π§ͺTesting Library π¦ - JEST
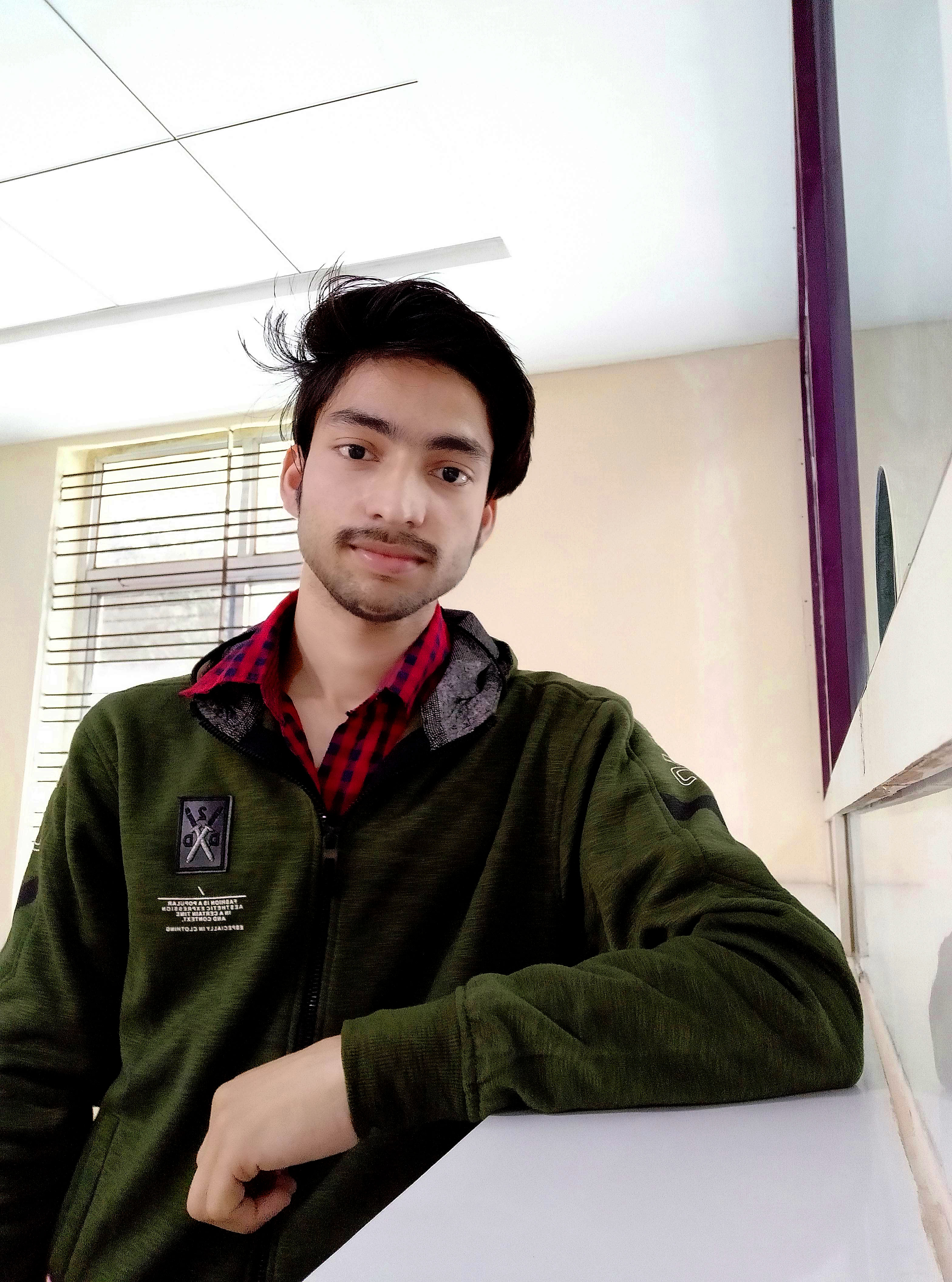
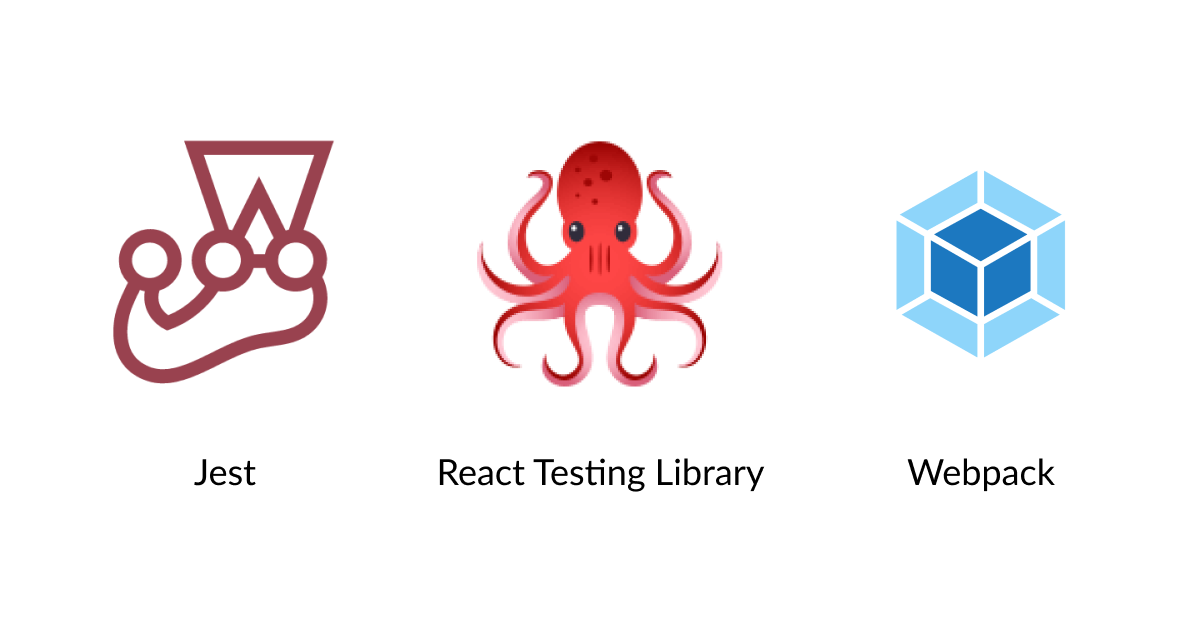
1. Types of testing (developer) :
Unit Testing : Testing One Component in Isolation || means seperately
Integration Testing : Testing Integration of Components
End to End Testing (or) e2e Testing :
End-to-end testing verifies that all components of a system can run under real-world scenarios. The goal of this form of testing is to simulate a user experience from start to finish. E2E testing can find software dependencies while also validating the system under test, its data integrity and integrations.
2.Setting up Testing in our app :
2.1 Install React Testing Library:
npm install --save-dev @testing-library/react @testing-library/dom
2.2 Install Jest
- Jest is a delightful JavaScript Testing Framework with a focus on simplicity.
npm install --save-dev jest
2.3 Additional Configurationβ β Using Babel
- To use Babel, install required dependencies:β
npm install --save-dev babel-jest @babel/core @babel/preset-env
Configure Babel to target your current version of Node by creating a babel.config.js
file in the root of your project:
Manually Create File: π babel.config.js and paste below code
2.4 Configuring Babel
// FileName: πbabel.config.js
module.exports = {
presets: [['@babel/preset-env', { targets: { node: 'current' } }]],
};
3. Only for Parcel js (Optional)
To disable Babel transpilation in Parcel:
Create File: .parcelrc
& paste below code
{
"extends": "@parcel/config-default",
"transformers": {
"*.{js,mjs,jsx,cjs,ts,tsx}": [
"@parcel/transformer-js",
"@parcel/transformer-react-refresh-wrap"
]
}
}
4. Running Tests
- Add a test script in your
package.json
to make running the tests easier:
{
"scripts": {
"test": "jest"
}
}
Now you can run your tests with:
npm test
or npm run test
5. Output
If it shows:
No tests found
It means you have β successfully setup the configuration.
6. JEST Configuration :
npx jest --init
- Select these below option when asked β¬οΈ
- A file will be now created with name: π
jest.config.mjs
7. jest-environment-jsdom
If you're using Jest 28 or later, jest-environment-jsdom package now must be installed separately.
npm install --save-dev jest-environment-jsdom
8. Test πFiles or π Folder Naming Conventions
Note: You can also use .js or .ts
1. Test File Naming Conventions:
.test.js
: Example:Button.test.js
.spec.js
: Example:Button.spec.js
These suffixes help testing tools like Jest automatically recognize the test files.
2. Folder Organization :
A. Colocated Test Files (Alongside Components)
B. Separate
tests
FolderC.
__tests__
Folder βββ
A. Colocated Test Files (Alongside Components)
Placing test files next to the component theyβre testing is a widely used approach for better context:
src/
βββ components/
β βββ Button.js
β βββ Button.test.js
Pros: Easier to navigate between the component and its test.
Cons: Can clutter the folder as the number of tests grows.
B. Separate tests
Folder
For large projects, you may prefer to isolate tests into a separate folder:
src/
βββ components/
β βββ Button.js
tests/
βββ components/
β βββ Button.test.js
Pros: Centralized test files, keeping the codebase tidy.
Cons: Might require switching between directories during development.
C. __tests__
Folder β
Another common option is to create a dedicated __tests__
folder inside relevant directories:
β starting and ending with double underscores β__β.
β Dunder here means βDouble Under (Underscores)β.
src/
βββ components/
β βββ Button.js
β βββ __tests__/
β βββ Button.test.js
3. Integration & E2E Test Naming
If you are writing integration or end-to-end (E2E) tests, differentiate them from unit tests:
Integration Tests:
ComponentName.integration.test.js
E2E Tests:
ComponentName.e2e.test.js
This makes it clear what kind of test is being written.
4. Test Description Naming
In your test cases, use descriptive names for better readability:
describe('Button Component', () => {
test('should render correctly', () => {
// test logic
});
test('should handle click event', () => {
// test logic
});
});
- Use phrases like "should" to clarify the expected behavior of the component.
Subscribe to my newsletter
Read articles from Ayush Kumar directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
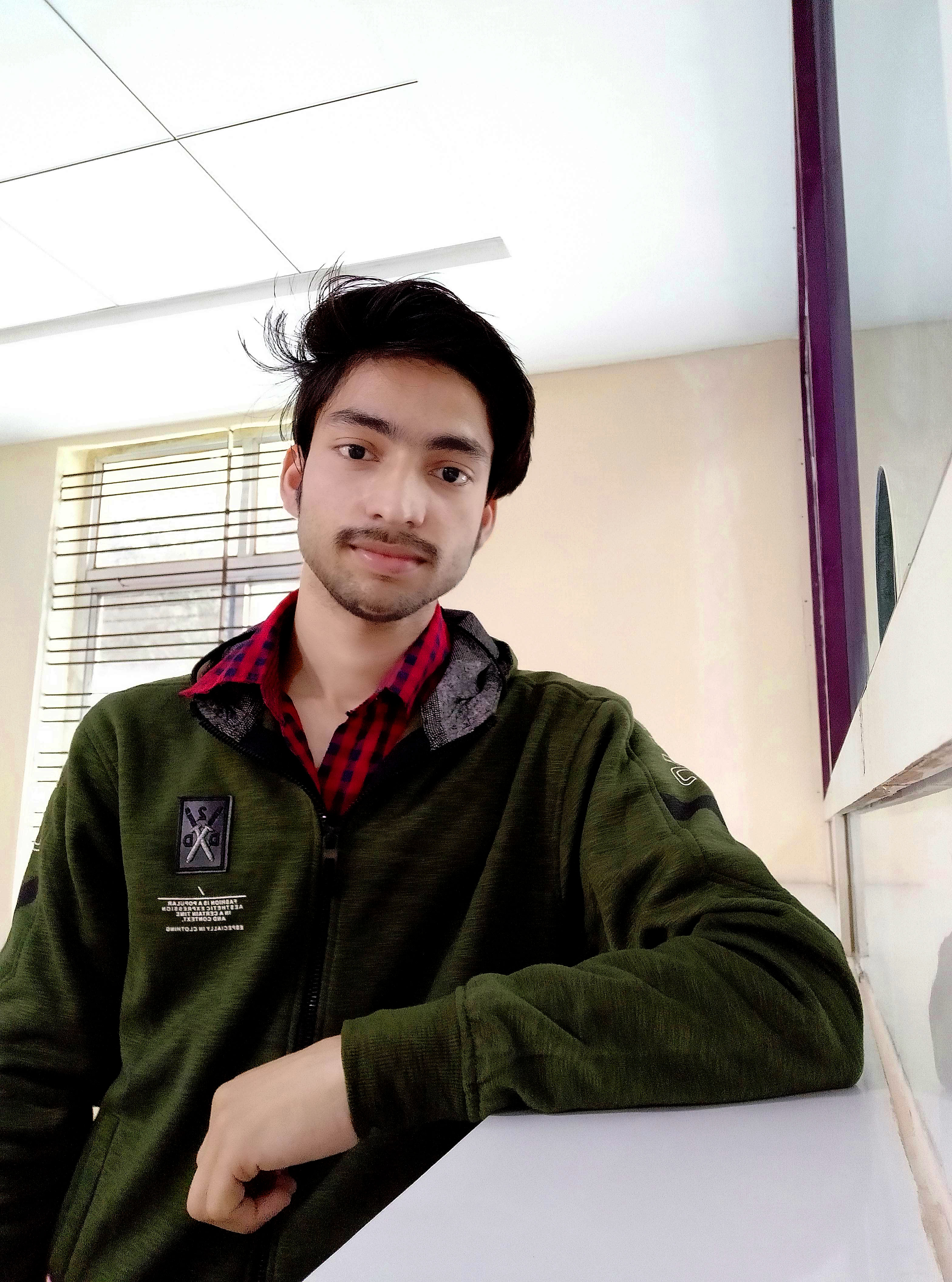
Ayush Kumar
Ayush Kumar
A passionate MERN Stack Developer from India I am a full stack web developer with experience in building responsive websites and applications using JavaScript frameworks like ReactJS, NodeJs, Express etc.,