Extends vs Implements in Java: A Comprehensive Guide

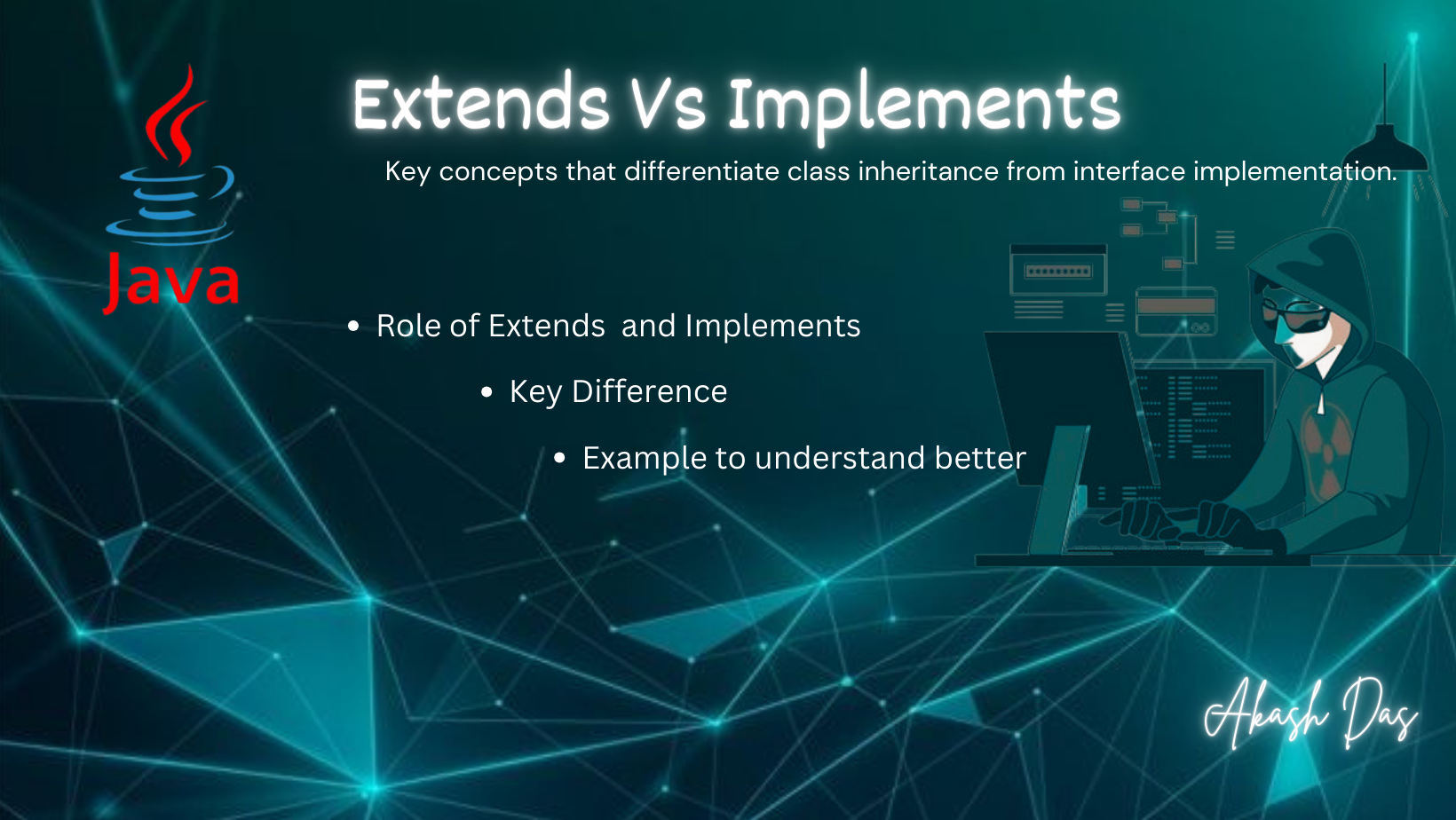
Have you ever thought about the difference between the extends
keyword and the implements
keyword? Have you noticed where these keywords are used? Now, don’t just say, “In Java, of course!” Just kidding! Obviously, we use these keywords in Java, but what’s their purpose? Let’s dive in.
I hope you have a solid grasp of the concept of inheritance. If not, no worries! Inheritance is a fundamental Object-Oriented Programming (OOP) concept where a subclass inherits the properties of its superclass. Now you might wonder, “What is a subclass and a superclass?” Think of it this way: if you inherit traits from your parents—like their genes or characteristics—you are the subclass, and your parents are the superclass. Similarly, in Java, a class that inherits from another class is called a subclass, while the class from which properties are inherited is known as the superclass.
The Role of extends
and implements
So, what’s the connection between inheritance and these two keywords? When inheriting properties of a superclass, how does a subclass inherit them? That’s where extends
and implements
come into play.
extends
Keyword: This keyword is used when a class is inheriting from another class. It establishes a parent-child relationship between classes. For instance, if you have a class calledAnimal
and a subclass calledDog
, you can define it like this:class Animal { void eat() { System.out.println("This animal eats food."); } } class Dog extends Animal { void bark() { System.out.println("The dog barks."); } }
In this example,
Dog
inherits theeat
method fromAnimal
. If you create an instance ofDog
, it can call botheat
andbark
methods.implements
Keyword: This keyword is used when a class implements an interface. Interfaces are like contracts; they define methods that the implementing class must provide. For instance, consider the following interface and class:interface CanFly { void fly(); } class Bird implements CanFly { public void fly() { System.out.println("The bird flies high."); } }
Here,
Bird
implements theCanFly
interface, providing its own implementation of thefly
method. A class can implement multiple interfaces, allowing for a more flexible design.
Key Differences :
Let’s summarize the differences between extends
and implements
:
Inheritance Type:
extends
is for class inheritance (single inheritance). A class can only extend one other class.implements
is for interface inheritance, allowing a class to implement multiple interfaces, enabling multiple inheritance of type.
Purpose:
extends
allows a subclass to inherit fields and methods from its superclass.implements
requires a class to provide implementations for all methods declared in the interface.
Constructor Behavior :
extends
: When creating an instance of a subclass, the constructor of the superclass is called first.implements
: Interfaces do not have constructors, and the methods defined in an interface are inherently abstract unless specified as default or static.
Feature | extends | implements |
Type | Used with classes | Used with interfaces |
Inheritance | Single inheritance only | Multiple inheritance allowed |
Method Implementation | Inherits methods from superclass | Must implement all methods from interface |
Constructor | during instantiation of the child class parent constructor will call first | Not applicable |
Example :
Let’s consider a real-world scenario. Imagine you’re building a transportation system. You might have a base class called Vehicle
, and specific vehicle types like Car
, Bicycle
, and Boat
:
class Vehicle {
void start() {
System.out.println("Vehicle is starting.");
}
}
class Car extends Vehicle {
void honk() {
System.out.println("Car honks!");
}
}
class Bicycle extends Vehicle {
void ringBell() {
System.out.println("Bicycle bell rings!");
}
}
Now, if you want to define behaviors that are not tied to the vehicle type but are shared across different vehicles, you might use interfaces:
interface Electric {
void charge();
}
class ElectricCar extends Car implements Electric {
public void charge() {
System.out.println("Charging the electric car.");
}
}
Conclusion :
Understanding the differences between extends
and implements
is crucial for effective Java programming. By using extends
, you can create a clear hierarchy and inherit common functionality. On the other hand, implements
offers flexibility and promotes a contract-based design, allowing multiple behaviors to be added to classes.
In summary, while extends
is about building upon existing classes and their functionalities, implements
allows you to define capabilities across unrelated classes. This distinction is vital in crafting clean, maintainable, and scalable Java applications. So next time you write Java code, think about which keyword suits your design needs best. Happy coding!
Subscribe to my newsletter
Read articles from Akash Das directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Akash Das
Akash Das
Java Developer | Tech Blogger | Spring Boot Enthusiast | DSA Advocate*I specialize in Java, Spring Boot, and Data Structures & Algorithms (DSA). Follow my blog for in-depth tutorials, best practices, and insights on Java development, Spring Boot, and DSA. Join me as I explore the latest trends and share valuable knowledge to help developers grow.