A Beginner's Guide to Rust: Understanding Functions, Control Flow, and Error Management
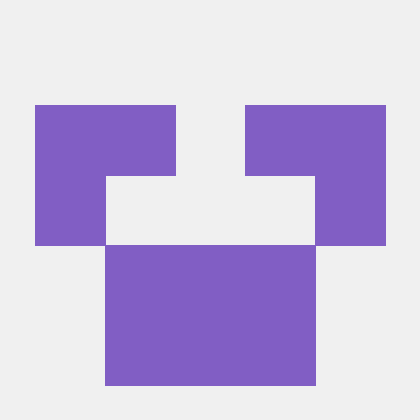
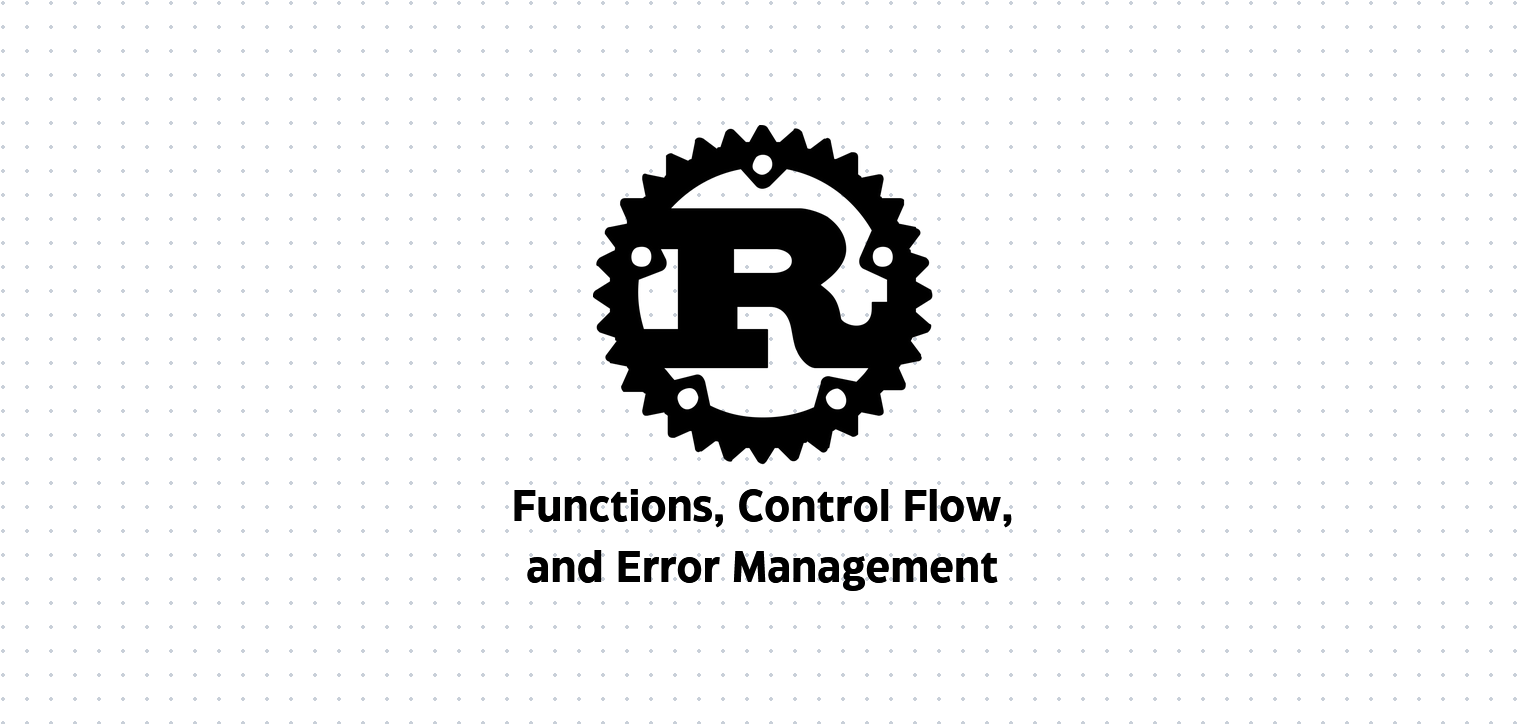
Rust is a modern programming language that emphasizes safety, concurrency, and performance. In this blog post, we'll explore three fundamental aspects of Rust: functions, control flow and error handling. These concepts are crucial for writing efficient and safe code in Rust.
Functions in Rust
Function Syntax and Signatures
In Rust, functions are declared using the fn
keyword. Here's a basic function declaration:
fn greet(name: &str) -> String {
format!("Hello, {}!", name)
}
Let's break this down:
fn
is the keyword used to declare a functiongreet
is the function name(name: &str)
defines the parameter list:name
is the parameter name, and&str
is its type (a string slice)-> String
specifies the return type
Argument Passing and Return Types
Rust is strict about types. You must specify the type of each argument and the return type (if any). If a function doesn't return a value, you can omit the return type or use -> ()
:
fn print_number(x: i32) {
println!("The number is: {}", x);
}
Rust supports multiple return values using tuples:
fn calculate_stats(numbers: &[i32]) -> (i32, i32) {
let sum: i32 = numbers.iter().sum();
let count = numbers.len() as i32;
(sum, count)
}
Expression-based Functions
In Rust, the last expression in a function is implicitly returned. This allows for concise function bodies:
fn add_one(x: i32) -> i32 {
x + 1 // No semicolon here; this is the return value
}
Control Flow in Rust
If Expressions
Rust's if
is an expression, which means it can return a value:
let number = 5;
let description = if number % 2 == 0 {
"even"
} else {
"odd"
};
println!("The number is {}", description);
In this example:
We use the modulo operator
%
to check ifnumber
is divisible by 2.The
if
expression evaluates to either "even" or "odd".The result is stored in
description
.Unlike many other languages, we don't need a ternary operator in Rust because
if
can be used as an expression.
Match Expressions
Rust's match
is a powerful control flow operator:
let number = 13;
match number {
1 => println!("One!"),
2 | 3 | 5 | 7 | 11 => println!("This is a prime"),
13..=19 => println!("A teen"),
_ => println!("Ain't special"),
}
Let's break this down:
The
match
expression comparesnumber
against each pattern in order.1 => ...
is a single value match.2 | 3 | 5 | 7 | 11 => ...
uses the|
operator to match multiple values.13..=19 => ...
is a range match, including both 13 and 19._ => ...
is a catch-all pattern that matches any value not matched by the previous patterns.
match
expressions must be exhaustive, meaning they must cover all possible values of the matched type.
Loops
Rust provides several loop constructs:
// Infinite loop
loop {
println!("Forever!");
break; // Exit the loop
}
// While loop
let mut count = 0;
while count < 5 {
println!("Count: {}", count);
count += 1;
}
// For loop
for number in 1..=5 {
println!("Number: {}", number);
}
Explanation:
The
loop
keyword creates an infinite loop. Usebreak
to exit the loop.while
loops continue as long as the condition is true.for
loops iterate over a range or any type that implements theIntoIterator
trait.1..=5
is a range that includes both 1 and 5.
Pattern Matching
Pattern matching in Rust goes beyond simple value matching. You can destructure structs, enums, and tuples:
struct Point {
x: i32,
y: i32,
}
let point = Point { x: 0, y: 7 };
match point {
Point { x, y: 0 } => println!("On the x-axis at {}", x),
Point { x: 0, y } => println!("On the y-axis at {}", y),
Point { x, y } => println!("On neither axis: ({}, {})", x, y),
}
This example demonstrates:
Destructuring a
struct
in amatch
expression.Matching specific values (
y: 0
andx: 0
).Using variable binding to capture values (
x
andy
).The last arm acts as a catch-all, binding both
x
andy
.
Error Handling: Result and Option Types
Rust's approach to error handling and dealing with nullable values is centered around two crucial enum types: Result
and Option
. These types are fundamental to Rust's philosophy of making errors and edge cases explicit, contributing to the language's focus on reliability and safety.
The Result Enum
The Result
enum is Rust's primary mechanism for handling operations that can fail. It's defined in the standard library as follows:
enum Result<T, E> {
Ok(T),
Err(E),
}
Here, T
represents the type of the value returned in the success case, and E
represents the type of the error returned in the failure case.
Theory and Usage
Explicit Error Handling: By using
Result
, Rust forces developers to explicitly consider and handle both success and failure cases. This helps prevent bugs caused by unhandled errors.Type Safety: The error type
E
is part of theResult
's type signature, allowing for type-specific error handling and preventing errors from being silently ignored or misinterpreted.Composition:
Result
types can be easily combined and chained using combinators likeand_then
,or_else
, andmap
, allowing for expressive and concise error handling.The
?
Operator: Rust provides the?
operator as syntactic sugar for propagating errors. It's equivalent to a match expression that returns early in case of an error.
Example of composing Result
operations:
use std::fs::File;
use std::io::{self, Read};
fn read_username_from_file() -> Result<String, io::Error> {
let mut username = String::new();
File::open("username.txt")?.read_to_string(&mut username)?;
Ok(username)
}
In this example, the ?
operator is used twice. If either File::open
or read_to_string
returns an Err
, that error will be immediately returned from the function.
Common Methods on Result
is_ok()
andis_err()
: Check if the Result is Ok or Err without consuming the value.unwrap()
: Returns the value if Ok, panics if Err (use with caution).expect(msg)
: Like unwrap, but with a custom panic message.unwrap_or(default)
: Returns the value if Ok, or a default value if Err.unwrap_or_else(f)
: Returns the value if Ok, or the result of calling f if Err.
The Option Enum
The Option
enum represents the presence or absence of a value. It's defined as:
enum Option<T> {
Some(T),
None,
}
Where T
is the type of the contained value when it's present.
Theory and Usage
Null Safety:
Option
eliminates null pointer exceptions by forcing explicit handling of the absence of a value.Expressiveness: It makes the intent clear in function signatures and data structures when a value might not be present.
Composition: Like
Result
,Option
can be composed using methods likemap
,and_then
, andor_else
.Pattern Matching:
Option
works seamlessly with Rust's pattern matching, allowing for concise and readable code.
Example of working with Option
:
fn find_user(id: u32) -> Option<String> {
// Simulating a database lookup
match id {
1 => Some(String::from("Alice")),
2 => Some(String::from("Bob")),
_ => None,
}
}
fn greet_user(id: u32) {
match find_user(id) {
Some(name) => println!("Hello, {}!", name),
None => println!("User not found"),
}
}
greet_user(1); // Outputs: Hello, Alice!
greet_user(3); // Outputs: User not found
Common Methods on Option
is_some()
andis_none()
: Check if the Option contains a value or is None.unwrap()
: Returns the value if Some, panics if None (use cautiously).expect(msg)
: Like unwrap, but with a custom panic message.unwrap_or(default)
: Returns the value if Some, or a default value if None.unwrap_or_else(f)
: Returns the value if Some, or the result of calling f if None.
Combining Result and Option
Rust's standard library provides methods to convert between Result
and Option
types:
ok()
: Converts aResult<T, E>
to anOption<T>
, discarding the error information.ok_or(err)
: Converts anOption<T>
to aResult<T, E>
, providing a default error value.transpose()
: Transposes aResult
of anOption
into anOption
of aResult
.
Example of converting between Result
and Option
:
fn divide(numerator: f64, denominator: f64) -> Option<f64> {
if denominator == 0.0 {
None
} else {
Some(numerator / denominator)
}
}
fn safe_divide(numerator: f64, denominator: f64) -> Result<f64, String> {
divide(numerator, denominator).ok_or("Division by zero".to_string())
}
println!("{:?}", safe_divide(10.0, 2.0)); // Ok(5.0)
println!("{:?}", safe_divide(1.0, 0.0)); // Err("Division by zero")
Best Practices
Use
Result
for operations that can fail due to reasons other than invalid/missing data.Use
Option
when a value may or may not exist, but its absence is not an error condition.Prefer using
match
or combinators overunwrap()
to handle both cases explicitly.Use the
?
operator to simplify error propagation in functions returningResult
.Consider creating custom error types for more expressive error handling in larger applications.
By leveraging Result
and Option
, Rust encourages a programming style that's both expressive and robust, helping developers write code that's easier to reason about and less prone to runtime errors.
Comparing Rust with C++ and JavaScript
Now that we've explored Rust's functions and control flow, let's compare these concepts with their counterparts in C++ and JavaScript:
Function Declarations:
Rust: Explicit type annotations for parameters and return values.
C++: Similar to Rust, requires type declarations.
JavaScript: No type annotations required, offers more flexibility but less compile-time safety.
Return Values:
Rust: Implicit returns for the last expression in a function.
C++: Explicit
return
statements required.JavaScript: Can use implicit returns with arrow functions, otherwise requires
return
.
Error Handling:
Rust: Uses
Result
andOption
types for explicit error handling.C++: Typically uses exceptions or error codes.
JavaScript: Uses exceptions and recently introduced optional chaining for null safety.
Pattern Matching:
Rust: Powerful
match
expression for complex pattern matching.C++: Limited to switch statements, enhanced in C++17 with if constexpr.
JavaScript: Basic switch statements and destructuring assignments.
Null Safety:
Rust:
Option<T>
type prevents null pointer exceptions.C++: Raw pointers can be null, introduced std::optional in C++17.
JavaScript: Has null and undefined, which can lead to runtime errors.
Type System:
Rust: Strong, static typing with type inference.
C++: Strong, static typing with some type inference (since C++11).
JavaScript: Dynamic typing with optional type annotations (using TypeScript).
Memory Management:
Rust: Ownership system with compile-time checks, no garbage collection.
C++: Manual memory management, RAII (Resource Acquisition Is Initialization).
JavaScript: Automatic garbage collection.
Conclusion
Rust's approach to functions, control flow, and error handling emphasizes safety, expressiveness, and reliability. While it may initially seem more verbose than languages like JavaScript, Rust provides stronger compile-time guarantees, reducing runtime errors and enhancing code safety. Compared to C++, Rust offers a more modern syntax and built-in patterns for addressing common programming challenges, such as error handling and null safety. As you continue your journey with Rust, you'll find that these foundational concepts enable you to write code that is not only safe and efficient but also clear and maintainable. The unique features of Rust, such as the ownership system and pattern matching, may take some time to master, but they provide powerful tools for creating robust and performant applications. Embracing these concepts will help you harness the full potential of Rust, leading to the development of high-quality software.
Happy coding!
Subscribe to my newsletter
Read articles from Siddhesh Parate directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
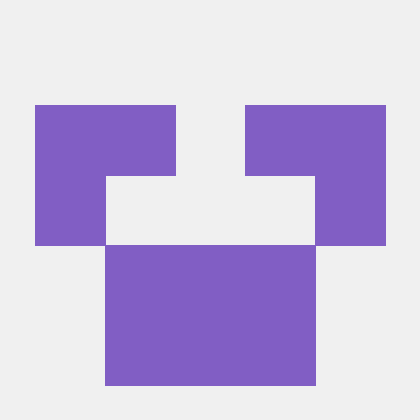