RBAC Policy as Code in K8s
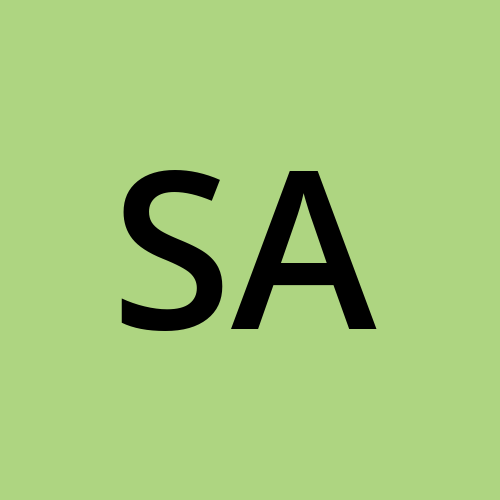
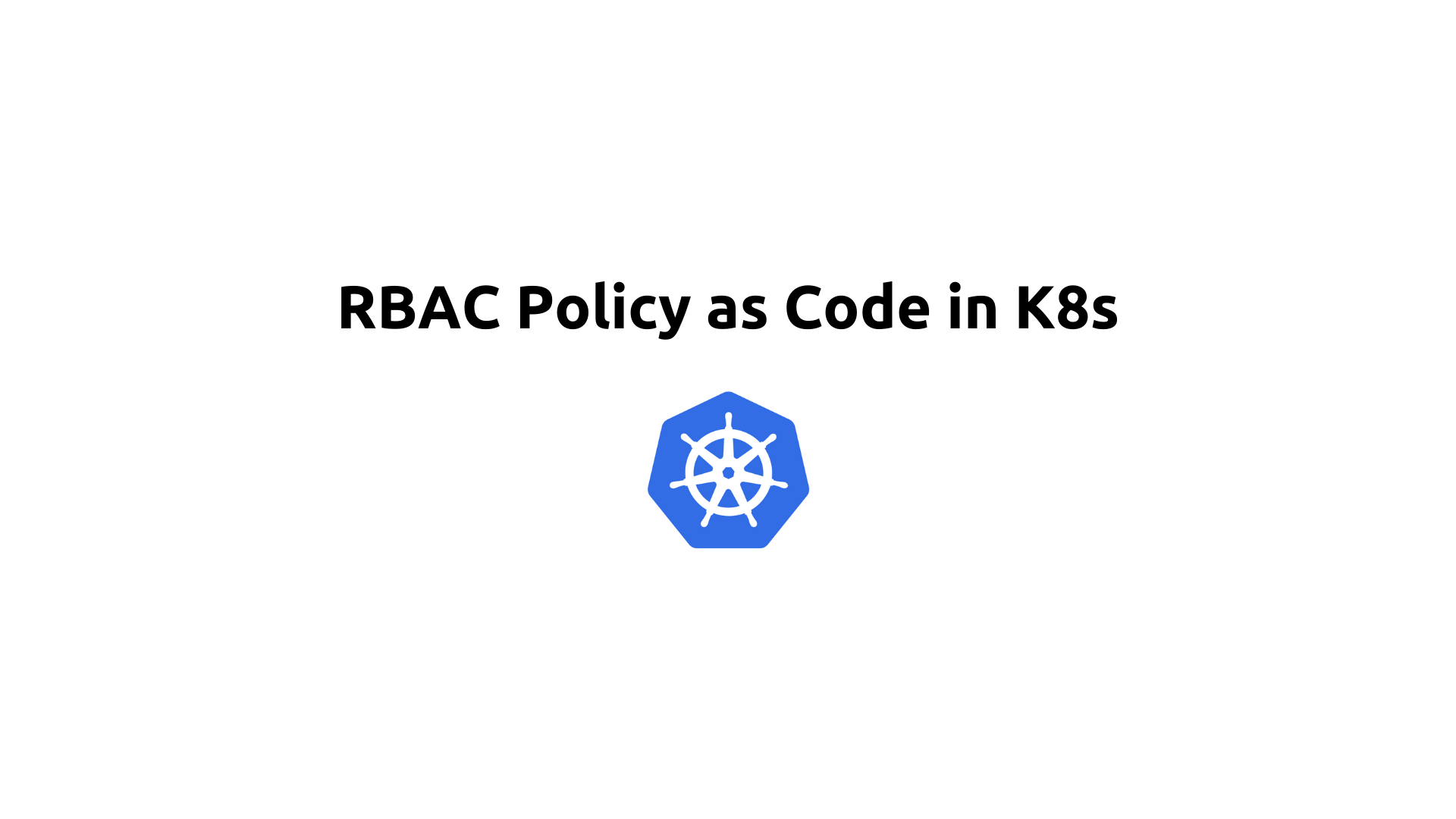
Introduction
Policy as Code is a practice that involves defining and managing policies through code. In the context of Role-Based Access Control (RBAC) in Kubernetes, Policy as Code means specifying access control policies (who can do what within the cluster) using code, usually in the form of configuration files. This approach enables version control, automated testing, and easier management of policies.
Key Concepts
Declarative Configuration
Version Control
Automated Validation
Continuous Integration/Continuous Deployment (CI/CD)
Declarative Configuration
In Kubernetes, RBAC policies are defined declaratively using YAML or JSON configuration files. These files specify Roles, ClusterRoles, RoleBindings, and ClusterRoleBindings.
Example of a Role:
apiVersion: rbac.authorization.k8s.io/v1
kind: Role
metadata:
namespace: my-namespace
name: pod-reader
rules:
- apiGroups: [""]
resources: ["pods"]
verbs: ["get", "list", "watch"]
Example of a RoleBinding:
apiVersion: rbac.authorization.k8s.io/v1
kind: RoleBinding
metadata:
name: read-pods-binding
namespace: my-namespace
subjects:
- kind: User
name: jane
apiGroup: rbac.authorization.k8s.io
roleRef:
kind: Role
name: pod-reader
apiGroup: rbac.authorization.k8s.io
Version Control
By defining RBAC policies as code, you can manage them using version control systems like Git. This approach provides several benefits:
History and Auditing: Track changes to policies over time.
Collaboration: Enable multiple team members to collaborate on policy definitions.
Rollback: Easily revert to previous versions of policies if needed.
Example in Version Control
Initialize a Git Repository: Initialize a Git repository in your local directory.
git init
Add RBAC Policy Files: Create and add your RBAC policy files (e.g.,
role.yaml
,rolebinding.yaml
) to the repository.git add role.yaml rolebinding.yaml
Commit Changes: Commit the changes with a descriptive message.
git commit -m "Add initial RBAC policies for pod-reader role"
Push to Remote Repository: Push the changes to a remote repository, such as GitHub or GitLab.
git remote add origin <remote-repo-url> git push -u origin master
Automated Validation
Automated validation tools can be used to ensure that RBAC policies are correctly defined and adhere to best practices. These tools can check for common issues such as:
Overly Broad Permissions: Identifying roles that grant excessive access.
Consistency: Ensuring that policies are consistent across different environments.
Examples of tools for policy validation:
OPA (Open Policy Agent): A powerful policy engine that can enforce custom policies.
kube-linter: A static analysis tool that checks Kubernetes YAML files for potential issues.
Example of an OPA policy to check for overly broad permissions:
package kubernetes.rbac
deny[msg] {
input.kind == "Role"
input.rules[_].verbs[_] == "*"
msg = sprintf("Role '%s' grants wildcard permissions.", [input.metadata.name])
}
Continuous Integration/Continuous Deployment (CI/CD)
By integrating RBAC policies into your CI/CD pipeline, you can automate the deployment and testing of policies. This ensures that changes to policies are applied consistently across environments and reduces the risk of manual errors.
Example CI/CD Pipeline with GitHub Actions
Create a GitHub Actions Workflow: Create a
.github/workflows/deploy-rbac.yaml
file in your repository.name: Deploy RBAC Policies on: push: branches: - main jobs: validate-and-deploy: runs-on: ubuntu-latest steps: - name: Checkout repository uses: actions/checkout@v2 - name: Set up Kubernetes CLI uses: azure/setup-kubectl@v1 with: version: 'v1.20.0' - name: Validate RBAC Policies run: | # Run OPA validation or other validation tools # opa eval --data rbac.rego --input role.yaml --format pretty - name: Apply RBAC Policies run: | kubectl apply -f role.yaml kubectl apply -f rolebinding.yaml
Commit and Push Workflow: Commit and push the GitHub Actions workflow file to your repository.
git add .github/workflows/deploy-rbac.yaml git commit -m "Add GitHub Actions workflow for RBAC policy deployment" git push origin main
Monitor the Workflow: Navigate to the "Actions" tab in your GitHub repository to monitor the CI/CD pipeline execution. The workflow will validate and deploy the RBAC policies whenever changes are pushed to the
main
branch.
Benefits of Policy as Code in RBAC
Consistency: Ensures that RBAC policies are applied consistently across different environments.
Audibility: Provides a clear audit trail of changes to policies.
Security: Reduces the risk of misconfigurations that could lead to security vulnerabilities.
Automation: Enables automated validation and deployment of policies, reducing manual effort and errors.
Collaboration: Facilitates collaboration among team members by using version control systems.
Conclusion
Policy as Code is a powerful approach to managing RBAC in Kubernetes. By defining policies declaratively, using version control, automating validation, and integrating with CI/CD pipelines, organizations can achieve greater consistency, security, and efficiency in managing access controls. This practice not only enhances the security posture of the cluster but also simplifies the process of managing and auditing access policies.
Subscribe to my newsletter
Read articles from Saurabh Adhau directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
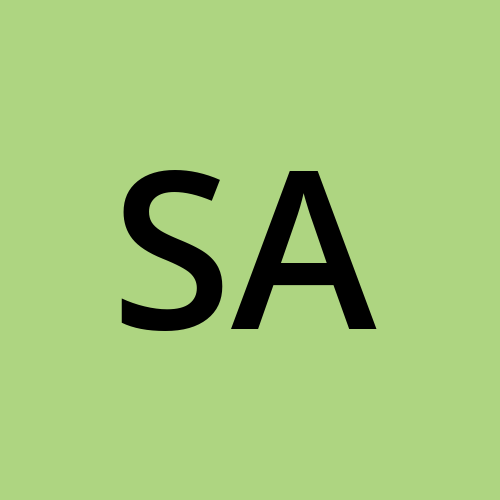
Saurabh Adhau
Saurabh Adhau
As a DevOps Engineer, I thrive in the cloud and command a vast arsenal of tools and technologies: โ๏ธ AWS and Azure Cloud: Where the sky is the limit, I ensure applications soar. ๐จ DevOps Toolbelt: Git, GitHub, GitLab โ I master them all for smooth development workflows. ๐งฑ Infrastructure as Code: Terraform and Ansible sculpt infrastructure like a masterpiece. ๐ณ Containerization: With Docker, I package applications for effortless deployment. ๐ Orchestration: Kubernetes conducts my application symphonies. ๐ Web Servers: Nginx and Apache, my trusted gatekeepers of the web.