Learning to Code on a Black Market
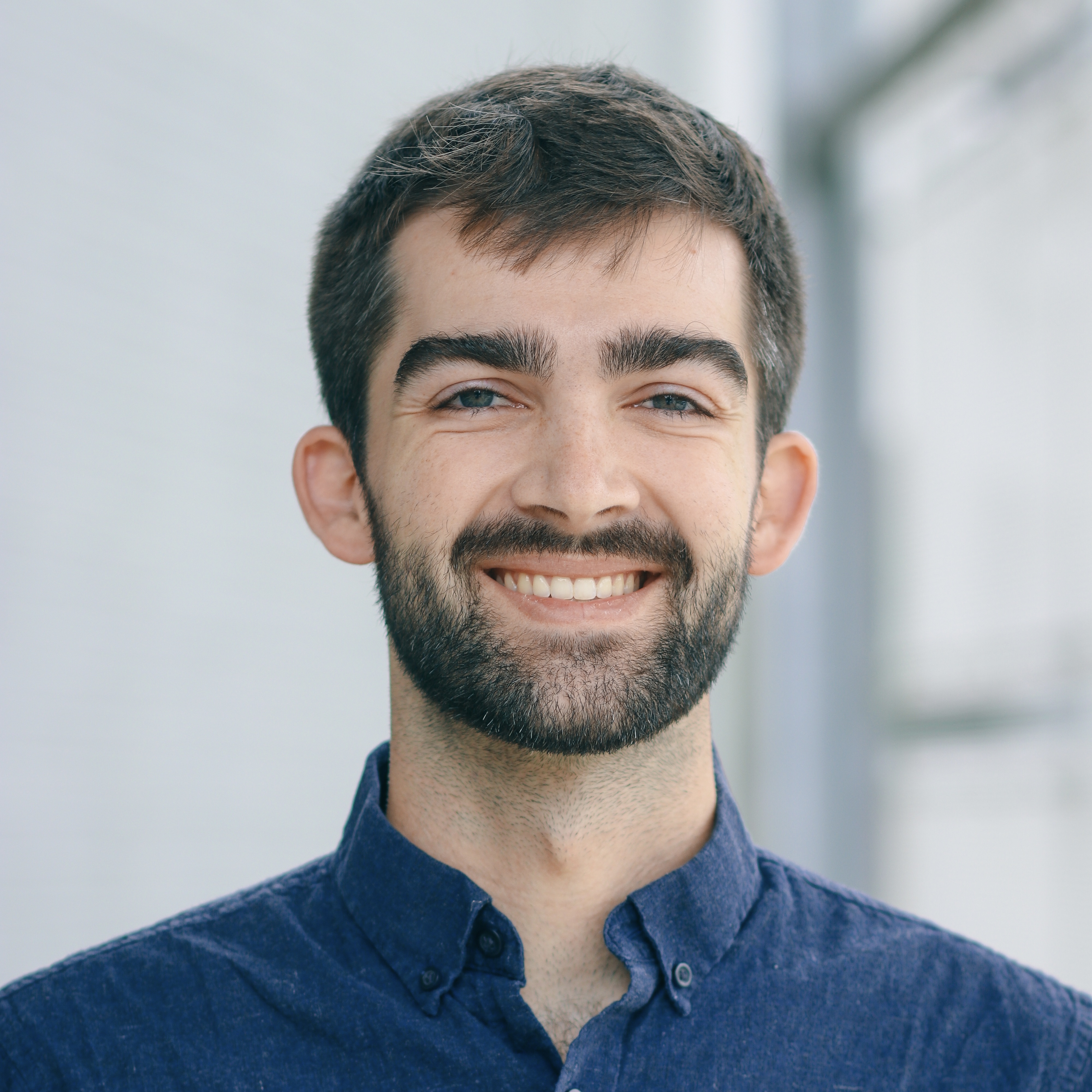
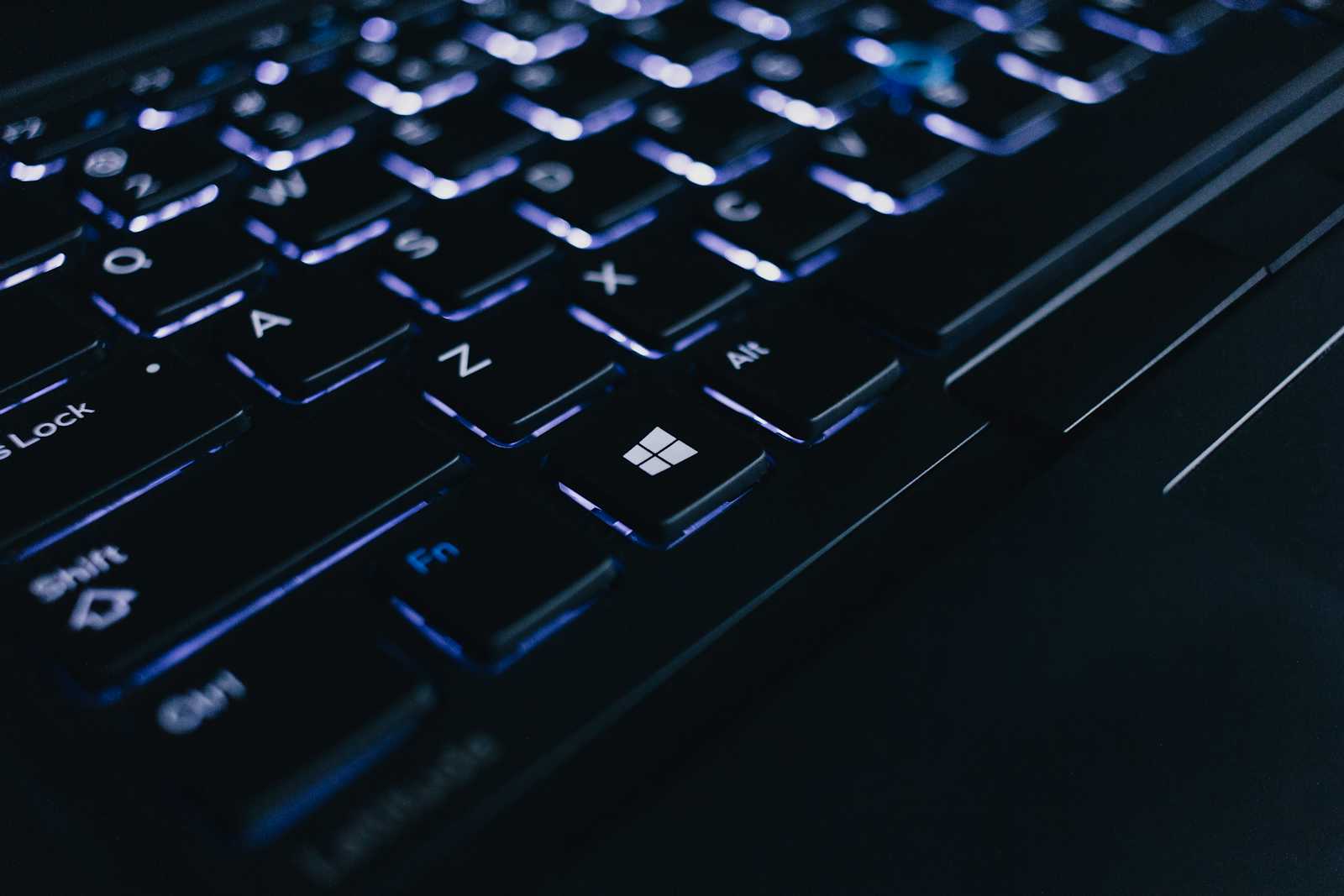
Hello JavaScript
Back in 2016, I started my career as a software consultant for a healthcare tech company. I worked with customers to learn about their needs and then configured their business logic into our product. We used a browser-based interface to enter these settings, and it often involved a LOT of mundane, repetitive work. Think checking hundreds of checkboxes or filling out a form hundreds of times. My muscle memory got pretty good, but my poor fingers could only move so fast. It was a grind to get through it once I had planned out what needed to be done.
During our initial training, we were learning how to use the app and there was a page that required us to enter a default number into many fields. Our instructor told us to create a new browser shortcut and shared a long line of code that started with javascript:
as the URL. Hmm 🤔, that doesn't look like a website... When I clicked the shortcut, it immediately filled out every field with a default value, and I was amazed. I was already excited to start my job, but now I was even more enticed by this sorcery I just witnessed.
I had taken a Matlab coding class in college, but dry engineering homework didn't really hit the same way as seeing manual work disappear in front of my eyes. I had to put my curiosity on the back burner for the first few months so I could get settled learning the product and the industry, but I knew I would be revisiting this magic string of text.
The company was growing rapidly, and lots of new work was coming in. It was an exciting place to be but also very busy. My teammates and I started working longer hours to stay afloat, and we needed a way to implement projects more quickly. There were a few other consultants who knew JavaScript and tinkered with creating new scripts, so I asked them to teach me.
I learned that they were making bookmarklets, or snippets of client-side JavaScript packaged as a browser bookmark. These bookmarklets had access to everything in the browser, and had the power to manipulate page elements however we wanted. My teammates showed me the basics, then on the weekends I studied Eloquent JavaScript with the goal of making my own.
All About Bookmarklets
Web apps store data in a database on a server, but they often need to store some data in the user's browser after the page is loaded so the page has quick access to it. In a modern framework, the client data can be tricky to access from outside the app, but this app was old enough that it used the browser's global scope to hold all the app's client-side variables. This meant all the client-side variables were available directly from the browser console and by extension, bookmarklets. Bingo.
Because bookmarklets only have access to client-side data, we used them to augment our own client-side human actions like filling out input fields, submitting forms, and navigating the app. We weren’t able to edit the backend, APIs, database, etc.
Our bookmarklets all had a similar style, though some were more complex than others. Some common themes were -
We wrapped everything in an IIFE (Immediately Invoked Function Expression), allowing us to define and execute our logic as soon as the bookmarklet was clicked.
We used
prompt()
to collect user input, such as the number to populate in multiple text fields.We implemented basic input validation was to ensure correct data entry, and used
alert()
to notify users of any errors.For more complex DOM element selection, we occasionally relied on JQuery.
Here is an example of a JavaScript snippet that prompts the user for a number, then fills that value into every number input field on the page. It also has some validation to ensure a number is entered.
(function() {
const number = prompt('Enter a number to fill into all number fields:');
if (number !== null) {
if (isNaN(number)) {
alert('Please enter a valid number.');
} else {
const inputs = document.querySelectorAll('input[type="number"]');
inputs.forEach(function(input) {
input.value = number;
});
}
}
})();
During development we would copy and paste our code from a code editor into the browser console to test it out. Once the script was finished, we would minify it to reduce it's size for sharing. Then we’d add javascript:
in front to tell the browser to execute the code rather than redirect to a website, and finally we’d create the browser bookmarklet with the minified script as the URL. The final code would look something like this.
javascript:!function(){
const e=prompt("Enter a number to fill into all number fields:");
null!==e&&(isNaN(e)?alert("Please enter a valid number.")
:document.querySelectorAll('input[type="number"]')
.forEach(function(l){l.value=e}))}();
I was hooked!
This was the perfect playground to learn how to code. The most important part was that I was naturally incentivized to build useful tools so that my teammates and I could finish our work faster and go home on time. We built scripts to -
Fill in many input fields
Check many boxes/radio buttons
Submit forms
Import/export data
Filter items on the page
Navigate to other pages
and much more
Eventually we created custom keyboard shortcuts with a special Chrome extension to increase our efficiency even more. All of this made learning how to code absolutely addictive because I could quickly whip something together and save my team of many hours of menial labor.
I don't think I've ever felt more powerful than the moment my first script executed properly. Now I could make our product do (almost) whatever I needed it to do, without anyone else's approval. I've been chasing that dragon ever since. 🐉
Turmoil Brews
More and more consultants became proficient in JavaScript and created scripts for their own use cases. We started to share these with each other and sometimes jokingly bartered them on a "black market" in return for favors around the office like covering the support phone or the kitchen cleanup shift. The black market provided yet another source of motivation to continue developing new scripts.
The company was aware of a few approved scripts like the ones we received in our training, but they were not onboard with all of these new ones popping up every week. They were concerned for a few reasons -
Having the ability to solve our own problems was great for the short term, but it concealed necessary product enhancements from our development team so they were not able to implement these features into the product.
Critical project timelines were relying on hobbyist code.
There was always a risk of a consultant sharing this code with a tech-savvy customer who could later demand support for these unsanctioned features. This would have been the cardinal sin, and never happened as far as I'm aware.
We knew their concerns, but the scripts made us so much more efficient and our project timelines were now relying on them. It would have been impossible to hit our deadlines without them.
Where Are They Now?
Eventually the company documented all of these scripts and prioritized building them into the product as first-class features. The app has since been fully rebuilt with modern tech and no longer puts everything into global variables in the browser, so the scripts aren't effective anymore. These scripts faded into the distance, relics of the past. Old-timers now talk of the good old days when you could imagine a feature, build it, and start using it that day.
I ended up with a decent understanding of JavaScript, but absolutely no other coding skills. As an activity during COVID, I tried freelancing with Google Apps Script, which is essentially just JavaScript with Google functionality added for managing G-Suite apps. It started off fine but quickly escalated in complexity, and I realized how in-over-my-head I was and had to quit. I had fallen off of the proverbial "Mount Stupid" of the Dunning-Kruger effect curve, headfirst into the "Valley of Despair".
It was a terrible feeling at first, and I felt disgusted when looking at code - like it was mocking me, and that I'd never be able to understand it. I took about a year off from coding before remembering how much I loved it in the first place, got started on a new project, and never stopped again.
My company required a Bachelors degree in computer science or equivalent experience to join their development team. Another degree would have taken me three years to complete while working, not to mention the cost. By this point I knew for sure I wanted to become a programmer and couldn't imagine waiting that long to make it a reality. At the time of writing this article, I’d still be working on that degree.
I tried learning on my own in the evenings but I wasn't sure where to focus. I needed outside help to cross the Valley of Despair, and found Carolina Code School, a full-time in-person bootcamp near me. After mulling it over for months, I took a leap of faith and quit my job to pursue a new career in software development. I completed the bootcamp and was fortunate to find a job before the post-COVID tech layoffs. This was the biggest risk I've taken in my life, but it felt like a bigger risk to do nothing.
Subscribe to my newsletter
Read articles from Will Braun directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
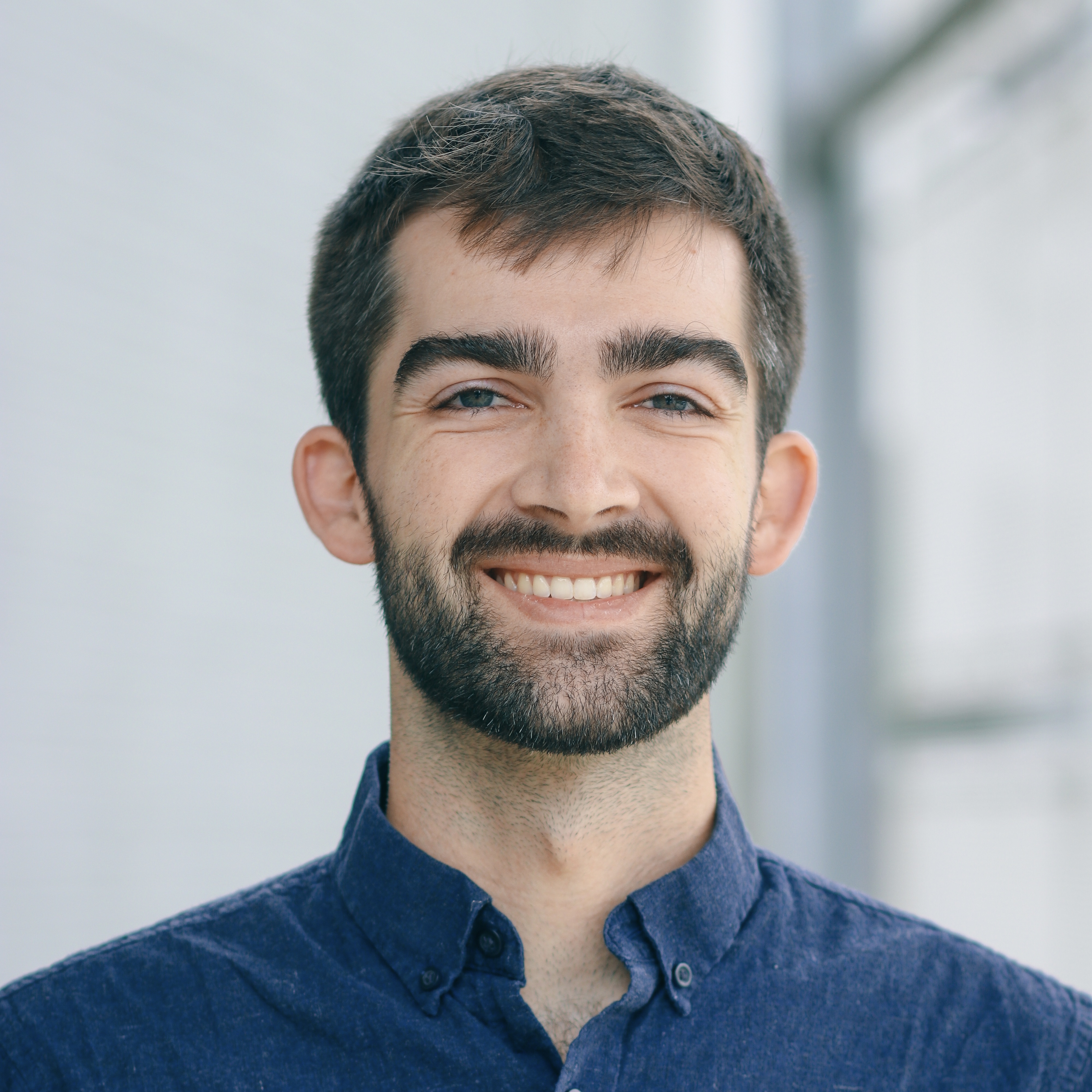
Will Braun
Will Braun
I am a developer currently living in Greenville, SC, USA. When I'm not coding, or writing about coding, I enjoy playing tennis and chess competitively.