Advance Shell Scripting in Linux

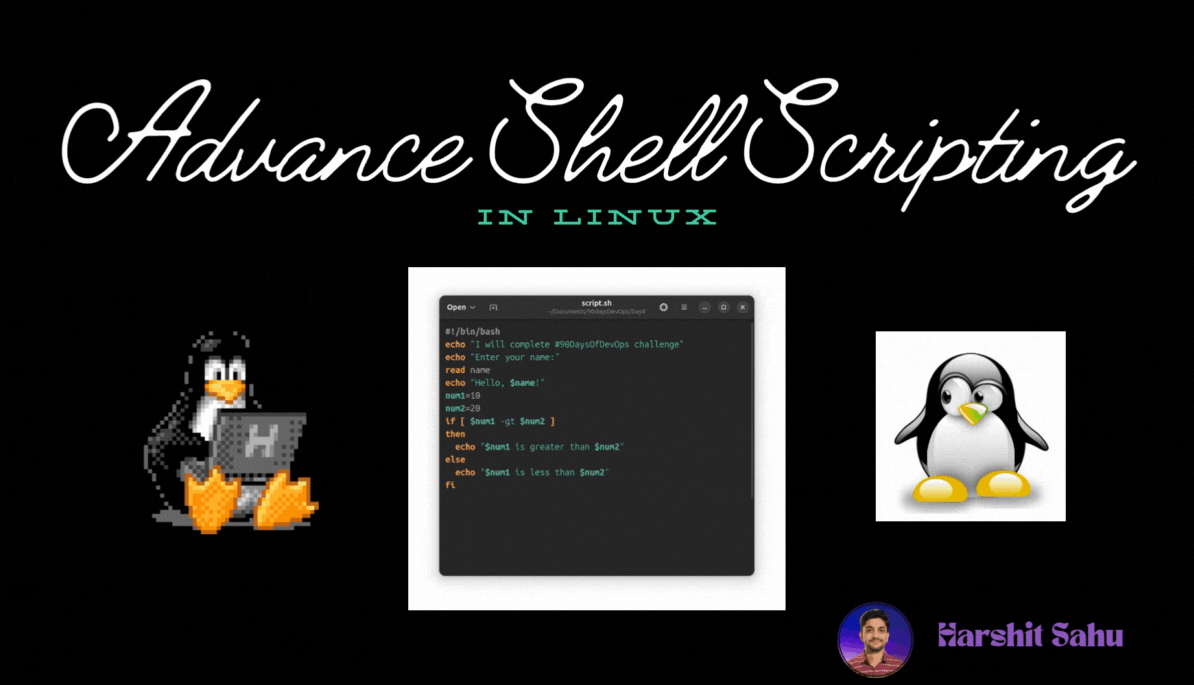
Tasks:
Create Directories Using Shell Script:
Write a bash script
createDirectories.sh
that, when executed with three arguments (directory name, start number of directories, and end number of directories), creates a specified number of directories with a dynamic directory name.Example 1: When executed as
./createDirectories.sh day 1 90
, it creates 90 directories asday1 day2 day3 ... day90
.Example 2: When executed as
./createDirectories.sh Movie 20 50
, it creates 31 directories asMovie20 Movie21 Movie22 ... Movie50
.
Shell Script: createDirectories.sh
#!/bin/bash
# Script to create a range of directories with dynamic names
# Check if the correct number of arguments is provided
if [ $# -ne 3 ]; then
echo "Usage: $0 <directory name> <start number> <end number>"
exit 1
fi
# Assign the arguments to variables
dir_name=$1
start=$2
end=$3
# Create the directories in the specified range
for ((i=start; i<=end; i++))
do
mkdir "${dir_name}${i}"
done
echo "Directories created from ${dir_name}${start} to ${dir_name}${end}."
Explanation:
$# -ne 3
: This checks if the script is provided with exactly 3 arguments.${dir_name}${i}
: Concatenates the directory name with the number (i
).for ((i=start; i<=end; i++))
: A loop to create directories from the start to the end number.mkdir "${dir_name}${i}"
: Creates each directory dynamically.
Steps to Run the Script:
Save the script as
createDirectories.sh
.Make the script executable:
chmod +x createDirectories.sh
Run the script with the required arguments. For example:
./createDirectories.sh day 1 90
Example:
$ ./createDirectories.sh day 1 90
Directories created from day1 to day90.
This will create directories named day1
, day2
, ..., day90
.
Create a Script to Backup All Your Work:
Backups are an important part of a DevOps Engineer's day-to-day activities.
Make a directory name backup
mkdir backup
cd backup
Shell Script: backup.sh
backup_dir="/root" # Change this to your backup directory
timestamp=$(date +"%Y%m%d_%H%M%S")
backup_file="${backup_dir}/backup_${timestamp}.tar.gz"
# Create a backup
tar -czf $backup_file /root/backup # Change this to the directory you want to backup
echo "Backup created at $backup_file"
Steps to Run the Script:
Save the script as
backup.sh
.Make the script executable:
chmod +x backup.sh
Run the script:
./backup.sh
Cron and Crontab to Automate the Backup Script:
- Cron is the system's main scheduler for running jobs or tasks unattended. A command called crontab allows the user to submit, edit, or delete entries to cron. A crontab file is a user file that holds the scheduling information.
Read About User Management:
A user is an entity in a Linux operating system that can manipulate files and perform several other operations. Each user is assigned an ID that is unique within the system. IDs 0 to 999 are assigned to system users, and local user IDs start from 1000 onwards.
Create 2 users and display their usernames.
#!/bin/bash
# Create User
useradd Harshit
useradd Shubham
# Display Usernames
echo "Created Users:"
cut -d: -f1 /etc/passwd | grep 'user1\|user2'
Subscribe to my newsletter
Read articles from Harshit Sahu directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Harshit Sahu
Harshit Sahu
Enthusiastic about DevOps tools like Docker, Kubernetes, Maven, Nagios, Chef, and Ansible and currently learning and gaining experience by doing some hands-on projects on these tools. Also, started learning about AWS and GCP (Cloud Computing Platforms).