Vue 2 vs. Vue 3
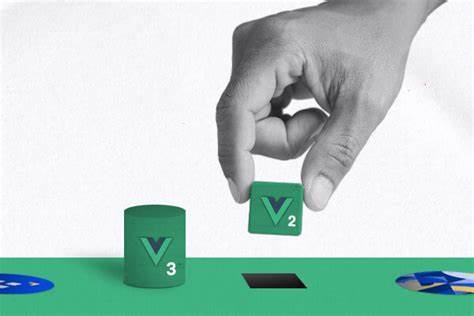
Vue has long been touted as a "progressive JavaScript framework," and if you're familiar with that term, you already know it speaks volumes about the philosophy behind Vue's evolution. Vue 2 was designed to be incrementally adoptable—whether you're working on a small component or a large-scale project, Vue allows you to integrate its features at your own pace. However, as the web evolves and demands on developers change, the need for more advanced features becomes apparent. Enter Vue 3, the next stage in the framework's progression. It builds on Vue 2's solid foundation, introducing enhancements that not only make it more powerful but also keep the essence of being a progressive framework.
In this article, we'll explore the key differences between Vue 2 and Vue 3, and why Vue 3 is a justified next step for anyone developing in this "progressive JavaScript framework." Let’s also use a few examples to break things down, including some code snippets that highlight the improvements.
Composition API vs. Options API
One of the most significant changes in Vue 3 is the introduction of the Composition API. If you've worked with Vue 2, you're familiar with the Options API, which organizes your code by component options like data
, methods
, computed
, and watch
. Here’s a typical example from Vue 2:
// Vue 2 Options API
export default {
data() {
return {
count: 0
}
},
methods: {
increment() {
this.count++
}
}
}
This approach is beginner-friendly, easy to understand, and one of the reasons Vue 2 gained so much popularity. However, as applications grow in complexity, you may find that it becomes harder to manage code that spans across multiple parts of your component (like keeping track of which methods relate to which pieces of data). This is where the Composition API comes in handy:
// Vue 3 Composition API
import { ref } from 'vue'
export default {
setup() {
const count = ref(0)
function increment() {
count.value++
}
return { count, increment }
}
}
With the Composition API, you group code by functionality rather than by type (data, methods, etc.). This makes components easier to maintain, especially as they grow in size. Imagine you’re building a cute to-do app where you track items based on categories. With the Composition API, the logic for each category can stay bundled together, making the code more intuitive and easier to refactor.
This flexibility makes Vue 3 better suited for larger applications while still being friendly to small projects. It’s a progressive way of organizing code, allowing developers to adopt more complex patterns when needed without breaking what’s already familiar.
Performance Boosts: Proxy-based Reactivity
Vue 2’s reactivity system was built using Object.defineProperty()
, which worked well but came with certain limitations, especially when handling arrays and adding new properties to objects dynamically. In Vue 3, this system is replaced by a Proxy-based reactivity system, which offers more power and flexibility.
Let’s say you’re building a fun shopping app where users can add and remove items from a cart. In Vue 2, you might run into issues tracking changes to arrays, but Vue 3’s Proxy-based reactivity handles this much more elegantly:
// Vue 3 Reactive Example
import { reactive } from 'vue'
export default {
setup() {
const cart = reactive([])
function addItem(item) {
cart.push(item)
}
return { cart, addItem }
}
}
In this snippet, the cart is fully reactive, meaning Vue will efficiently track changes to the array without the performance overhead or workarounds needed in Vue 2. The result is faster, smoother updates to the DOM and a more scalable reactivity system. This performance boost is especially noticeable in larger applications, making Vue 3 the better choice for teams building complex, data-heavy web apps.
Fragment Support and Cleaner Markup
If you’ve ever had to deal with wrapping your components in unnecessary div
elements just to satisfy Vue 2’s single-root requirement, you’re not alone. Many developers found this aspect a bit clunky. In Vue 3, this problem is solved with fragments—a feature that allows you to return multiple root elements from a component.
<!-- Vue 3 Fragment -->
<template>
<header>Welcome to My Site</header>
<footer>Contact Us</footer>
</template>
No more div
soup! This feature helps keep your markup cleaner and more semantic. Whether you’re building a complex layout with headers, footers, and sidebars, or a simple form, fragments make Vue 3 more flexible and efficient.
Tree-shaking and Smaller Bundle Sizes
Vue 3 is built with modern development standards in mind, meaning it supports tree-shaking out of the box. This allows unused code to be automatically removed from your final build, reducing your application’s bundle size. It’s a crucial feature, especially as web apps become more complex, with many dependencies. The smaller your bundle, the faster your app loads.
Vue 3 is also smaller and faster by default, thanks to this focus on optimization. The result is a progressive framework that scales effortlessly, whether you’re building a tiny widget or a massive single-page application.
Why Choose Vue 3?
Vue 3 is not just a minor update; it’s a progressive leap forward. The new Composition API offers flexibility without forcing developers to abandon the more familiar Options API. Meanwhile, the Proxy-based reactivity system improves performance, making it easier to build fast, dynamic interfaces. Features like fragment support and tree-shaking reduce bloat and enhance your application’s efficiency. With the introduction of the Composition API, you now have the flexibility to organize code in a way that feels intuitive and scalable. The Proxy-based reactivity system ensures your apps perform better, especially when handling complex data structures, while fragment support and tree-shaking offer cleaner markup and faster.
Subscribe to my newsletter
Read articles from olagunju oluwabukola directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by