React Day 6/40
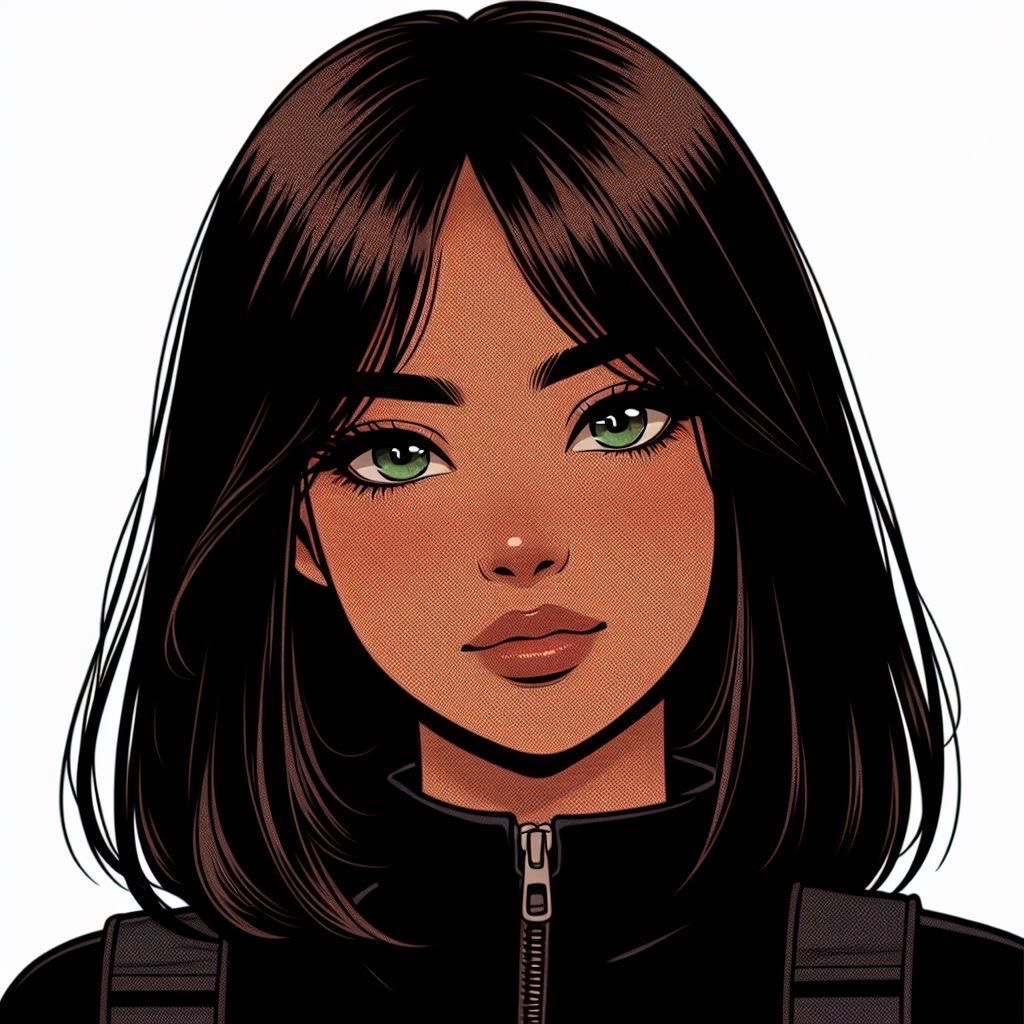
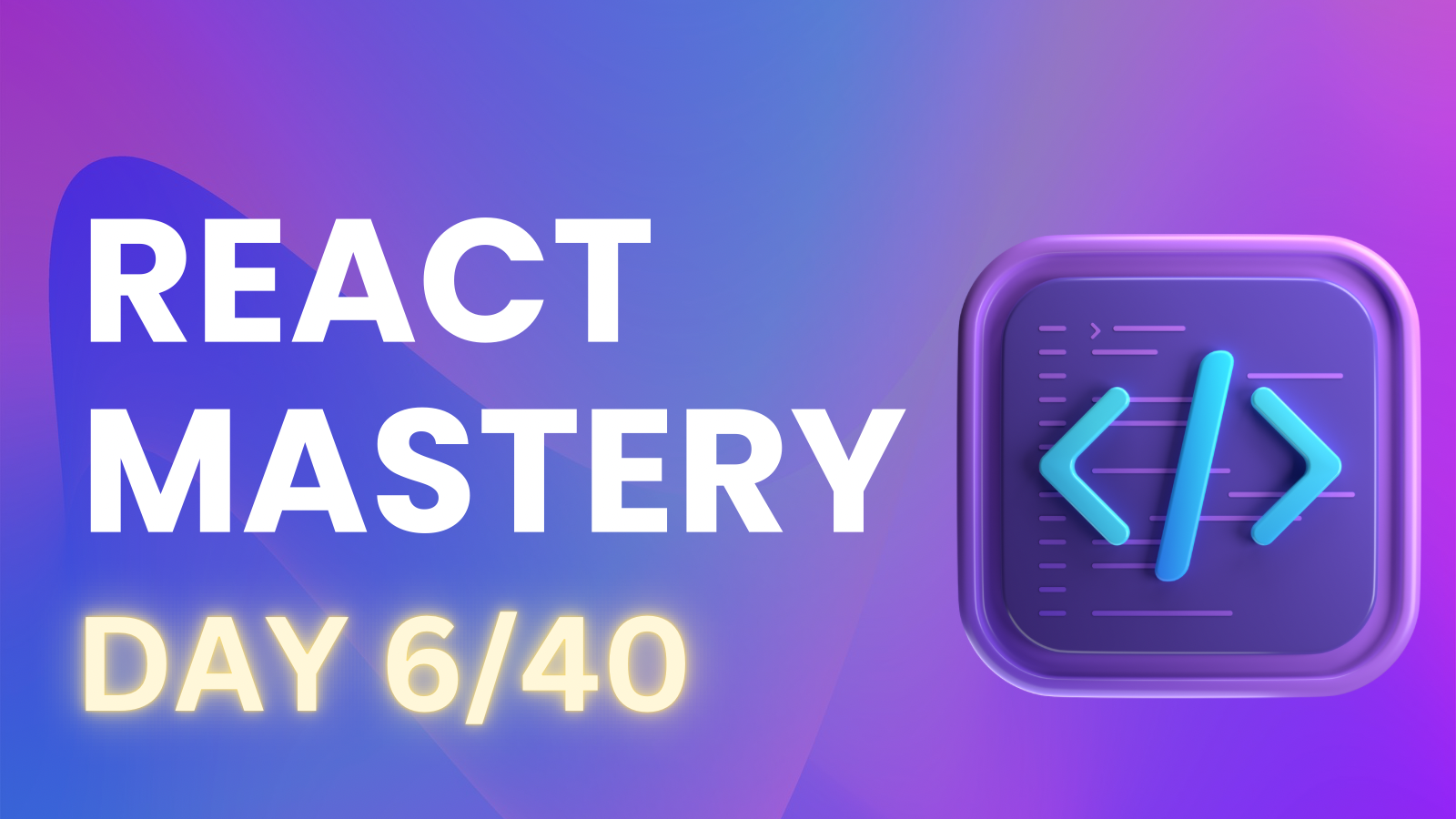
IntegratingTailwind and React props
As I continue my React journey, I've been excited to explore how to integrate Tailwind CSS into my projects. Today, I experimented with a card component from the Tailwind CSS documentation. This hands-on experience taught me valuable lessons about JSX syntax and the importance of props in making components reusable. I'm eager to share my discoveries and the steps I took to successfully incorporate Tailwind CSS into my React application.
Playing with Tailwind CSS in React
Today, I explored how to integrate Tailwind CSS with React by experimenting with a card component from the Tailwind CSS website.
To start, I copied a card component from the Tailwind CSS documentation to see how it works in a React project. However, I quickly realized there are a few key differences between regular HTML and JSX (the syntax used in React).
In JSX, only one element can be returned from a component. This means if you copy a component that contains multiple root elements, you'll run into errors. To fix this, you can wrap the elements inside an empty fragment <>...</>
or a single parent container.
Another thing to watch out for is that all tags in JSX must be properly closed. For example, in HTML, the <img>
tag doesn’t require to be closed but in JSX, you need to use <img />
with the self-closing slash. If you don't, React will throw an error when you try to render the component.
Implementing the Card
After addressing these minor adjustments, I was able to successfully integrate the Tailwind card component into my App.jsx file.
Understanding React Props
In React, props allow us to make our components reusable by passing dynamic data into them. This is one of the most powerful features of React, as it helps keep our code clean, maintainable, and flexible.
Moving from Traditional HTML/CSS/JS to React Components
In traditional web development, we keep our HTML, CSS, and JavaScript in separate files. React changes this approach by encouraging us to group our code based on functionality rather than by technology. Instead of having separate files for structure, styling, and behavior, React bundles everything related to a specific component together.
Making the Card Component Reusable
Remember the card component we worked on earlier? Now, let’s take it a step further and make it reusable. We'll create a new folder called components
and move the card code into its own component file.
Create a folder named
components
in your project directory.Inside this folder, create a file called
Card.jsx
.
Now, instead of hardcoding everything inside the card, we will pass data into it using props.
Setting Up the React Snippet Extension
If you’re using React Snippets, simply type rfce
inside the Card.jsx
file. This shortcut will generate a basic functional component template like this:
import React from 'react'
function cards() {
return (
<div>
</div>
)
}
export default cards
Using the Card Component in App.jsx
Now that we’ve created our Card
component, it's time to load and use it in the App.jsx
file. Here's how we can do it:
- Import the Card Component
In your App.jsx
file, import the Card
component like this:
import Card from './components/Card';
- Use the Card Component
To render the card component, we can simply use the <Card />
tag inside our App.jsx
file. Here’s a basic example:
import Card from './components/Card';
codefunction App() {
return (
<>
<Card />
</>
);
}
export default App;
Implementing Reusability
Now, imagine you want to use the Card
component multiple times. You can easily do this by rendering it multiple times in your code. However, each card should display different information, and this is where props come in handy.
By passing unique props to each instance of the Card
component, we can control the data displayed on each card. This allows us to use the same component structure while showing different content.We’ll explore how to do that in a minute. Stay tuned!
Here, we are using same card twice:
<Card />
<Card />
Accessing Props in the Component
Inside the Card
component, we need to access the props that are being passed. To do this, in card.jsx
, we modify the Card
function to take props
as an argument:
function Card(props){
console.log(“props”, props);
//
}
In React, props are passed to components as an object. This object contains key-value pairs where each key represents the name of a prop, and its value represents the actual data passed from the parent component.
Initially, when we render a component without passing any props, the props
object is empty. However, when we pass values to the component, the object gets populated with those key-value pairs.
Accessing Values and Passing Arrays or Objects
Now, any value that you pass into the <Card />
component as a prop will be available in the props
object inside the component. For example, if you pass a name
prop like this:
<Card name="Aaks" />
When you log props
inside the Card
component in (card.jsx) using:
console.log("props", props);
Initially, the props
object was empty, but now it contains the value you passed:
{ name: 'Aaks' }
So, you can now access props.name
to display or use the value "Aaks"
inside the component.
Can We Pass Arrays and Objects as Props?
- Passing an Array
For example, if you want to pass an array like this:
jsxCopy code<Card name="Aaks" myArr=[1, 2, 3] />
This resulted in an error.
- Passing an Object
Similarly, if you want to pass an object like this:
jsxCopy code<Card name="Aaks" myObj={name: "Aaks"} />
This too resulted in an error .
Finding the Right Way
To correctly pass an object, first define it outside the component and then use it within the Card
. For instance:
let myObj = {
username: "aaks",
age: 21
}
Now, we can use this object in our Card
component like this:
<Card name="Aaks" someObj={myObj} />
Found the correct syntax, No error YAY
Similarly, we can create an array and pass it to the <Card />
component in a manner consistent with what we've just discussed.
By using {myObj}
, instead of trying to pass myObj
directly, we follow the correct JSX syntax. This aligns with what we learned on Day 4 of our React journey, where we explored the concept of using our custom React components.
Using Props in the Card Component
However, we haven't used the name
prop in the Card
component yet. Since props
is an object, we can access it in card.jsx
by using props.name
, and we can see its value by logging it to the console.
function Card({ someObj }) {}
Now, if we simply use <h1>{someObj?.name}</h1>
, the Card
component will display "Aaks".
<h1>{someObj?.username}</h1>
Similarly, to change the button text in our card, we can use the following in App.jsx
:
<Card someObj={myObj} btnText="Click Me" />
However, we need to handle the btnText
property in the Card
component. In card.jsx
, we can define it as follows:
function Card({ someObj, btnText }) {}
Then, we can replace the button's text with {btnText}
instead of the static text that was previously used.
In case btnText
is not passed, we should set a default value. We can do this by using:
<button className="">
{btnText || "Visit Me"}
</button>
However, a better approach is to directly assign a default value in the function definition:
function Card({ someObj, btnText = "Visit Me" }) {}
This way, if btnText
is not provided, it will default to "Visit Me."
In this journey of React, I've learned to integrate Tailwind CSS with React, and learned the importance of React props. They allow me to make components reusable and dynamic. This knowledge will be essential as I build more complex applications in the future. I’m looking forward to using props to enhance my React projects further.
Subscribe to my newsletter
Read articles from Aaks directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
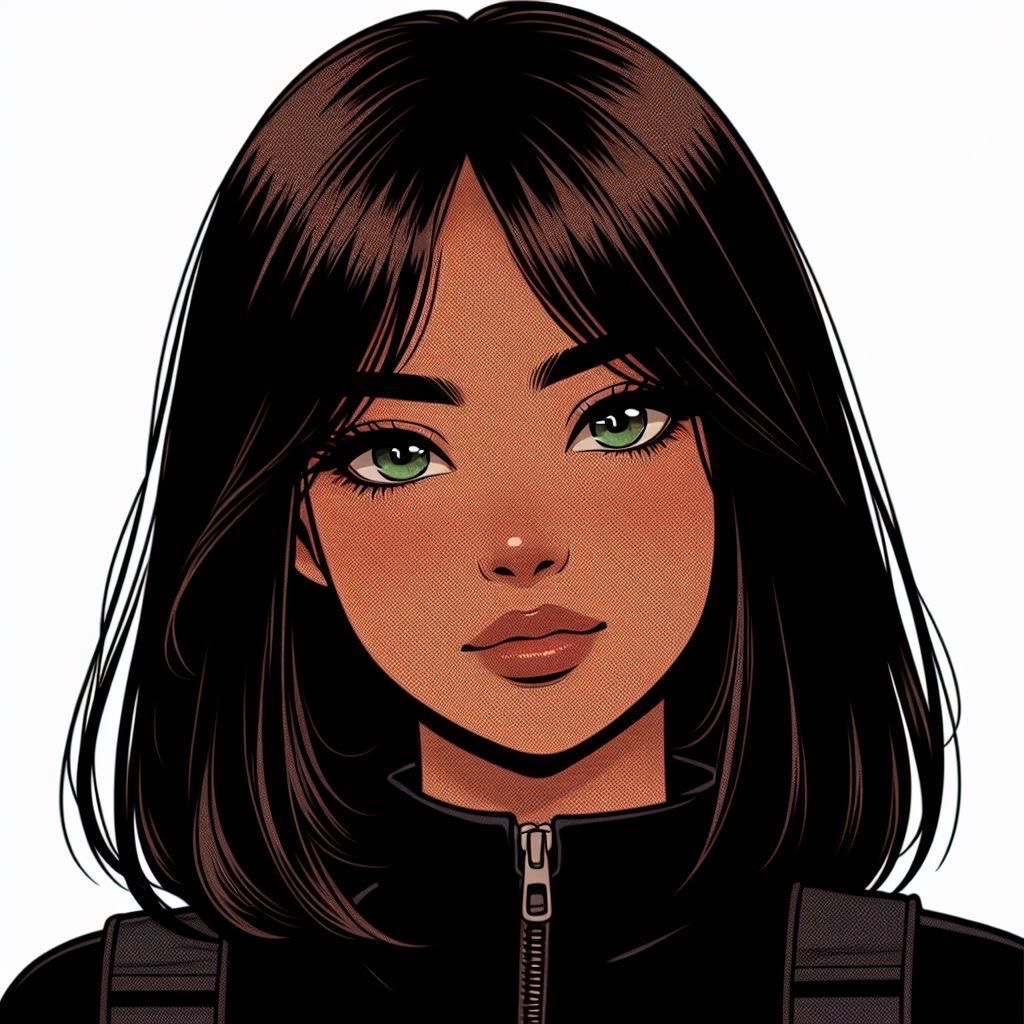