Nextjs Firebase
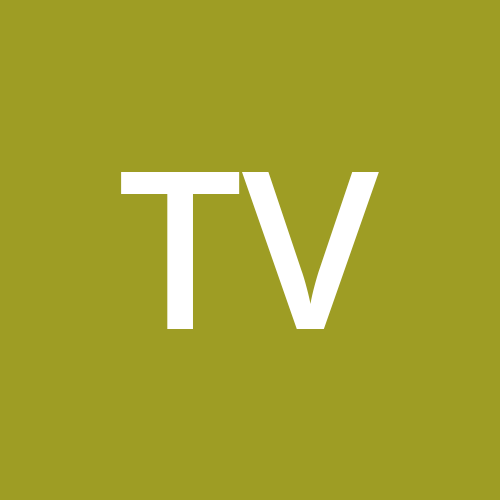
Next.js Firebase Integration: A Comprehensive Guide
When building modern web applications, combining Next.js with Firebase can offer a powerful and efficient solution. In this article, we'll explore how Next.js Firebase integration works, the benefits of using these two technologies together, and provide a step-by-step guide to set up a project.
There are pros and cons of using firebase and might describe the use of firebase as cumbersome however it has it’s advantages and is listed with a free tire as cloud storage service. and regards to nextjs, if you want to learn nextjs more i’d recommend to use ai tools like gpteachus - now let’s dig in!
Why Use Next.js with Firebase?
Next.js Firebase integration brings together the best of both worlds:
Next.js is a powerful React framework that offers features like server-side rendering (SSR), static site generation (SSG), and API routes.
Firebase is a Backend-as-a-Service (BaaS) platform that provides a variety of tools, including real-time databases, authentication, hosting, and cloud functions.
Combining Next.js Firebase allows developers to create highly scalable, full-stack applications with minimal configuration, offering seamless deployment and integration of backend features.
Step-by-Step Guide for Next.js Firebase Integration
1. Set Up a Next.js Project
Start by creating a Next.js project. You can do this by using the command:
npx create-next-app nextjs-firebase-example
cd nextjs-firebase-example
This will create a new Next.js project in the nextjs-firebase-example
directory.
2. Install Firebase SDK
Next, install the Firebase SDK to enable Next.js Firebase integration:
npm install firebase
3. Initialize Firebase in the Project
To integrate Next.js Firebase, you need to configure Firebase in your project. Create a new file called firebase.js
in the root directory and initialize Firebase with your project credentials.
First, go to the Firebase Console, create a project, and add a web app. You will get your Firebase config, which will look something like this:
const firebaseConfig = {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_PROJECT_ID.firebaseapp.com",
projectId: "YOUR_PROJECT_ID",
storageBucket: "YOUR_PROJECT_ID.appspot.com",
messagingSenderId: "YOUR_MESSAGING_SENDER_ID",
appId: "YOUR_APP_ID"
};
Now, in your firebase.js
file, add:
import { initializeApp } from 'firebase/app';
const firebaseConfig = {
apiKey: "YOUR_API_KEY",
authDomain: "YOUR_PROJECT_ID.firebaseapp.com",
projectId: "YOUR_PROJECT_ID",
storageBucket: "YOUR_PROJECT_ID.appspot.com",
messagingSenderId: "YOUR_MESSAGING_SENDER_ID",
appId: "YOUR_APP_ID"
};
// Initialize Firebase
const app = initializeApp(firebaseConfig);
export default app;
This will initialize Firebase in your Next.js Firebase app.
4. Set Up Firebase Authentication
To implement authentication in your Next.js Firebase project, you can use Firebase's Authentication service. Add the following code to firebase.js
:
import { getAuth, signInWithPopup, GoogleAuthProvider } from 'firebase/auth';
const auth = getAuth();
const provider = new GoogleAuthProvider();
export const signInWithGoogle = () => {
signInWithPopup(auth, provider)
.then((result) => {
console.log(result.user);
})
.catch((error) => {
console.error(error);
});
};
Then, in your pages/index.js
, use this function to handle Google sign-in:
import { signInWithGoogle } from '../firebase';
export default function Home() {
return (
<div>
<h1>Next.js Firebase Authentication</h1>
<button onClick={signInWithGoogle}>Sign in with Google</button>
</div>
);
}
5. Deploying Next.js with Firebase Hosting
Firebase offers a Hosting service that works perfectly for deploying your Next.js Firebase application. To deploy:
Install Firebase CLI:
npm install -g firebase-tools
Log in to Firebase:
firebase login
Initialize Firebase Hosting in your project:
firebase init hosting
Select your Firebase project, and choose the
build
folder as the public directory. When prompted, configure the app as a single-page application by answering "Yes."Build the Next.js app:
npm run build
Finally, deploy the app to Firebase Hosting:
firebase deploy
Now, your Next.js Firebase app is live and accessible via Firebase Hosting.
Benefits of Next.js Firebase Integration
Seamless SSR & SSG: With Next.js Firebase, you can leverage server-side rendering and static generation for faster page load times and improved SEO.
Scalability: Firebase's real-time database, cloud functions, and hosting services make it easy to scale your Next.js Firebase app without managing backend infrastructure.
Authentication: Firebase provides pre-built authentication services that integrate easily with your Next.js app, supporting methods like Google, Facebook, and email/password login.
Real-Time Data: Firebase's real-time database ensures that your Next.js Firebase app can update data across users and devices without needing complex websockets or backend code.
Conclusion
By integrating Next.js Firebase, you get the full power of both a frontend framework and a backend-as-a-service platform, allowing you to build modern web applications efficiently. With features like real-time data, authentication, server-side rendering, and easy deployment, Next.js Firebase integration is a great choice for scalable and secure web development.
Subscribe to my newsletter
Read articles from Turing Vang directly inside your inbox. Subscribe to the newsletter, and don't miss out.
Written by
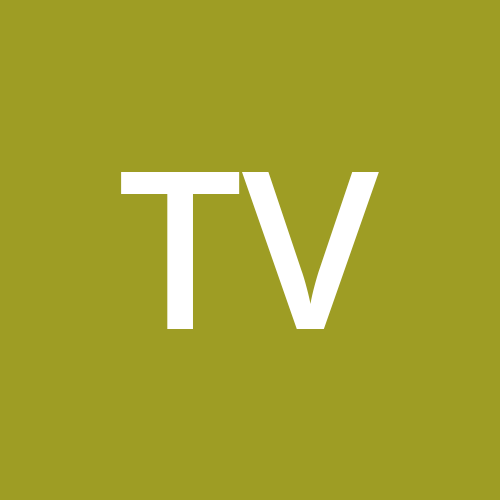