Demystifying Value Objects in PHP

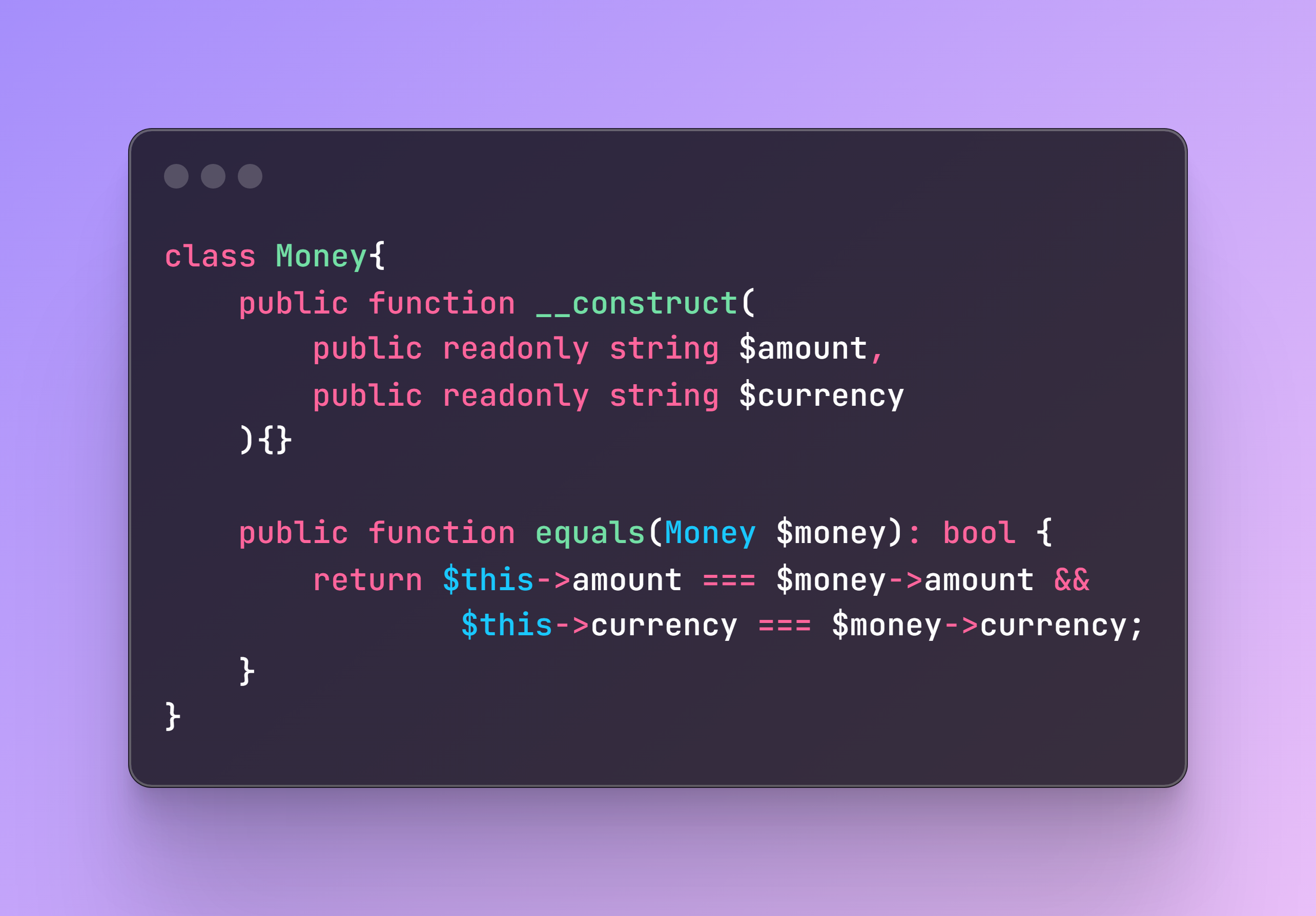
Value Objects are simple PHP classes that allow us to compare the values of an entity that could be in different formats. For example, money could be in multiple currencies, and it cannot be directly compared with just an amount.
Let’s dive right into an example!
class Money{
public function __construct(
public readonly string $amount,
public readonly string $currency
){}
public function equals(Money $money): bool {
return $this->amount === $money->amount &&
$this->currency === $money->currency;
}
}
$tenDollar = new Money(10, 'USD');
$tenRupee = new Money(10, 'INR');
$tenDollar->equals($tenRupee); // false
As you can see value objects are just simple simple classes with a function that computes equality. Here we can specify our custom logic to compare entities.
Other bonus advantages of Value Objects:
Can be used as DTOs to pass data around different services.
Reliable data due to readonly properties of the class which means the data cannot be changed.
Hope this post makes it easier to understand Value Objects in PHP. I’ve kept it brief on purpose to not overcomplicate things.
If you have any questions, I will be happy to answer them in the comments.
Subscribe to my newsletter
Read articles from Mayank Jani directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
