Exploring Python Dictionaries: An In-Depth Guide

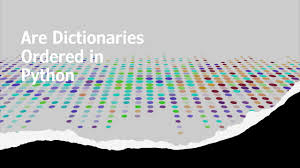
In Python, a dictionary is a built-in data type that allows us to store key-value pairs, enabling fast lookups and efficient data storage. Python dictionaries are ordered as of Python 3.7+, meaning that they maintain the order in which the keys were added. Let’s dive into how dictionaries work and explore some common methods.
Creating a Dictionary
A dictionary in Python can be created using curly braces {}
and separating keys and values with a colon (:
):
pythonCopy code# Example of a simple dictionary
student = {
'name': 'Alice',
'age': 22,
'major': 'Computer Science'
}
Accessing Dictionary Values
You can access values by referencing the key:
pythonCopy codeprint(student['name']) # Output: Alice
If you try to access a key that doesn’t exist, it will raise a KeyError
. To avoid this, use the .get()
method:
pythonCopy codeprint(student.get('name')) # Output: Alice
print(student.get('gpa', 'N/A')) # Output: N/A
Adding or Updating Entries
To add or update a key-value pair, assign a value to a key:
pythonCopy codestudent['gpa'] = 3.8 # Adds 'gpa': 3.8 to the dictionary
student['age'] = 23 # Updates the value of 'age' to 23
Removing Entries
You can remove entries using pop()
, del
, or popitem()
:
pythonCopy code# Using pop() to remove a specific key
student.pop('gpa') # Removes the key 'gpa'
# Using del to delete a key
del student['major'] # Removes 'major'
# Using popitem() to remove the last inserted key-value pair
student.popitem() # Removes the last item
Dictionary Methods
Here are some useful dictionary methods:
keys()
– Returns a view of all keys:pythonCopy codeprint(student.keys()) # Output: dict_keys(['name', 'age'])
values()
– Returns a view of all values:pythonCopy codeprint(student.values()) # Output: dict_values(['Alice', 23])
items()
– Returns a view of all key-value pairs:pythonCopy codeprint(student.items()) # Output: dict_items([('name', 'Alice'), ('age', 23)])
update()
– Merges one dictionary into another:pythonCopy codeadditional_info = {'university': 'MIT'} student.update(additional_info) print(student) # Output: {'name': 'Alice', 'age': 23, 'university': 'MIT'}
Looping Through a Dictionary
You can loop through keys and values using items()
:
pythonCopy codefor key, value in student.items():
print(f'{key}: {value}')
Use Cases for Python Dictionaries
Storing Configurations: Use dictionaries to store configuration settings where you need key-value mapping.
pythonCopy codeconfig = {"debug": True, "version": 1.0}
Database Records: Use dictionaries to represent a record in a database, where each field is mapped to its value.
pythonCopy codeemployee = {"id": 101, "name": "John", "department": "HR"}
Counting Occurrences: Counting occurrences of items in a list or string.
pythonCopy codetext = "hello world" count = {} for char in text: count[char] = count.get(char, 0) + 1 print(count) # Output: {'h': 1, 'e': 1, 'l': 3, 'o': 2, ' ': 1, 'w': 1, 'r': 1, 'd': 1}
Caching Results: Using dictionaries to store the results of expensive function calls for quick look-up later.
Conclusion
Python dictionaries are incredibly versatile and efficient for handling key-value data. With various methods and functionalities, they offer flexibility for many programming needs. Since Python 3.7, dictionaries have become ordered, making them even more predictable and useful for developers.
Happy coding! 🐍
Subscribe to my newsletter
Read articles from Aakanchha Sharma directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Aakanchha Sharma
Aakanchha Sharma
👋 Welcome to my Hashnode blog! I'm a tech enthusiast and cloud advocate with expertise in IT, backup solutions, and cloud technologies. Currently, I’m diving deep into Python and exploring its applications in automation and data management. I share insights, tutorials, and tips on all things tech, aiming to help fellow developers and enthusiasts. Join me on this journey of learning and innovation!