CSS Best Practices for Modern Web Development

Table of contents
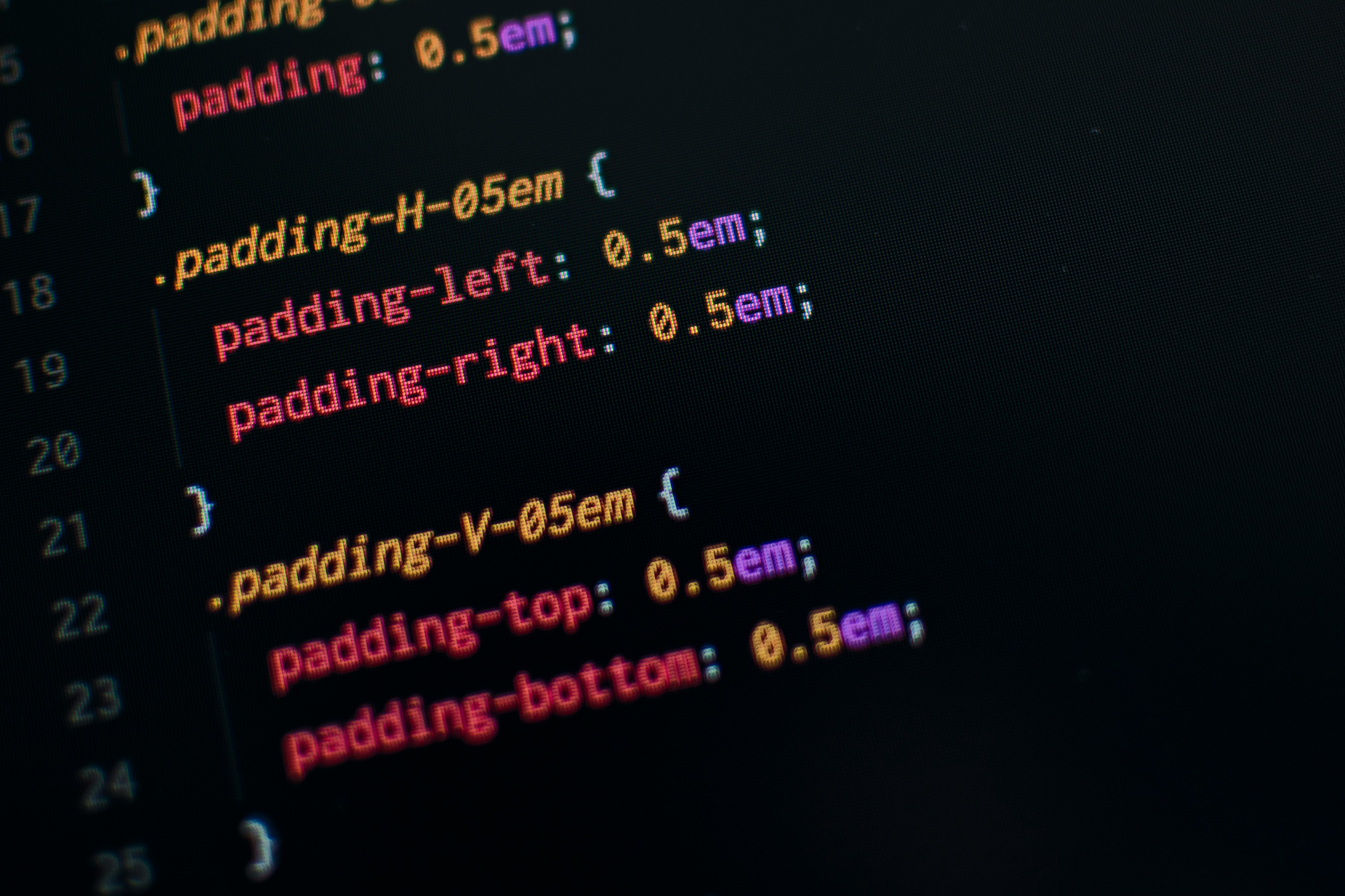
When it comes to web development, mastering CSS (Cascading Style Sheets) is key to creating visually stunning, responsive, and maintainable websites. However, it’s not just about knowing the syntax—following best practices is essential to ensuring your CSS remains clean, scalable, and efficient over time. Whether you’re a seasoned developer or just getting started, these best practices will help you write better CSS for modern web projects.
1. Use a Consistent Naming Convention
One of the most crucial aspects of writing scalable CSS is adopting a consistent naming convention for classes and IDs. The most popular convention today is the BEM (Block, Element, Modifier) methodology. This method ensures that your CSS structure is clear and easy to understand.
Example of BEM:
/* Block: header */
.header { ... }
/* Element: logo inside header */
.header__logo { ... }
/* Modifier: large logo inside header */
.header__logo--large { ... }
By following a system like BEM, you avoid conflicts and make your CSS more predictable, especially in large-scale projects.
2. Minimize the Use of IDs for Styling
Although IDs are powerful in the CSS specificity hierarchy, they can be problematic when trying to keep styles modular and maintainable. Since IDs have higher specificity than classes, overriding them can lead to complicated and difficult-to-maintain code.
Stick to using classes for most of your styling, and reserve IDs for elements that are truly unique and unlikely to be reused elsewhere on the page.
/* Avoid this */
#header { ... }
/* Prefer this */
.header { ... }
3. Leverage CSS Variables
CSS Variables (also known as custom properties) have gained traction for making stylesheets more flexible and easier to maintain. By defining reusable values such as colors, font sizes, and spacing in variables, you can easily update them across your site without having to rewrite your entire CSS.
Example of CSS Variables:
:root {
--primary-color: #3498db;
--secondary-color: #2ecc71;
}
button {
background-color: var(--primary-color);
}
This practice not only reduces redundancy but also allows for easy theme customization.
4. Organize Your CSS by Component
Modular CSS is essential for maintainability. Instead of writing one long stylesheet, break your CSS into logical components or sections. Each file should correspond to a specific part of the UI—like buttons, forms, or headers—making it easier to manage as the project grows.
For example, if you’re working on a large project, it’s common to structure files like this:
/css
/components
_buttons.css
_header.css
_forms.css
/layout
_grid.css
_typography.css
main.css
This component-based approach allows you to scale your CSS more efficiently while keeping your styles clean and organized.
5. Keep Your Specificity Low
High specificity can make CSS difficult to maintain, as overly specific selectors are harder to override without the use of !important. As a best practice, aim to keep your specificity low by avoiding long or overly specific selectors, and avoid using inline styles.
Example:
/* Avoid overly specific selectors */
#main .content .article-title h1 { ... }
/* Use simpler selectors */
.article-title h1 { ... }
Simpler selectors are easier to manage and override, allowing for more flexibility.
6. Use Shorthand Properties Where Appropriate
CSS provides shorthand properties that allow you to set multiple related properties in a single declaration. This not only keeps your styles concise but also reduces file size and improves readability.
Example of Shorthand:
/* Instead of this */
margin-top: 10px;
margin-right: 15px;
margin-bottom: 10px;
margin-left: 15px;
/* Use this */
margin: 10px 15px;
However, be mindful when using shorthand properties, as they will reset all values within that property group. Make sure this behavior is intentional.
7. Optimize for Performance
Although CSS is inherently fast, there are ways to optimize it for even better performance. Some common performance considerations include:
• Avoid inline styles: Inline styles are harder to maintain and can negatively impact performance as they bypass caching mechanisms.
• Minimize reflows: Overuse of layout-triggering properties like width, height, margin, or padding can cause reflows, slowing down rendering.
• Limit the use of @import: Using @import to bring in external stylesheets can increase load times as each import requires a separate HTTP request.
Always aim for clean, minimal, and well-structured CSS to ensure the best performance.
8. Use Preprocessors and Tools
CSS preprocessors like SASS and LESS add powerful features such as nesting, mixins, and inheritance, which are not natively available in CSS. These tools can significantly reduce repetition in your code and make it easier to manage complex styles.
Example of SASS (SCSS syntax):
$primary-color: #3498db;
.button {
background-color: $primary-color;
&:hover {
background-color: darken($primary-color, 10%);
}
}
Additionally, tools like PostCSS and autoprefixer can help ensure your CSS works across all modern browsers by automatically adding vendor prefixes where necessary.
9. Always Consider Accessibility
Good CSS not only makes a site look great but also enhances usability. When designing your styles, consider how they will affect users with disabilities. This includes ensuring sufficient color contrast, using focus states for interactive elements, and designing with screen readers in mind.
Example of focus styles:
button:focus {
outline: 3px solid #3498db;
}
By making accessibility a priority, you create a more inclusive experience for all users.
10. Use CSS Grid and Flexbox for Layouts
When it comes to layout, CSS Grid and Flexbox are the go-to tools for modern web development. Both are incredibly powerful and make it easy to create responsive designs without relying on bulky frameworks like Bootstrap.
Example of Flexbox:
.container {
display: flex;
justify-content: space-between;
}
.item {
flex: 1;
}
Example of CSS Grid:
.grid-container {
display: grid;
grid-template-columns: repeat(3, 1fr);
gap: 20px;
}
Mastering these layout tools will not only make your code cleaner but also ensure your site is adaptable across different screen sizes.
Conclusion
Writing clean, efficient CSS is crucial for maintaining scalable web projects. By following these best practices—such as using a consistent naming convention, organizing your CSS by components, and leveraging modern layout techniques like CSS Grid and Flexbox—you can create stylesheets that are not only easier to manage but also optimized for performance and accessibility.
Whether you’re starting a new project or refactoring an existing one, keeping these principles in mind will help ensure your CSS is robust and future-proof.
Subscribe to my newsletter
Read articles from Daniel Fernández Vega directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
