Day 19 Guide: How to Master Anonymous and Generator Functions in Python

Table of contents
- Introduction :
- Anonymous Function :
- lambda Function :
- syntax :
- Example :
- Write a program to create lambda function to find square of given number
- write a program to create a lambda function to find sum of two number
- write a lambda function to get max between two number
- Function Recursion
- example:
- GENERATOR FUNCTION :
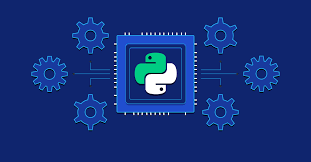
Introduction :
Welcome back to my Python journey! Yesterday, I laid the foundation with control structures and functions in python.
Today, I dove into Anonymous and Generative function, Let's explore what I learned!
Anonymous Function :
some time we can declare a function without any name, and this type of nameless function is known as anonymous function / lambda function / generator function .
the main purpose of anonymous function is just instant use .
in python function is basically two types.
. STANDARD FUNCTION
. LAMBDA FUNCTION
C++ / Java anonymous object : THE OBJECT HAVING NO ANY NAME
def standardHello () : return 'hello sir i am standard function !'
return 'hello sir i am standard function !'
lambdaHello = lambda : 'hello sir i am lambda function !'
print ( standardHello () )
print ( lambdaHello () )
lambda Function :
we can define by using lambda keyword .
syntax :
lambda argument list : expression by default it return value , do not write return key word inside lambda function .
Example :
Write a program to create lambda function to find square of given number
lambda n : n* n
print ("the square of 4 is ", s(4))
print ("the square of 5 is ", s(5))
write a program to create a lambda function to find sum of two number
s= lambda a, b :a+b
print ("sum of 10,20 are ", s(10,20))
write a lambda function to get max between two number
s= lambda a, b: a if a> b else b
print ("max of two number is", s(10,20))
Function Recursion
A function that calls itself is known as Recursive Function
example:
def Main () : #function defination
print ("i am a recursion function")
Main () #function calling itself
Main () #function calling
GENERATOR FUNCTION :
Generator is a function which is responsible to generate a sequence of values
. if a function contains yield keyword that function is known as generator function.
. the default return type of generator function it not None
. the default return type of generator function is a generator object.
. to fetch the value from generator object we have to use next () predefined function.
. if we will apply next () after fetching all values it will raise StopIteration exception.
def channelGenerator () :
subchlist = [ 'start sports', 'max', 'sony', 'nd tv', 'discovery' ]
for channel in subchlist :
yield channel
remote = channelGenerator ()
print ( next ( remote ) )
print ( next ( remote ) )
print ( next ( remote ) )
print ( next ( remote ) )
print ( next ( remote ) )
print ( next ( remote ) )
The main advantages of recursive functions are:
We can reduce length of the code and improves readability
We can solve complex problems very easily.
Q. Write a Python Function to find reverse of given number with recursion
Q. Write a Python Function to find sum of digit of given number with recursion
Q. Write a Python Function to find factorial of given number with recursion
Challenges :
Understanding various types of functions .
Handling errors.
Resources :
Official Python Documentation: Functions
W3Schools' Python Tutorial: Functions
Scaler's Python Course: Functions
Goals for Tomorrow :
Explore something more on Functions.
that is type of arguments .
Conclusion :
Day 19’s a success!
What are your favorite Python resources? Share in the comments below.
Connect with me :
GitHub: [ https://github.com/p-archana1 ]
LinkedIn : [ linkedin.com/in/archana-prusty-4aa0b827a ]
Join the conversation :
Share your own learning experiences or ask questions in the comments.
HAPPY LEARNING!!
THANK YOU!!
Subscribe to my newsletter
Read articles from Archana Prusty directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Archana Prusty
Archana Prusty
I'm Archana, pursuing Graduation in Information technology and Management. I'm a fresher with expertise in Python programming. I'm excited to apply my skills in AI/ML learning , Python, Java and web development. Looking forward to collaborating and learning from industry experts.