Build Your Own Gym Buddy ChatBot with React: Ready to Flex Some Code?

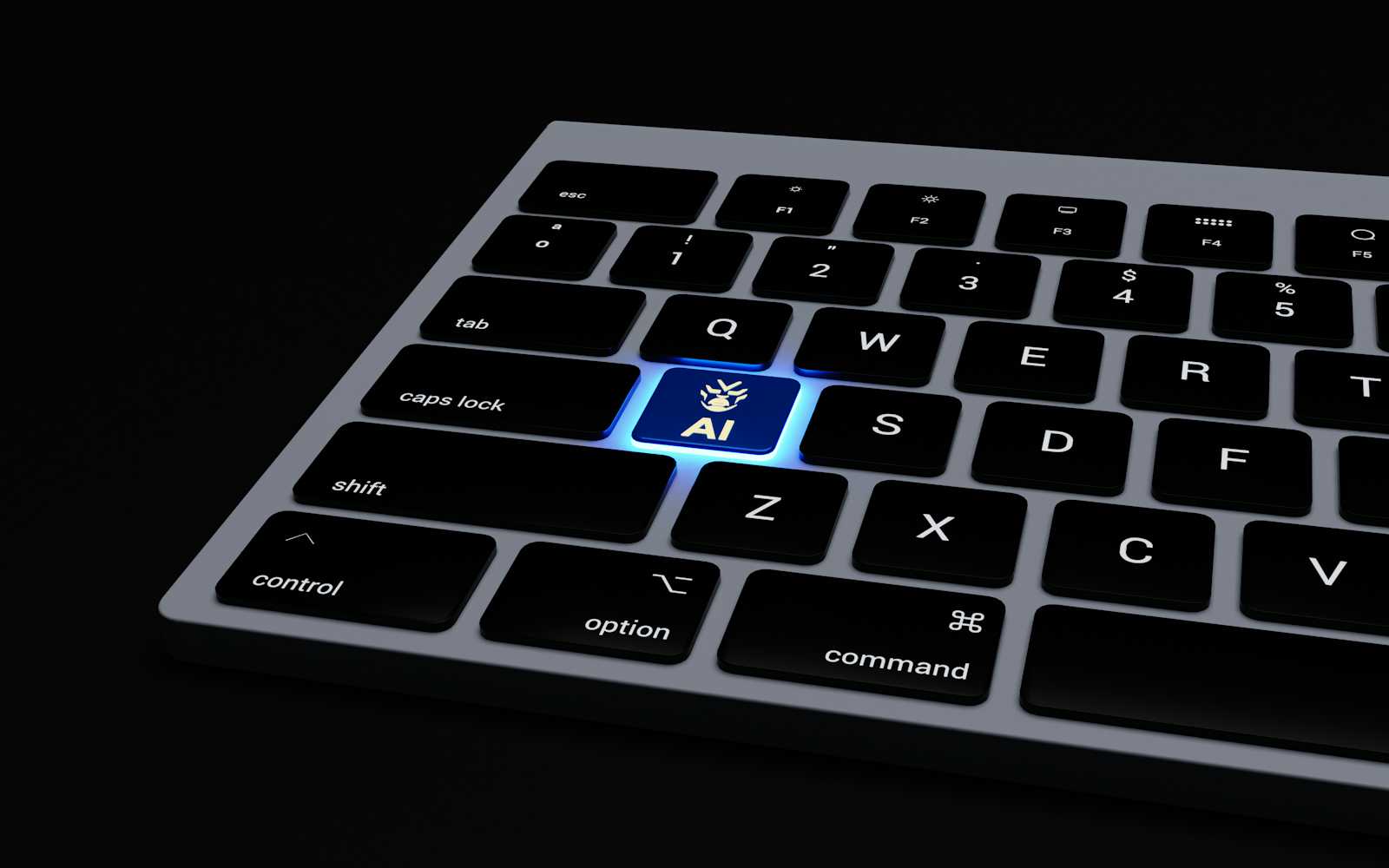
Hey, fitness buffs and coding wizards! Today, we’re diving into a project that’s all about pumping up both your coding and workout game. Imagine having a chatbot that not only chats back but motivates you like a gym buddy who's got your back.
Ready? Let’s build Gym Buddy, your personal workout chatbot—complete with style, smarts, and a bit of sass!. Pun intended😊.
What’s Gym Buddy?
Gym Buddy is a chatbot built with React that can take your questions and respond like a real friend (or at least pretend to be one!). But, unlike your lazy friend who skips leg day, this chatbot will always reply—no excuses!
Here’s what we’ll do:
Make it talk: You ask, Gym Buddy answers. Simple!
Keep it sharp: We’ll design a modern UI because Gym Buddy needs to look as good as you.
Integrate Axios: We’ll communicate with a backend server to power Gym Buddy’s responses.
Project Structure 🏗️
Here’s the React app structure:
my-gym-buddy-chatbot
│
├── public
├── src
│ ├── assets
│ │ └── striveFit.webp # Logo for Gym Buddy
│ ├── components
│ │ └── ChatBot.jsx # ChatBot component
│ ├── App.jsx # Main app component that uses ChatBot
│ ├── main.jsx # Entry point for React
│ └── index.css # Global styles
└── package.json
This structure helps keep your components and assets neatly organized, and it’s easy to scale as your app grows.
Step 1: Set Up the Project
First, let’s set up the React app. If you haven’t already:
npx create-react-app my-gym-buddy-chatbot
Once the setup is complete, navigate into your project directory:
cd my-gym-buddy-chatbot
You’ll also need to install Axios:
npm install axios
Step 2: Building the ChatBot Component
Inside the src/components/ChatBot.jsx
, we’ll build the brain of Gym Buddy. This is where the messages will be sent and received.
import React, { useState, useEffect } from 'react';
import axios from 'axios';
import './chatbot.css';
const ChatBot = () => {
const [messages, setMessages] = useState([]);
const [question, setQuestion] = useState('');
const handleSend = async (e) => {
e.preventDefault();
if (question.trim()) {
setMessages((prevMessages) => [
...prevMessages,
{ sender: 'user', text: question },
]);
try {
const response = await axios.post(http://localhost:5000/chat, {
message: question,
});
// Add bot response to the message list
setMessages((prevMessages) => [
...prevMessages,
{ sender: 'Gym Buddy', text: response.data.response },
]);
} catch (error) {
console.error('Error fetching response:', error);
return;
}
setQuestion('');
}
};
const handleOnChange = (e) => {
setQuestion(e.target.value);
};
const handleClearQuestion = () => {
setQuestion('');
};
const handleKeyPress = (e) => {
if (e.key === 'Enter' && !e.shiftKey) {
handleSend(e);
}
};
return (
<div className='chat-container'>
<div className='chat-header'>
<img
src='../src/assets/striveFit.webp'
alt='Gym Buddy Logo'
className='chat-logo'
/>
<h2>Gym Buddy</h2>
</div>
<div className='chat-window'>
{messages.map((msg, index) => (
<div
key={index}
className={`message-bubble ${
msg.sender === 'user' ? 'user-bubble' : 'bot-bubble'
}`}
>
<strong>{msg.sender === 'user' ? 'You' : 'Gym Buddy'}:</strong>{' '}
{msg.text}
</div>
))}
</div>
<form className='chat-input-form' onSubmit={handleSend}>
<textarea
required
value={question}
onChange={handleOnChange}
onKeyDown={handleKeyPress} // Detect Enter key
placeholder='Type your message...'
className='chat-input'
/>
<div className='chat-actions'>
<button
disabled={!question.trim()}
type='submit'
className='send-button'
>
Send
</button>
<button
disabled={!question.trim()}
type='button'
onClick={handleClearQuestion}
className='clear-button'
>
Clear
</button>
</div>
</form>
</div>
);
};
export default ChatBot;
Now, let’s connect this ChatBot to the main App.jsx file.
Step 3: Connecting to the App
In src/App.jsx
, we’ll import the ChatBot component and render it within the main application layout.
import { useState } from 'react';
import reactLogo from './assets/react.svg';
import viteLogo from '/vite.svg';
import './App.css';
import ChatBot from './components/ChatBot';
function App() {
return (
<>
<h1>Gym Buddy Chatbot</h1>
<ChatBot />
</>
);
}
export default App;
You might want to add your styles using App.css
.
#root {
max-width: 1280px;
margin: 0 auto;
padding: 2rem;
text-align: center;
}
.logo {
height: 6em;
padding: 1.5em;
will-change: filter;
transition: filter 300ms;
}
.logo:hover {
filter: drop-shadow(0 0 2em #646cffaa);
}
.logo.react:hover {
filter: drop-shadow(0 0 2em #61dafbaa);
}
@keyframes logo-spin {
from {
transform: rotate(0deg);
}
to {
transform: rotate(360deg);
}
}
@media (prefers-reduced-motion: no-preference) {
a:nth-of-type(2) .logo {
animation: logo-spin infinite 20s linear;
}
}
.card {
padding: 2em;
}
.read-the-docs {
color: #888;
}
In src/main.jsx
, we’ll ensure the App.jsx file is correctly mounted to the root div.
import React from 'react'
import ReactDOM from 'react-dom/client'
import App from './App.jsx'
import './index.css'
ReactDOM.createRoot(document.getElementById('root')).render(
<React.StrictMode>
<App />
</React.StrictMode>,
)
index.css
:root {
font-family: Inter, system-ui, Avenir, Helvetica, Arial, sans-serif;
line-height: 1.5;
font-weight: 400;
color-scheme: light dark;
color: rgba(255, 255, 255, 0.87);
background-color: #242424;
font-synthesis: none;
text-rendering: optimizeLegibility;
-webkit-font-smoothing: antialiased;
-moz-osx-font-smoothing: grayscale;
}
a {
font-weight: 500;
color: #646cff;
text-decoration: inherit;
}
a:hover {
color: #535bf2;
}
body {
margin: 0;
display: flex;
place-items: center;
min-width: 320px;
min-height: 100vh;
}
h1 {
font-size: 3.2em;
line-height: 1.1;
}
button {
border-radius: 8px;
border: 1px solid transparent;
padding: 0.6em 1.2em;
font-size: 1em;
font-weight: 500;
font-family: inherit;
background-color: #1a1a1a;
cursor: pointer;
transition: border-color 0.25s;
}
button:hover {
border-color: #646cff;
}
button:focus,
button:focus-visible {
outline: 4px auto -webkit-focus-ring-color;
}
@media (prefers-color-scheme: light) {
:root {
color: #213547;
background-color: #ffffff;
}
a:hover {
color: #747bff;
}
button {
background-color: #f9f9f9;
}
}
Getting Started with the Backend (Python) 🐍
Now that we’ve covered the frontend, let’s build the brain behind Gym Buddy using Python. The backend will handle incoming messages, process them, and respond with some well-earned advice (or witty banter).
Step 1: Set Up a Python Virtual Environment
Before we start coding, we’ll need a Python environment for our backend. Setting up a virtual environment keeps your project dependencies clean and organized.
Open your terminal or command prompt.
Navigate to your project directory:
cd my-gym-buddy-chatbot
- Create a virtual environment using venv:
python -m venv venv
This will create a directory called venv
that holds the Python environment.
- Activate the virtual environment:
On Windows:
venv\Scripts\activate
On macOS/Linux:
source venv/bin/activate
You should now see (venv)
at the start of your terminal prompt, indicating that the environment is active.
- Install required Python packages. We’ll be using Flask for our web server:
pip install Flask
Step 2: Create the Backend Files
Now, let’s write the backend code. In your project directory, create a new folder called backend:
mkdir backend
cd backend
Inside this folder, we’ll create the Python files to handle the chatbot’s logic.
Step 3: Writing the Flask Server
Create a file called gym_buddy.py in the backend folder. This file will handle the incoming requests and send back the chatbot's response.
gym_buddy.py :
from flask import Flask, jsonify, request
from flask_cors import CORS
import nltk
from chatterbot import ChatBot
from chatterbot.trainers import ListTrainer
from training_data import training_data # Import the training data
app = Flask(__name__)
CORS(app)
# Download necessary NLTK data
nltk.download('punkt')
nltk.download('averaged_perceptron_tagger')
nltk.download('stopwords')
# Initialize chatbot
chatbot = ChatBot('Gym Buddy')
# Wipe the database
chatbot.storage.drop()
trainer = ListTrainer(chatbot)
# Train the chatbot with the imported data
trainer.train(training_data)
# Define API route to receive responses
@app.route('/chat', methods=['POST'])
def chatBot():
data = request.json
user_message = data.get('message')
if not user_message:
return jsonify({"error": "Message Content missing"}), 400
response = chatbot.get_response(user_message)
return jsonify({"response": str(response)})
if __name__ == "__main__":
app.run(debug=True)
training_data
training_data = [
# Greetings
"Hello", "Hi there! Welcome to Gym Buddy! How can I assist you today?",
"Hi", "Hello! Ready to hit the gym? How can I help you?",
"Good morning", "Good morning! Let's make today a great workout day!",
"Good afternoon", "Good afternoon! How can I support your fitness journey today?",
"Good evening", "Good evening! Ready to unwind with a good workout?",
# Getting Started
"Do I need to be a member to use the gym?", "Yes, our gym operates on a membership basis. We offer various membership options to suit your needs and budget.",
"How do I become a member?", "There are three ways to sign up for a membership: Visit our front desk and speak with a friendly staff member. Sign up online through our website. Call us at 079556432 to discuss membership options.",
"What if I'm new to exercise?", "We welcome people of all fitness levels! We offer introductory classes and personal training sessions to help you get started on the right foot.",
"Do I need to bring my own workout clothes and towels?", "Yes, please bring comfortable workout clothing and a water bottle. Towels are available for purchase at the front desk.",
"What are your gym hours?", "Our gym is open for 24 hours, every day. Please check your membership category to know what times you’ll have access.",
# Nutrition Advice
"What should I eat before a workout?", "It's best to have a balanced meal with carbohydrates and protein 1-2 hours before your workout. Options include a banana with peanut butter or oatmeal with berries.",
"What should I eat after a workout?", "After your workout, focus on protein to aid muscle recovery. Consider options like a protein shake, Greek yogurt, or grilled chicken with vegetables.",
"How can I stay hydrated?", "Drink water throughout the day, especially before, during, and after your workout. Aim for at least 8 glasses a day, more if you're exercising vigorously.",
"Do you have any healthy snack recommendations?", "Absolutely! Try nuts, fruits, yogurt, or protein bars for quick and healthy snacks.",
# Calendars for Routines
"Can you help me create a workout routine?", "Of course! Let’s design a routine. What are your fitness goals: weight loss, muscle gain, or general fitness?",
"What does a weekly workout routine look like?", "A typical weekly routine could include: Monday - Upper body strength, Tuesday - Cardio, Wednesday - Lower body strength, Thursday - Rest, Friday - Full-body workout, Saturday - Active recovery (like yoga), Sunday - Rest.",
"What about a daily routine?", "A daily routine might include: Warm-up (5-10 min), Strength training (30-40 min), Cardio (20-30 min), Cool down (5-10 min), Stretching (5-10 min).",
"How can I track my progress?", "Consider using a fitness app or journal to log your workouts and nutrition. Regularly check your progress against your goals.",
# Membership Options
"What types of memberships do you offer?", "We offer a variety of membership options to fit your needs and budget. These include: Individual memberships, Couples memberships, Family memberships, Student discounts, Senior citizen discounts, Pay-as-you-go options.",
"Can I switch my membership type?", "Yes, you can upgrade or downgrade your membership at any time by contacting our front desk staff.",
"What are the payment terms for memberships?", "Most memberships require a monthly commitment with automatic payments. However, we also offer annual memberships at a discounted rate.",
"Is there a contract involved with a membership?", "Some memberships require a minimum commitment period, typically one month or one year. Please refer to the specific membership details for more information.",
"What happens if I need to cancel my membership?", "We understand that life happens! You can cancel your membership by giving us [Notice Period] written notice. Please refer to your membership agreement for details on cancellation fees.",
# Gym Facilities and Services
"What are your peak and off-peak hours?", "Generally, our peak hours are: Weekdays: Early mornings (before 8am) and evenings (after 4pm), Weekends: Mornings (between 9am and 12pm).",
"Do my membership discounts apply during peak hours?", "Some membership discounts, such as student discounts, may not apply during peak hours. Please refer to your specific membership agreement for details.",
"Is the gym less crowded during off-peak hours?", "Yes, the gym is typically less crowded during off-peak hours. This can be a good option if you prefer a quieter workout environment.",
"What kind of equipment do you have?", "Our gym boasts a wide variety of state-of-the-art equipment, including: Cardio machines (treadmills, ellipticals, stationary bikes), Strength training machines (weight benches, free weights, cable machines), Functional training equipment (medicine balls, TRX trainers, kettlebells), Group fitness studios for classes.",
"Do you offer locker rooms with showers?", "Yes, we have clean and well-maintained locker rooms with showers, toilets, and changing areas. Get your padlocks for the lockers.",
"Do you have Wi-Fi available?", "Yes, we offer free Wi-Fi access for our members.",
"Is there a juice bar or cafe on-site?", "We do not have a full-service cafe, but we offer a selection of healthy snacks and beverages for purchase. There is water at the water fountain, free for everyone.",
"Do you provide childcare services?", "Unfortunately, we do not offer on-site childcare services at this time.",
# Personal Training
"Do you offer personal training services?", "Absolutely! We have a team of certified and experienced personal trainers who can create a personalized workout program to help you reach your fitness goals.",
"How much does personal training cost?", "The cost of personal training varies depending on the trainer's experience, the number of sessions purchased, and the package chosen. We offer consultations to discuss your needs and provide a personalized quote.",
"What if I'm new to exercise and need some guidance?", "Personal training is a great option for beginners! Your trainer will design a safe and effective program tailored to your fitness level and goals.",
"Can I train with a friend or group?", "Yes, we offer small group personal training sessions, which can be a cost-effective and motivating way to train with friends.",
"How do I schedule a personal training consultation?", "Contact our front desk staff or visit our website to book a consultation with a personal trainer.",
# Policies
"What is your guest policy?", "Members are welcome to bring a guest for a day fee. Guests must complete a waiver form before using the gym facilities.",
"What is your dress code?", "We require proper workout attire that is clean and modest. Please refrain from wearing denim, street shoes, or revealing clothing.",
"Do you have a lost and found?", "Yes, we have a lost and found for any items left behind in the gym. Unclaimed items are donated to charity after a certain period.",
"What is your policy on food and drinks in the gym?", "We ask that members refrain from bringing outside food or drinks into the gym. We offer a selection of healthy snacks and beverages for purchase.",
"Do you allow pets in the gym?", "Unfortunately, no pets are allowed in the gym for safety and hygiene reasons. Service animals are permitted.",
# Safety
"Do you have staff on-site to assist with workouts?", "Yes, we have certified fitness professionals available on the gym floor to answer questions and provide guidance.",
"What kind of safety equipment do you have?", "We provide first-aid kits and automated external defibrillators (AEDs) on-site in case of emergencies.",
"Do you require members to undergo a fitness assessment?", "While not mandatory, we recommend a fitness assessment for new members, especially those with pre-existing health conditions. This helps us personalize your workout program and ensure your safety.",
"What should I do if I get injured while working out?", "Inform a staff member immediately if you experience any pain or injury while using the gym facilities.",
"Do you offer CPR or first-aid training?", "We may offer occasional CPR or first-aid training courses. Please inquire at the front desk for details.",
# Community
"How can I get involved in the gym community?", "We offer a variety of group fitness classes, workshops, and social events throughout the month. These are great opportunities to meet other members, stay motivated, and learn something new.",
"If I have further questions, how can I contact you?", "If you have any further questions, please don't hesitate to contact our friendly staff at 079556432 or visit our website at sample.com."
]
This basic Flask app listens for POST requests on the /chat
endpoint. It takes the user message, processes it, and sends back a response. You can expand the get\_bot\_response
function to make Gym Buddy even smarter!
Step 4: Running the Flask Server
To run the server, make sure you’re in the backend folder and have your virtual environment activated:
python3 chatbot.py
Your server will start on http://localhost:5000
. This is where the React frontend will send user messages and get responses.
Step 5: Connecting Frontend and Backend
Now that the Flask server is running, we need to ensure our React app sends requests to this server.
In the ChatBot component of the React app, we’re already using Axios to send requests to the backend. Make sure the URL points to http://localhost:5000/chat
, which is where our Flask app is running.
Wrapping Up the Backend 🧠
At this point, you should have both the React frontend and Flask backend working together:
The user types a message in the chatbox.
The message is sent to the Flask backend.
Gym Buddy processes the message and sends a response back to the frontend.
The user sees the response on the chat window.
Check Out the Code
Want to see the full code and contribute? Head over to the GitHub repository
Try It Live!
Coming soon! You’ll be able to chat with Gym Buddy yourself
Next Steps 🔥
Now that you’ve got the basics down, here are some cool features you can add:
Natural Language Processing (NLP): Integrate GPT-3, DialogFlow, or other AI models to make Gym Buddy smarter.
More Features: Gym Buddy could give personalized workout advice, track user progress, or suggest routines.
Let’s keep this project moving! You’ve got your chatbot built and working. What’s next? Get it hosted, share it with friends, and keep improving. You’re one step closer to building the ultimate fitness assistant!
Subscribe to my newsletter
Read articles from Etiene James directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Etiene James
Etiene James
I am a Software Engineer, Tech Writer based in Lagos, Nigeria. I am passionate about solving problems, sharing knowledge gained during my Tech journey, and excited in meeting people.