Day 4 - Basic Linux Shell Scripting for DevOps Engineers

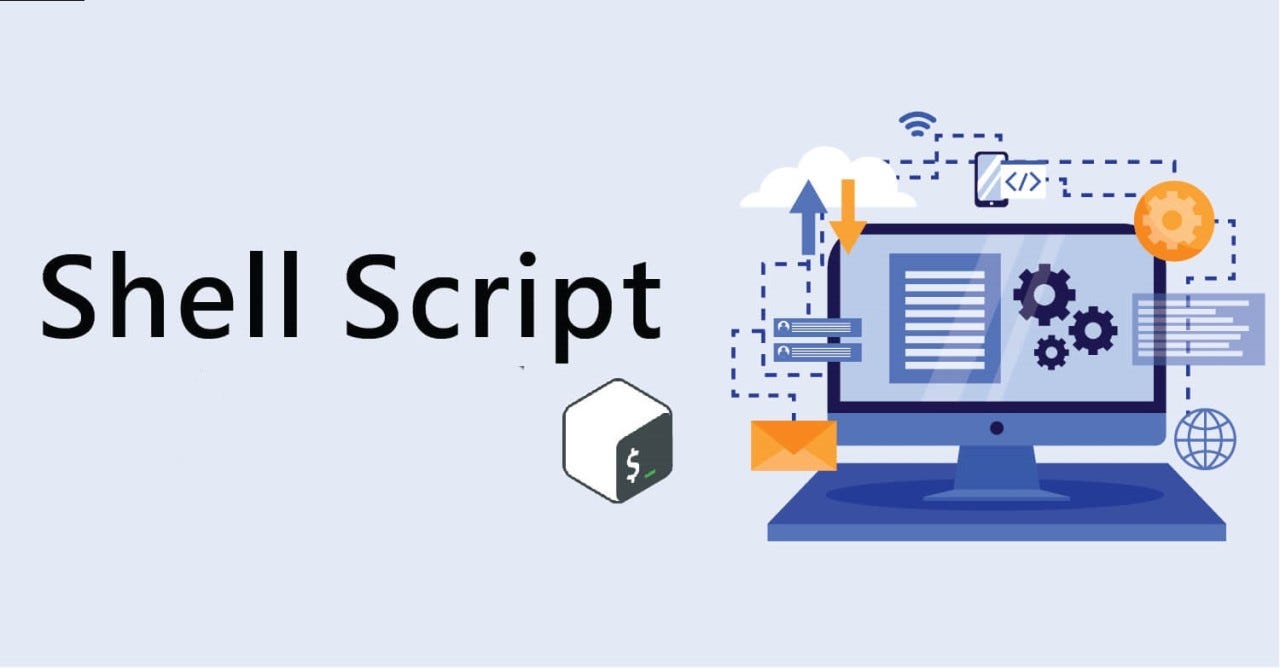
What is kernel?
A kernel is like the "boss" of your computer. It controls everything that happens inside the computer, making sure that different parts, like your programs and hardware, can work together smoothly.
Imagine your computer as a big company:
The kernel is the manager who tells the employees (the programs) how to do their job.
It decides how much time each employee gets to use the company’s tools (like the computer's CPU or memory).
It makes sure that when one employee is done using something, another can take over without crashing the system.
Without the kernel, the programs wouldn't know how to use the computer's resources like memory, processing power, and other hardware. It keeps everything organized and running efficiently behind the scenes!
What is Shell ?
Think of a Shell like the company's receptionist who communicates between you (the user) and the internal team (the computer's operating system).
Company Example:
The Receptionist (Shell):
When you walk into a company, you don't directly go to the CEO (operating system), right? Instead, you first talk to the receptionist (the shell).
You tell the receptionist what you need (e.g., "I want to meet the finance team"). The receptionist understands your request and sends it to the right department (operating system), which fulfills your request.
The Operating System (Internal Team):
Once the receptionist gets your request, they communicate with the internal team (operating system) and pass on the instructions.
The team (OS) processes the request (e.g., retrieving data, running a program) and sends the result back to the receptionist.
Shell Response:
- The receptionist (shell) then informs you about the result of your request (e.g., "The finance team is ready to meet you" or "The requested report is here").
In the Tech World:
Shell is a program that allows you to give commands to your computer's operating system, just like how you give instructions to the receptionist.
It translates your requests (commands like
ls
,cd
,mkdir
) into actions the OS understands and then shows you the results (e.g., listing files, changing directories).
It's the middle person that makes sure your requests are understood and processed correctly by the system.
What is Linux Shell Scripting ?
Linux Shell Scripting is like giving a set of instructions to your computer, written in a file, that it can follow to perform tasks automatically, much like an employee following a checklist at a company.
Example: Automating Company Tasks with Shell Scripting
Imagine a small company where employees have to perform repetitive tasks every day, like:
Sending out daily reports.
Backing up important files.
Cleaning up temporary files at the end of the day.
Instead of manually doing these tasks every day, the company can write a shell script that tells the computer to do these things automatically. This is similar to how an employee would follow a checklist to complete tasks.
How it Works in the Company Example:
Sending Daily Reports:
Every day, the company needs to send a report to the manager. A shell script can:
Gather the data from a specific folder.
Format it as a report.
Send an email with the report attached automatically using commands like
mail
orsendmail
.
Example command inside a script:
codecat /path/to/report.txt | mail -s "Daily Report" manager@company.com
Backing Up Files:
The company needs to backup important documents every evening. Instead of an employee doing it manually, a shell script can:
Copy all important files to a backup folder.
Compress them into a zip file for storage.
Send the zip to a remote server.
Example command inside a script:
codetar -czf backup_$(date +%F).tar.gz /path/to/documents
scp backup_*.tar.gz backup@backup-server:/path/to/backup
Cleaning Up Temporary Files:
At the end of the day, the company wants to delete all temporary files to free up space. A shell script can:
- Automatically find and delete temporary files from a specific folder.
Example command inside a script:
coderm -rf /path/to/temp/*
Key Points:
Automation: Just like an employee using a checklist, the computer uses a shell script to follow instructions.
Efficiency: Saves time by automating repetitive tasks.
Simplicity: Scripts are written using simple Linux commands that the computer understands.
In a real-world company, shell scripting helps automate routine tasks, making the business more efficient and freeing up employees for more valuable work.
Tasks:
1) Explain in your own words and with examples what Shell Scripting means for DevOps.
Shell scripting in DevOps is like giving the computer a list of instructions to automate repetitive tasks. Instead of manually typing commands every time, we write a script (a small program) to handle the work for us.
Why is it Important in DevOps?
In DevOps, automation is key. DevOps engineers use shell scripts to:
Automate deployments (e.g., push new code to servers).
Manage servers (e.g., restart services or clean up logs).
Monitor systems (e.g., check if a server is running smoothly).
Shell scripting helps in automating these daily operations, saving time, and reducing human errors.
Simple Company Example
Imagine you're working in a company that hosts a web app, and every day the following tasks need to be done:
Check if the web server is running.
Clean up old log files to free up space.
Take a backup of the database.
Now, instead of doing these tasks manually every day, we can create a shell script that runs these commands automatically!
2) What is `#!/bin/bash`? Can we write `#!/bin/sh` as well?
The line #!/bin/bash
(or #!/bin/sh
) at the beginning of a script is known as a shebang. It tells the operating system which interpreter to use to execute the script. Here’s a simple breakdown, along with an example relevant to a company context:
What is #!/bin/bash
?
Purpose: This line indicates that the script should be run using the Bash shell. Bash is a common shell used for scripting on Unix-like operating systems.
Location: The path
/bin/bash
specifies where the Bash interpreter is located on the system.
What about #!/bin/sh
?
Purpose: Similarly,
#!/bin/sh
indicates that the script should be executed using the sh shell (often referred to as the Bourne shell). This is a more minimal shell and often has fewer features than Bash.Usage: Using
#!/bin/sh
is sometimes preferred for compatibility, especially if the script is simple and doesn’t require Bash-specific features.
3) Write a Shell Script that prints `I will complete #90DaysOfDevOps challenge`.
4) Write a Shell Script that takes user input, input from arguments, and prints the variables.
5) Provide an example of an If-Else statement in Shell Scripting by comparing two numbers.
Subscribe to my newsletter
Read articles from Faizan Shaikh directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
