Python Beanie and FastAPI: Building a Data Empire

Table of contents
- The King’s Vision: Why Choose Beanie?
- Step 1: Building the Castle’s Foundation (Setting Up Beanie and FastAPI)
- Step 2: Drafting the Blueprints (Modeling with Beanie)
- Step 3: Making Royal Decrees (Creating FastAPI Endpoints)
- Step 4: Running the Kingdom Smoothly (Handling Complex Queries)
- Step 5: Expanding the Castle (Schema Migrations)
- Step 6: Royal Predictions
- Step 7: Protecting the Realm (Ensuring Data Consistency)
- Conclusion: Building Your Data Kingdom with Beanie and FastAPI
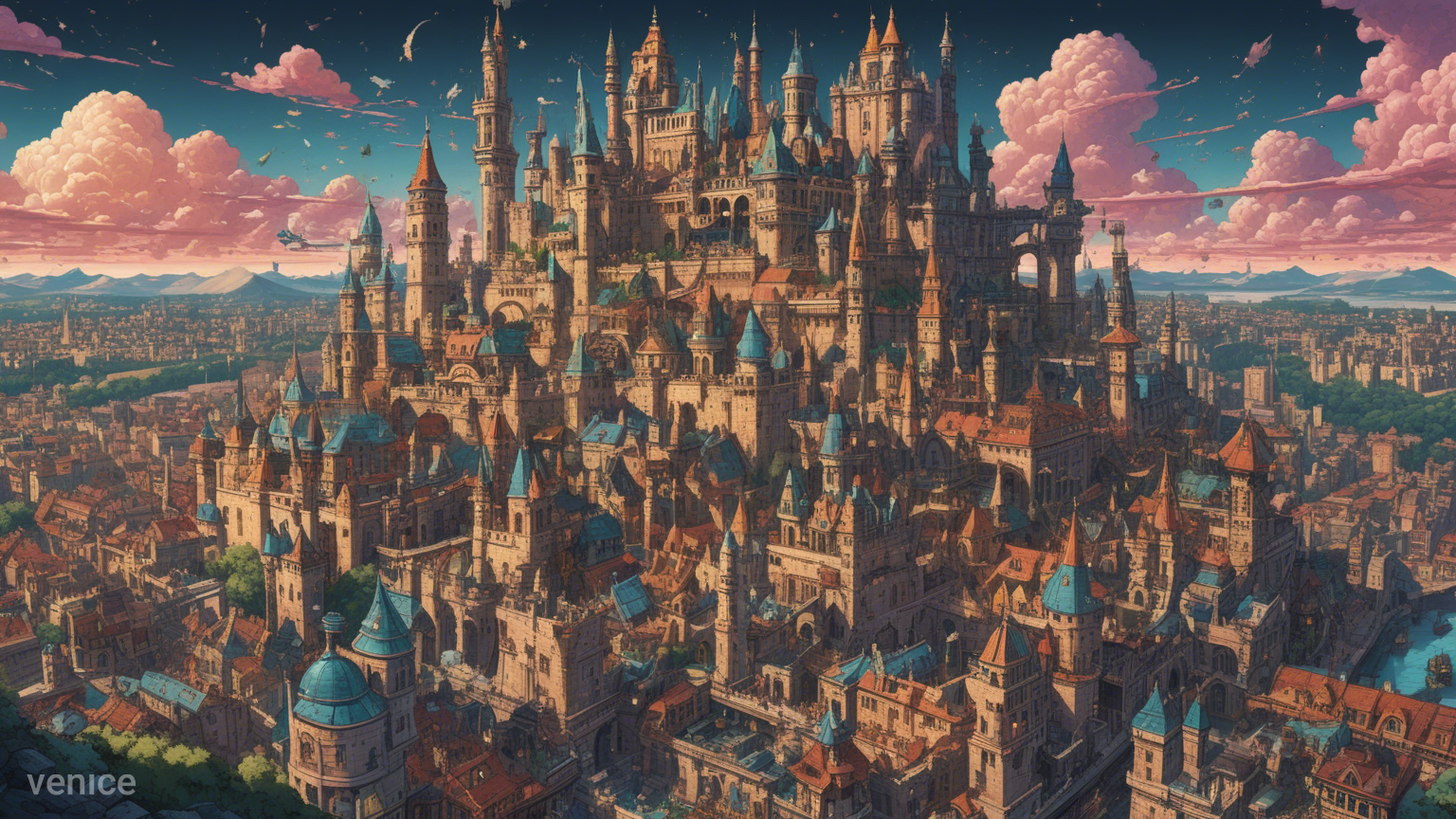
Imagine you’re the proud ruler of a kingdom. Not just any kingdom, though—a data kingdom where every brick in the castle represents a piece of information, and your citizens (users) are always hungry for efficient services. As the monarch of this empire, you need a way to handle all your resources (data), communicate with your citizens, and build systems that grow as your kingdom expands. This is where Python Beanie and FastAPI come in, turning your modest village into a thriving metropolis.
The King’s Vision: Why Choose Beanie?
Every great kingdom needs a clear vision. You wouldn’t want your kingdom’s libraries (databases) to be cluttered, your roads (data flow) blocked, or your citizens waiting in line for ages (latency). This is where Beanie shines. Beanie is your kingdom’s wise advisor, managing data effectively, without the headaches of manual bookkeeping (CRUD operations).
Why Beanie?
Asynchronous: Like a team of knights who can joust, guard, and entertain the crowd all at once, Beanie handles multiple database operations simultaneously without slowing down.
Pydantic-powered models: Beanie ensures that the data entering the kingdom follows the correct protocols (data validation), so no one sneaks in with invalid passports (bad data).
Schema migrations: Need to add a new wing to your castle? No problem! Beanie allows you to expand your data model seamlessly without disturbing the foundation.
Now that we know what Beanie can do for your kingdom, let’s start building!
Step 1: Building the Castle’s Foundation (Setting Up Beanie and FastAPI)
No kingdom can function without a castle. In our case, the castle is your backend—built using Beanie, MongoDB, and FastAPI. But before we lay the first brick, we need to install the right tools to ensure our kingdom runs like a well-oiled machine.
pip install fastapi beanie motor uvicorn
FastAPI is the front gate of your castle, allowing citizens to interact with your kingdom through API endpoints.
Beanie is the architect, keeping the data organized and accessible.
Motor is the messenger, ensuring communication between your kingdom (app) and MongoDB.
Uvicorn is the herald, broadcasting your kingdom’s greatness to the world (by running the development server).
Step 2: Drafting the Blueprints (Modeling with Beanie)
Before you start constructing new buildings in your kingdom, you need blueprints for each structure. These blueprints will ensure that all your houses (data documents) are built correctly and are easy to maintain.
In Beanie, blueprints are created using Pydantic models. Let’s design the citizens of your kingdom (user profiles):
from beanie import Document, init_beanie
from pydantic import BaseModel
from motor.motor_asyncio import AsyncIOMotorClient
from fastapi import FastAPI
import asyncio
# Define a Document model with Beanie
class Citizen(Document):
name: str
profession: str
loyalty_points: int = 0 # Every citizen starts out loyal... for now.
class Settings:
collection = "citizens"
# FastAPI app initialization
app = FastAPI()
# Database connection and Beanie initialization
async def init_db():
client = AsyncIOMotorClient("mongodb://localhost:27017")
database = client["kingdom_db"]
await init_beanie(database, document_models=[Citizen])
@app.on_event("startup")
async def on_startup():
await init_db()
Breaking It Down:
Citizen is your user profile model—a blueprint for each citizen in your kingdom. It contains attributes like
name
,profession
, andloyalty_points
.The Settings class specifies which collection these citizens will be stored in (
citizens
). Think of it as deciding whether to put your citizens in the town square or the royal archives.init_db is the magic that connects your kingdom (app) to MongoDB, ensuring all data is organized neatly.
Step 3: Making Royal Decrees (Creating FastAPI Endpoints)
Every monarch needs a way to communicate with their people. In this case, your royal decrees are API endpoints that allow users to interact with the kingdom.
Let’s create a decree for adding new citizens to the kingdom and checking on their status:
# Endpoint to create a new citizen
@app.post("/citizen")
async def create_citizen(citizen: Citizen):
await citizen.insert()
return {"message": "Citizen has been added to the kingdom!"}
# Endpoint to get a citizen’s information
@app.get("/citizen/{name}")
async def get_citizen(name: str):
citizen = await Citizen.find_one(Citizen.name == name)
if citizen:
return citizen
return {"error": "Citizen not found in the archives!"}
The Royal Analogy:
create_citizen is like opening the castle gates and letting a new citizen into the kingdom. They are added to the royal records (database).
get_citizen is like sending a scribe to check on a specific citizen’s details in the royal archives. If they exist, the scribe brings back their information; if not, the scribe returns empty-handed.
Step 4: Running the Kingdom Smoothly (Handling Complex Queries)
As your kingdom grows, you’ll need a more efficient way to manage resources and citizens. You don’t want to check each one manually, right? Let’s automate some of the royal duties by adding an endpoint to retrieve all loyal citizens.
@app.get("/citizens/loyal")
async def get_loyal_citizens():
loyal_citizens = await Citizen.find(Citizen.loyalty_points >= 50).to_list()
return loyal_citizens
The Royal Analogy:
Imagine you’re organizing a grand feast, but you only want to invite citizens who’ve been particularly loyal. Instead of checking every name in the archives, you send out an automated decree to retrieve only those who have loyalty_points
above a certain number. This is your royal banquet guest list.
Step 5: Expanding the Castle (Schema Migrations)
As your kingdom prospers, you might want to add new buildings, like a library or a blacksmith’s forge. Similarly, in Beanie, you’ll eventually need to expand your data models without breaking existing functionality.
Let’s say you want to track each citizen’s favorite tavern. Easy! Just add a new field to your Citizen model:
class Citizen(Document):
name: str
profession: str
loyalty_points: int = 0
favorite_tavern: Optional[str] = None
class Settings:
collection = "citizens"
The Royal Analogy:
This is like adding a new wing to your castle. You don’t need to demolish the whole building—just add the new feature and continue as usual. Beanie handles these “renovations” with grace, ensuring no data is lost during the process.
Step 6: Royal Predictions
As a wise ruler, you want to stay ahead of the game. How can you predict which citizens are most likely to stay loyal? By analyzing past behavior, of course! With Beanie’s querying capabilities, you can predict future trends based on historical data.
Here’s how to fetch the top 5 most loyal citizens:
@app.get("/citizens/top_loyal")
async def predict_loyalty():
loyal_citizens = await Citizen.find().sort("-loyalty_points").limit(5).to_list()
return loyal_citizens
The Royal Analogy:
Imagine your kingdom’s knights taking a vote on who’s most likely to defend the castle in a crisis. You can look at past battles (loyalty points) to predict which citizens will rise to the occasion. Similarly, this query pulls the top citizens based on loyalty, giving you a shortlist of who’s most loyal.
Step 7: Protecting the Realm (Ensuring Data Consistency)
No kingdom is complete without proper defenses! You wouldn’t let just anyone into the castle, would you? Similarly, you need to protect your data with validation and consistency checks to keep things in order.
Here’s how to validate that every new citizen has a proper profession:
class Citizen(Document):
name: str
profession: str
loyalty_points: int = 0
favorite_tavern: Optional[str] = None
@root_validator
def validate_profession(cls, values):
profession = values.get("profession")
if profession not in ["Knight", "Mage", "Peasant"]:
raise ValueError("Invalid profession!")
return values
The Royal Analogy:
This is your kingdom’s security guard. Every time someone tries to enter, the guard checks their credentials to ensure they belong. No imposters allowed! The validation ensures every new citizen has a valid profession, keeping the kingdom orderly.
Conclusion: Building Your Data Kingdom with Beanie and FastAPI
By now, you've gone from ruling over a small village to commanding a vast, data-driven kingdom—all thanks to the powers of Beanie and FastAPI. From creating blueprints (data models) to managing citizens (documents) efficiently, you’ve built a kingdom that runs like clockwork.
Just like any great kingdom, your app is scalable, flexible, and ready to grow. Beanie’s asynchronous power means that no matter how many new citizens arrive, you’re always ready to handle them—without breaking a sweat.
As a proud ruler, I’d like to share that I have developed an application that interacts with a fine-tuned model (or any other model) using Python Beanie and FastAPI. You can explore the code and its functionalities by visiting my GitHub repository.
Subscribe to my newsletter
Read articles from Ashish Sam T George directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by

Ashish Sam T George
Ashish Sam T George
👋 Hey there! I'm getwithashish, a seasoned software engineer with a passion for AI. Whether it's diving deep into neural networks or architecting scalable systems, I thrive on challenges that push the boundaries of what's possible. 💻 My journey? It's a blend of code, creativity, and coffee-fueled brainstorming sessions. I believe in not just writing SOLID code, but crafting solutions that make a difference. 🚀 Apart from my ability to turn caffeine into code, I'm your go-to guy for transforming ideas into reality. 📫 Let's connect! Whether you're looking to collaborate on AI-driven innovations or simply want to geek out over the latest tech, drop me a message.