if-fi
if-else-fi
if-elif-else-fi
nested if-else
The syntax for the simplest one will be –
Syntax of If-else statement:
if [ expression ]; then
statements
fi
Example Script:
Name="Satyajit"
if [ "$Name" = "Aditya" ]; then
echo "His name is Aditya. It is true."
fi
Output of if-else statement:
His name is Aditya. It is true.
In the above example, during the condition checking the name matches and the condition becomes true. Hence, the block of code present within the if block gets executed. In case the name doesn’t match then will not have an output. Below is the terminal shell pictorial depiction after executing the following script.
2. case-sac statement:
case-sac is basically working the same as switch statement in programming. Sometimes if we have to check multiple conditions, then it may get complicated using if statements. At those moments we can use a case-sac statement. The syntax will be –
Syntax of case-sac statement:
case $var in
Pattern 1) Statement 1;;
Pattern n) Statement n;;
esac
Example Script:
Name="Aditya"
case "$Name" in
#case 1
"Aditya") echo "Profession : Software Engineer" ;;
#case 2
"Vikas") echo "Profession : Web Developer" ;;
#case 3
"Satyajit") echo "Profession : Technical Content Writer" ;;
esac
Output of case-sac statement:
Profession : Software Engineer
In the above example, the case-sac statement executed the statement which is a part of the matched pattern here the ‘Name’.
Loops in Shell Scripting :
For Loop:
for i in 1 2 3 4 5; do echo "Iteration $i" done
While Loop:
counter=1 while [ $counter -le 5 ]; do echo "Counter: $counter" counter=$((counter+1)) done
Until Loop:
until [ $counter -gt 5 ]; do echo "Counter: $counter" counter=$((counter+1)) done
String and Numeric Comparisons :
The string comparison means in the shell scripting we can take decisions by doing comparisons within strings as well. Here is a descriptive table with all the operators –
Operator | Description |
\== | Returns true if the strings are equal |
!= | Returns true if the strings are not equal |
-n | Returns true if the string to be tested is not null |
-z | Returns true if the string to be tested is null |
Arithmetic operators are used for checking the arithmetic-based conditions. Like less than, greater than, equals to, etc. Here is a descriptive table with all the operators –
Operator | Description |
-eq | Equal |
-ge | Greater Than or Equal |
-gt | Greater Than |
-le | Less Than or Equal |
-lt | Less Than |
-ne | Not Equal |
Below is a simple example of the same –
Example Script:
if [ 10 -eq 10 ];then
echo "Equal"
fi
if [ 'Aditya' == 'Aditya' ];
then
echo "same" #output
else
echo "not same"
fi
Output of String and Numeric Comparisons:
Equal
same
Exit Status:
Every command in a shell script returns an exit status:
0
: Success.Non-zero: Failure.
Check exit status:
if [ $? -eq 0 ]; then echo "Success" else echo "Failure" fi
Functions :
In programming, A function is a block of code that performs some tasks and it can be called multiple times for performing tasks.
Syntax of Functions:
#for defining
function_name(){
commands
.....
}
function_name # for calling
The simplest example of the use of function in Bash scripting can be given as –
Example Script:
#!/bin/bash
#It is a function
myFunction () {
echo Hello World from Aditya Blogs
}
#function call
myFunction
Output of Functions:
Hello World from Aditya Blogs
The above example shows a function that prints something when called.
Conclusion
Shell scripting is a versatile and efficient tool for automating repetitive tasks and managing system operations in Unix-based systems. By mastering shell scripting, you can streamline workflows, automate complex operations, and enhance your productivity. From basic commands and variable handling to advanced control structures like loops, conditionals, and functions, shell scripts provide a flexible environment to manipulate files, process text, and execute system commands.
Subscribe to my newsletter
Read articles from Aditya Gadhave directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
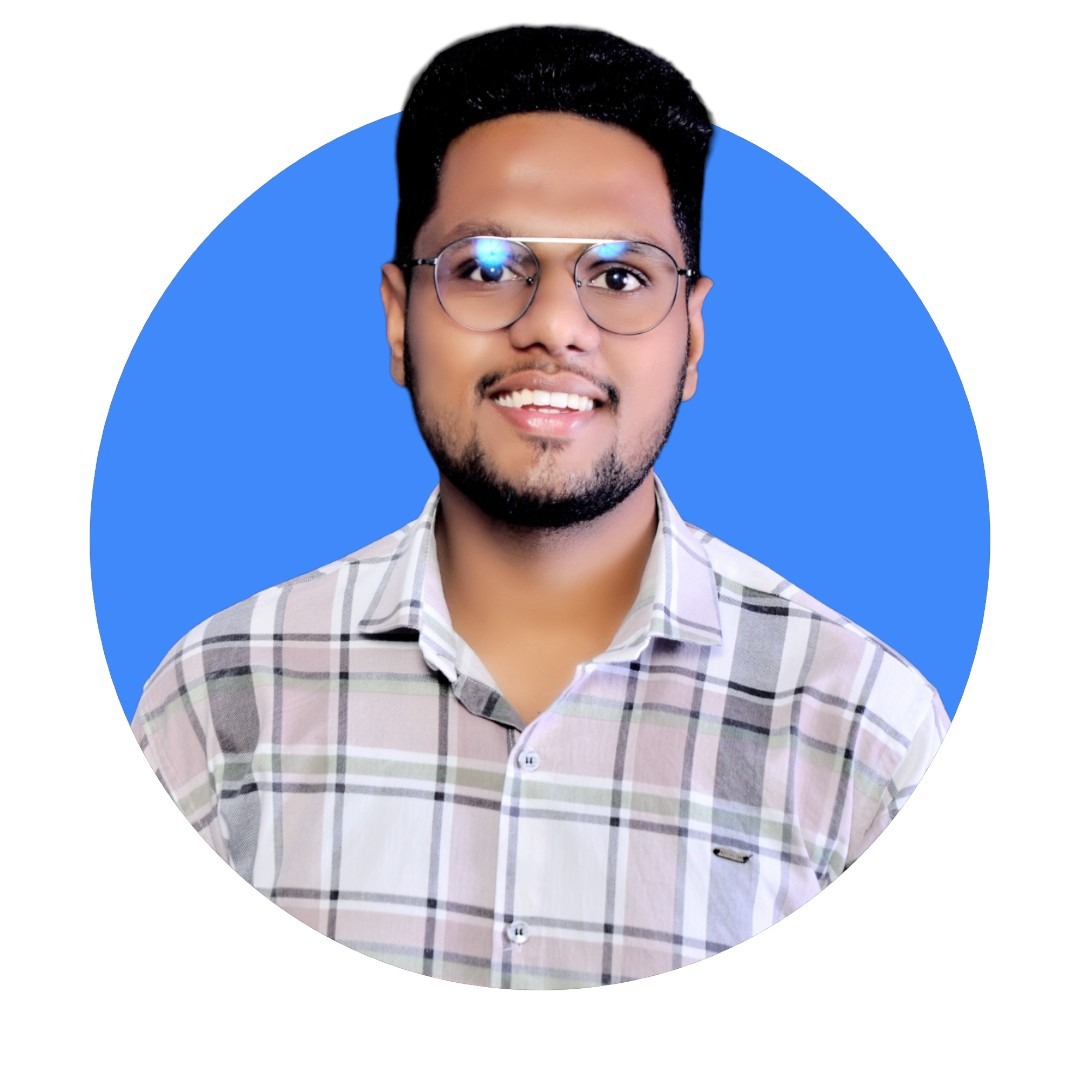
Aditya Gadhave
Aditya Gadhave
👋 Hello! I'm Aditya Gadhave, an enthusiastic Computer Engineering Undergraduate Student. My passion for technology has led me on an exciting journey where I'm honing my skills and making meaningful contributions.