Laravel Directory Structure

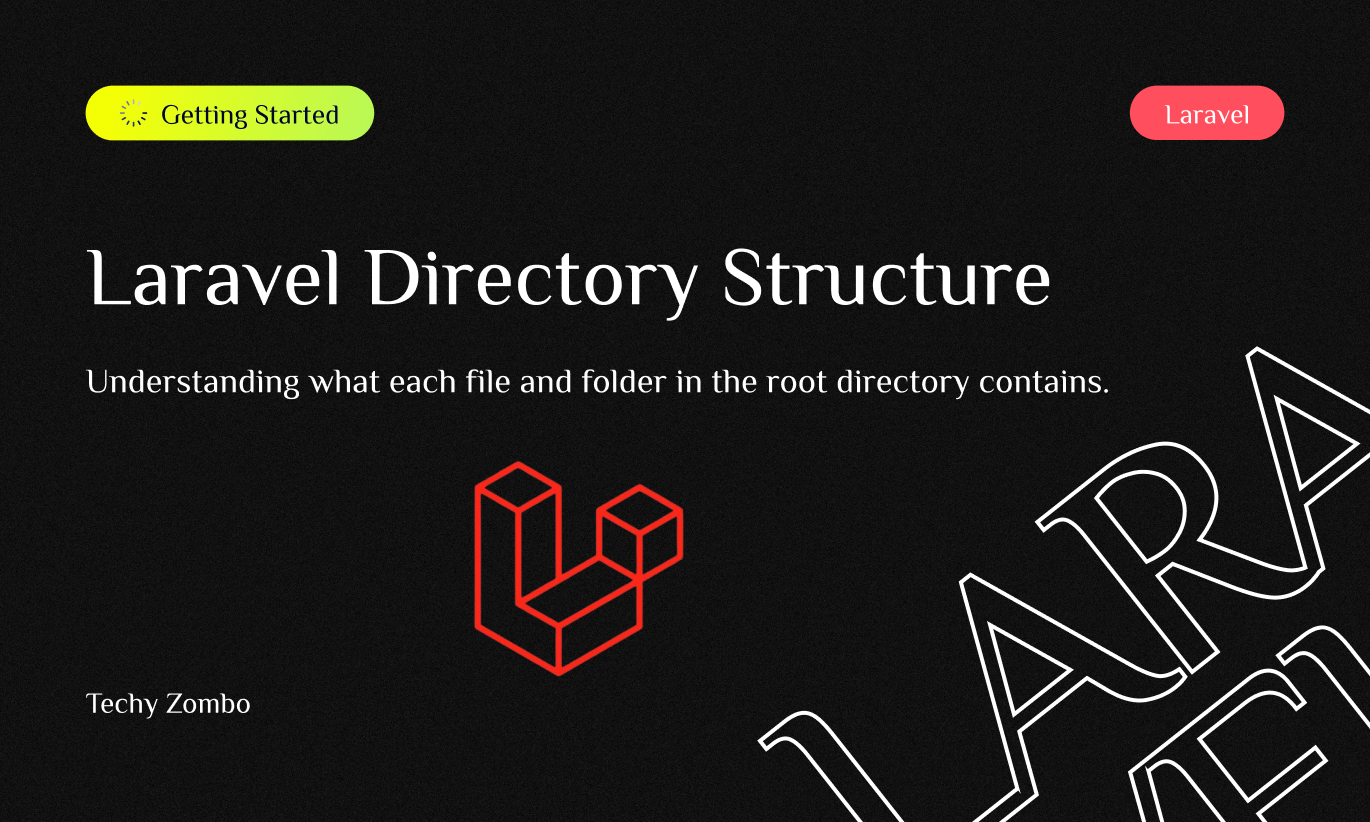
Hello 🌸
Nice to see you again. Today we are demystifying Laravel’s directory structure, which is a lot to take in for beginners. We'll be using the journal-app
we created in the last article. Let’s start by opening our Laravel app in VS Code or any IDE of your choice.
Take Off 🚀
When you open the journal-app
in VS Code, you will see a directory structure like this:
Let’s have a quick overview of these files and folders.
Directory Overview 📂
app Directory: This contains the core code of your application. This is where the major part of the backend logic specific to your application will go. Thus it is the home of your middlewares, controllers, models, and more. More folders and files get added to this directory as your app grows.
bootstrap Directory: This directory contains the file named
app.php
that bootstraps your Laravel application. This means theapp.php
file sets the Laravel framework and gets it ready for you to build your application. This directory also contains thecache
directory that stores or caches files generated by the framework. As the Laravel documentation puts it, “You should not typically need to modify any files within this directory.”config Directory: As the name suggests this is where the configurations for your app live. All the files in this directory are configuration files and the name of each file tells you what part of your application it configures. For example, the
app.php
file contains configuration for your application such as your application name, URL, environment, etc; theauth.php
file contains configuration for the authentication process of your application, it specifies how users are authenticated, which guards and providers are used, etc.database Directory: This contains your database migrations, seeders, and factories. Now this database directory can also be used to store your SQLite database.
If you are new to migrations, seeders, and factories, you can think of them (for now) as bunches of code that we use to set up and fill our database with data. The set of code that we use to build the structure of our database is called a migration. A factory is a set of code that creates or manufactures fake data for the tables in your database. A seeder is a set of code that controls multiple factories. Each set of code is organized into files and these files are sorted into directories. The migrations directory contains your migration files, the seeders directory contains your seeder files, and so on.public Directory: It serves as the document root for your web server. This means it is the directory that is publicly accessible over the web, and it hosts all the assets and entry points that your application needs to serve to clients (like web browsers). It contains the
index.php
which is the entry point of your application for all incoming requests. This file is the first file that gets run when the Laravel application starts. This file also configures your Laravel application to automatically include class files when the classes are needed, without using require or any import statement, this is called autoloading. The public directory also hosts static files like your Javascript, CSS, and images. It also contains miscellaneous public files such as the robots.txt.resources Directory: This directory is a crucial part of the Laravel framework’s structure. It serves as a central location for all the front-end files, views, and assets that your application uses. It contains raw, un-compiled assets such as your javascript and CSS files. Raw, un-compiled assets are the original source files for your front-end assets that may require processing before they can be served to the browser. Examples: CSS preprocessors like SCSS/SASS (.scss, .sass), LESS (.less), JavaScript Variants like ES6+, TypeScript (.ts), Module Bundlers: JavaScript modules that need to be bundled.
routes Directory: This is where you define all the routes (endpoints) that your application responds to. A route is essentially a mapping between an HTTP request (like a URL the user visits) and a controller action or closure that handles that request. By organizing your routes in this directory, Laravel provides a clean and maintainable way to handle all incoming requests to your application. By default, this directory comes with two files,
web.php
andconsole.php
. Theweb.php
file is used to define routes that handle HTTP requests, and theconsole.php
defines routes that handle requests from the console. Other files will be added such as theapi.php
that contains routes intended for API endpoints.storage Directory: It contains the files generated by your application while it is running. It stores files such as the app’s log files, which have records of events, errors, and other information that can help in debugging and monitoring the application, the app’s compiled blade templates, which are processed versions of your Blade templates that are cached for performance optimization, and more. The
app
directory is used to store files generated by your application that are specific to it, theframework
directory contains files generated by the Laravel framework, and thelog
directory contains your log files.tests Directory: As its name suggests it contains your test files, in other words, it contains the files that have code you’ve written to test your code.
vendor Directory: This is the no-go area directory 😂. This is where your app’s and Laravel’s dependencies are located. So you do not want to touch it.
File Overview 📑
That was a lot for the root directories of a Laravel application. So we move to the root files and this should be quick.
.editorconfig: The .editorconfig file helps maintain consistent coding styles between different editors and IDEs by defining coding style rules. Specifying settings like indentation style, line endings, and character encoding, ensures that multiple developers working on the same project adhere to the same coding standards. Supported editors read this file and automatically apply the specified settings when you open a file from the project. It contains content like this:
root = true [*] indent_style = space indent_size = 4 end_of_line = lf charset = utf-8 trim_trailing_whitespace = true insert_final_newline = true
.env: The .env file stores environment configuration variables for your application. It contains key-value pairs that define settings like database credentials, API keys, and other sensitive information. It should be excluded from version control (e.g., Git) because it contains sensitive data. It contains content like this:
APP_NAME=Laravel APP_ENV=local APP_KEY=base64:YourAppKeyHere APP_DEBUG=true APP_URL=http://localhost DB_CONNECTION=mysql DB_HOST=127.0.0.1 DB_PORT=3306 DB_DATABASE=your_database DB_USERNAME=your_username DB_PASSWORD=your_password
Laravel’s
config
files can access these variables using the env() helper function. Using.env
allows you to change configuration settings without altering code..env.example: Serves as a template for the .env file, providing default values and indicating what environment variables are needed. It helps new team members set up their own
.env
files by providing a list of required variables. Unlike.env
, this file is committed to version control so that it’s available to everyone cloning the repository. So when setting up an existing project, that you maybe cloned from Github, you can create your own.env
file by copying and pasting the content from.env.example
file and assigning the appropriate values to the various variables..gitattributes: This file specifies Git attributes for pathnames, which can affect how Git handles line endings and file merging. It ensures consistent line endings (e.g., LF vs. CRLF) across different operating systems. It also helps prevent issues that arise from different default line endings on Windows (CRLF) and Unix/Linux/macOS (LF). You can also use it to define custom merge strategies for specific file types.
.gitignore: This file contains the lise of files and directories that Git should ignore, preventing them from being tracked in version control. In order words it contains a list of files and directories that you do not want to push to Github, such as your
.env
file, cache files, compiled code, and logs.artisan: This file is the Artisan command-line interface (CLI) script for Laravel. It is the script that gives you access to the
artisan
command that you will fall in love with in subsequent articles.artisan
provides a wide range of commands to assist in building Laravel applications.composer.json: This file defines PHP dependencies and other project metadata for Composer, PHP’s dependency manager. It lists the packages required by your application.
composer.lock: The composer.lock file records the exact versions of the dependencies that were installed, locking them to specific versions to ensure consistency across installations.
package.json: Defines JavaScript dependencies and scripts for npm (Node Package Manager). It lists front-end packages required by your application, such as vue, react, or axios and contains scripts for common tasks like compiling assets or running tests.
phpunit.xml: This is the configuration file for PHPUnit, the PHP testing framework. It specifies settings like bootstrap files, test suites, code coverage options, and environment variables for testing. It allows you to override
.env
variables during testing, so that you can run test effectively.README.md: Provides documentation or instructions about the Laravel project. It’s written in Markdown for easy readability on platforms like GitHub.
vite.config.js: Configuration file for Vite, a modern front-end build tool. It defines how front-end assets like JavaScript and CSS are compiled and bundled. You can modify this file to change how assets are processed.
Summary
That was really a lot and I wish I could make it shorter. I skipped some details of some directories and files as that would have made this blog longer. Hopefully I will write seperate articles, independent of this series, to explain each directory and file in great details.
Let’s wrap up with this simple summary.
Dircectory / File Name | Description |
1- app Directory | Contains core application code like middlewares, controllers, and models. |
2- bootstrap Directory | Holds app.php to bootstrap Laravel and a cache directory for framework-generated files. |
3- config Directory | Stores configuration files defining settings for various parts of the application. |
4- database Directory | Contains migrations, seeders, and factories for setting up and populating the database. |
5- public Directory | Web server’s document root which hosts assets and entry points like index.php, and static files. |
6- resources Directory | Central location for front-end files, views, and raw uncompiled assets like JS and CSS. |
7- routes Directory | Defines all application routes mapping HTTP requests to controllers or closures. |
8- storage Directory | Stores generated files like logs, cached views, and other runtime data during application run. |
9- tests Directory | Contains test files written to test your application code. |
10- vendor Directory | Contains application and Laravel dependencies. |
11- .editorconfig File | Defines coding style rules to maintain consistent styles across different editors and IDEs. |
12- .env File | Stores environment variables like database credentials; should be excluded from version control. |
13- .env.example File | Template for .env file, showing required environment variables without sensitive data. |
14- .gitattributes File | Specifies Git attributes affecting line endings and file merging across operating systems. |
15- .gitignore File | Lists files and directories to exclude from version control, like .env , cache, and logs. |
16- artisan File | Laravel’s command-line interface script providing various development commands. |
17- composer.json File | Defines PHP dependencies and project metadata for Composer, PHP’s dependency manager. |
18- composer.lock File | Locks dependencies to specific versions for consistent installations across environments. |
19- package.json File | Defines JavaScript dependencies and scripts for npm, the Node Package Manager. |
20- phpunit.xml File | Configuration file for PHPUnit, specifying test settings such as environment variables for testing. |
21- README.md File | Contains project documentation or instructions, written in Markdown format. |
22- vite.config.js File | Configures Vite for compiling and bundling front-end assets like JavaScript and CSS. |
Thank You 💐
Thank you so much for making it this far. I hope you learnt something new or meaningful. See you in the next articles where we will be dicussing the Laravel MVC Architecture 🔥
Oh and please don’t forget to like this article 😁
Subscribe to my newsletter
Read articles from M'mah Zombo directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

M'mah Zombo
M'mah Zombo
I am a born-again Christian and a software engineer at Korlie Limited. I'm an ALX graduate and I'm studying software engineering at Limkokwing University. I like chess ❤️